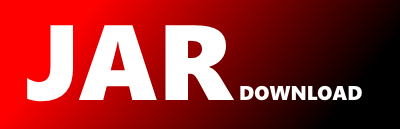
com.zuunr.json.schema.validation.node.array.ItemNode Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of json Show documentation
Show all versions of json Show documentation
Immutable JSON representation in Java
package com.zuunr.json.schema.validation.node.array;
import com.zuunr.json.JsonArray;
import com.zuunr.json.JsonArrayBuilder;
import com.zuunr.json.JsonValue;
import com.zuunr.json.UnsupportedTypeException;
import com.zuunr.json.schema.JsonSchema;
import com.zuunr.json.schema.Keywords;
import com.zuunr.json.schema.validation.ValidationContext;
import com.zuunr.json.schema.validation.node.SchemaNode;
import com.zuunr.json.schema.validation.node.ValidationNode;
/**
* @author Niklas Eldberger
*/
public class ItemNode extends ValidationNode {
private static final JsonValue ITEMS = JsonValue.of(Keywords.ITEMS);
private static final JsonValue UNEVALUATED_ITEMS = JsonValue.of(Keywords.UNEVALUATED_ITEMS);
private int instanceIndex;
private JsonValue keyword = ITEMS;
private JsonArrayBuilder filtrateBuilder;
/**
* TODO: Document
*
* @param instance the JsonValue to be validated
* @param schema the JsonSchema to be used when validated
* @param keywordIndex the index of the keyword in the schema that is to be validated in by this ValidationNode
* @param validationContext a context that remains the same for all ValidationNodes created to evaluate a JsonValue instance according to a JsonSchema instance
* @param rootInstance the root JsonValue where the validation stated
* @param instanceIndex the index of the element in the JsonArray that is being validated
*/
public ItemNode(JsonValue instance, JsonSchema schema, int keywordIndex, ValidationContext validationContext, JsonValue rootInstance, int instanceIndex) {
super(instance, schema, keywordIndex, validationContext, rootInstance);
this.instanceIndex = instanceIndex;
this.filtrateBuilder = validationContext.returnFiltrate() ? JsonArray.EMPTY.builder() : null;
}
@Override
protected ValidationNode createFirstChildNode() {
JsonValue items = schema.getKeyword(Keywords.ITEMS);
if (items == null) {
items = schema.getKeyword(Keywords.UNEVALUATED_ITEMS);
keyword = UNEVALUATED_ITEMS;
}
JsonArray instanceArray = instance.getJsonArray();
if (items != null && instanceIndex < instanceArray.size()) {
if (items.isJsonObject() || items.isBoolean()) {
JsonValue instanceItem = instanceArray.get(instanceIndex);
if (instanceItem != null) {
return new SchemaNode(instanceItem, items.as(JsonSchema.class), validationContext(), rootInstance);
} else {
return null;
}
} else {
throw new UnsupportedTypeException("Only JSON Object and boolean is currently supported as items. JSON Array should be supported too!");
}
}
return null;
}
@Override
protected void doAfterAllChildNodesAreCompleted() {
if (getValid() == null) {
setValid(true);
filtrate = instance;
} else if (validationContext().returnFiltrate() && filtrateBuilder != null) {
filtrate = filtrateBuilder.build().jsonValue();
}
}
@Override
protected void childNodeCompleted(ValidationNode subnode) {
if (!subnode.getValid()) {
setValid(false);
}
if (filtrateBuilder != null) {
if (subnode.filtrate() == null) {
// There is nothing to put at this position/index and that means the array cannot be preserved
// If we choose to allow for JSON null when items are Java null this is where it should be put! :)
filtrateBuilder = null;
} else {
filtrateBuilder.add(subnode.filtrate());
}
}
}
@Override
protected ValidationNode createNextChildNodeOfParent() {
int nextInstanceIndex = instanceIndex + 1;
if (instance.getJsonArray().size() > nextInstanceIndex) {
return new ItemNode(instance, schema, keywordSchemaIndex(), validationContext(), rootInstance, nextInstanceIndex);
} else {
return null;
}
}
public int index() {
return instanceIndex;
}
@Override
protected Boolean calculateValid() {
return null; //NOSONAR
}
@Override
protected JsonValue keyword() {
return keyword;
}
public JsonValue instanceIndex() {
return JsonValue.of(instanceIndex);
}
@Override
public Location location() {
if (location == null) {
location = new Location(
parentNode.location.instance.add(instanceIndex),
null,
parentNode.location.keyword.add(keyword),
schema.getKeyword(keyword.getString()));
}
return location;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy