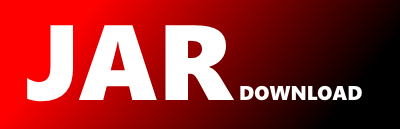
org.openapitools.client.model.SubscriptionPreviewBillingDocumentItemResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.List;
import org.openapitools.client.model.SubscriptionPreviewBillingDocumentTaxationItemResponse;
import org.openapitools.client.JSON.CustomFieldAdapter;
import org.openapitools.client.JSON.NullableFieldAdapter;
/**
* SubscriptionPreviewBillingDocumentItemResponse
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class SubscriptionPreviewBillingDocumentItemResponse {
public static final String SERIALIZED_NAME_SUBSCRIPTION_ITEM_DESCRIPTION = "subscription_item_description";
@SerializedName(SERIALIZED_NAME_SUBSCRIPTION_ITEM_DESCRIPTION)
private String subscriptionItemDescription;
public static final String SERIALIZED_NAME_SUBSCRIPTION_ITEM_NAME = "subscription_item_name";
@SerializedName(SERIALIZED_NAME_SUBSCRIPTION_ITEM_NAME)
private String subscriptionItemName;
public static final String SERIALIZED_NAME_SUBSCRIPTION_ITEM_NUMBER = "subscription_item_number";
@SerializedName(SERIALIZED_NAME_SUBSCRIPTION_ITEM_NUMBER)
private String subscriptionItemNumber;
/**
* Gets or Sets processingType
*/
@JsonAdapter(ProcessingTypeEnum.Adapter.class)
public enum ProcessingTypeEnum {
SUBSCRIPTION_ITEM("subscription_item"),
DISCOUNT("discount"),
PREPAYMENT("prepayment"),
TAX("tax"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
ProcessingTypeEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static ProcessingTypeEnum fromValue(String value) {
for (ProcessingTypeEnum b : ProcessingTypeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final ProcessingTypeEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public ProcessingTypeEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return ProcessingTypeEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_PROCESSING_TYPE = "processing_type";
@SerializedName(SERIALIZED_NAME_PROCESSING_TYPE)
private ProcessingTypeEnum processingType;
public static final String SERIALIZED_NAME_PRODUCT_NAME = "product_name";
@SerializedName(SERIALIZED_NAME_PRODUCT_NAME)
private String productName;
public static final String SERIALIZED_NAME_PRICE_ID = "price_id";
@SerializedName(SERIALIZED_NAME_PRICE_ID)
private String priceId;
public static final String SERIALIZED_NAME_SERVICE_END_DATE = "service_end_date";
@SerializedName(SERIALIZED_NAME_SERVICE_END_DATE)
private String serviceEndDate;
public static final String SERIALIZED_NAME_SERVICE_START_DATE = "service_start_date";
@SerializedName(SERIALIZED_NAME_SERVICE_START_DATE)
private String serviceStartDate;
public static final String SERIALIZED_NAME_TAX = "tax";
@SerializedName(SERIALIZED_NAME_TAX)
private BigDecimal tax;
public static final String SERIALIZED_NAME_QUANTITY = "quantity";
@SerializedName(SERIALIZED_NAME_QUANTITY)
private BigDecimal quantity;
public static final String SERIALIZED_NAME_UNIT_OF_MEASURE = "unit_of_measure";
@SerializedName(SERIALIZED_NAME_UNIT_OF_MEASURE)
private String unitOfMeasure;
public static final String SERIALIZED_NAME_SUBTOTAL = "subtotal";
@SerializedName(SERIALIZED_NAME_SUBTOTAL)
private BigDecimal subtotal;
public static final String SERIALIZED_NAME_TOTAL = "total";
@SerializedName(SERIALIZED_NAME_TOTAL)
private BigDecimal total;
public static final String SERIALIZED_NAME_TAXATION_ITEMS = "taxation_items";
@SerializedName(SERIALIZED_NAME_TAXATION_ITEMS)
private List taxationItems = null;
public SubscriptionPreviewBillingDocumentItemResponse() {
}
public SubscriptionPreviewBillingDocumentItemResponse subscriptionItemDescription(String subscriptionItemDescription) {
this.subscriptionItemDescription = subscriptionItemDescription;
return this;
}
/**
* Get subscriptionItemDescription
* @return subscriptionItemDescription
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getSubscriptionItemDescription() {
return subscriptionItemDescription;
}
public void setSubscriptionItemDescription(String subscriptionItemDescription) {
this.subscriptionItemDescription = subscriptionItemDescription;
}
public SubscriptionPreviewBillingDocumentItemResponse subscriptionItemName(String subscriptionItemName) {
this.subscriptionItemName = subscriptionItemName;
return this;
}
/**
* Get subscriptionItemName
* @return subscriptionItemName
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getSubscriptionItemName() {
return subscriptionItemName;
}
public void setSubscriptionItemName(String subscriptionItemName) {
this.subscriptionItemName = subscriptionItemName;
}
public SubscriptionPreviewBillingDocumentItemResponse subscriptionItemNumber(String subscriptionItemNumber) {
this.subscriptionItemNumber = subscriptionItemNumber;
return this;
}
/**
* Get subscriptionItemNumber
* @return subscriptionItemNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getSubscriptionItemNumber() {
return subscriptionItemNumber;
}
public void setSubscriptionItemNumber(String subscriptionItemNumber) {
this.subscriptionItemNumber = subscriptionItemNumber;
}
public SubscriptionPreviewBillingDocumentItemResponse processingType(ProcessingTypeEnum processingType) {
this.processingType = processingType;
return this;
}
/**
* Get processingType
* @return processingType
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public ProcessingTypeEnum getProcessingType() {
return processingType;
}
public void setProcessingType(ProcessingTypeEnum processingType) {
this.processingType = processingType;
}
public SubscriptionPreviewBillingDocumentItemResponse productName(String productName) {
this.productName = productName;
return this;
}
/**
* Get productName
* @return productName
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public String getProductName() {
return productName;
}
public void setProductName(String productName) {
this.productName = productName;
}
public SubscriptionPreviewBillingDocumentItemResponse priceId(String priceId) {
this.priceId = priceId;
return this;
}
/**
* The identifier of the price this billing document item is associated with.
* @return priceId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The identifier of the price this billing document item is associated with.")
public String getPriceId() {
return priceId;
}
public void setPriceId(String priceId) {
this.priceId = priceId;
}
public SubscriptionPreviewBillingDocumentItemResponse serviceEndDate(String serviceEndDate) {
this.serviceEndDate = serviceEndDate;
return this;
}
/**
* The end date of the service period associated with this billing document item. If the associated charge is a one-time fee, then this date is the date of that charge.
* @return serviceEndDate
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The end date of the service period associated with this billing document item. If the associated charge is a one-time fee, then this date is the date of that charge.")
public String getServiceEndDate() {
return serviceEndDate;
}
public void setServiceEndDate(String serviceEndDate) {
this.serviceEndDate = serviceEndDate;
}
public SubscriptionPreviewBillingDocumentItemResponse serviceStartDate(String serviceStartDate) {
this.serviceStartDate = serviceStartDate;
return this;
}
/**
* The start date of the service period associated with this billing document item. If the associated charge is a one-time fee, then this date is the date of that charge.
* @return serviceStartDate
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The start date of the service period associated with this billing document item. If the associated charge is a one-time fee, then this date is the date of that charge.")
public String getServiceStartDate() {
return serviceStartDate;
}
public void setServiceStartDate(String serviceStartDate) {
this.serviceStartDate = serviceStartDate;
}
public SubscriptionPreviewBillingDocumentItemResponse tax(BigDecimal tax) {
this.tax = tax;
return this;
}
/**
* The amount of tax applied to the billing document item.
* @return tax
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The amount of tax applied to the billing document item.")
public BigDecimal getTax() {
return tax;
}
public void setTax(BigDecimal tax) {
this.tax = tax;
}
public SubscriptionPreviewBillingDocumentItemResponse quantity(BigDecimal quantity) {
this.quantity = quantity;
return this;
}
/**
* The number of units of this item.
* @return quantity
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The number of units of this item.")
public BigDecimal getQuantity() {
return quantity;
}
public void setQuantity(BigDecimal quantity) {
this.quantity = quantity;
}
public SubscriptionPreviewBillingDocumentItemResponse unitOfMeasure(String unitOfMeasure) {
this.unitOfMeasure = unitOfMeasure;
return this;
}
/**
* Specifies the units used to measure usage.
* @return unitOfMeasure
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Specifies the units used to measure usage.")
public String getUnitOfMeasure() {
return unitOfMeasure;
}
public void setUnitOfMeasure(String unitOfMeasure) {
this.unitOfMeasure = unitOfMeasure;
}
public SubscriptionPreviewBillingDocumentItemResponse subtotal(BigDecimal subtotal) {
this.subtotal = subtotal;
return this;
}
/**
* The total amount of this billing document item exclusive of tax.
* @return subtotal
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The total amount of this billing document item exclusive of tax.")
public BigDecimal getSubtotal() {
return subtotal;
}
public void setSubtotal(BigDecimal subtotal) {
this.subtotal = subtotal;
}
public SubscriptionPreviewBillingDocumentItemResponse total(BigDecimal total) {
this.total = total;
return this;
}
/**
* The total amount of this billing document item.
* @return total
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The total amount of this billing document item.")
public BigDecimal getTotal() {
return total;
}
public void setTotal(BigDecimal total) {
this.total = total;
}
public SubscriptionPreviewBillingDocumentItemResponse taxationItems(List taxationItems) {
this.taxationItems = taxationItems;
return this;
}
public SubscriptionPreviewBillingDocumentItemResponse addTaxationItemsItem(SubscriptionPreviewBillingDocumentTaxationItemResponse taxationItemsItem) {
if (this.taxationItems == null) {
this.taxationItems = new ArrayList();
}
this.taxationItems.add(taxationItemsItem);
return this;
}
/**
* Get taxationItems
* @return taxationItems
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public List getTaxationItems() {
return taxationItems;
}
public void setTaxationItems(List taxationItems) {
this.taxationItems = taxationItems;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
SubscriptionPreviewBillingDocumentItemResponse subscriptionPreviewBillingDocumentItemResponse = (SubscriptionPreviewBillingDocumentItemResponse) o;
return Objects.equals(this.subscriptionItemDescription, subscriptionPreviewBillingDocumentItemResponse.subscriptionItemDescription) &&
Objects.equals(this.subscriptionItemName, subscriptionPreviewBillingDocumentItemResponse.subscriptionItemName) &&
Objects.equals(this.subscriptionItemNumber, subscriptionPreviewBillingDocumentItemResponse.subscriptionItemNumber) &&
Objects.equals(this.processingType, subscriptionPreviewBillingDocumentItemResponse.processingType) &&
Objects.equals(this.productName, subscriptionPreviewBillingDocumentItemResponse.productName) &&
Objects.equals(this.priceId, subscriptionPreviewBillingDocumentItemResponse.priceId) &&
Objects.equals(this.serviceEndDate, subscriptionPreviewBillingDocumentItemResponse.serviceEndDate) &&
Objects.equals(this.serviceStartDate, subscriptionPreviewBillingDocumentItemResponse.serviceStartDate) &&
Objects.equals(this.tax, subscriptionPreviewBillingDocumentItemResponse.tax) &&
Objects.equals(this.quantity, subscriptionPreviewBillingDocumentItemResponse.quantity) &&
Objects.equals(this.unitOfMeasure, subscriptionPreviewBillingDocumentItemResponse.unitOfMeasure) &&
Objects.equals(this.subtotal, subscriptionPreviewBillingDocumentItemResponse.subtotal) &&
Objects.equals(this.total, subscriptionPreviewBillingDocumentItemResponse.total) &&
Objects.equals(this.taxationItems, subscriptionPreviewBillingDocumentItemResponse.taxationItems);
}
@Override
public int hashCode() {
return Objects.hash(subscriptionItemDescription, subscriptionItemName, subscriptionItemNumber, processingType, productName, priceId, serviceEndDate, serviceStartDate, tax, quantity, unitOfMeasure, subtotal, total, taxationItems);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class SubscriptionPreviewBillingDocumentItemResponse {\n");
sb.append(" subscriptionItemDescription: ").append(toIndentedString(subscriptionItemDescription)).append("\n");
sb.append(" subscriptionItemName: ").append(toIndentedString(subscriptionItemName)).append("\n");
sb.append(" subscriptionItemNumber: ").append(toIndentedString(subscriptionItemNumber)).append("\n");
sb.append(" processingType: ").append(toIndentedString(processingType)).append("\n");
sb.append(" productName: ").append(toIndentedString(productName)).append("\n");
sb.append(" priceId: ").append(toIndentedString(priceId)).append("\n");
sb.append(" serviceEndDate: ").append(toIndentedString(serviceEndDate)).append("\n");
sb.append(" serviceStartDate: ").append(toIndentedString(serviceStartDate)).append("\n");
sb.append(" tax: ").append(toIndentedString(tax)).append("\n");
sb.append(" quantity: ").append(toIndentedString(quantity)).append("\n");
sb.append(" unitOfMeasure: ").append(toIndentedString(unitOfMeasure)).append("\n");
sb.append(" subtotal: ").append(toIndentedString(subtotal)).append("\n");
sb.append(" total: ").append(toIndentedString(total)).append("\n");
sb.append(" taxationItems: ").append(toIndentedString(taxationItems)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy