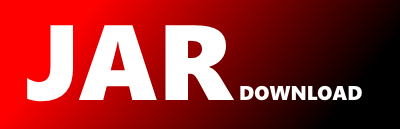
org.openapitools.client.model.SubscriptionPatchRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.openapitools.client.model.CancelSubscriptionRequest;
import org.openapitools.client.model.FlexibleBillingDocumentSettings;
import org.openapitools.client.model.PauseSubscriptionRequest;
import org.openapitools.client.model.ResumeSubscriptionRequest;
import org.openapitools.client.model.StartOn;
import org.openapitools.client.model.SubscriptionAddPlanPatchRequest;
import org.openapitools.client.model.SubscriptionRemovePlanPatchRequest;
import org.openapitools.client.model.SubscriptionRenewPatchRequest;
import org.openapitools.client.model.SubscriptionReplacePlanPatchRequest;
import org.openapitools.client.model.SubscriptionTermPatchRequest;
import org.openapitools.client.model.SubscriptionUpdatePlanPatchRequest;
import org.openapitools.jackson.nullable.JsonNullable;
import org.openapitools.client.JSON.CustomFieldAdapter;
import org.openapitools.client.JSON.NullableFieldAdapter;
/**
* SubscriptionPatchRequest
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class SubscriptionPatchRequest {
public static final String SERIALIZED_NAME_DESCRIPTION = "description";
@SerializedName(SERIALIZED_NAME_DESCRIPTION)
private String description;
public static final String SERIALIZED_NAME_CUSTOM_FIELDS = "custom_fields";
@SerializedName(SERIALIZED_NAME_CUSTOM_FIELDS)
@JsonAdapter(CustomFieldAdapter.class)
private Map customFields = null;
public static final String SERIALIZED_NAME_TERMS = "terms";
@SerializedName(SERIALIZED_NAME_TERMS)
private SubscriptionTermPatchRequest terms;
public static final String SERIALIZED_NAME_START_ON = "start_on";
@SerializedName(SERIALIZED_NAME_START_ON)
private StartOn startOn;
public static final String SERIALIZED_NAME_INVOICE_OWNER_ACCOUNT_ID = "invoice_owner_account_id";
@SerializedName(SERIALIZED_NAME_INVOICE_OWNER_ACCOUNT_ID)
private String invoiceOwnerAccountId;
public static final String SERIALIZED_NAME_INVOICE_OWNER_ACCOUNT_NUMBER = "invoice_owner_account_number";
@SerializedName(SERIALIZED_NAME_INVOICE_OWNER_ACCOUNT_NUMBER)
private String invoiceOwnerAccountNumber;
public static final String SERIALIZED_NAME_ACCOUNT_ID = "account_id";
@SerializedName(SERIALIZED_NAME_ACCOUNT_ID)
private String accountId;
public static final String SERIALIZED_NAME_ACCOUNT_NUMBER = "account_number";
@SerializedName(SERIALIZED_NAME_ACCOUNT_NUMBER)
private String accountNumber;
public static final String SERIALIZED_NAME_ADD_SUBSCRIPTION_PLANS = "add_subscription_plans";
@SerializedName(SERIALIZED_NAME_ADD_SUBSCRIPTION_PLANS)
private List addSubscriptionPlans = null;
public static final String SERIALIZED_NAME_REMOVE_SUBSCRIPTION_PLANS = "remove_subscription_plans";
@SerializedName(SERIALIZED_NAME_REMOVE_SUBSCRIPTION_PLANS)
private List removeSubscriptionPlans = null;
public static final String SERIALIZED_NAME_REPLACE_SUBSCRIPTION_PLANS = "replace_subscription_plans";
@SerializedName(SERIALIZED_NAME_REPLACE_SUBSCRIPTION_PLANS)
private List replaceSubscriptionPlans = null;
public static final String SERIALIZED_NAME_UPDATE_SUBSCRIPTION_PLANS = "update_subscription_plans";
@SerializedName(SERIALIZED_NAME_UPDATE_SUBSCRIPTION_PLANS)
private List updateSubscriptionPlans = null;
public static final String SERIALIZED_NAME_RENEW = "renew";
@SerializedName(SERIALIZED_NAME_RENEW)
private SubscriptionRenewPatchRequest renew;
public static final String SERIALIZED_NAME_RENEWALS = "renewals";
@SerializedName(SERIALIZED_NAME_RENEWALS)
private List renewals = null;
public static final String SERIALIZED_NAME_CANCEL = "cancel";
@SerializedName(SERIALIZED_NAME_CANCEL)
private CancelSubscriptionRequest cancel;
public static final String SERIALIZED_NAME_PAUSE = "pause";
@SerializedName(SERIALIZED_NAME_PAUSE)
private PauseSubscriptionRequest pause;
public static final String SERIALIZED_NAME_RESUME = "resume";
@SerializedName(SERIALIZED_NAME_RESUME)
private ResumeSubscriptionRequest resume;
public static final String SERIALIZED_NAME_BILL_TO_ID = "bill_to_id";
@SerializedName(SERIALIZED_NAME_BILL_TO_ID)
@JsonAdapter(NullableFieldAdapter.class)
private String billToId;
public static final String SERIALIZED_NAME_PAYMENT_TERMS = "payment_terms";
@SerializedName(SERIALIZED_NAME_PAYMENT_TERMS)
@JsonAdapter(NullableFieldAdapter.class)
private String paymentTerms;
public static final String SERIALIZED_NAME_BILLING_DOCUMENT_SETTINGS = "billing_document_settings";
@SerializedName(SERIALIZED_NAME_BILLING_DOCUMENT_SETTINGS)
private FlexibleBillingDocumentSettings billingDocumentSettings;
public static final String SERIALIZED_NAME_SOLD_TO_ID = "sold_to_id";
@SerializedName(SERIALIZED_NAME_SOLD_TO_ID)
@JsonAdapter(NullableFieldAdapter.class)
private String soldToId;
public static final String SERIALIZED_NAME_INVOICE_SEPARATELY = "invoice_separately";
@SerializedName(SERIALIZED_NAME_INVOICE_SEPARATELY)
private Boolean invoiceSeparately;
public static final String SERIALIZED_NAME_CHANGE_REASON = "change_reason";
@SerializedName(SERIALIZED_NAME_CHANGE_REASON)
private String changeReason;
public SubscriptionPatchRequest() {
}
public SubscriptionPatchRequest description(String description) {
this.description = description;
return this;
}
/**
* Description of the subscription.
* @return description
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Description of the subscription.")
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public SubscriptionPatchRequest customFields(Map customFields) {
this.customFields = customFields;
return this;
}
public SubscriptionPatchRequest putCustomFieldsItem(String key, String customFieldsItem) {
if (this.customFields == null) {
this.customFields = new HashMap();
}
this.customFields.put(key, customFieldsItem);
return this;
}
/**
* Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.
* @return customFields
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.")
public Map getCustomFields() {
return customFields;
}
public void setCustomFields(Map customFields) {
this.customFields = customFields;
}
public SubscriptionPatchRequest terms(SubscriptionTermPatchRequest terms) {
this.terms = terms;
return this;
}
/**
* Get terms
* @return terms
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public SubscriptionTermPatchRequest getTerms() {
return terms;
}
public void setTerms(SubscriptionTermPatchRequest terms) {
this.terms = terms;
}
public SubscriptionPatchRequest startOn(StartOn startOn) {
this.startOn = startOn;
return this;
}
/**
* Get startOn
* @return startOn
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public StartOn getStartOn() {
return startOn;
}
public void setStartOn(StartOn startOn) {
this.startOn = startOn;
}
public SubscriptionPatchRequest invoiceOwnerAccountId(String invoiceOwnerAccountId) {
this.invoiceOwnerAccountId = invoiceOwnerAccountId;
return this;
}
/**
* Identifier of the account that owns the invoice associated with this subscription.
* @return invoiceOwnerAccountId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Identifier of the account that owns the invoice associated with this subscription.")
public String getInvoiceOwnerAccountId() {
return invoiceOwnerAccountId;
}
public void setInvoiceOwnerAccountId(String invoiceOwnerAccountId) {
this.invoiceOwnerAccountId = invoiceOwnerAccountId;
}
public SubscriptionPatchRequest invoiceOwnerAccountNumber(String invoiceOwnerAccountNumber) {
this.invoiceOwnerAccountNumber = invoiceOwnerAccountNumber;
return this;
}
/**
* Identifier of the account that owns the invoice associated with this subscription.
* @return invoiceOwnerAccountNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Identifier of the account that owns the invoice associated with this subscription.")
public String getInvoiceOwnerAccountNumber() {
return invoiceOwnerAccountNumber;
}
public void setInvoiceOwnerAccountNumber(String invoiceOwnerAccountNumber) {
this.invoiceOwnerAccountNumber = invoiceOwnerAccountNumber;
}
public SubscriptionPatchRequest accountId(String accountId) {
this.accountId = accountId;
return this;
}
/**
* Identifier of the account that owns the subscription. Subscription owner account can be different from the invoice owner account.
* @return accountId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Identifier of the account that owns the subscription. Subscription owner account can be different from the invoice owner account.")
public String getAccountId() {
return accountId;
}
public void setAccountId(String accountId) {
this.accountId = accountId;
}
public SubscriptionPatchRequest accountNumber(String accountNumber) {
this.accountNumber = accountNumber;
return this;
}
/**
* Identifier of the account that owns the subscription. Subscription owner account can be different from the invoice owner account.
* @return accountNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Identifier of the account that owns the subscription. Subscription owner account can be different from the invoice owner account.")
public String getAccountNumber() {
return accountNumber;
}
public void setAccountNumber(String accountNumber) {
this.accountNumber = accountNumber;
}
public SubscriptionPatchRequest addSubscriptionPlans(List addSubscriptionPlans) {
this.addSubscriptionPlans = addSubscriptionPlans;
return this;
}
public SubscriptionPatchRequest addAddSubscriptionPlansItem(SubscriptionAddPlanPatchRequest addSubscriptionPlansItem) {
if (this.addSubscriptionPlans == null) {
this.addSubscriptionPlans = new ArrayList();
}
this.addSubscriptionPlans.add(addSubscriptionPlansItem);
return this;
}
/**
* Specify this field if you want to add one or multiple subscription plans to this subscription.
* @return addSubscriptionPlans
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Specify this field if you want to add one or multiple subscription plans to this subscription.")
public List getAddSubscriptionPlans() {
return addSubscriptionPlans;
}
public void setAddSubscriptionPlans(List addSubscriptionPlans) {
this.addSubscriptionPlans = addSubscriptionPlans;
}
public SubscriptionPatchRequest removeSubscriptionPlans(List removeSubscriptionPlans) {
this.removeSubscriptionPlans = removeSubscriptionPlans;
return this;
}
public SubscriptionPatchRequest addRemoveSubscriptionPlansItem(SubscriptionRemovePlanPatchRequest removeSubscriptionPlansItem) {
if (this.removeSubscriptionPlans == null) {
this.removeSubscriptionPlans = new ArrayList();
}
this.removeSubscriptionPlans.add(removeSubscriptionPlansItem);
return this;
}
/**
* Specify this field if you want to remove one or multiple subscription plans from this subscription.
* @return removeSubscriptionPlans
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Specify this field if you want to remove one or multiple subscription plans from this subscription.")
public List getRemoveSubscriptionPlans() {
return removeSubscriptionPlans;
}
public void setRemoveSubscriptionPlans(List removeSubscriptionPlans) {
this.removeSubscriptionPlans = removeSubscriptionPlans;
}
public SubscriptionPatchRequest replaceSubscriptionPlans(List replaceSubscriptionPlans) {
this.replaceSubscriptionPlans = replaceSubscriptionPlans;
return this;
}
public SubscriptionPatchRequest addReplaceSubscriptionPlansItem(SubscriptionReplacePlanPatchRequest replaceSubscriptionPlansItem) {
if (this.replaceSubscriptionPlans == null) {
this.replaceSubscriptionPlans = new ArrayList();
}
this.replaceSubscriptionPlans.add(replaceSubscriptionPlansItem);
return this;
}
/**
* Specify this field if you want to replace one or multiple subscription plans to this subscription. <br /> **Note**: This field is currently not supported if you have Billing - Revenue Integration enabled. When Billing - Revenue Integration is enabled, the replace subscription plan type of order action will no longer be applicable in Zuora Billing.
* @return replaceSubscriptionPlans
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Specify this field if you want to replace one or multiple subscription plans to this subscription.
**Note**: This field is currently not supported if you have Billing - Revenue Integration enabled. When Billing - Revenue Integration is enabled, the replace subscription plan type of order action will no longer be applicable in Zuora Billing. ")
public List getReplaceSubscriptionPlans() {
return replaceSubscriptionPlans;
}
public void setReplaceSubscriptionPlans(List replaceSubscriptionPlans) {
this.replaceSubscriptionPlans = replaceSubscriptionPlans;
}
public SubscriptionPatchRequest updateSubscriptionPlans(List updateSubscriptionPlans) {
this.updateSubscriptionPlans = updateSubscriptionPlans;
return this;
}
public SubscriptionPatchRequest addUpdateSubscriptionPlansItem(SubscriptionUpdatePlanPatchRequest updateSubscriptionPlansItem) {
if (this.updateSubscriptionPlans == null) {
this.updateSubscriptionPlans = new ArrayList();
}
this.updateSubscriptionPlans.add(updateSubscriptionPlansItem);
return this;
}
/**
* Get updateSubscriptionPlans
* @return updateSubscriptionPlans
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public List getUpdateSubscriptionPlans() {
return updateSubscriptionPlans;
}
public void setUpdateSubscriptionPlans(List updateSubscriptionPlans) {
this.updateSubscriptionPlans = updateSubscriptionPlans;
}
public SubscriptionPatchRequest renew(SubscriptionRenewPatchRequest renew) {
this.renew = renew;
return this;
}
/**
* Get renew
* @return renew
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public SubscriptionRenewPatchRequest getRenew() {
return renew;
}
public void setRenew(SubscriptionRenewPatchRequest renew) {
this.renew = renew;
}
public SubscriptionPatchRequest renewals(List renewals) {
this.renewals = renewals;
return this;
}
public SubscriptionPatchRequest addRenewalsItem(SubscriptionRenewPatchRequest renewalsItem) {
if (this.renewals == null) {
this.renewals = new ArrayList();
}
this.renewals.add(renewalsItem);
return this;
}
/**
* Specify this field when renewing a subscription.
* @return renewals
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Specify this field when renewing a subscription.")
public List getRenewals() {
return renewals;
}
public void setRenewals(List renewals) {
this.renewals = renewals;
}
public SubscriptionPatchRequest cancel(CancelSubscriptionRequest cancel) {
this.cancel = cancel;
return this;
}
/**
* Get cancel
* @return cancel
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public CancelSubscriptionRequest getCancel() {
return cancel;
}
public void setCancel(CancelSubscriptionRequest cancel) {
this.cancel = cancel;
}
public SubscriptionPatchRequest pause(PauseSubscriptionRequest pause) {
this.pause = pause;
return this;
}
/**
* Get pause
* @return pause
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public PauseSubscriptionRequest getPause() {
return pause;
}
public void setPause(PauseSubscriptionRequest pause) {
this.pause = pause;
}
public SubscriptionPatchRequest resume(ResumeSubscriptionRequest resume) {
this.resume = resume;
return this;
}
/**
* Get resume
* @return resume
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public ResumeSubscriptionRequest getResume() {
return resume;
}
public void setResume(ResumeSubscriptionRequest resume) {
this.resume = resume;
}
public SubscriptionPatchRequest billToId(String billToId) {
this.billToId = billToId;
return this;
}
/**
* ID of the bill-to contact.
* @return billToId
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "2c92c0f86a8dd422016a9e7a70116b0d", value = "ID of the bill-to contact.")
public String getBillToId() {
return billToId;
}
public void setBillToId(String billToId) {
this.billToId = billToId;
}
public SubscriptionPatchRequest paymentTerms(String paymentTerms) {
this.paymentTerms = paymentTerms;
return this;
}
/**
* The name of payment term associated with the invoice.
* @return paymentTerms
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The name of payment term associated with the invoice.")
public String getPaymentTerms() {
return paymentTerms;
}
public void setPaymentTerms(String paymentTerms) {
this.paymentTerms = paymentTerms;
}
public SubscriptionPatchRequest billingDocumentSettings(FlexibleBillingDocumentSettings billingDocumentSettings) {
this.billingDocumentSettings = billingDocumentSettings;
return this;
}
/**
* The billing document settings for the customer.
* @return billingDocumentSettings
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The billing document settings for the customer.")
public FlexibleBillingDocumentSettings getBillingDocumentSettings() {
return billingDocumentSettings;
}
public void setBillingDocumentSettings(FlexibleBillingDocumentSettings billingDocumentSettings) {
this.billingDocumentSettings = billingDocumentSettings;
}
public SubscriptionPatchRequest soldToId(String soldToId) {
this.soldToId = soldToId;
return this;
}
/**
* ID of the sold-to contact.
* @return soldToId
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "2c92c0f86a8dd422016a9e7a70116b0d", value = "ID of the sold-to contact.")
public String getSoldToId() {
return soldToId;
}
public void setSoldToId(String soldToId) {
this.soldToId = soldToId;
}
public SubscriptionPatchRequest invoiceSeparately(Boolean invoiceSeparately) {
this.invoiceSeparately = invoiceSeparately;
return this;
}
/**
* Separates a single subscription from other subscriptions and creates an invoice for this subscription. If the value is `true`, the subscription is billed separately from other subscriptions. If the value is `false`, the subscription is included with other subscriptions in the account invoice.
* @return invoiceSeparately
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Separates a single subscription from other subscriptions and creates an invoice for this subscription. If the value is `true`, the subscription is billed separately from other subscriptions. If the value is `false`, the subscription is included with other subscriptions in the account invoice.")
public Boolean getInvoiceSeparately() {
return invoiceSeparately;
}
public void setInvoiceSeparately(Boolean invoiceSeparately) {
this.invoiceSeparately = invoiceSeparately;
}
public SubscriptionPatchRequest changeReason(String changeReason) {
this.changeReason = changeReason;
return this;
}
/**
* A brief description of the reason for this change.
* @return changeReason
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A brief description of the reason for this change.")
public String getChangeReason() {
return changeReason;
}
public void setChangeReason(String changeReason) {
this.changeReason = changeReason;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
SubscriptionPatchRequest subscriptionPatchRequest = (SubscriptionPatchRequest) o;
return Objects.equals(this.description, subscriptionPatchRequest.description) &&
Objects.equals(this.customFields, subscriptionPatchRequest.customFields) &&
Objects.equals(this.terms, subscriptionPatchRequest.terms) &&
Objects.equals(this.startOn, subscriptionPatchRequest.startOn) &&
Objects.equals(this.invoiceOwnerAccountId, subscriptionPatchRequest.invoiceOwnerAccountId) &&
Objects.equals(this.invoiceOwnerAccountNumber, subscriptionPatchRequest.invoiceOwnerAccountNumber) &&
Objects.equals(this.accountId, subscriptionPatchRequest.accountId) &&
Objects.equals(this.accountNumber, subscriptionPatchRequest.accountNumber) &&
Objects.equals(this.addSubscriptionPlans, subscriptionPatchRequest.addSubscriptionPlans) &&
Objects.equals(this.removeSubscriptionPlans, subscriptionPatchRequest.removeSubscriptionPlans) &&
Objects.equals(this.replaceSubscriptionPlans, subscriptionPatchRequest.replaceSubscriptionPlans) &&
Objects.equals(this.updateSubscriptionPlans, subscriptionPatchRequest.updateSubscriptionPlans) &&
Objects.equals(this.renew, subscriptionPatchRequest.renew) &&
Objects.equals(this.renewals, subscriptionPatchRequest.renewals) &&
Objects.equals(this.cancel, subscriptionPatchRequest.cancel) &&
Objects.equals(this.pause, subscriptionPatchRequest.pause) &&
Objects.equals(this.resume, subscriptionPatchRequest.resume) &&
Objects.equals(this.billToId, subscriptionPatchRequest.billToId) &&
Objects.equals(this.paymentTerms, subscriptionPatchRequest.paymentTerms) &&
Objects.equals(this.billingDocumentSettings, subscriptionPatchRequest.billingDocumentSettings) &&
Objects.equals(this.soldToId, subscriptionPatchRequest.soldToId) &&
Objects.equals(this.invoiceSeparately, subscriptionPatchRequest.invoiceSeparately) &&
Objects.equals(this.changeReason, subscriptionPatchRequest.changeReason);
}
private static boolean equalsNullable(JsonNullable a, JsonNullable b) {
return a == b || (a != null && b != null && a.isPresent() && b.isPresent() && Objects.deepEquals(a.get(), b.get()));
}
@Override
public int hashCode() {
return Objects.hash(description, customFields, terms, startOn, invoiceOwnerAccountId, invoiceOwnerAccountNumber, accountId, accountNumber, addSubscriptionPlans, removeSubscriptionPlans, replaceSubscriptionPlans, updateSubscriptionPlans, renew, renewals, cancel, pause, resume, billToId, paymentTerms, billingDocumentSettings, soldToId, invoiceSeparately, changeReason);
}
private static int hashCodeNullable(JsonNullable a) {
if (a == null) {
return 1;
}
return a.isPresent() ? Arrays.deepHashCode(new Object[]{a.get()}) : 31;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class SubscriptionPatchRequest {\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" customFields: ").append(toIndentedString(customFields)).append("\n");
sb.append(" terms: ").append(toIndentedString(terms)).append("\n");
sb.append(" startOn: ").append(toIndentedString(startOn)).append("\n");
sb.append(" invoiceOwnerAccountId: ").append(toIndentedString(invoiceOwnerAccountId)).append("\n");
sb.append(" invoiceOwnerAccountNumber: ").append(toIndentedString(invoiceOwnerAccountNumber)).append("\n");
sb.append(" accountId: ").append(toIndentedString(accountId)).append("\n");
sb.append(" accountNumber: ").append(toIndentedString(accountNumber)).append("\n");
sb.append(" addSubscriptionPlans: ").append(toIndentedString(addSubscriptionPlans)).append("\n");
sb.append(" removeSubscriptionPlans: ").append(toIndentedString(removeSubscriptionPlans)).append("\n");
sb.append(" replaceSubscriptionPlans: ").append(toIndentedString(replaceSubscriptionPlans)).append("\n");
sb.append(" updateSubscriptionPlans: ").append(toIndentedString(updateSubscriptionPlans)).append("\n");
sb.append(" renew: ").append(toIndentedString(renew)).append("\n");
sb.append(" renewals: ").append(toIndentedString(renewals)).append("\n");
sb.append(" cancel: ").append(toIndentedString(cancel)).append("\n");
sb.append(" pause: ").append(toIndentedString(pause)).append("\n");
sb.append(" resume: ").append(toIndentedString(resume)).append("\n");
sb.append(" billToId: ").append(toIndentedString(billToId)).append("\n");
sb.append(" paymentTerms: ").append(toIndentedString(paymentTerms)).append("\n");
sb.append(" billingDocumentSettings: ").append(toIndentedString(billingDocumentSettings)).append("\n");
sb.append(" soldToId: ").append(toIndentedString(soldToId)).append("\n");
sb.append(" invoiceSeparately: ").append(toIndentedString(invoiceSeparately)).append("\n");
sb.append(" changeReason: ").append(toIndentedString(changeReason)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy