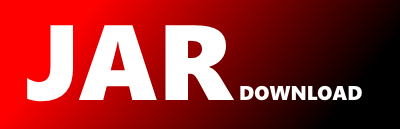
org.openapitools.client.api.OrderLineItemsApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zuora-sdk-java Show documentation
Show all versions of zuora-sdk-java Show documentation
The SDK of JAVA language for Zuora pricing system
The newest version!
/*
* Quickstart API Reference
* Zuora Quickstart API is the API that helps you achieve fundamental use cases.
* It provides a much simplified object model and improved performance, enabling developers to easily learn and use.
*/
package org.openapitools.client.api;
import org.openapitools.client.ApiCallback;
import org.openapitools.client.ApiClient;
import org.openapitools.client.ApiException;
import org.openapitools.client.model.Headers;
import org.openapitools.client.model.ListQueryParams;
import org.openapitools.client.model.GetByIdQueryParams;
import org.openapitools.client.ApiResponse;
import org.openapitools.client.Configuration;
import org.openapitools.client.Pair;
import org.openapitools.client.ProgressRequestBody;
import org.openapitools.client.ProgressResponseBody;
import com.google.gson.reflect.TypeToken;
import java.io.IOException;
import java.math.BigDecimal;
import org.openapitools.client.model.ErrorResponse;
import org.openapitools.client.model.LineItemPatchRequest;
import org.openapitools.client.model.OrderLineItem;
import org.openapitools.client.model.ErrorResponse;
import java.lang.reflect.Type;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class OrderLineItemsApi {
private ApiClient localVarApiClient;
private int localHostIndex;
private String localCustomBaseUrl;
public OrderLineItemsApi() {
this(Configuration.getDefaultApiClient());
}
public OrderLineItemsApi(ApiClient apiClient) {
this.localVarApiClient = apiClient;
}
public ApiClient getApiClient() {
return localVarApiClient;
}
public void setApiClient(ApiClient apiClient) {
this.localVarApiClient = apiClient;
}
public int getHostIndex() {
return localHostIndex;
}
public void setHostIndex(int hostIndex) {
this.localHostIndex = hostIndex;
}
public String getCustomBaseUrl() {
return localCustomBaseUrl;
}
public void setCustomBaseUrl(String customBaseUrl) {
this.localCustomBaseUrl = customBaseUrl;
}
private okhttp3.Call getOrderLineItemCall(String orderLineItemId, List fields, List lineItemFields, List invoiceItemsFields, List expand, List filter, Integer pageSize, String zuoraTrackId, Boolean async, Boolean zuoraCacheEnabled, String zuoraEntityIds, String idempotencyKey, String acceptEncoding, String contentEncoding, String zuoraOrgIds, final ApiCallback _callback) throws ApiException {
String basePath = null;
// Operation Servers
String[] localBasePaths = new String[] { };
// Determine Base Path to Use
if (localCustomBaseUrl != null){
basePath = localCustomBaseUrl;
} else if ( localBasePaths.length > 0 ) {
basePath = localBasePaths[localHostIndex];
} else {
basePath = null;
}
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/order_line_items/{order_line_item_id}"
.replace("{" + "order_line_item_id" + "}", localVarApiClient.escapeString(orderLineItemId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
if (fields != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "fields[]", fields));
}
if (lineItemFields != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "line_item.fields[]", lineItemFields));
}
if (invoiceItemsFields != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "invoice_items.fields[]", invoiceItemsFields));
}
if (expand != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "expand[]", expand));
}
if (filter != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "filter[]", filter));
}
if (pageSize != null) {
localVarQueryParams.addAll(localVarApiClient.parameterToPair("page_size", pageSize));
}
if (zuoraTrackId != null) {
localVarHeaderParams.put("zuora-track-id", localVarApiClient.parameterToString(zuoraTrackId));
}
if (async != null) {
localVarHeaderParams.put("async", localVarApiClient.parameterToString(async));
}
if (zuoraCacheEnabled != null) {
localVarHeaderParams.put("zuora-cache-enabled", localVarApiClient.parameterToString(zuoraCacheEnabled));
}
if (zuoraEntityIds != null) {
localVarHeaderParams.put("zuora-entity-ids", localVarApiClient.parameterToString(zuoraEntityIds));
}
if (idempotencyKey != null) {
localVarHeaderParams.put("idempotency-key", localVarApiClient.parameterToString(idempotencyKey));
}
if (acceptEncoding != null) {
localVarHeaderParams.put("accept-encoding", localVarApiClient.parameterToString(acceptEncoding));
}
if (contentEncoding != null) {
localVarHeaderParams.put("content-encoding", localVarApiClient.parameterToString(contentEncoding));
}
if (zuoraOrgIds != null) {
localVarHeaderParams.put("zuora-org-ids", localVarApiClient.parameterToString(zuoraOrgIds));
}
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = localVarApiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
final String[] localVarContentTypes = {
};
final String localVarContentType = localVarApiClient.selectHeaderContentType(localVarContentTypes);
if (localVarContentType != null) {
localVarHeaderParams.put("Content-Type", localVarContentType);
}
String[] localVarAuthNames = new String[] { "bearerAuth" };
return localVarApiClient.buildCall(basePath, localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarCookieParams, localVarFormParams, localVarAuthNames, _callback);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call getOrderLineItemValidateBeforeCall(String orderLineItemId, List fields, List lineItemFields, List invoiceItemsFields, List expand, List filter, Integer pageSize, String zuoraTrackId, Boolean async, Boolean zuoraCacheEnabled, String zuoraEntityIds, String idempotencyKey, String acceptEncoding, String contentEncoding, String zuoraOrgIds, final ApiCallback _callback) throws ApiException {
// verify the required parameter 'orderLineItemId' is set
if (orderLineItemId == null) {
throw new ApiException("Missing the required parameter 'orderLineItemId' when calling getOrderLineItem(Async)");
}
return getOrderLineItemCall(orderLineItemId, fields, lineItemFields, invoiceItemsFields, expand, filter, pageSize, zuoraTrackId, async, zuoraCacheEnabled, zuoraEntityIds, idempotencyKey, acceptEncoding, contentEncoding, zuoraOrgIds, _callback);
}
private ApiResponse getOrderLineItemWithHttpInfo(String orderLineItemId, List fields, List lineItemFields, List invoiceItemsFields, List expand, List filter, Integer pageSize, String zuoraTrackId, Boolean async, Boolean zuoraCacheEnabled, String zuoraEntityIds, String idempotencyKey, String acceptEncoding, String contentEncoding, String zuoraOrgIds) throws ApiException {
okhttp3.Call localVarCall = getOrderLineItemValidateBeforeCall(orderLineItemId, fields, lineItemFields, invoiceItemsFields, expand, filter, pageSize, zuoraTrackId, async, zuoraCacheEnabled, zuoraEntityIds, idempotencyKey, acceptEncoding, contentEncoding, zuoraOrgIds, null);
try {
Type localVarReturnType = new TypeToken(){}.getType();
return localVarApiClient.execute(localVarCall, localVarReturnType);
} catch (ApiException e) {
e.setErrorObject(localVarApiClient.getJSON().getGson().fromJson(e.getResponseBody(), new TypeToken(){}.getType()));
throw e;
}
}
private okhttp3.Call getOrderLineItemAsync(String orderLineItemId, List fields, List lineItemFields, List invoiceItemsFields, List expand, List filter, Integer pageSize, String zuoraTrackId, Boolean async, Boolean zuoraCacheEnabled, String zuoraEntityIds, String idempotencyKey, String acceptEncoding, String contentEncoding, String zuoraOrgIds, final ApiCallback _callback) throws ApiException {
okhttp3.Call localVarCall = getOrderLineItemValidateBeforeCall(orderLineItemId, fields, lineItemFields, invoiceItemsFields, expand, filter, pageSize, zuoraTrackId, async, zuoraCacheEnabled, zuoraEntityIds, idempotencyKey, acceptEncoding, contentEncoding, zuoraOrgIds, _callback);
Type localVarReturnType = new TypeToken(){}.getType();
localVarApiClient.executeAsync(localVarCall, localVarReturnType, _callback);
return localVarCall;
}
public static class GetOrderLineItemParams {
private final String orderLineItemId;
private Headers headers;
/*
* Check for list params
*/
private GetByIdQueryParams getByIdQueryParams;
private List fields;
private List lineItemFields;
private List invoiceItemsFields;
private List expand;
private List filter;
private Integer pageSize;
private String zuoraTrackId;
private Boolean async;
private Boolean zuoraCacheEnabled;
private String zuoraEntityIds;
private String idempotencyKey;
private String acceptEncoding;
private String contentEncoding;
private String zuoraOrgIds;
public GetOrderLineItemParams(String orderLineItemId) {
this.orderLineItemId = orderLineItemId;
}
/**
* Set getByIdQueryParams
* @param getByIdQueryParams GetByIdQueryParams
* @return GetOrderLineItemParams
*/
public GetOrderLineItemParams getByIdQueryParams(GetByIdQueryParams getByIdQueryParams) {
this.getByIdQueryParams = getByIdQueryParams;
if (expand != null) {
this.expand = getByIdQueryParams.getExpand();
}
if (filter != null) {
this.filter = getByIdQueryParams.getFilter();
}
if (pageSize != null) {
this.pageSize = getByIdQueryParams.getPageSize();
}
return this;
}
/**
* Set headers
* @param headers Headers
* @return GetOrderLineItemParams
*/
public GetOrderLineItemParams headers(Headers headers) {
this.headers = headers;
if (headers.getZuoraTrackId() != null) {
this.zuoraTrackId = headers.getZuoraTrackId();
}
if (headers.getAsync() != null) {
this.async = headers.getAsync();
}
if (headers.getZuoraCacheEnabled() != null) {
this.zuoraCacheEnabled = headers.getZuoraCacheEnabled();
}
if (headers.getZuoraEntityIds() != null) {
this.zuoraEntityIds = headers.getZuoraEntityIds();
}
if (headers.getIdempotencyKey() != null) {
this.idempotencyKey = headers.getIdempotencyKey();
}
if (headers.getAcceptEncoding() != null) {
this.acceptEncoding = headers.getAcceptEncoding();
}
if (headers.getContentEncoding() != null) {
this.contentEncoding = headers.getContentEncoding();
}
if (headers.getZuoraOrgIds() != null) {
this.zuoraOrgIds = headers.getZuoraOrgIds();
}
return this;
}
/**
* Set fields
* @param fields Allows you to specify which fields are returned in the response. <details> <summary> Accepted values </summary> `custom_fields`, `created_by_id`, `updated_by_id`, `created_time`, `id`, `updated_time`, `total`, `subtotal`, `quantity_fulfilled`, `quantity_pending_fulfillment`, `unit_of_measure`, `accounting_code`, `adjustment_liability_account`, `unit_amount`, `target_date`, `billing_rule`, `contract_asset_account`, `contract_liability_account`, `description`, `discount_total`, `revenue`, `discount_unit_amount`, `discount_percent`, `category`, `name`, `item_number`, `type`, `list_price`, `list_unit_price`, `original_order_date`, `original_order_id`, `original_order_line_item_id`, `original_order_line_item_number`, `original_order_number`, `product_code`, `price_id`, `purchase_order_number`, `quantity`, `quantity_available_for_return`, `related_subscription_number`, `requires_fulfillment`, `sold_to_id`, `original_sold_to_id`, `tax_code`, `tax_inclusive`, `end_date`, `start_date`, `unbilled_receivables_account`, `state`, `order_id` </details> (optional)
* @return GetOrderLineItemParams
*/
public GetOrderLineItemParams fields(List fields) {
this.fields = fields;
return this;
}
/**
* Set lineItemFields
* @param lineItemFields Allows you to specify which fields are returned in the response. <details> <summary> Accepted values </summary> `custom_fields`, `created_by_id`, `updated_by_id`, `created_time`, `id`, `updated_time`, `total`, `subtotal`, `quantity_fulfilled`, `quantity_pending_fulfillment`, `unit_of_measure`, `accounting_code`, `adjustment_liability_account`, `unit_amount`, `target_date`, `billing_rule`, `contract_asset_account`, `contract_liability_account`, `description`, `discount_total`, `revenue`, `discount_unit_amount`, `discount_percent`, `category`, `name`, `item_number`, `type`, `list_price`, `list_unit_price`, `original_order_date`, `original_order_id`, `original_order_line_item_id`, `original_order_line_item_number`, `original_order_number`, `product_code`, `price_id`, `purchase_order_number`, `quantity`, `quantity_available_for_return`, `related_subscription_number`, `requires_fulfillment`, `sold_to_id`, `original_sold_to_id`, `tax_code`, `tax_inclusive`, `end_date`, `start_date`, `unbilled_receivables_account`, `state`, `order_id` </details> (optional)
* @return GetOrderLineItemParams
*/
public GetOrderLineItemParams lineItemFields(List lineItemFields) {
this.lineItemFields = lineItemFields;
return this;
}
/**
* Set invoiceItemsFields
* @param invoiceItemsFields Allows you to specify which fields are returned in the response. <details> <summary> Accepted values </summary> `custom_fields`, `created_by_id`, `updated_by_id`, `created_time`, `id`, `updated_time`, `amount`, `booking_reference`, `applied_to_item_id`, `price_id`, `discount_item`, `deferred_revenue_account`, `description`, `invoice_id`, `sku`, `name`, `purchase_order_number`, `quantity`, `recognized_revenue_account`, `remaining_balance`, `revenue_recognition_rule_name`, `accounting_code`, `service_end`, `service_start`, `accounts_receivable_account`, `subscription_id`, `subscription_item_id`, `tax`, `tax_code`, `tax_inclusive`, `unit_amount`, `unit_of_measure`, `document_item_date` </details> (optional)
* @return GetOrderLineItemParams
*/
public GetOrderLineItemParams invoiceItemsFields(List invoiceItemsFields) {
this.invoiceItemsFields = invoiceItemsFields;
return this;
}
/**
* Set expand
* @param expand Allows you to expand responses by including related object information in a single call. See the [Expand responses](https://developer.zuora.com/quickstart-api/tutorial/expand-responses/) section of the Quickstart API Tutorials for detailed instructions. (optional)
* @return GetOrderLineItemParams
*/
public GetOrderLineItemParams expand(List expand) {
this.expand = expand;
return this;
}
/**
* Set filter
* @param filter A case-sensitive filter on the list. See the [Filter lists](https://developer.zuora.com/quickstart-api/tutorial/filter-lists/) section of the Quickstart API Tutorial for detailed instructions. Note that the filters on this operation are only applicable to the related objects. For example, when you are calling the \"Retrieve a billing document\" operation, you can use the `filter[]` parameter on the related objects such as `filter[]=items[account_id].EQ:8ad09e208858b5cf0188595208151c63` (optional)
* @return GetOrderLineItemParams
*/
public GetOrderLineItemParams filter(List filter) {
this.filter = filter;
return this;
}
/**
* Set pageSize
* @param pageSize The maximum number of results to return in a single page. If the specified `page_size` is less than 1 or greater than 99, Zuora will return a 400 error. (optional)
* @return GetOrderLineItemParams
*/
public GetOrderLineItemParams pageSize(Integer pageSize) {
this.pageSize = pageSize;
return this;
}
}
/**
* Retrieve an order line item
* Use this operation to retrieve the detailed information about a specific order line item.
* @param orderLineItemId Identifier for the order line item. (required)
* @return OrderLineItem
* @http.response.details
Status Code Description Response Headers
200 Default Response * ratelimit-limit - The request limit quota for the time window closest to exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* ratelimit-remaining - The number of requests remaining in the time window closest to quota exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* ratelimit-reset - The number of seconds until the quota resets for the time window closest to quota exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* zuora-request-id - Zuora’s internal identifier for this request.
* zuora-track-id - A user-supplied identifier for this request. If you supply a `zuora-track-id` as a request header, Zuora returns the `zuora-track-id` as a response header.
400 Bad Request -
401 Unauthorized -
404 Not Found -
405 Method Not Allowed -
429 Too Many Requests -
500 Internal Server Error -
502 Bad Gateway -
503 Service Unavailable -
504 Gateway Timeout -
*/
public GetOrderLineItemParams getOrderLineItemParams(String orderLineItemId) {
return new GetOrderLineItemParams(orderLineItemId);
}
public OrderLineItem getOrderLineItem(String orderLineItemId) throws ApiException {
GetOrderLineItemParams params = new GetOrderLineItemParams(orderLineItemId);
return executeGetOrderLineItemAPICall(params).getData();
}
/**
* Expose HTTP info
public ApiResponse getOrderLineItemWithHttpInfo(String orderLineItemId) throws ApiException {
GetOrderLineItemParams params = new GetOrderLineItemParams(orderLineItemId);
return executeGetOrderLineItemAPICall(params);
}
**/
/**
Legacy Query Params Requests
**/
public OrderLineItem getOrderLineItem( String orderLineItemId , List expand) throws ApiException {
GetOrderLineItemParams params = new GetOrderLineItemParams(orderLineItemId);
params.expand(expand);
return executeGetOrderLineItemAPICall(params).getData();
}
/*
* add header methods
*/
public OrderLineItem getOrderLineItem(String orderLineItemId ,List expand,Headers headers) throws ApiException {
GetOrderLineItemParams params = new GetOrderLineItemParams(orderLineItemId)
.expand(expand)
.headers(headers);
return executeGetOrderLineItemAPICall(params).getData();
}
/**
* Retrieve an order line item
* Use this operation to retrieve the detailed information about a specific order line item.
* @param params GetOrderLineItemParams
* @return OrderLineItem
* @http.response.details
Status Code Description Response Headers
200 Default Response * ratelimit-limit - The request limit quota for the time window closest to exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* ratelimit-remaining - The number of requests remaining in the time window closest to quota exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* ratelimit-reset - The number of seconds until the quota resets for the time window closest to quota exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* zuora-request-id - Zuora’s internal identifier for this request.
* zuora-track-id - A user-supplied identifier for this request. If you supply a `zuora-track-id` as a request header, Zuora returns the `zuora-track-id` as a response header.
400 Bad Request -
401 Unauthorized -
404 Not Found -
405 Method Not Allowed -
429 Too Many Requests -
500 Internal Server Error -
502 Bad Gateway -
503 Service Unavailable -
504 Gateway Timeout -
*/
public OrderLineItem getOrderLineItem(GetOrderLineItemParams params) throws ApiException {
return executeGetOrderLineItemAPICall(params).getData();
}
public ApiResponse getOrderLineItemWithHttpInfo(GetOrderLineItemParams params) throws ApiException {
return executeGetOrderLineItemAPICall(params);
}
ApiResponse executeGetOrderLineItemAPICall(GetOrderLineItemParams params) throws ApiException {
return getOrderLineItemWithHttpInfo(params.orderLineItemId, params.fields, params.lineItemFields, params.invoiceItemsFields, params.expand, params.filter, params.pageSize, params.zuoraTrackId, params.async, params.zuoraCacheEnabled, params.zuoraEntityIds, params.idempotencyKey, params.acceptEncoding, params.contentEncoding, params.zuoraOrgIds);
}
private okhttp3.Call patchOrderLineItemCall(String orderLineItemId, LineItemPatchRequest lineItemPatchRequest, List fields, List lineItemFields, List invoiceItemsFields, List expand, List filter, Integer pageSize, String zuoraTrackId, Boolean async, Boolean zuoraCacheEnabled, String zuoraEntityIds, String idempotencyKey, String acceptEncoding, String contentEncoding, String zuoraOrgIds, final ApiCallback _callback) throws ApiException {
String basePath = null;
// Operation Servers
String[] localBasePaths = new String[] { };
// Determine Base Path to Use
if (localCustomBaseUrl != null){
basePath = localCustomBaseUrl;
} else if ( localBasePaths.length > 0 ) {
basePath = localBasePaths[localHostIndex];
} else {
basePath = null;
}
Object localVarPostBody = lineItemPatchRequest;
// create path and map variables
String localVarPath = "/order_line_items/{order_line_item_id}"
.replace("{" + "order_line_item_id" + "}", localVarApiClient.escapeString(orderLineItemId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
if (fields != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "fields[]", fields));
}
if (lineItemFields != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "line_item.fields[]", lineItemFields));
}
if (invoiceItemsFields != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "invoice_items.fields[]", invoiceItemsFields));
}
if (expand != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "expand[]", expand));
}
if (filter != null) {
localVarCollectionQueryParams.addAll(localVarApiClient.parameterToPairs("multi", "filter[]", filter));
}
if (pageSize != null) {
localVarQueryParams.addAll(localVarApiClient.parameterToPair("page_size", pageSize));
}
if (zuoraTrackId != null) {
localVarHeaderParams.put("zuora-track-id", localVarApiClient.parameterToString(zuoraTrackId));
}
if (async != null) {
localVarHeaderParams.put("async", localVarApiClient.parameterToString(async));
}
if (zuoraCacheEnabled != null) {
localVarHeaderParams.put("zuora-cache-enabled", localVarApiClient.parameterToString(zuoraCacheEnabled));
}
if (zuoraEntityIds != null) {
localVarHeaderParams.put("zuora-entity-ids", localVarApiClient.parameterToString(zuoraEntityIds));
}
if (idempotencyKey != null) {
localVarHeaderParams.put("idempotency-key", localVarApiClient.parameterToString(idempotencyKey));
}
if (acceptEncoding != null) {
localVarHeaderParams.put("accept-encoding", localVarApiClient.parameterToString(acceptEncoding));
}
if (contentEncoding != null) {
localVarHeaderParams.put("content-encoding", localVarApiClient.parameterToString(contentEncoding));
}
if (zuoraOrgIds != null) {
localVarHeaderParams.put("zuora-org-ids", localVarApiClient.parameterToString(zuoraOrgIds));
}
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = localVarApiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = localVarApiClient.selectHeaderContentType(localVarContentTypes);
if (localVarContentType != null) {
localVarHeaderParams.put("Content-Type", localVarContentType);
}
String[] localVarAuthNames = new String[] { "bearerAuth" };
return localVarApiClient.buildCall(basePath, localVarPath, "PATCH", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarCookieParams, localVarFormParams, localVarAuthNames, _callback);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call patchOrderLineItemValidateBeforeCall(String orderLineItemId, LineItemPatchRequest lineItemPatchRequest, List fields, List lineItemFields, List invoiceItemsFields, List expand, List filter, Integer pageSize, String zuoraTrackId, Boolean async, Boolean zuoraCacheEnabled, String zuoraEntityIds, String idempotencyKey, String acceptEncoding, String contentEncoding, String zuoraOrgIds, final ApiCallback _callback) throws ApiException {
// verify the required parameter 'orderLineItemId' is set
if (orderLineItemId == null) {
throw new ApiException("Missing the required parameter 'orderLineItemId' when calling patchOrderLineItem(Async)");
}
// verify the required parameter 'lineItemPatchRequest' is set
if (lineItemPatchRequest == null) {
throw new ApiException("Missing the required parameter 'lineItemPatchRequest' when calling patchOrderLineItem(Async)");
}
return patchOrderLineItemCall(orderLineItemId, lineItemPatchRequest, fields, lineItemFields, invoiceItemsFields, expand, filter, pageSize, zuoraTrackId, async, zuoraCacheEnabled, zuoraEntityIds, idempotencyKey, acceptEncoding, contentEncoding, zuoraOrgIds, _callback);
}
private ApiResponse patchOrderLineItemWithHttpInfo(String orderLineItemId, LineItemPatchRequest lineItemPatchRequest, List fields, List lineItemFields, List invoiceItemsFields, List expand, List filter, Integer pageSize, String zuoraTrackId, Boolean async, Boolean zuoraCacheEnabled, String zuoraEntityIds, String idempotencyKey, String acceptEncoding, String contentEncoding, String zuoraOrgIds) throws ApiException {
okhttp3.Call localVarCall = patchOrderLineItemValidateBeforeCall(orderLineItemId, lineItemPatchRequest, fields, lineItemFields, invoiceItemsFields, expand, filter, pageSize, zuoraTrackId, async, zuoraCacheEnabled, zuoraEntityIds, idempotencyKey, acceptEncoding, contentEncoding, zuoraOrgIds, null);
try {
Type localVarReturnType = new TypeToken(){}.getType();
return localVarApiClient.execute(localVarCall, localVarReturnType);
} catch (ApiException e) {
e.setErrorObject(localVarApiClient.getJSON().getGson().fromJson(e.getResponseBody(), new TypeToken(){}.getType()));
throw e;
}
}
private okhttp3.Call patchOrderLineItemAsync(String orderLineItemId, LineItemPatchRequest lineItemPatchRequest, List fields, List lineItemFields, List invoiceItemsFields, List expand, List filter, Integer pageSize, String zuoraTrackId, Boolean async, Boolean zuoraCacheEnabled, String zuoraEntityIds, String idempotencyKey, String acceptEncoding, String contentEncoding, String zuoraOrgIds, final ApiCallback _callback) throws ApiException {
okhttp3.Call localVarCall = patchOrderLineItemValidateBeforeCall(orderLineItemId, lineItemPatchRequest, fields, lineItemFields, invoiceItemsFields, expand, filter, pageSize, zuoraTrackId, async, zuoraCacheEnabled, zuoraEntityIds, idempotencyKey, acceptEncoding, contentEncoding, zuoraOrgIds, _callback);
Type localVarReturnType = new TypeToken(){}.getType();
localVarApiClient.executeAsync(localVarCall, localVarReturnType, _callback);
return localVarCall;
}
public static class PatchOrderLineItemParams {
private final String orderLineItemId;
private final LineItemPatchRequest lineItemPatchRequest;
private Headers headers;
/*
* Check for list params
*/
private GetByIdQueryParams getByIdQueryParams;
private List fields;
private List lineItemFields;
private List invoiceItemsFields;
private List expand;
private List filter;
private Integer pageSize;
private String zuoraTrackId;
private Boolean async;
private Boolean zuoraCacheEnabled;
private String zuoraEntityIds;
private String idempotencyKey;
private String acceptEncoding;
private String contentEncoding;
private String zuoraOrgIds;
public PatchOrderLineItemParams(String orderLineItemId, LineItemPatchRequest lineItemPatchRequest) {
this.orderLineItemId = orderLineItemId;
this.lineItemPatchRequest = lineItemPatchRequest;
}
/**
* Set getByIdQueryParams
* @param getByIdQueryParams GetByIdQueryParams
* @return PatchOrderLineItemParams
*/
public PatchOrderLineItemParams getByIdQueryParams(GetByIdQueryParams getByIdQueryParams) {
this.getByIdQueryParams = getByIdQueryParams;
if (expand != null) {
this.expand = getByIdQueryParams.getExpand();
}
if (filter != null) {
this.filter = getByIdQueryParams.getFilter();
}
if (pageSize != null) {
this.pageSize = getByIdQueryParams.getPageSize();
}
return this;
}
/**
* Set headers
* @param headers Headers
* @return PatchOrderLineItemParams
*/
public PatchOrderLineItemParams headers(Headers headers) {
this.headers = headers;
if (headers.getZuoraTrackId() != null) {
this.zuoraTrackId = headers.getZuoraTrackId();
}
if (headers.getAsync() != null) {
this.async = headers.getAsync();
}
if (headers.getZuoraCacheEnabled() != null) {
this.zuoraCacheEnabled = headers.getZuoraCacheEnabled();
}
if (headers.getZuoraEntityIds() != null) {
this.zuoraEntityIds = headers.getZuoraEntityIds();
}
if (headers.getIdempotencyKey() != null) {
this.idempotencyKey = headers.getIdempotencyKey();
}
if (headers.getAcceptEncoding() != null) {
this.acceptEncoding = headers.getAcceptEncoding();
}
if (headers.getContentEncoding() != null) {
this.contentEncoding = headers.getContentEncoding();
}
if (headers.getZuoraOrgIds() != null) {
this.zuoraOrgIds = headers.getZuoraOrgIds();
}
return this;
}
/**
* Set fields
* @param fields Allows you to specify which fields are returned in the response. <details> <summary> Accepted values </summary> `custom_fields`, `created_by_id`, `updated_by_id`, `created_time`, `id`, `updated_time`, `total`, `subtotal`, `quantity_fulfilled`, `quantity_pending_fulfillment`, `unit_of_measure`, `accounting_code`, `adjustment_liability_account`, `unit_amount`, `target_date`, `billing_rule`, `contract_asset_account`, `contract_liability_account`, `description`, `discount_total`, `revenue`, `discount_unit_amount`, `discount_percent`, `category`, `name`, `item_number`, `type`, `list_price`, `list_unit_price`, `original_order_date`, `original_order_id`, `original_order_line_item_id`, `original_order_line_item_number`, `original_order_number`, `product_code`, `price_id`, `purchase_order_number`, `quantity`, `quantity_available_for_return`, `related_subscription_number`, `requires_fulfillment`, `sold_to_id`, `original_sold_to_id`, `tax_code`, `tax_inclusive`, `end_date`, `start_date`, `unbilled_receivables_account`, `state`, `order_id` </details> (optional)
* @return PatchOrderLineItemParams
*/
public PatchOrderLineItemParams fields(List fields) {
this.fields = fields;
return this;
}
/**
* Set lineItemFields
* @param lineItemFields Allows you to specify which fields are returned in the response. <details> <summary> Accepted values </summary> `custom_fields`, `created_by_id`, `updated_by_id`, `created_time`, `id`, `updated_time`, `total`, `subtotal`, `quantity_fulfilled`, `quantity_pending_fulfillment`, `unit_of_measure`, `accounting_code`, `adjustment_liability_account`, `unit_amount`, `target_date`, `billing_rule`, `contract_asset_account`, `contract_liability_account`, `description`, `discount_total`, `revenue`, `discount_unit_amount`, `discount_percent`, `category`, `name`, `item_number`, `type`, `list_price`, `list_unit_price`, `original_order_date`, `original_order_id`, `original_order_line_item_id`, `original_order_line_item_number`, `original_order_number`, `product_code`, `price_id`, `purchase_order_number`, `quantity`, `quantity_available_for_return`, `related_subscription_number`, `requires_fulfillment`, `sold_to_id`, `original_sold_to_id`, `tax_code`, `tax_inclusive`, `end_date`, `start_date`, `unbilled_receivables_account`, `state`, `order_id` </details> (optional)
* @return PatchOrderLineItemParams
*/
public PatchOrderLineItemParams lineItemFields(List lineItemFields) {
this.lineItemFields = lineItemFields;
return this;
}
/**
* Set invoiceItemsFields
* @param invoiceItemsFields Allows you to specify which fields are returned in the response. <details> <summary> Accepted values </summary> `custom_fields`, `created_by_id`, `updated_by_id`, `created_time`, `id`, `updated_time`, `amount`, `booking_reference`, `applied_to_item_id`, `price_id`, `discount_item`, `deferred_revenue_account`, `description`, `invoice_id`, `sku`, `name`, `purchase_order_number`, `quantity`, `recognized_revenue_account`, `remaining_balance`, `revenue_recognition_rule_name`, `accounting_code`, `service_end`, `service_start`, `accounts_receivable_account`, `subscription_id`, `subscription_item_id`, `tax`, `tax_code`, `tax_inclusive`, `unit_amount`, `unit_of_measure`, `document_item_date` </details> (optional)
* @return PatchOrderLineItemParams
*/
public PatchOrderLineItemParams invoiceItemsFields(List invoiceItemsFields) {
this.invoiceItemsFields = invoiceItemsFields;
return this;
}
/**
* Set expand
* @param expand Allows you to expand responses by including related object information in a single call. See the [Expand responses](https://developer.zuora.com/quickstart-api/tutorial/expand-responses/) section of the Quickstart API Tutorials for detailed instructions. (optional)
* @return PatchOrderLineItemParams
*/
public PatchOrderLineItemParams expand(List expand) {
this.expand = expand;
return this;
}
/**
* Set filter
* @param filter A case-sensitive filter on the list. See the [Filter lists](https://developer.zuora.com/quickstart-api/tutorial/filter-lists/) section of the Quickstart API Tutorial for detailed instructions. Note that the filters on this operation are only applicable to the related objects. For example, when you are calling the \"Retrieve a billing document\" operation, you can use the `filter[]` parameter on the related objects such as `filter[]=items[account_id].EQ:8ad09e208858b5cf0188595208151c63` (optional)
* @return PatchOrderLineItemParams
*/
public PatchOrderLineItemParams filter(List filter) {
this.filter = filter;
return this;
}
/**
* Set pageSize
* @param pageSize The maximum number of results to return in a single page. If the specified `page_size` is less than 1 or greater than 99, Zuora will return a 400 error. (optional)
* @return PatchOrderLineItemParams
*/
public PatchOrderLineItemParams pageSize(Integer pageSize) {
this.pageSize = pageSize;
return this;
}
}
/**
* Update an order line item
* Use this operation to update the information of a specific order line item.
* @param orderLineItemId Identifier for the order line item. (required)
* @param lineItemPatchRequest (required)
* @return OrderLineItem
* @http.response.details
Status Code Description Response Headers
200 Default Response * ratelimit-limit - The request limit quota for the time window closest to exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* ratelimit-remaining - The number of requests remaining in the time window closest to quota exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* ratelimit-reset - The number of seconds until the quota resets for the time window closest to quota exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* zuora-request-id - Zuora’s internal identifier for this request.
* zuora-track-id - A user-supplied identifier for this request. If you supply a `zuora-track-id` as a request header, Zuora returns the `zuora-track-id` as a response header.
400 Bad Request -
401 Unauthorized -
404 Not Found -
405 Method Not Allowed -
429 Too Many Requests -
500 Internal Server Error -
502 Bad Gateway -
503 Service Unavailable -
504 Gateway Timeout -
*/
public PatchOrderLineItemParams patchOrderLineItemParams(String orderLineItemId, LineItemPatchRequest lineItemPatchRequest) {
return new PatchOrderLineItemParams(orderLineItemId, lineItemPatchRequest);
}
public OrderLineItem patchOrderLineItem(String orderLineItemId, LineItemPatchRequest lineItemPatchRequest) throws ApiException {
PatchOrderLineItemParams params = new PatchOrderLineItemParams(orderLineItemId, lineItemPatchRequest);
return executePatchOrderLineItemAPICall(params).getData();
}
/**
* Expose HTTP info
public ApiResponse patchOrderLineItemWithHttpInfo(String orderLineItemId, LineItemPatchRequest lineItemPatchRequest) throws ApiException {
PatchOrderLineItemParams params = new PatchOrderLineItemParams(orderLineItemId, lineItemPatchRequest);
return executePatchOrderLineItemAPICall(params);
}
**/
/**
Legacy Query Params Requests
**/
public OrderLineItem patchOrderLineItem( String orderLineItemId , LineItemPatchRequest lineItemPatchRequest , List expand) throws ApiException {
PatchOrderLineItemParams params = new PatchOrderLineItemParams(orderLineItemId, lineItemPatchRequest);
params.expand(expand);
return executePatchOrderLineItemAPICall(params).getData();
}
/*
* add header methods
*/
public OrderLineItem patchOrderLineItem(String orderLineItemId , LineItemPatchRequest lineItemPatchRequest ,List expand,Headers headers) throws ApiException {
PatchOrderLineItemParams params = new PatchOrderLineItemParams(orderLineItemId, lineItemPatchRequest)
.expand(expand)
.headers(headers);
return executePatchOrderLineItemAPICall(params).getData();
}
/**
* Update an order line item
* Use this operation to update the information of a specific order line item.
* @param params PatchOrderLineItemParams
* @return OrderLineItem
* @http.response.details
Status Code Description Response Headers
200 Default Response * ratelimit-limit - The request limit quota for the time window closest to exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* ratelimit-remaining - The number of requests remaining in the time window closest to quota exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* ratelimit-reset - The number of seconds until the quota resets for the time window closest to quota exhaustion. See [rate limits](https://developer.zuora.com/rest-api/general-concepts/rate-concurrency-limits/#rate-limits) for more information.
* zuora-request-id - Zuora’s internal identifier for this request.
* zuora-track-id - A user-supplied identifier for this request. If you supply a `zuora-track-id` as a request header, Zuora returns the `zuora-track-id` as a response header.
400 Bad Request -
401 Unauthorized -
404 Not Found -
405 Method Not Allowed -
429 Too Many Requests -
500 Internal Server Error -
502 Bad Gateway -
503 Service Unavailable -
504 Gateway Timeout -
*/
public OrderLineItem patchOrderLineItem(PatchOrderLineItemParams params) throws ApiException {
return executePatchOrderLineItemAPICall(params).getData();
}
public ApiResponse patchOrderLineItemWithHttpInfo(PatchOrderLineItemParams params) throws ApiException {
return executePatchOrderLineItemAPICall(params);
}
ApiResponse executePatchOrderLineItemAPICall(PatchOrderLineItemParams params) throws ApiException {
return patchOrderLineItemWithHttpInfo(params.orderLineItemId, params.lineItemPatchRequest, params.fields, params.lineItemFields, params.invoiceItemsFields, params.expand, params.filter, params.pageSize, params.zuoraTrackId, params.async, params.zuoraCacheEnabled, params.zuoraEntityIds, params.idempotencyKey, params.acceptEncoding, params.contentEncoding, params.zuoraOrgIds);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy