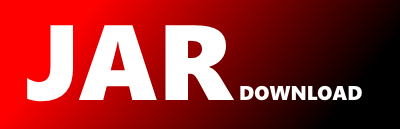
org.openapitools.client.model.SubscriptionPreviewRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.openapitools.client.model.AccountCreateRequest;
import org.openapitools.client.model.Contact;
import org.openapitools.client.model.FlexibleBillingDocumentSettings;
import org.openapitools.client.model.ProcessingOptions;
import org.openapitools.client.model.StartOn;
import org.openapitools.client.model.SubscriptionPlanCreateRequest;
import org.openapitools.client.model.SubscriptionPreviewAccountRequest;
import org.openapitools.client.model.Term;
import org.openapitools.jackson.nullable.JsonNullable;
import org.threeten.bp.LocalDate;
import org.openapitools.client.JSON.CustomFieldAdapter;
import org.openapitools.client.JSON.NullableFieldAdapter;
/**
* SubscriptionPreviewRequest
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class SubscriptionPreviewRequest {
public static final String SERIALIZED_NAME_INVOICE_OWNER_ACCOUNT_ID = "invoice_owner_account_id";
@SerializedName(SERIALIZED_NAME_INVOICE_OWNER_ACCOUNT_ID)
private String invoiceOwnerAccountId;
public static final String SERIALIZED_NAME_INVOICE_OWNER_ACCOUNT_NUMBER = "invoice_owner_account_number";
@SerializedName(SERIALIZED_NAME_INVOICE_OWNER_ACCOUNT_NUMBER)
private String invoiceOwnerAccountNumber;
public static final String SERIALIZED_NAME_INVOICE_OWNER_ACCOUNT_DATA = "invoice_owner_account_data";
@SerializedName(SERIALIZED_NAME_INVOICE_OWNER_ACCOUNT_DATA)
private AccountCreateRequest invoiceOwnerAccountData;
public static final String SERIALIZED_NAME_ACCOUNT_ID = "account_id";
@SerializedName(SERIALIZED_NAME_ACCOUNT_ID)
private String accountId;
public static final String SERIALIZED_NAME_ACCOUNT_NUMBER = "account_number";
@SerializedName(SERIALIZED_NAME_ACCOUNT_NUMBER)
private String accountNumber;
public static final String SERIALIZED_NAME_ACCOUNT_DATA = "account_data";
@SerializedName(SERIALIZED_NAME_ACCOUNT_DATA)
private SubscriptionPreviewAccountRequest accountData;
public static final String SERIALIZED_NAME_AUTO_RENEW = "auto_renew";
@SerializedName(SERIALIZED_NAME_AUTO_RENEW)
private Boolean autoRenew;
public static final String SERIALIZED_NAME_SUBSCRIPTION_NUMBER = "subscription_number";
@SerializedName(SERIALIZED_NAME_SUBSCRIPTION_NUMBER)
private String subscriptionNumber;
public static final String SERIALIZED_NAME_INITIAL_TERM = "initial_term";
@SerializedName(SERIALIZED_NAME_INITIAL_TERM)
private Term initialTerm;
public static final String SERIALIZED_NAME_RENEWAL_TERM = "renewal_term";
@SerializedName(SERIALIZED_NAME_RENEWAL_TERM)
private Term renewalTerm;
public static final String SERIALIZED_NAME_START_ON = "start_on";
@SerializedName(SERIALIZED_NAME_START_ON)
private StartOn startOn;
public static final String SERIALIZED_NAME_DESCRIPTION = "description";
@SerializedName(SERIALIZED_NAME_DESCRIPTION)
private String description;
public static final String SERIALIZED_NAME_INVOICE_SEPARATELY = "invoice_separately";
@SerializedName(SERIALIZED_NAME_INVOICE_SEPARATELY)
private Boolean invoiceSeparately;
public static final String SERIALIZED_NAME_PROCESSING_OPTIONS = "processing_options";
@SerializedName(SERIALIZED_NAME_PROCESSING_OPTIONS)
private ProcessingOptions processingOptions;
public static final String SERIALIZED_NAME_CUSTOM_FIELDS = "custom_fields";
@SerializedName(SERIALIZED_NAME_CUSTOM_FIELDS)
@JsonAdapter(CustomFieldAdapter.class)
private Map customFields = null;
public static final String SERIALIZED_NAME_SUBSCRIPTION_PLANS = "subscription_plans";
@SerializedName(SERIALIZED_NAME_SUBSCRIPTION_PLANS)
private List subscriptionPlans = null;
public static final String SERIALIZED_NAME_BILL_TO_ID = "bill_to_id";
@SerializedName(SERIALIZED_NAME_BILL_TO_ID)
@JsonAdapter(NullableFieldAdapter.class)
private String billToId;
public static final String SERIALIZED_NAME_PAYMENT_TERMS = "payment_terms";
@SerializedName(SERIALIZED_NAME_PAYMENT_TERMS)
@JsonAdapter(NullableFieldAdapter.class)
private String paymentTerms;
public static final String SERIALIZED_NAME_BILL_TO = "bill_to";
@SerializedName(SERIALIZED_NAME_BILL_TO)
private Contact billTo;
public static final String SERIALIZED_NAME_BILLING_DOCUMENT_SETTINGS = "billing_document_settings";
@SerializedName(SERIALIZED_NAME_BILLING_DOCUMENT_SETTINGS)
private FlexibleBillingDocumentSettings billingDocumentSettings;
public static final String SERIALIZED_NAME_SOLD_TO_ID = "sold_to_id";
@SerializedName(SERIALIZED_NAME_SOLD_TO_ID)
@JsonAdapter(NullableFieldAdapter.class)
private String soldToId;
public static final String SERIALIZED_NAME_SOLD_TO = "sold_to";
@SerializedName(SERIALIZED_NAME_SOLD_TO)
private Contact soldTo;
public static final String SERIALIZED_NAME_CURRENCY = "currency";
@SerializedName(SERIALIZED_NAME_CURRENCY)
private String currency;
public static final String SERIALIZED_NAME_CHANGE_REASON = "change_reason";
@SerializedName(SERIALIZED_NAME_CHANGE_REASON)
private String changeReason;
public static final String SERIALIZED_NAME_NUMBER_OF_PERIODS = "number_of_periods";
@SerializedName(SERIALIZED_NAME_NUMBER_OF_PERIODS)
private Integer numberOfPeriods;
public static final String SERIALIZED_NAME_TERM_END = "term_end";
@SerializedName(SERIALIZED_NAME_TERM_END)
private Boolean termEnd;
/**
* Gets or Sets metrics
*/
@JsonAdapter(MetricsEnum.Adapter.class)
public enum MetricsEnum {
BILLING_DOCUMENTS("billing_documents"),
DELTA_METRICS("delta_metrics"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
MetricsEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static MetricsEnum fromValue(String value) {
for (MetricsEnum b : MetricsEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final MetricsEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public MetricsEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return MetricsEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_METRICS = "metrics";
@SerializedName(SERIALIZED_NAME_METRICS)
private List metrics = null;
public static final String SERIALIZED_NAME_END_DATE = "end_date";
@SerializedName(SERIALIZED_NAME_END_DATE)
private LocalDate endDate;
public SubscriptionPreviewRequest() {
}
public SubscriptionPreviewRequest(
Contact billTo,
Contact soldTo
) {
this();
this.billTo = billTo;
this.soldTo = soldTo;
}
public SubscriptionPreviewRequest invoiceOwnerAccountId(String invoiceOwnerAccountId) {
this.invoiceOwnerAccountId = invoiceOwnerAccountId;
return this;
}
/**
* Identifier of the account that owns the invoice associated with this subscription. If you specify this field, do not specify `invoice_owner_account_data`.
* @return invoiceOwnerAccountId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Identifier of the account that owns the invoice associated with this subscription. If you specify this field, do not specify `invoice_owner_account_data`.")
public String getInvoiceOwnerAccountId() {
return invoiceOwnerAccountId;
}
public void setInvoiceOwnerAccountId(String invoiceOwnerAccountId) {
this.invoiceOwnerAccountId = invoiceOwnerAccountId;
}
public SubscriptionPreviewRequest invoiceOwnerAccountNumber(String invoiceOwnerAccountNumber) {
this.invoiceOwnerAccountNumber = invoiceOwnerAccountNumber;
return this;
}
/**
* Identifier of the account that owns the invoice associated with this subscription. If you specify this field, do not specify `invoice_owner_account_data`.
* @return invoiceOwnerAccountNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Identifier of the account that owns the invoice associated with this subscription. If you specify this field, do not specify `invoice_owner_account_data`.")
public String getInvoiceOwnerAccountNumber() {
return invoiceOwnerAccountNumber;
}
public void setInvoiceOwnerAccountNumber(String invoiceOwnerAccountNumber) {
this.invoiceOwnerAccountNumber = invoiceOwnerAccountNumber;
}
public SubscriptionPreviewRequest invoiceOwnerAccountData(AccountCreateRequest invoiceOwnerAccountData) {
this.invoiceOwnerAccountData = invoiceOwnerAccountData;
return this;
}
/**
* The information of the new account that owns the invoice associated with this subscription. If you specify this field, do not specify `invoice_owner_account_id`.
* @return invoiceOwnerAccountData
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The information of the new account that owns the invoice associated with this subscription. If you specify this field, do not specify `invoice_owner_account_id`.")
public AccountCreateRequest getInvoiceOwnerAccountData() {
return invoiceOwnerAccountData;
}
public void setInvoiceOwnerAccountData(AccountCreateRequest invoiceOwnerAccountData) {
this.invoiceOwnerAccountData = invoiceOwnerAccountData;
}
public SubscriptionPreviewRequest accountId(String accountId) {
this.accountId = accountId;
return this;
}
/**
* Identifier of the account that owns the subscription. Subscription owner account can be different from the invoice owner account. If you specify this field, do not specify `account_data`.
* @return accountId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Identifier of the account that owns the subscription. Subscription owner account can be different from the invoice owner account. If you specify this field, do not specify `account_data`.")
public String getAccountId() {
return accountId;
}
public void setAccountId(String accountId) {
this.accountId = accountId;
}
public SubscriptionPreviewRequest accountNumber(String accountNumber) {
this.accountNumber = accountNumber;
return this;
}
/**
* Identifier of the account that owns the subscription. Subscription owner account can be different from the invoice owner account. If you specify this field, do not specify `account_data`.
* @return accountNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Identifier of the account that owns the subscription. Subscription owner account can be different from the invoice owner account. If you specify this field, do not specify `account_data`.")
public String getAccountNumber() {
return accountNumber;
}
public void setAccountNumber(String accountNumber) {
this.accountNumber = accountNumber;
}
public SubscriptionPreviewRequest accountData(SubscriptionPreviewAccountRequest accountData) {
this.accountData = accountData;
return this;
}
/**
* Get accountData
* @return accountData
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public SubscriptionPreviewAccountRequest getAccountData() {
return accountData;
}
public void setAccountData(SubscriptionPreviewAccountRequest accountData) {
this.accountData = accountData;
}
public SubscriptionPreviewRequest autoRenew(Boolean autoRenew) {
this.autoRenew = autoRenew;
return this;
}
/**
* If true, the subscription automatically renews at the end of the current term.
* @return autoRenew
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "If true, the subscription automatically renews at the end of the current term.")
public Boolean getAutoRenew() {
return autoRenew;
}
public void setAutoRenew(Boolean autoRenew) {
this.autoRenew = autoRenew;
}
public SubscriptionPreviewRequest subscriptionNumber(String subscriptionNumber) {
this.subscriptionNumber = subscriptionNumber;
return this;
}
/**
* Human-readable identifier of the subscription; maybe user-supplied.
* @return subscriptionNumber
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Human-readable identifier of the subscription; maybe user-supplied.")
public String getSubscriptionNumber() {
return subscriptionNumber;
}
public void setSubscriptionNumber(String subscriptionNumber) {
this.subscriptionNumber = subscriptionNumber;
}
public SubscriptionPreviewRequest initialTerm(Term initialTerm) {
this.initialTerm = initialTerm;
return this;
}
/**
* Initial term information for the subscription.
* @return initialTerm
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Initial term information for the subscription.")
public Term getInitialTerm() {
return initialTerm;
}
public void setInitialTerm(Term initialTerm) {
this.initialTerm = initialTerm;
}
public SubscriptionPreviewRequest renewalTerm(Term renewalTerm) {
this.renewalTerm = renewalTerm;
return this;
}
/**
* Renewal term information for the subscription
* @return renewalTerm
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Renewal term information for the subscription")
public Term getRenewalTerm() {
return renewalTerm;
}
public void setRenewalTerm(Term renewalTerm) {
this.renewalTerm = renewalTerm;
}
public SubscriptionPreviewRequest startOn(StartOn startOn) {
this.startOn = startOn;
return this;
}
/**
* Get startOn
* @return startOn
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public StartOn getStartOn() {
return startOn;
}
public void setStartOn(StartOn startOn) {
this.startOn = startOn;
}
public SubscriptionPreviewRequest description(String description) {
this.description = description;
return this;
}
/**
* Description of the subscription. Often useful for displaying to users.
* @return description
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Description of the subscription. Often useful for displaying to users.")
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public SubscriptionPreviewRequest invoiceSeparately(Boolean invoiceSeparately) {
this.invoiceSeparately = invoiceSeparately;
return this;
}
/**
* Separates a single subscription from other subscriptions and creates an invoice for this subscription. If the value is `true`, the subscription is billed separately from other subscriptions. If the value is `false`, the subscription is included with other subscriptions in the account invoice.
* @return invoiceSeparately
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Separates a single subscription from other subscriptions and creates an invoice for this subscription. If the value is `true`, the subscription is billed separately from other subscriptions. If the value is `false`, the subscription is included with other subscriptions in the account invoice.")
public Boolean getInvoiceSeparately() {
return invoiceSeparately;
}
public void setInvoiceSeparately(Boolean invoiceSeparately) {
this.invoiceSeparately = invoiceSeparately;
}
public SubscriptionPreviewRequest processingOptions(ProcessingOptions processingOptions) {
this.processingOptions = processingOptions;
return this;
}
/**
* Get processingOptions
* @return processingOptions
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public ProcessingOptions getProcessingOptions() {
return processingOptions;
}
public void setProcessingOptions(ProcessingOptions processingOptions) {
this.processingOptions = processingOptions;
}
public SubscriptionPreviewRequest customFields(Map customFields) {
this.customFields = customFields;
return this;
}
public SubscriptionPreviewRequest putCustomFieldsItem(String key, String customFieldsItem) {
if (this.customFields == null) {
this.customFields = new HashMap();
}
this.customFields.put(key, customFieldsItem);
return this;
}
/**
* Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.
* @return customFields
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.")
public Map getCustomFields() {
return customFields;
}
public void setCustomFields(Map customFields) {
this.customFields = customFields;
}
public SubscriptionPreviewRequest subscriptionPlans(List subscriptionPlans) {
this.subscriptionPlans = subscriptionPlans;
return this;
}
public SubscriptionPreviewRequest addSubscriptionPlansItem(SubscriptionPlanCreateRequest subscriptionPlansItem) {
if (this.subscriptionPlans == null) {
this.subscriptionPlans = new ArrayList();
}
this.subscriptionPlans.add(subscriptionPlansItem);
return this;
}
/**
* The plans associated with the new subscription.
* @return subscriptionPlans
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The plans associated with the new subscription.")
public List getSubscriptionPlans() {
return subscriptionPlans;
}
public void setSubscriptionPlans(List subscriptionPlans) {
this.subscriptionPlans = subscriptionPlans;
}
public SubscriptionPreviewRequest billToId(String billToId) {
this.billToId = billToId;
return this;
}
/**
* ID of the bill-to contact.
* @return billToId
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "2c92c0f86a8dd422016a9e7a70116b0d", value = "ID of the bill-to contact.")
public String getBillToId() {
return billToId;
}
public void setBillToId(String billToId) {
this.billToId = billToId;
}
public SubscriptionPreviewRequest paymentTerms(String paymentTerms) {
this.paymentTerms = paymentTerms;
return this;
}
/**
* The name of payment term associated with the invoice.
* @return paymentTerms
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The name of payment term associated with the invoice.")
public String getPaymentTerms() {
return paymentTerms;
}
public void setPaymentTerms(String paymentTerms) {
this.paymentTerms = paymentTerms;
}
/**
* The billing address for the customer.
* @return billTo
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The billing address for the customer.")
public Contact getBillTo() {
return billTo;
}
public SubscriptionPreviewRequest billingDocumentSettings(FlexibleBillingDocumentSettings billingDocumentSettings) {
this.billingDocumentSettings = billingDocumentSettings;
return this;
}
/**
* The billing document settings for the customer.
* @return billingDocumentSettings
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The billing document settings for the customer.")
public FlexibleBillingDocumentSettings getBillingDocumentSettings() {
return billingDocumentSettings;
}
public void setBillingDocumentSettings(FlexibleBillingDocumentSettings billingDocumentSettings) {
this.billingDocumentSettings = billingDocumentSettings;
}
public SubscriptionPreviewRequest soldToId(String soldToId) {
this.soldToId = soldToId;
return this;
}
/**
* ID of the sold-to contact.
* @return soldToId
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "2c92c0f86a8dd422016a9e7a70116b0d", value = "ID of the sold-to contact.")
public String getSoldToId() {
return soldToId;
}
public void setSoldToId(String soldToId) {
this.soldToId = soldToId;
}
/**
* The selling address for the customer.
* @return soldTo
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The selling address for the customer.")
public Contact getSoldTo() {
return soldTo;
}
public SubscriptionPreviewRequest currency(String currency) {
this.currency = currency;
return this;
}
/**
* 3-letter ISO 4217 currency code. This field is available only if you have the [Multiple Currencies](https://knowledgecenter.zuora.com/Zuora_Billing/Bill_your_customers/Flexible_Billing/Multiple_Currencies) feature enabled.
* @return currency
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "3-letter ISO 4217 currency code. This field is available only if you have the [Multiple Currencies](https://knowledgecenter.zuora.com/Zuora_Billing/Bill_your_customers/Flexible_Billing/Multiple_Currencies) feature enabled.")
public String getCurrency() {
return currency;
}
public void setCurrency(String currency) {
this.currency = currency;
}
public SubscriptionPreviewRequest changeReason(String changeReason) {
this.changeReason = changeReason;
return this;
}
/**
* A brief description of the reason for this change.
* @return changeReason
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A brief description of the reason for this change.")
public String getChangeReason() {
return changeReason;
}
public void setChangeReason(String changeReason) {
this.changeReason = changeReason;
}
public SubscriptionPreviewRequest numberOfPeriods(Integer numberOfPeriods) {
this.numberOfPeriods = numberOfPeriods;
return this;
}
/**
* Specifies how many billing periods you want to preview.
* @return numberOfPeriods
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Specifies how many billing periods you want to preview.")
public Integer getNumberOfPeriods() {
return numberOfPeriods;
}
public void setNumberOfPeriods(Integer numberOfPeriods) {
this.numberOfPeriods = numberOfPeriods;
}
public SubscriptionPreviewRequest termEnd(Boolean termEnd) {
this.termEnd = termEnd;
return this;
}
/**
* Indicates whether to preview the subscription till the end of the current term.
* @return termEnd
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Indicates whether to preview the subscription till the end of the current term.")
public Boolean getTermEnd() {
return termEnd;
}
public void setTermEnd(Boolean termEnd) {
this.termEnd = termEnd;
}
public SubscriptionPreviewRequest metrics(List metrics) {
this.metrics = metrics;
return this;
}
public SubscriptionPreviewRequest addMetricsItem(MetricsEnum metricsItem) {
if (this.metrics == null) {
this.metrics = new ArrayList();
}
this.metrics.add(metricsItem);
return this;
}
/**
* Specifies the metrics you want to preview. You can preivew metrics of billing documents, the order delta metrics, or both.
* @return metrics
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Specifies the metrics you want to preview. You can preivew metrics of billing documents, the order delta metrics, or both.")
public List getMetrics() {
return metrics;
}
public void setMetrics(List metrics) {
this.metrics = metrics;
}
public SubscriptionPreviewRequest endDate(LocalDate endDate) {
this.endDate = endDate;
return this;
}
/**
* End date of the period for which you want to preview the subscription
* @return endDate
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "End date of the period for which you want to preview the subscription")
public LocalDate getEndDate() {
return endDate;
}
public void setEndDate(LocalDate endDate) {
this.endDate = endDate;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
SubscriptionPreviewRequest subscriptionPreviewRequest = (SubscriptionPreviewRequest) o;
return Objects.equals(this.invoiceOwnerAccountId, subscriptionPreviewRequest.invoiceOwnerAccountId) &&
Objects.equals(this.invoiceOwnerAccountNumber, subscriptionPreviewRequest.invoiceOwnerAccountNumber) &&
Objects.equals(this.invoiceOwnerAccountData, subscriptionPreviewRequest.invoiceOwnerAccountData) &&
Objects.equals(this.accountId, subscriptionPreviewRequest.accountId) &&
Objects.equals(this.accountNumber, subscriptionPreviewRequest.accountNumber) &&
Objects.equals(this.accountData, subscriptionPreviewRequest.accountData) &&
Objects.equals(this.autoRenew, subscriptionPreviewRequest.autoRenew) &&
Objects.equals(this.subscriptionNumber, subscriptionPreviewRequest.subscriptionNumber) &&
Objects.equals(this.initialTerm, subscriptionPreviewRequest.initialTerm) &&
Objects.equals(this.renewalTerm, subscriptionPreviewRequest.renewalTerm) &&
Objects.equals(this.startOn, subscriptionPreviewRequest.startOn) &&
Objects.equals(this.description, subscriptionPreviewRequest.description) &&
Objects.equals(this.invoiceSeparately, subscriptionPreviewRequest.invoiceSeparately) &&
Objects.equals(this.processingOptions, subscriptionPreviewRequest.processingOptions) &&
Objects.equals(this.customFields, subscriptionPreviewRequest.customFields) &&
Objects.equals(this.subscriptionPlans, subscriptionPreviewRequest.subscriptionPlans) &&
Objects.equals(this.billToId, subscriptionPreviewRequest.billToId) &&
Objects.equals(this.paymentTerms, subscriptionPreviewRequest.paymentTerms) &&
Objects.equals(this.billTo, subscriptionPreviewRequest.billTo) &&
Objects.equals(this.billingDocumentSettings, subscriptionPreviewRequest.billingDocumentSettings) &&
Objects.equals(this.soldToId, subscriptionPreviewRequest.soldToId) &&
Objects.equals(this.soldTo, subscriptionPreviewRequest.soldTo) &&
Objects.equals(this.currency, subscriptionPreviewRequest.currency) &&
Objects.equals(this.changeReason, subscriptionPreviewRequest.changeReason) &&
Objects.equals(this.numberOfPeriods, subscriptionPreviewRequest.numberOfPeriods) &&
Objects.equals(this.termEnd, subscriptionPreviewRequest.termEnd) &&
Objects.equals(this.metrics, subscriptionPreviewRequest.metrics) &&
Objects.equals(this.endDate, subscriptionPreviewRequest.endDate);
}
private static boolean equalsNullable(JsonNullable a, JsonNullable b) {
return a == b || (a != null && b != null && a.isPresent() && b.isPresent() && Objects.deepEquals(a.get(), b.get()));
}
@Override
public int hashCode() {
return Objects.hash(invoiceOwnerAccountId, invoiceOwnerAccountNumber, invoiceOwnerAccountData, accountId, accountNumber, accountData, autoRenew, subscriptionNumber, initialTerm, renewalTerm, startOn, description, invoiceSeparately, processingOptions, customFields, subscriptionPlans, billToId, paymentTerms, billTo, billingDocumentSettings, soldToId, soldTo, currency, changeReason, numberOfPeriods, termEnd, metrics, endDate);
}
private static int hashCodeNullable(JsonNullable a) {
if (a == null) {
return 1;
}
return a.isPresent() ? Arrays.deepHashCode(new Object[]{a.get()}) : 31;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class SubscriptionPreviewRequest {\n");
sb.append(" invoiceOwnerAccountId: ").append(toIndentedString(invoiceOwnerAccountId)).append("\n");
sb.append(" invoiceOwnerAccountNumber: ").append(toIndentedString(invoiceOwnerAccountNumber)).append("\n");
sb.append(" invoiceOwnerAccountData: ").append(toIndentedString(invoiceOwnerAccountData)).append("\n");
sb.append(" accountId: ").append(toIndentedString(accountId)).append("\n");
sb.append(" accountNumber: ").append(toIndentedString(accountNumber)).append("\n");
sb.append(" accountData: ").append(toIndentedString(accountData)).append("\n");
sb.append(" autoRenew: ").append(toIndentedString(autoRenew)).append("\n");
sb.append(" subscriptionNumber: ").append(toIndentedString(subscriptionNumber)).append("\n");
sb.append(" initialTerm: ").append(toIndentedString(initialTerm)).append("\n");
sb.append(" renewalTerm: ").append(toIndentedString(renewalTerm)).append("\n");
sb.append(" startOn: ").append(toIndentedString(startOn)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" invoiceSeparately: ").append(toIndentedString(invoiceSeparately)).append("\n");
sb.append(" processingOptions: ").append(toIndentedString(processingOptions)).append("\n");
sb.append(" customFields: ").append(toIndentedString(customFields)).append("\n");
sb.append(" subscriptionPlans: ").append(toIndentedString(subscriptionPlans)).append("\n");
sb.append(" billToId: ").append(toIndentedString(billToId)).append("\n");
sb.append(" paymentTerms: ").append(toIndentedString(paymentTerms)).append("\n");
sb.append(" billTo: ").append(toIndentedString(billTo)).append("\n");
sb.append(" billingDocumentSettings: ").append(toIndentedString(billingDocumentSettings)).append("\n");
sb.append(" soldToId: ").append(toIndentedString(soldToId)).append("\n");
sb.append(" soldTo: ").append(toIndentedString(soldTo)).append("\n");
sb.append(" currency: ").append(toIndentedString(currency)).append("\n");
sb.append(" changeReason: ").append(toIndentedString(changeReason)).append("\n");
sb.append(" numberOfPeriods: ").append(toIndentedString(numberOfPeriods)).append("\n");
sb.append(" termEnd: ").append(toIndentedString(termEnd)).append("\n");
sb.append(" metrics: ").append(toIndentedString(metrics)).append("\n");
sb.append(" endDate: ").append(toIndentedString(endDate)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy