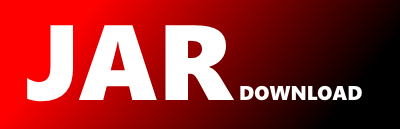
com.zuunr.json.schema.generation.SchemaPatcher Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of json Show documentation
Show all versions of json Show documentation
Immutable JSON representation in Java
package com.zuunr.json.schema.generation;
import com.zuunr.json.JsonObject;
import com.zuunr.json.JsonObjectBuilder;
import com.zuunr.json.JsonValue;
import java.util.Iterator;
import static com.zuunr.json.schema.Keywords.ITEMS;
import static com.zuunr.json.schema.Keywords.KEYWORDS_WHERE_VALUE_IS_SCHEMA;
import static com.zuunr.json.schema.Keywords.PROPERTIES;
/**
* @author Niklas Eldberger
*/
public class SchemaPatcher {
private static JsonObject subschemaKeywords;
private static JsonObject subschemaOrNestedSchemaKeywords() {
if (subschemaKeywords == null) {
JsonObjectBuilder builder = JsonObject.EMPTY.builder();
for (JsonValue keyword : KEYWORDS_WHERE_VALUE_IS_SCHEMA) {
builder.put(keyword.getString(), keyword);
}
subschemaKeywords = builder.build();
}
return subschemaKeywords;
}
public JsonObject patch(JsonObject toBePatchedSchema, JsonObject overriderSchema) {
JsonObject patchedSchema = patchNonSubschemaKeywords(toBePatchedSchema, overriderSchema);
patchedSchema = patchSubschemaKeywords(patchedSchema, overriderSchema);
return patchedSchema;
}
private JsonObject patchSubschemaKeywords(JsonObject toBePatchedSchema, JsonObject overriderSchema) {
JsonObjectBuilder schemaBuilder = toBePatchedSchema.builder();
JsonObject overriderProperties = overriderSchema.get(PROPERTIES, JsonValue.NULL).getJsonObject();
if (overriderProperties != null) {
JsonObject toBePatchedPropertiesSchema = toBePatchedSchema.get(PROPERTIES, JsonValue.NULL).getJsonObject();
schemaBuilder.put(PROPERTIES, patchProperties(toBePatchedPropertiesSchema, overriderProperties));
}
JsonObject overriderItems = overriderSchema.get(ITEMS, JsonValue.NULL).getJsonObject();
if (overriderItems != null) {
JsonObject toBePatchedItemsSchema = toBePatchedSchema.get(ITEMS, JsonValue.NULL).getJsonObject();
schemaBuilder.put(ITEMS, patchProperties(toBePatchedItemsSchema, overriderItems));
}
return schemaBuilder.build();
}
private JsonObject patchProperties(JsonObject toBePatchedProperties, JsonObject overriderProperties) {
JsonObjectBuilder propertiesBuilder = toBePatchedProperties.builder();
Iterator values = overriderProperties.values().iterator();
for (Iterator iterator = overriderProperties.keys().iterator(); iterator.hasNext(); ) {
String propertyKey = iterator.next().getString();
JsonValue verriderPropertyValue = values.next();
JsonValue propertySchemaToBePatched = toBePatchedProperties.get(propertyKey);
if (propertySchemaToBePatched != null && verriderPropertyValue.isJsonObject()) {
propertiesBuilder.put(propertyKey, patch(propertySchemaToBePatched.getJsonObject(), verriderPropertyValue.getJsonObject()));
}
}
return propertiesBuilder.build();
}
public JsonObject patchNonSubschemaKeywords(JsonObject toBePatchedSchema, JsonObject overriderSchema) {
JsonObjectBuilder schemaBuilder = toBePatchedSchema.builder();
Iterator overriderValues = overriderSchema.values().iterator();
for (Iterator overriderKeys = overriderSchema.keys().iterator(); overriderKeys.hasNext(); ) {
String overriderKey = overriderKeys.next().getString();
JsonValue overriderValue = overriderValues.next();
if (!subschemaOrNestedSchemaKeywords().containsKey(overriderKey)) {
schemaBuilder.put(overriderKey, overriderValue);
}
}
return schemaBuilder.build();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy