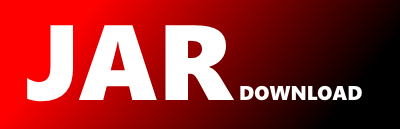
org.openapitools.client.model.SubscriptionReplacePlanPatchResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.openapitools.client.model.SubscriptionItemListResponse;
import org.openapitools.jackson.nullable.JsonNullable;
import org.openapitools.client.JSON.CustomFieldAdapter;
import org.openapitools.client.JSON.NullableFieldAdapter;
/**
* SubscriptionReplacePlanPatchResponse
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class SubscriptionReplacePlanPatchResponse {
public static final String SERIALIZED_NAME_PREVIOUS_SUBSCRIPTION_PLAN_ID = "previous_subscription_plan_id";
@SerializedName(SERIALIZED_NAME_PREVIOUS_SUBSCRIPTION_PLAN_ID)
private String previousSubscriptionPlanId;
public static final String SERIALIZED_NAME_PREVIOUS_PLAN_ID = "previous_plan_id";
@SerializedName(SERIALIZED_NAME_PREVIOUS_PLAN_ID)
private String previousPlanId;
/**
* The date when the replacement occurs. It can be either the current date or the end of the current billing period.
*/
@JsonAdapter(ReplaceAtEnum.Adapter.class)
public enum ReplaceAtEnum {
NOW("now"),
END_OF_BILLING_PERIOD("end_of_billing_period"),
SPECIFIC_DATE("specific_date"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
ReplaceAtEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static ReplaceAtEnum fromValue(String value) {
for (ReplaceAtEnum b : ReplaceAtEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final ReplaceAtEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public ReplaceAtEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return ReplaceAtEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_REPLACE_AT = "replace_at";
@SerializedName(SERIALIZED_NAME_REPLACE_AT)
private ReplaceAtEnum replaceAt;
/**
* The type of the replacement.
*/
@JsonAdapter(ReplacementTypeEnum.Adapter.class)
public enum ReplacementTypeEnum {
UPGRADE("upgrade"),
DOWNGRADE("downgrade"),
CROSSGRADE("crossgrade"),
OTHER("other"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
ReplacementTypeEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static ReplacementTypeEnum fromValue(String value) {
for (ReplacementTypeEnum b : ReplacementTypeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final ReplacementTypeEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public ReplacementTypeEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return ReplacementTypeEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_REPLACEMENT_TYPE = "replacement_type";
@SerializedName(SERIALIZED_NAME_REPLACEMENT_TYPE)
private ReplacementTypeEnum replacementType;
public static final String SERIALIZED_NAME_UNIQUE_TOKEN = "unique_token";
@SerializedName(SERIALIZED_NAME_UNIQUE_TOKEN)
private String uniqueToken;
public static final String SERIALIZED_NAME_PLAN_ID = "plan_id";
@SerializedName(SERIALIZED_NAME_PLAN_ID)
private String planId;
public static final String SERIALIZED_NAME_CUSTOM_FIELDS = "custom_fields";
@SerializedName(SERIALIZED_NAME_CUSTOM_FIELDS)
@JsonAdapter(CustomFieldAdapter.class)
private Map customFields = null;
public static final String SERIALIZED_NAME_SUBSCRIPTION_ITEMS = "subscription_items";
@SerializedName(SERIALIZED_NAME_SUBSCRIPTION_ITEMS)
private SubscriptionItemListResponse subscriptionItems;
public SubscriptionReplacePlanPatchResponse() {
}
public SubscriptionReplacePlanPatchResponse previousSubscriptionPlanId(String previousSubscriptionPlanId) {
this.previousSubscriptionPlanId = previousSubscriptionPlanId;
return this;
}
/**
* Identifier of the subscription plan.
* @return previousSubscriptionPlanId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Identifier of the subscription plan.")
public String getPreviousSubscriptionPlanId() {
return previousSubscriptionPlanId;
}
public void setPreviousSubscriptionPlanId(String previousSubscriptionPlanId) {
this.previousSubscriptionPlanId = previousSubscriptionPlanId;
}
public SubscriptionReplacePlanPatchResponse previousPlanId(String previousPlanId) {
this.previousPlanId = previousPlanId;
return this;
}
/**
* Identifier of the plan to be removed. Only provide one of `previous_plan_id` or `subscription_plan_id` in your request, not both.
* @return previousPlanId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Identifier of the plan to be removed. Only provide one of `previous_plan_id` or `subscription_plan_id` in your request, not both.")
public String getPreviousPlanId() {
return previousPlanId;
}
public void setPreviousPlanId(String previousPlanId) {
this.previousPlanId = previousPlanId;
}
public SubscriptionReplacePlanPatchResponse replaceAt(ReplaceAtEnum replaceAt) {
this.replaceAt = replaceAt;
return this;
}
/**
* The date when the replacement occurs. It can be either the current date or the end of the current billing period.
* @return replaceAt
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The date when the replacement occurs. It can be either the current date or the end of the current billing period.")
public ReplaceAtEnum getReplaceAt() {
return replaceAt;
}
public void setReplaceAt(ReplaceAtEnum replaceAt) {
this.replaceAt = replaceAt;
}
public SubscriptionReplacePlanPatchResponse replacementType(ReplacementTypeEnum replacementType) {
this.replacementType = replacementType;
return this;
}
/**
* The type of the replacement.
* @return replacementType
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The type of the replacement.")
public ReplacementTypeEnum getReplacementType() {
return replacementType;
}
public void setReplacementType(ReplacementTypeEnum replacementType) {
this.replacementType = replacementType;
}
public SubscriptionReplacePlanPatchResponse uniqueToken(String uniqueToken) {
this.uniqueToken = uniqueToken;
return this;
}
/**
* A unique string to represent the subscription plan in the order. The unique token is used to perform multiple actions against a newly added subscription plan. For example, if you want to add and update a product in the same order, assign a unique token to the newly added subscription plan and use that token in future order actions.
* @return uniqueToken
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A unique string to represent the subscription plan in the order. The unique token is used to perform multiple actions against a newly added subscription plan. For example, if you want to add and update a product in the same order, assign a unique token to the newly added subscription plan and use that token in future order actions.")
public String getUniqueToken() {
return uniqueToken;
}
public void setUniqueToken(String uniqueToken) {
this.uniqueToken = uniqueToken;
}
public SubscriptionReplacePlanPatchResponse planId(String planId) {
this.planId = planId;
return this;
}
/**
* The id of the subscription plan to be updated. It can be the latest version or any history version id.
* @return planId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The id of the subscription plan to be updated. It can be the latest version or any history version id.")
public String getPlanId() {
return planId;
}
public void setPlanId(String planId) {
this.planId = planId;
}
public SubscriptionReplacePlanPatchResponse customFields(Map customFields) {
this.customFields = customFields;
return this;
}
public SubscriptionReplacePlanPatchResponse putCustomFieldsItem(String key, String customFieldsItem) {
if (this.customFields == null) {
this.customFields = new HashMap();
}
this.customFields.put(key, customFieldsItem);
return this;
}
/**
* Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.
* @return customFields
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.")
public Map getCustomFields() {
return customFields;
}
public void setCustomFields(Map customFields) {
this.customFields = customFields;
}
public SubscriptionReplacePlanPatchResponse subscriptionItems(SubscriptionItemListResponse subscriptionItems) {
this.subscriptionItems = subscriptionItems;
return this;
}
/**
* Get subscriptionItems
* @return subscriptionItems
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public SubscriptionItemListResponse getSubscriptionItems() {
return subscriptionItems;
}
public void setSubscriptionItems(SubscriptionItemListResponse subscriptionItems) {
this.subscriptionItems = subscriptionItems;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
SubscriptionReplacePlanPatchResponse subscriptionReplacePlanPatchResponse = (SubscriptionReplacePlanPatchResponse) o;
return Objects.equals(this.previousSubscriptionPlanId, subscriptionReplacePlanPatchResponse.previousSubscriptionPlanId) &&
Objects.equals(this.previousPlanId, subscriptionReplacePlanPatchResponse.previousPlanId) &&
Objects.equals(this.replaceAt, subscriptionReplacePlanPatchResponse.replaceAt) &&
Objects.equals(this.replacementType, subscriptionReplacePlanPatchResponse.replacementType) &&
Objects.equals(this.uniqueToken, subscriptionReplacePlanPatchResponse.uniqueToken) &&
Objects.equals(this.planId, subscriptionReplacePlanPatchResponse.planId) &&
Objects.equals(this.customFields, subscriptionReplacePlanPatchResponse.customFields) &&
Objects.equals(this.subscriptionItems, subscriptionReplacePlanPatchResponse.subscriptionItems);
}
private static boolean equalsNullable(JsonNullable a, JsonNullable b) {
return a == b || (a != null && b != null && a.isPresent() && b.isPresent() && Objects.deepEquals(a.get(), b.get()));
}
@Override
public int hashCode() {
return Objects.hash(previousSubscriptionPlanId, previousPlanId, replaceAt, replacementType, uniqueToken, planId, customFields, subscriptionItems);
}
private static int hashCodeNullable(JsonNullable a) {
if (a == null) {
return 1;
}
return a.isPresent() ? Arrays.deepHashCode(new Object[]{a.get()}) : 31;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class SubscriptionReplacePlanPatchResponse {\n");
sb.append(" previousSubscriptionPlanId: ").append(toIndentedString(previousSubscriptionPlanId)).append("\n");
sb.append(" previousPlanId: ").append(toIndentedString(previousPlanId)).append("\n");
sb.append(" replaceAt: ").append(toIndentedString(replaceAt)).append("\n");
sb.append(" replacementType: ").append(toIndentedString(replacementType)).append("\n");
sb.append(" uniqueToken: ").append(toIndentedString(uniqueToken)).append("\n");
sb.append(" planId: ").append(toIndentedString(planId)).append("\n");
sb.append(" customFields: ").append(toIndentedString(customFields)).append("\n");
sb.append(" subscriptionItems: ").append(toIndentedString(subscriptionItems)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy