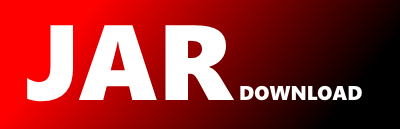
com.zuunr.restbed.repository.sqlserver.impl.SaveItem Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of restbed-repository-sqlserver Show documentation
Show all versions of restbed-repository-sqlserver Show documentation
SQL Server implementation of the reactive repository interface in core project
/*
* Copyright 2018 Zuunr AB
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.zuunr.restbed.repository.sqlserver.impl;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.jdbc.core.namedparam.MapSqlParameterSource;
import org.springframework.jdbc.core.namedparam.NamedParameterJdbcTemplate;
import org.springframework.stereotype.Component;
import com.zuunr.json.JsonObject;
import com.zuunr.json.JsonObjectSupport;
import com.zuunr.restbed.core.exchange.Exchange;
import com.zuunr.restbed.core.exchange.Response;
import com.zuunr.restbed.core.exchange.StatusCode;
import com.zuunr.restbed.repository.ReactiveRepository;
import com.zuunr.restbed.repository.sqlserver.util.CollectionNameProvider;
import com.zuunr.restbed.repository.sqlserver.util.EtagProvider;
import com.zuunr.restbed.repository.sqlserver.util.ResourceDeserializer;
import com.zuunr.restbed.repository.sqlserver.util.SchemaNameProvider;
import reactor.core.publisher.Mono;
import reactor.core.scheduler.Schedulers;
/**
* Primary saveItem implementation of {@link ReactiveRepository}.
*
* @see ReactiveRepository
*/
@Component
public class SaveItem {
private final Logger logger = LoggerFactory.getLogger(SaveItem.class);
private NamedParameterJdbcTemplate namedParameterJdbcTemplate;
private CollectionNameProvider collectionNameProvider;
private SchemaNameProvider schemaNameProvider;
private EtagProvider etagProvider;
private ResourceDeserializer resourceDeserializer;
@Autowired
public SaveItem(
NamedParameterJdbcTemplate namedParameterJdbcTemplate,
CollectionNameProvider collectionNameProvider,
SchemaNameProvider schemaNameProvider,
EtagProvider etagProvider,
ResourceDeserializer resourceDeserializer) {
this.namedParameterJdbcTemplate = namedParameterJdbcTemplate;
this.collectionNameProvider = collectionNameProvider;
this.schemaNameProvider = schemaNameProvider;
this.etagProvider = etagProvider;
this.resourceDeserializer = resourceDeserializer;
}
/**
* @see ReactiveRepository#saveItem(Mono, Class)
*/
public Mono> saveItem(Exchange exchange, Class resourceClass) {
String collectionName = collectionNameProvider.getCollectionName(exchange.getRequest());
String schemaName = schemaNameProvider.getSchemaName();
JsonObject body = exchange.getRequest().getBodyAsJsonObject();
JsonObject bodyWithEtag = etagProvider.appendEtag(body);
String sql = "INSERT INTO " + schemaName + "." + collectionName + " (document) values (:document)";
MapSqlParameterSource parameters = new MapSqlParameterSource("document", bodyWithEtag.asJson());
return Mono.fromCallable(() -> insertDocument(sql, parameters))
.subscribeOn(Schedulers.elastic())
.flatMap(o -> successSave(exchange, bodyWithEtag, resourceClass));
}
private int insertDocument(String sql, MapSqlParameterSource parameters) {
return namedParameterJdbcTemplate.update(sql, parameters);
}
private Mono> successSave(Exchange exchange, JsonObject savedDocument, Class resourceClass) {
T body = resourceDeserializer.deserializeJsonObject(savedDocument, resourceClass);
if (logger.isDebugEnabled()) {
logger.debug("Successfully saved item: {}", exchange.getRequest().getUrl());
}
return Mono.just(exchange
.response(Response.create(StatusCode._201_CREATED)
.body(body)));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy