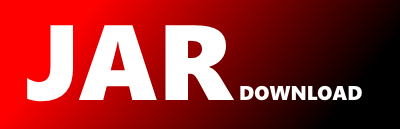
com.zuunr.json.JsonValueDeserializer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of json Show documentation
Show all versions of json Show documentation
Immutable JSON representation in Java
/*
* Copyright 2020 Zuunr AB
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.zuunr.json;
import com.fasterxml.jackson.core.JsonParser;
import com.fasterxml.jackson.databind.DeserializationContext;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.deser.std.StdDeserializer;
import java.io.IOException;
import java.util.Iterator;
import java.util.Map;
public class JsonValueDeserializer extends StdDeserializer {
private static final long serialVersionUID = 1L;
public JsonValueDeserializer() {
this(null);
}
public JsonValueDeserializer(Class> vc) {
super(vc);
}
@Override
public JsonValue deserialize(JsonParser jp, DeserializationContext ctxt)
throws IOException {
JsonNode node = jp.getCodec().readTree(jp);
return deserialize(node);
}
private JsonValue deserialize(JsonNode jsonNode) {
// TODO: Remove recursion in impl
JsonValue result;
switch (jsonNode.getNodeType()) {
case OBJECT: {
JsonObjectBuilder builder = JsonObject.EMPTY.builder();
for (Iterator> fields = jsonNode.fields(); fields.hasNext(); ) {
Map.Entry entry = fields.next();
JsonNode valueNode = entry.getValue();
builder.put(entry.getKey(), deserialize(valueNode));
}
result = builder.build().jsonValue();
break;
}
case ARRAY: {
JsonArrayBuilder builder = JsonArray.EMPTY.builder();
for (int i = 0; i < jsonNode.size(); i++) {
builder.add(deserialize(jsonNode.get(i)));
}
result = builder.build().jsonValue();
break;
}
case NULL: {
result = JsonValue.NULL;
break;
}
case NUMBER: {
result = JsonValue.of(jsonNode.numberValue());
break;
}
case STRING: {
result = JsonValue.of(jsonNode.textValue());
break;
}
case BOOLEAN: {
result = JsonValue.of(jsonNode.booleanValue());
break;
}
default: {
throw new UnsupportedTypeException("Type not supported");
}
}
return result;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy