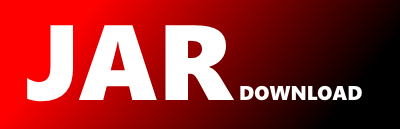
org.openapitools.client.model.SubscriptionPreviewExistingRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.openapitools.client.model.SubscriptionAddPlanPatchRequest;
import org.openapitools.client.model.SubscriptionPreviewAccountRequest;
import org.openapitools.client.model.SubscriptionRemovePlanPatchRequest;
import org.openapitools.client.model.SubscriptionReplacePlanPatchRequest;
import org.openapitools.client.model.SubscriptionUpdatePlanPatchRequest;
import org.threeten.bp.LocalDate;
import org.openapitools.client.JSON.CustomFieldAdapter;
import org.openapitools.client.JSON.NullableFieldAdapter;
/**
* SubscriptionPreviewExistingRequest
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class SubscriptionPreviewExistingRequest {
public static final String SERIALIZED_NAME_CUSTOM_FIELDS = "custom_fields";
@SerializedName(SERIALIZED_NAME_CUSTOM_FIELDS)
@JsonAdapter(CustomFieldAdapter.class)
private Map customFields = null;
public static final String SERIALIZED_NAME_DESCRIPTION = "description";
@SerializedName(SERIALIZED_NAME_DESCRIPTION)
private String description;
public static final String SERIALIZED_NAME_ACCOUNT_ID = "account_id";
@SerializedName(SERIALIZED_NAME_ACCOUNT_ID)
private String accountId;
public static final String SERIALIZED_NAME_ACCOUNT_NUMBER = "account_number";
@SerializedName(SERIALIZED_NAME_ACCOUNT_NUMBER)
private String accountNumber;
public static final String SERIALIZED_NAME_ACCOUNT_DATA = "account_data";
@SerializedName(SERIALIZED_NAME_ACCOUNT_DATA)
private SubscriptionPreviewAccountRequest accountData;
public static final String SERIALIZED_NAME_NUMBER_OF_PERIODS = "number_of_periods";
@SerializedName(SERIALIZED_NAME_NUMBER_OF_PERIODS)
private Integer numberOfPeriods;
public static final String SERIALIZED_NAME_TERM_END = "term_end";
@SerializedName(SERIALIZED_NAME_TERM_END)
private Boolean termEnd;
/**
* Gets or Sets metrics
*/
@JsonAdapter(MetricsEnum.Adapter.class)
public enum MetricsEnum {
BILLING_DOCUMENTS("billing_documents"),
DELTA_METRICS("delta_metrics"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
MetricsEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static MetricsEnum fromValue(String value) {
for (MetricsEnum b : MetricsEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final MetricsEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public MetricsEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return MetricsEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_METRICS = "metrics";
@SerializedName(SERIALIZED_NAME_METRICS)
private List metrics = null;
public static final String SERIALIZED_NAME_END_DATE = "end_date";
@SerializedName(SERIALIZED_NAME_END_DATE)
private LocalDate endDate;
public static final String SERIALIZED_NAME_ADD_SUBSCRIPTION_PLANS = "add_subscription_plans";
@SerializedName(SERIALIZED_NAME_ADD_SUBSCRIPTION_PLANS)
private List addSubscriptionPlans = null;
public static final String SERIALIZED_NAME_REPLACE_SUBSCRIPTION_PLANS = "replace_subscription_plans";
@SerializedName(SERIALIZED_NAME_REPLACE_SUBSCRIPTION_PLANS)
private List replaceSubscriptionPlans = null;
public static final String SERIALIZED_NAME_UPDATE_SUBSCRIPTION_PLANS = "update_subscription_plans";
@SerializedName(SERIALIZED_NAME_UPDATE_SUBSCRIPTION_PLANS)
private List updateSubscriptionPlans = null;
public static final String SERIALIZED_NAME_REMOVE_SUBSCRIPTION_PLANS = "remove_subscription_plans";
@SerializedName(SERIALIZED_NAME_REMOVE_SUBSCRIPTION_PLANS)
private List removeSubscriptionPlans = null;
public SubscriptionPreviewExistingRequest() {
}
public SubscriptionPreviewExistingRequest customFields(Map customFields) {
this.customFields = customFields;
return this;
}
public SubscriptionPreviewExistingRequest putCustomFieldsItem(String key, String customFieldsItem) {
if (this.customFields == null) {
this.customFields = new HashMap();
}
this.customFields.put(key, customFieldsItem);
return this;
}
/**
* Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.
* @return customFields
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.")
public Map getCustomFields() {
return customFields;
}
public void setCustomFields(Map customFields) {
this.customFields = customFields;
}
public SubscriptionPreviewExistingRequest description(String description) {
this.description = description;
return this;
}
/**
* Description of the subscription.
* @return description
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Description of the subscription.")
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public SubscriptionPreviewExistingRequest accountId(String accountId) {
this.accountId = accountId;
return this;
}
/**
* Identifier of the account that owns the subscription. Subscription owner account can be different from the invoice owner account.
* @return accountId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Identifier of the account that owns the subscription. Subscription owner account can be different from the invoice owner account.")
public String getAccountId() {
return accountId;
}
public void setAccountId(String accountId) {
this.accountId = accountId;
}
public SubscriptionPreviewExistingRequest accountNumber(String accountNumber) {
this.accountNumber = accountNumber;
return this;
}
/**
* Identifier of the account that owns the subscription. Subscription owner account can be different from the invoice owner account.
* @return accountNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Identifier of the account that owns the subscription. Subscription owner account can be different from the invoice owner account.")
public String getAccountNumber() {
return accountNumber;
}
public void setAccountNumber(String accountNumber) {
this.accountNumber = accountNumber;
}
public SubscriptionPreviewExistingRequest accountData(SubscriptionPreviewAccountRequest accountData) {
this.accountData = accountData;
return this;
}
/**
* Get accountData
* @return accountData
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public SubscriptionPreviewAccountRequest getAccountData() {
return accountData;
}
public void setAccountData(SubscriptionPreviewAccountRequest accountData) {
this.accountData = accountData;
}
public SubscriptionPreviewExistingRequest numberOfPeriods(Integer numberOfPeriods) {
this.numberOfPeriods = numberOfPeriods;
return this;
}
/**
* Specifies how many billing periods you want to preview.
* @return numberOfPeriods
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Specifies how many billing periods you want to preview.")
public Integer getNumberOfPeriods() {
return numberOfPeriods;
}
public void setNumberOfPeriods(Integer numberOfPeriods) {
this.numberOfPeriods = numberOfPeriods;
}
public SubscriptionPreviewExistingRequest termEnd(Boolean termEnd) {
this.termEnd = termEnd;
return this;
}
/**
* Indicates whether to preview the subscription till the end of the current term.
* @return termEnd
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Indicates whether to preview the subscription till the end of the current term.")
public Boolean getTermEnd() {
return termEnd;
}
public void setTermEnd(Boolean termEnd) {
this.termEnd = termEnd;
}
public SubscriptionPreviewExistingRequest metrics(List metrics) {
this.metrics = metrics;
return this;
}
public SubscriptionPreviewExistingRequest addMetricsItem(MetricsEnum metricsItem) {
if (this.metrics == null) {
this.metrics = new ArrayList();
}
this.metrics.add(metricsItem);
return this;
}
/**
* Specifies the metrics you want to preview. You can preview metrics of billing documents, the order delta metrics, or both.
* @return metrics
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Specifies the metrics you want to preview. You can preview metrics of billing documents, the order delta metrics, or both.")
public List getMetrics() {
return metrics;
}
public void setMetrics(List metrics) {
this.metrics = metrics;
}
public SubscriptionPreviewExistingRequest endDate(LocalDate endDate) {
this.endDate = endDate;
return this;
}
/**
* End date of the period for which you want to preview the subscription
* @return endDate
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "End date of the period for which you want to preview the subscription")
public LocalDate getEndDate() {
return endDate;
}
public void setEndDate(LocalDate endDate) {
this.endDate = endDate;
}
public SubscriptionPreviewExistingRequest addSubscriptionPlans(List addSubscriptionPlans) {
this.addSubscriptionPlans = addSubscriptionPlans;
return this;
}
public SubscriptionPreviewExistingRequest addAddSubscriptionPlansItem(SubscriptionAddPlanPatchRequest addSubscriptionPlansItem) {
if (this.addSubscriptionPlans == null) {
this.addSubscriptionPlans = new ArrayList();
}
this.addSubscriptionPlans.add(addSubscriptionPlansItem);
return this;
}
/**
* Specify this field if you want to add one or multiple subscription plans to this subscription.
* @return addSubscriptionPlans
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Specify this field if you want to add one or multiple subscription plans to this subscription.")
public List getAddSubscriptionPlans() {
return addSubscriptionPlans;
}
public void setAddSubscriptionPlans(List addSubscriptionPlans) {
this.addSubscriptionPlans = addSubscriptionPlans;
}
public SubscriptionPreviewExistingRequest replaceSubscriptionPlans(List replaceSubscriptionPlans) {
this.replaceSubscriptionPlans = replaceSubscriptionPlans;
return this;
}
public SubscriptionPreviewExistingRequest addReplaceSubscriptionPlansItem(SubscriptionReplacePlanPatchRequest replaceSubscriptionPlansItem) {
if (this.replaceSubscriptionPlans == null) {
this.replaceSubscriptionPlans = new ArrayList();
}
this.replaceSubscriptionPlans.add(replaceSubscriptionPlansItem);
return this;
}
/**
* Specify this field if you want to replace one or multiple subscription plans to this subscription. <br /> **Note**: This field is currently not supported if you have Billing - Revenue Integration enabled. When Billing - Revenue Integration is enabled, the replace subscription plan type of order action will no longer be applicable in Zuora Billing.
* @return replaceSubscriptionPlans
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Specify this field if you want to replace one or multiple subscription plans to this subscription.
**Note**: This field is currently not supported if you have Billing - Revenue Integration enabled. When Billing - Revenue Integration is enabled, the replace subscription plan type of order action will no longer be applicable in Zuora Billing. ")
public List getReplaceSubscriptionPlans() {
return replaceSubscriptionPlans;
}
public void setReplaceSubscriptionPlans(List replaceSubscriptionPlans) {
this.replaceSubscriptionPlans = replaceSubscriptionPlans;
}
public SubscriptionPreviewExistingRequest updateSubscriptionPlans(List updateSubscriptionPlans) {
this.updateSubscriptionPlans = updateSubscriptionPlans;
return this;
}
public SubscriptionPreviewExistingRequest addUpdateSubscriptionPlansItem(SubscriptionUpdatePlanPatchRequest updateSubscriptionPlansItem) {
if (this.updateSubscriptionPlans == null) {
this.updateSubscriptionPlans = new ArrayList();
}
this.updateSubscriptionPlans.add(updateSubscriptionPlansItem);
return this;
}
/**
* Get updateSubscriptionPlans
* @return updateSubscriptionPlans
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public List getUpdateSubscriptionPlans() {
return updateSubscriptionPlans;
}
public void setUpdateSubscriptionPlans(List updateSubscriptionPlans) {
this.updateSubscriptionPlans = updateSubscriptionPlans;
}
public SubscriptionPreviewExistingRequest removeSubscriptionPlans(List removeSubscriptionPlans) {
this.removeSubscriptionPlans = removeSubscriptionPlans;
return this;
}
public SubscriptionPreviewExistingRequest addRemoveSubscriptionPlansItem(SubscriptionRemovePlanPatchRequest removeSubscriptionPlansItem) {
if (this.removeSubscriptionPlans == null) {
this.removeSubscriptionPlans = new ArrayList();
}
this.removeSubscriptionPlans.add(removeSubscriptionPlansItem);
return this;
}
/**
* Specify this field if you want to remove one or multiple subscription plans from this subscription.
* @return removeSubscriptionPlans
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Specify this field if you want to remove one or multiple subscription plans from this subscription.")
public List getRemoveSubscriptionPlans() {
return removeSubscriptionPlans;
}
public void setRemoveSubscriptionPlans(List removeSubscriptionPlans) {
this.removeSubscriptionPlans = removeSubscriptionPlans;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
SubscriptionPreviewExistingRequest subscriptionPreviewExistingRequest = (SubscriptionPreviewExistingRequest) o;
return Objects.equals(this.customFields, subscriptionPreviewExistingRequest.customFields) &&
Objects.equals(this.description, subscriptionPreviewExistingRequest.description) &&
Objects.equals(this.accountId, subscriptionPreviewExistingRequest.accountId) &&
Objects.equals(this.accountNumber, subscriptionPreviewExistingRequest.accountNumber) &&
Objects.equals(this.accountData, subscriptionPreviewExistingRequest.accountData) &&
Objects.equals(this.numberOfPeriods, subscriptionPreviewExistingRequest.numberOfPeriods) &&
Objects.equals(this.termEnd, subscriptionPreviewExistingRequest.termEnd) &&
Objects.equals(this.metrics, subscriptionPreviewExistingRequest.metrics) &&
Objects.equals(this.endDate, subscriptionPreviewExistingRequest.endDate) &&
Objects.equals(this.addSubscriptionPlans, subscriptionPreviewExistingRequest.addSubscriptionPlans) &&
Objects.equals(this.replaceSubscriptionPlans, subscriptionPreviewExistingRequest.replaceSubscriptionPlans) &&
Objects.equals(this.updateSubscriptionPlans, subscriptionPreviewExistingRequest.updateSubscriptionPlans) &&
Objects.equals(this.removeSubscriptionPlans, subscriptionPreviewExistingRequest.removeSubscriptionPlans);
}
@Override
public int hashCode() {
return Objects.hash(customFields, description, accountId, accountNumber, accountData, numberOfPeriods, termEnd, metrics, endDate, addSubscriptionPlans, replaceSubscriptionPlans, updateSubscriptionPlans, removeSubscriptionPlans);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class SubscriptionPreviewExistingRequest {\n");
sb.append(" customFields: ").append(toIndentedString(customFields)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" accountId: ").append(toIndentedString(accountId)).append("\n");
sb.append(" accountNumber: ").append(toIndentedString(accountNumber)).append("\n");
sb.append(" accountData: ").append(toIndentedString(accountData)).append("\n");
sb.append(" numberOfPeriods: ").append(toIndentedString(numberOfPeriods)).append("\n");
sb.append(" termEnd: ").append(toIndentedString(termEnd)).append("\n");
sb.append(" metrics: ").append(toIndentedString(metrics)).append("\n");
sb.append(" endDate: ").append(toIndentedString(endDate)).append("\n");
sb.append(" addSubscriptionPlans: ").append(toIndentedString(addSubscriptionPlans)).append("\n");
sb.append(" replaceSubscriptionPlans: ").append(toIndentedString(replaceSubscriptionPlans)).append("\n");
sb.append(" updateSubscriptionPlans: ").append(toIndentedString(updateSubscriptionPlans)).append("\n");
sb.append(" removeSubscriptionPlans: ").append(toIndentedString(removeSubscriptionPlans)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy