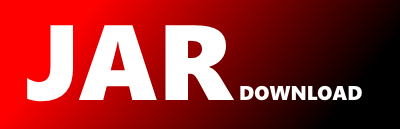
org.openapitools.client.model.TaxationItem Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.math.BigDecimal;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.openapitools.client.model.CustomObject;
import org.openapitools.client.model.ListCustomObjectResponse;
import org.threeten.bp.LocalDate;
import org.threeten.bp.OffsetDateTime;
import org.openapitools.client.JSON.CustomFieldAdapter;
import org.openapitools.client.JSON.NullableFieldAdapter;
/**
* taxation information for this billing document item.
*/
@ApiModel(description = "taxation information for this billing document item.")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class TaxationItem {
public static final String SERIALIZED_NAME_ID = "id";
@SerializedName(SERIALIZED_NAME_ID)
private String id;
public static final String SERIALIZED_NAME_UPDATED_BY_ID = "updated_by_id";
@SerializedName(SERIALIZED_NAME_UPDATED_BY_ID)
private String updatedById;
public static final String SERIALIZED_NAME_UPDATED_TIME = "updated_time";
@SerializedName(SERIALIZED_NAME_UPDATED_TIME)
private OffsetDateTime updatedTime;
public static final String SERIALIZED_NAME_CREATED_BY_ID = "created_by_id";
@SerializedName(SERIALIZED_NAME_CREATED_BY_ID)
private String createdById;
public static final String SERIALIZED_NAME_CREATED_TIME = "created_time";
@SerializedName(SERIALIZED_NAME_CREATED_TIME)
private OffsetDateTime createdTime;
public static final String SERIALIZED_NAME_CUSTOM_FIELDS = "custom_fields";
@SerializedName(SERIALIZED_NAME_CUSTOM_FIELDS)
@JsonAdapter(CustomFieldAdapter.class)
private Map customFields = null;
public static final String SERIALIZED_NAME_CUSTOM_OBJECTS = "custom_objects";
@SerializedName(SERIALIZED_NAME_CUSTOM_OBJECTS)
private Map customObjects = null;
public static final String SERIALIZED_NAME_JURISDICTION = "jurisdiction";
@SerializedName(SERIALIZED_NAME_JURISDICTION)
private String jurisdiction;
public static final String SERIALIZED_NAME_LOCATION_CODE = "location_code";
@SerializedName(SERIALIZED_NAME_LOCATION_CODE)
private String locationCode;
public static final String SERIALIZED_NAME_NAME = "name";
@SerializedName(SERIALIZED_NAME_NAME)
private String name;
public static final String SERIALIZED_NAME_AMOUNT = "amount";
@SerializedName(SERIALIZED_NAME_AMOUNT)
private BigDecimal amount;
public static final String SERIALIZED_NAME_TAX_CODE = "tax_code";
@SerializedName(SERIALIZED_NAME_TAX_CODE)
private String taxCode;
public static final String SERIALIZED_NAME_TAX_CODE_NAME = "tax_code_name";
@SerializedName(SERIALIZED_NAME_TAX_CODE_NAME)
private String taxCodeName;
public static final String SERIALIZED_NAME_TAX_DATE = "tax_date";
@SerializedName(SERIALIZED_NAME_TAX_DATE)
private LocalDate taxDate;
public static final String SERIALIZED_NAME_TAX_RATE = "tax_rate";
@SerializedName(SERIALIZED_NAME_TAX_RATE)
private BigDecimal taxRate;
public static final String SERIALIZED_NAME_TAX_RATE_NAME = "tax_rate_name";
@SerializedName(SERIALIZED_NAME_TAX_RATE_NAME)
private String taxRateName;
public static final String SERIALIZED_NAME_AMOUNT_EXEMPT = "amount_exempt";
@SerializedName(SERIALIZED_NAME_AMOUNT_EXEMPT)
private BigDecimal amountExempt;
public static final String SERIALIZED_NAME_SOURCE_TAX_ITEM_ID = "source_tax_item_id";
@SerializedName(SERIALIZED_NAME_SOURCE_TAX_ITEM_ID)
private String sourceTaxItemId;
public static final String SERIALIZED_NAME_REMAINING_BALANCE = "remaining_balance";
@SerializedName(SERIALIZED_NAME_REMAINING_BALANCE)
private BigDecimal remainingBalance;
public static final String SERIALIZED_NAME_AMOUNT_CREDITED = "amount_credited";
@SerializedName(SERIALIZED_NAME_AMOUNT_CREDITED)
private BigDecimal amountCredited;
public static final String SERIALIZED_NAME_AMOUNT_PAID = "amount_paid";
@SerializedName(SERIALIZED_NAME_AMOUNT_PAID)
private BigDecimal amountPaid;
public static final String SERIALIZED_NAME_AMOUNT_REFUNDED = "amount_refunded";
@SerializedName(SERIALIZED_NAME_AMOUNT_REFUNDED)
private BigDecimal amountRefunded;
public static final String SERIALIZED_NAME_AMOUNT_APPLIED = "amount_applied";
@SerializedName(SERIALIZED_NAME_AMOUNT_APPLIED)
private BigDecimal amountApplied;
public static final String SERIALIZED_NAME_SALES_TAX_PAYABLE_ACCOUNT = "sales_tax_payable_account";
@SerializedName(SERIALIZED_NAME_SALES_TAX_PAYABLE_ACCOUNT)
private String salesTaxPayableAccount;
public static final String SERIALIZED_NAME_ON_ACCOUNT_ACCOUNT = "on_account_account";
@SerializedName(SERIALIZED_NAME_ON_ACCOUNT_ACCOUNT)
private String onAccountAccount;
public static final String SERIALIZED_NAME_TAX_INCLUSIVE = "tax_inclusive";
@SerializedName(SERIALIZED_NAME_TAX_INCLUSIVE)
private Boolean taxInclusive;
/**
* Indicates whether the tax rate is an amount or a percentage.
*/
@JsonAdapter(TaxRateTypeEnum.Adapter.class)
public enum TaxRateTypeEnum {
PERCENT("percent"),
AMOUNT("amount"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
TaxRateTypeEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static TaxRateTypeEnum fromValue(String value) {
for (TaxRateTypeEnum b : TaxRateTypeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final TaxRateTypeEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public TaxRateTypeEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return TaxRateTypeEnum.fromValue(value);
}
}
}
public static final String SERIALIZED_NAME_TAX_RATE_TYPE = "tax_rate_type";
@SerializedName(SERIALIZED_NAME_TAX_RATE_TYPE)
private TaxRateTypeEnum taxRateType;
public TaxationItem() {
}
public TaxationItem(
String updatedById,
OffsetDateTime updatedTime,
String createdById,
OffsetDateTime createdTime,
Map customObjects,
BigDecimal remainingBalance,
BigDecimal amountCredited,
BigDecimal amountPaid,
BigDecimal amountRefunded,
BigDecimal amountApplied
) {
this();
this.updatedById = updatedById;
this.updatedTime = updatedTime;
this.createdById = createdById;
this.createdTime = createdTime;
this.customObjects = customObjects;
this.remainingBalance = remainingBalance;
this.amountCredited = amountCredited;
this.amountPaid = amountPaid;
this.amountRefunded = amountRefunded;
this.amountApplied = amountApplied;
}
public TaxationItem id(String id) {
this.id = id;
return this;
}
/**
* Identifier of the taxation item related to the invoice. Only applicable for credit memos created from invoices.
* @return id
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Identifier of the taxation item related to the invoice. Only applicable for credit memos created from invoices.")
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
/**
* Unique identifier of the Zuora user who last updated the object
* @return updatedById
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier of the Zuora user who last updated the object")
public String getUpdatedById() {
return updatedById;
}
/**
* The date and time when the object was last updated in ISO 8601 UTC format.
* @return updatedTime
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The date and time when the object was last updated in ISO 8601 UTC format.")
public OffsetDateTime getUpdatedTime() {
return updatedTime;
}
/**
* Unique identifier of the Zuora user who created the object
* @return createdById
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Unique identifier of the Zuora user who created the object")
public String getCreatedById() {
return createdById;
}
/**
* The date and time when the object was created in ISO 8601 UTC format.
* @return createdTime
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The date and time when the object was created in ISO 8601 UTC format.")
public OffsetDateTime getCreatedTime() {
return createdTime;
}
public TaxationItem customFields(Map customFields) {
this.customFields = customFields;
return this;
}
public TaxationItem putCustomFieldsItem(String key, String customFieldsItem) {
if (this.customFields == null) {
this.customFields = new HashMap();
}
this.customFields.put(key, customFieldsItem);
return this;
}
/**
* Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.
* @return customFields
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Set of user-defined fields associated with this object. Useful for storing additional information about the object in a structured format.")
public Map getCustomFields() {
return customFields;
}
public void setCustomFields(Map customFields) {
this.customFields = customFields;
}
/**
* The custom objects associated with a Zuora standard object.
* @return customObjects
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The custom objects associated with a Zuora standard object.")
public Map getCustomObjects() {
return customObjects;
}
public TaxationItem jurisdiction(String jurisdiction) {
this.jurisdiction = jurisdiction;
return this;
}
/**
* The jurisdiction that applies the tax or VAT. This value is typically a state, province, county, or city.
* @return jurisdiction
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The jurisdiction that applies the tax or VAT. This value is typically a state, province, county, or city.")
public String getJurisdiction() {
return jurisdiction;
}
public void setJurisdiction(String jurisdiction) {
this.jurisdiction = jurisdiction;
}
public TaxationItem locationCode(String locationCode) {
this.locationCode = locationCode;
return this;
}
/**
* The identifier for the location based on the value of the `tax_code` field.
* @return locationCode
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The identifier for the location based on the value of the `tax_code` field.")
public String getLocationCode() {
return locationCode;
}
public void setLocationCode(String locationCode) {
this.locationCode = locationCode;
}
public TaxationItem name(String name) {
this.name = name;
return this;
}
/**
* The name of the taxation item.
* @return name
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The name of the taxation item.")
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public TaxationItem amount(BigDecimal amount) {
this.amount = amount;
return this;
}
/**
* The amount of the tax applied to the total price.
* @return amount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The amount of the tax applied to the total price.")
public BigDecimal getAmount() {
return amount;
}
public void setAmount(BigDecimal amount) {
this.amount = amount;
}
public TaxationItem taxCode(String taxCode) {
this.taxCode = taxCode;
return this;
}
/**
* A tax code identifier. If a `tax_code` of a price is not provided when you create or update a price, Zuora will treat the charged amount as non-taxable. If this code is provide, Zuora considers that this price is taxable and the charged amount will be handled accordingly.
* @return taxCode
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A tax code identifier. If a `tax_code` of a price is not provided when you create or update a price, Zuora will treat the charged amount as non-taxable. If this code is provide, Zuora considers that this price is taxable and the charged amount will be handled accordingly.")
public String getTaxCode() {
return taxCode;
}
public void setTaxCode(String taxCode) {
this.taxCode = taxCode;
}
public TaxationItem taxCodeName(String taxCodeName) {
this.taxCodeName = taxCodeName;
return this;
}
/**
* The amount of the tax applied to the total price.
* @return taxCodeName
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The amount of the tax applied to the total price.")
public String getTaxCodeName() {
return taxCodeName;
}
public void setTaxCodeName(String taxCodeName) {
this.taxCodeName = taxCodeName;
}
public TaxationItem taxDate(LocalDate taxDate) {
this.taxDate = taxDate;
return this;
}
/**
* The date on which the tax is applied.
* @return taxDate
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "Sat Jan 01 00:00:00 GMT 2022", value = "The date on which the tax is applied.")
public LocalDate getTaxDate() {
return taxDate;
}
public void setTaxDate(LocalDate taxDate) {
this.taxDate = taxDate;
}
public TaxationItem taxRate(BigDecimal taxRate) {
this.taxRate = taxRate;
return this;
}
/**
* The amount of the tax applied to the total price.
* @return taxRate
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The amount of the tax applied to the total price.")
public BigDecimal getTaxRate() {
return taxRate;
}
public void setTaxRate(BigDecimal taxRate) {
this.taxRate = taxRate;
}
public TaxationItem taxRateName(String taxRateName) {
this.taxRateName = taxRateName;
return this;
}
/**
* The name of the tax rate, such as sales tax or GST. This name is displayed on billing documents.
* @return taxRateName
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The name of the tax rate, such as sales tax or GST. This name is displayed on billing documents.")
public String getTaxRateName() {
return taxRateName;
}
public void setTaxRateName(String taxRateName) {
this.taxRateName = taxRateName;
}
public TaxationItem amountExempt(BigDecimal amountExempt) {
this.amountExempt = amountExempt;
return this;
}
/**
* The calculated tax amount excluded due to the exemption.
* @return amountExempt
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The calculated tax amount excluded due to the exemption.")
public BigDecimal getAmountExempt() {
return amountExempt;
}
public void setAmountExempt(BigDecimal amountExempt) {
this.amountExempt = amountExempt;
}
public TaxationItem sourceTaxItemId(String sourceTaxItemId) {
this.sourceTaxItemId = sourceTaxItemId;
return this;
}
/**
* The ID of the taxation item of the invoice, from which the credit or debit memo is created. This field is only applicable when the `type` of the billing document is `credit_memo` and `debit_memo`.
* @return sourceTaxItemId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The ID of the taxation item of the invoice, from which the credit or debit memo is created. This field is only applicable when the `type` of the billing document is `credit_memo` and `debit_memo`.")
public String getSourceTaxItemId() {
return sourceTaxItemId;
}
public void setSourceTaxItemId(String sourceTaxItemId) {
this.sourceTaxItemId = sourceTaxItemId;
}
/**
* The remaining balance of the taxation item.
* @return remainingBalance
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The remaining balance of the taxation item.")
public BigDecimal getRemainingBalance() {
return remainingBalance;
}
/**
* The credit memo amount applied to the taxation item.
* @return amountCredited
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The credit memo amount applied to the taxation item.")
public BigDecimal getAmountCredited() {
return amountCredited;
}
/**
* The payment amount applied to the taxation item.
* @return amountPaid
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The payment amount applied to the taxation item.")
public BigDecimal getAmountPaid() {
return amountPaid;
}
/**
* The refund amount applied to the taxation item.
* @return amountRefunded
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The refund amount applied to the taxation item.")
public BigDecimal getAmountRefunded() {
return amountRefunded;
}
/**
* The credit memo item amount applied to the taxation item.
* @return amountApplied
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The credit memo item amount applied to the taxation item.")
public BigDecimal getAmountApplied() {
return amountApplied;
}
public TaxationItem salesTaxPayableAccount(String salesTaxPayableAccount) {
this.salesTaxPayableAccount = salesTaxPayableAccount;
return this;
}
/**
* An active account in your Zuora Chart of Accounts.
* @return salesTaxPayableAccount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An active account in your Zuora Chart of Accounts.")
public String getSalesTaxPayableAccount() {
return salesTaxPayableAccount;
}
public void setSalesTaxPayableAccount(String salesTaxPayableAccount) {
this.salesTaxPayableAccount = salesTaxPayableAccount;
}
public TaxationItem onAccountAccount(String onAccountAccount) {
this.onAccountAccount = onAccountAccount;
return this;
}
/**
* An active account in your Zuora Chart of Accounts.
* @return onAccountAccount
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An active account in your Zuora Chart of Accounts.")
public String getOnAccountAccount() {
return onAccountAccount;
}
public void setOnAccountAccount(String onAccountAccount) {
this.onAccountAccount = onAccountAccount;
}
public TaxationItem taxInclusive(Boolean taxInclusive) {
this.taxInclusive = taxInclusive;
return this;
}
/**
* If set to `true`, it indicates that amounts are inclusive of tax.
* @return taxInclusive
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "If set to `true`, it indicates that amounts are inclusive of tax.")
public Boolean getTaxInclusive() {
return taxInclusive;
}
public void setTaxInclusive(Boolean taxInclusive) {
this.taxInclusive = taxInclusive;
}
public TaxationItem taxRateType(TaxRateTypeEnum taxRateType) {
this.taxRateType = taxRateType;
return this;
}
/**
* Indicates whether the tax rate is an amount or a percentage.
* @return taxRateType
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Indicates whether the tax rate is an amount or a percentage.")
public TaxRateTypeEnum getTaxRateType() {
return taxRateType;
}
public void setTaxRateType(TaxRateTypeEnum taxRateType) {
this.taxRateType = taxRateType;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
TaxationItem taxationItem = (TaxationItem) o;
return Objects.equals(this.id, taxationItem.id) &&
Objects.equals(this.updatedById, taxationItem.updatedById) &&
Objects.equals(this.updatedTime, taxationItem.updatedTime) &&
Objects.equals(this.createdById, taxationItem.createdById) &&
Objects.equals(this.createdTime, taxationItem.createdTime) &&
Objects.equals(this.customFields, taxationItem.customFields) &&
Objects.equals(this.customObjects, taxationItem.customObjects) &&
Objects.equals(this.jurisdiction, taxationItem.jurisdiction) &&
Objects.equals(this.locationCode, taxationItem.locationCode) &&
Objects.equals(this.name, taxationItem.name) &&
Objects.equals(this.amount, taxationItem.amount) &&
Objects.equals(this.taxCode, taxationItem.taxCode) &&
Objects.equals(this.taxCodeName, taxationItem.taxCodeName) &&
Objects.equals(this.taxDate, taxationItem.taxDate) &&
Objects.equals(this.taxRate, taxationItem.taxRate) &&
Objects.equals(this.taxRateName, taxationItem.taxRateName) &&
Objects.equals(this.amountExempt, taxationItem.amountExempt) &&
Objects.equals(this.sourceTaxItemId, taxationItem.sourceTaxItemId) &&
Objects.equals(this.remainingBalance, taxationItem.remainingBalance) &&
Objects.equals(this.amountCredited, taxationItem.amountCredited) &&
Objects.equals(this.amountPaid, taxationItem.amountPaid) &&
Objects.equals(this.amountRefunded, taxationItem.amountRefunded) &&
Objects.equals(this.amountApplied, taxationItem.amountApplied) &&
Objects.equals(this.salesTaxPayableAccount, taxationItem.salesTaxPayableAccount) &&
Objects.equals(this.onAccountAccount, taxationItem.onAccountAccount) &&
Objects.equals(this.taxInclusive, taxationItem.taxInclusive) &&
Objects.equals(this.taxRateType, taxationItem.taxRateType);
}
@Override
public int hashCode() {
return Objects.hash(id, updatedById, updatedTime, createdById, createdTime, customFields, customObjects, jurisdiction, locationCode, name, amount, taxCode, taxCodeName, taxDate, taxRate, taxRateName, amountExempt, sourceTaxItemId, remainingBalance, amountCredited, amountPaid, amountRefunded, amountApplied, salesTaxPayableAccount, onAccountAccount, taxInclusive, taxRateType);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class TaxationItem {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" updatedById: ").append(toIndentedString(updatedById)).append("\n");
sb.append(" updatedTime: ").append(toIndentedString(updatedTime)).append("\n");
sb.append(" createdById: ").append(toIndentedString(createdById)).append("\n");
sb.append(" createdTime: ").append(toIndentedString(createdTime)).append("\n");
sb.append(" customFields: ").append(toIndentedString(customFields)).append("\n");
sb.append(" customObjects: ").append(toIndentedString(customObjects)).append("\n");
sb.append(" jurisdiction: ").append(toIndentedString(jurisdiction)).append("\n");
sb.append(" locationCode: ").append(toIndentedString(locationCode)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" amount: ").append(toIndentedString(amount)).append("\n");
sb.append(" taxCode: ").append(toIndentedString(taxCode)).append("\n");
sb.append(" taxCodeName: ").append(toIndentedString(taxCodeName)).append("\n");
sb.append(" taxDate: ").append(toIndentedString(taxDate)).append("\n");
sb.append(" taxRate: ").append(toIndentedString(taxRate)).append("\n");
sb.append(" taxRateName: ").append(toIndentedString(taxRateName)).append("\n");
sb.append(" amountExempt: ").append(toIndentedString(amountExempt)).append("\n");
sb.append(" sourceTaxItemId: ").append(toIndentedString(sourceTaxItemId)).append("\n");
sb.append(" remainingBalance: ").append(toIndentedString(remainingBalance)).append("\n");
sb.append(" amountCredited: ").append(toIndentedString(amountCredited)).append("\n");
sb.append(" amountPaid: ").append(toIndentedString(amountPaid)).append("\n");
sb.append(" amountRefunded: ").append(toIndentedString(amountRefunded)).append("\n");
sb.append(" amountApplied: ").append(toIndentedString(amountApplied)).append("\n");
sb.append(" salesTaxPayableAccount: ").append(toIndentedString(salesTaxPayableAccount)).append("\n");
sb.append(" onAccountAccount: ").append(toIndentedString(onAccountAccount)).append("\n");
sb.append(" taxInclusive: ").append(toIndentedString(taxInclusive)).append("\n");
sb.append(" taxRateType: ").append(toIndentedString(taxRateType)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy