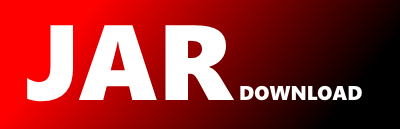
org.openapitools.client.model.SubscriptionReplacePlanPatchRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.openapitools.client.model.StartOn;
import org.openapitools.client.model.SubscriptionPlanCreateRequest;
import org.openapitools.client.JSON.CustomFieldAdapter;
import org.openapitools.client.JSON.NullableFieldAdapter;
/**
* SubscriptionReplacePlanPatchRequest
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class SubscriptionReplacePlanPatchRequest {
public static final String SERIALIZED_NAME_SUBSCRIPTION_PLAN_ID = "subscription_plan_id";
@SerializedName(SERIALIZED_NAME_SUBSCRIPTION_PLAN_ID)
private String subscriptionPlanId;
public static final String SERIALIZED_NAME_PREVIOUS_PLAN_ID = "previous_plan_id";
@SerializedName(SERIALIZED_NAME_PREVIOUS_PLAN_ID)
private String previousPlanId;
/**
* The default value for the `replace_at` field is as follows: <ul> <li>If the subscription plan change (from old to new) is an upgrade, the `replace_at` is `now` by default. </li> <li>If the subscription change (from old to new) is a downgrade, the `replace_at` is `end_of_billing_period` by default. </li> </ul> Otherwise, the `replace_at` is `specific_date` by default. **Notes**: <br /> When setting this field to `end_of_billing_period`, you cannot set the billing start dates for the subscription as the system will automatically set the start dates to the end of billing period, and you cannot set the following billing trigger date settings to `Yes`: <ul> <li> <a href=\"https://knowledgecenter.zuora.com/Zuora_Billing/Configure_billing_settings/Subscription_and_order_settings/A_Define_Default_Subscription_and_Order_Settings#Require_Customer_Acceptance_of_Orders.3F\" target=\"_blank\">Require Customer Acceptance of Orders</a> </li> <li> <a href=\"https://knowledgecenter.zuora.com/Zuora_Billing/Billing_and_Invoicing/Billing_Settings/Define_Default_Subscription_and_Order_Settings#Require_Service_Activation_of_Orders.3F\" target=\"_blank\">Require Service Activation of Orders</a> </li> </ul> When setting this field to `specific_date`, you must also specify a date for the `contract_effective` date in the `start_on` field.
*/
@JsonAdapter(ReplaceAtEnum.Adapter.class)
public enum ReplaceAtEnum {
NOW("now"),
END_OF_BILLING_PERIOD("end_of_billing_period"),
SPECIFIC_DATE("specific_date"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
ReplaceAtEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static ReplaceAtEnum fromValue(String value) {
for (ReplaceAtEnum b : ReplaceAtEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final ReplaceAtEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public ReplaceAtEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return ReplaceAtEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_REPLACE_AT = "replace_at";
@SerializedName(SERIALIZED_NAME_REPLACE_AT)
private ReplaceAtEnum replaceAt;
/**
* Gets or Sets replacementType
*/
@JsonAdapter(ReplacementTypeEnum.Adapter.class)
public enum ReplacementTypeEnum {
UPGRADE("upgrade"),
DOWNGRADE("downgrade"),
CROSSGRADE("crossgrade"),
OTHER("other"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
ReplacementTypeEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static ReplacementTypeEnum fromValue(String value) {
for (ReplacementTypeEnum b : ReplacementTypeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final ReplacementTypeEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public ReplacementTypeEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return ReplacementTypeEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_REPLACEMENT_TYPE = "replacement_type";
@SerializedName(SERIALIZED_NAME_REPLACEMENT_TYPE)
private ReplacementTypeEnum replacementType;
public static final String SERIALIZED_NAME_SUBSCRIPTION_PLAN = "subscription_plan";
@SerializedName(SERIALIZED_NAME_SUBSCRIPTION_PLAN)
private SubscriptionPlanCreateRequest subscriptionPlan;
public static final String SERIALIZED_NAME_START_ON = "start_on";
@SerializedName(SERIALIZED_NAME_START_ON)
private StartOn startOn;
public static final String SERIALIZED_NAME_CHANGE_REASON = "change_reason";
@SerializedName(SERIALIZED_NAME_CHANGE_REASON)
private String changeReason;
public static final String SERIALIZED_NAME_CUSTOM_FIELDS = "custom_fields";
@SerializedName(SERIALIZED_NAME_CUSTOM_FIELDS)
@JsonAdapter(CustomFieldAdapter.class)
private Map customFields = null;
public SubscriptionReplacePlanPatchRequest() {
}
public SubscriptionReplacePlanPatchRequest subscriptionPlanId(String subscriptionPlanId) {
this.subscriptionPlanId = subscriptionPlanId;
return this;
}
/**
* Identifier of the subscription plan. Only provide one of `previous_plan_id` or `subscription_plan_id` in your request, not both.
* @return subscriptionPlanId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Identifier of the subscription plan. Only provide one of `previous_plan_id` or `subscription_plan_id` in your request, not both.")
public String getSubscriptionPlanId() {
return subscriptionPlanId;
}
public void setSubscriptionPlanId(String subscriptionPlanId) {
this.subscriptionPlanId = subscriptionPlanId;
}
public SubscriptionReplacePlanPatchRequest previousPlanId(String previousPlanId) {
this.previousPlanId = previousPlanId;
return this;
}
/**
* Identifier of the plan to be removed. Only provide one of `previous_plan_id` or `subscription_plan_id` in your request, not both.
* @return previousPlanId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Identifier of the plan to be removed. Only provide one of `previous_plan_id` or `subscription_plan_id` in your request, not both.")
public String getPreviousPlanId() {
return previousPlanId;
}
public void setPreviousPlanId(String previousPlanId) {
this.previousPlanId = previousPlanId;
}
public SubscriptionReplacePlanPatchRequest replaceAt(ReplaceAtEnum replaceAt) {
this.replaceAt = replaceAt;
return this;
}
/**
* The default value for the `replace_at` field is as follows: <ul> <li>If the subscription plan change (from old to new) is an upgrade, the `replace_at` is `now` by default. </li> <li>If the subscription change (from old to new) is a downgrade, the `replace_at` is `end_of_billing_period` by default. </li> </ul> Otherwise, the `replace_at` is `specific_date` by default. **Notes**: <br /> When setting this field to `end_of_billing_period`, you cannot set the billing start dates for the subscription as the system will automatically set the start dates to the end of billing period, and you cannot set the following billing trigger date settings to `Yes`: <ul> <li> <a href=\"https://knowledgecenter.zuora.com/Zuora_Billing/Configure_billing_settings/Subscription_and_order_settings/A_Define_Default_Subscription_and_Order_Settings#Require_Customer_Acceptance_of_Orders.3F\" target=\"_blank\">Require Customer Acceptance of Orders</a> </li> <li> <a href=\"https://knowledgecenter.zuora.com/Zuora_Billing/Billing_and_Invoicing/Billing_Settings/Define_Default_Subscription_and_Order_Settings#Require_Service_Activation_of_Orders.3F\" target=\"_blank\">Require Service Activation of Orders</a> </li> </ul> When setting this field to `specific_date`, you must also specify a date for the `contract_effective` date in the `start_on` field.
* @return replaceAt
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The default value for the `replace_at` field is as follows: - If the subscription plan change (from old to new) is an upgrade, the `replace_at` is `now` by default.
- If the subscription change (from old to new) is a downgrade, the `replace_at` is `end_of_billing_period` by default.
Otherwise, the `replace_at` is `specific_date` by default. **Notes**:
When setting this field to `end_of_billing_period`, you cannot set the billing start dates for the subscription as the system will automatically set the start dates to the end of billing period, and you cannot set the following billing trigger date settings to `Yes`: When setting this field to `specific_date`, you must also specify a date for the `contract_effective` date in the `start_on` field. ")
public ReplaceAtEnum getReplaceAt() {
return replaceAt;
}
public void setReplaceAt(ReplaceAtEnum replaceAt) {
this.replaceAt = replaceAt;
}
public SubscriptionReplacePlanPatchRequest replacementType(ReplacementTypeEnum replacementType) {
this.replacementType = replacementType;
return this;
}
/**
* Get replacementType
* @return replacementType
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public ReplacementTypeEnum getReplacementType() {
return replacementType;
}
public void setReplacementType(ReplacementTypeEnum replacementType) {
this.replacementType = replacementType;
}
public SubscriptionReplacePlanPatchRequest subscriptionPlan(SubscriptionPlanCreateRequest subscriptionPlan) {
this.subscriptionPlan = subscriptionPlan;
return this;
}
/**
* Get subscriptionPlan
* @return subscriptionPlan
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "")
public SubscriptionPlanCreateRequest getSubscriptionPlan() {
return subscriptionPlan;
}
public void setSubscriptionPlan(SubscriptionPlanCreateRequest subscriptionPlan) {
this.subscriptionPlan = subscriptionPlan;
}
public SubscriptionReplacePlanPatchRequest startOn(StartOn startOn) {
this.startOn = startOn;
return this;
}
/**
* Get startOn
* @return startOn
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public StartOn getStartOn() {
return startOn;
}
public void setStartOn(StartOn startOn) {
this.startOn = startOn;
}
public SubscriptionReplacePlanPatchRequest changeReason(String changeReason) {
this.changeReason = changeReason;
return this;
}
/**
* A brief description of the reason for this change.
* @return changeReason
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A brief description of the reason for this change.")
public String getChangeReason() {
return changeReason;
}
public void setChangeReason(String changeReason) {
this.changeReason = changeReason;
}
public SubscriptionReplacePlanPatchRequest customFields(Map customFields) {
this.customFields = customFields;
return this;
}
public SubscriptionReplacePlanPatchRequest putCustomFieldsItem(String key, String customFieldsItem) {
if (this.customFields == null) {
this.customFields = new HashMap();
}
this.customFields.put(key, customFieldsItem);
return this;
}
/**
* Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.
* @return customFields
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.")
public Map getCustomFields() {
return customFields;
}
public void setCustomFields(Map customFields) {
this.customFields = customFields;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
SubscriptionReplacePlanPatchRequest subscriptionReplacePlanPatchRequest = (SubscriptionReplacePlanPatchRequest) o;
return Objects.equals(this.subscriptionPlanId, subscriptionReplacePlanPatchRequest.subscriptionPlanId) &&
Objects.equals(this.previousPlanId, subscriptionReplacePlanPatchRequest.previousPlanId) &&
Objects.equals(this.replaceAt, subscriptionReplacePlanPatchRequest.replaceAt) &&
Objects.equals(this.replacementType, subscriptionReplacePlanPatchRequest.replacementType) &&
Objects.equals(this.subscriptionPlan, subscriptionReplacePlanPatchRequest.subscriptionPlan) &&
Objects.equals(this.startOn, subscriptionReplacePlanPatchRequest.startOn) &&
Objects.equals(this.changeReason, subscriptionReplacePlanPatchRequest.changeReason) &&
Objects.equals(this.customFields, subscriptionReplacePlanPatchRequest.customFields);
}
@Override
public int hashCode() {
return Objects.hash(subscriptionPlanId, previousPlanId, replaceAt, replacementType, subscriptionPlan, startOn, changeReason, customFields);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class SubscriptionReplacePlanPatchRequest {\n");
sb.append(" subscriptionPlanId: ").append(toIndentedString(subscriptionPlanId)).append("\n");
sb.append(" previousPlanId: ").append(toIndentedString(previousPlanId)).append("\n");
sb.append(" replaceAt: ").append(toIndentedString(replaceAt)).append("\n");
sb.append(" replacementType: ").append(toIndentedString(replacementType)).append("\n");
sb.append(" subscriptionPlan: ").append(toIndentedString(subscriptionPlan)).append("\n");
sb.append(" startOn: ").append(toIndentedString(startOn)).append("\n");
sb.append(" changeReason: ").append(toIndentedString(changeReason)).append("\n");
sb.append(" customFields: ").append(toIndentedString(customFields)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy