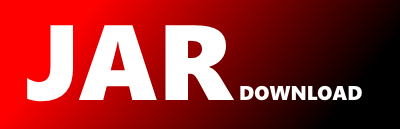
com.zuunr.json.schema.validation.node.DependentSchemasNode Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of json Show documentation
Show all versions of json Show documentation
Immutable JSON representation in Java
package com.zuunr.json.schema.validation.node;
import com.zuunr.json.JsonArray;
import com.zuunr.json.JsonArrayBuilder;
import com.zuunr.json.JsonObject;
import com.zuunr.json.JsonValue;
import com.zuunr.json.schema.JsonSchema;
import com.zuunr.json.schema.Keywords;
import com.zuunr.json.schema.validation.ValidationContext;
/**
* @author Niklas Eldberger
*/
public class DependentSchemasNode extends ValidationNode {
private static final JsonValue DEPENDENT_SCHEMAS = JsonValue.of(Keywords.DEPENDENT_SCHEMAS);
public DependentSchemasNode(JsonValue instance, JsonSchema schema, int schemaKeywordIndex, ValidationContext validationContext, JsonValue rootInstance) {
super(instance, schema, schemaKeywordIndex, validationContext, rootInstance);
}
protected void doAfterAllChildNodesAreCompleted() {
if (getValid() == null) {
setValid(true);
}
}
@Override
protected void childNodeCompleted(ValidationNode subnode) {
if (!subnode.getValid()) {
setValid(false);
allChildNodesAreCompleted();
}
}
protected ValidationNode createFirstChildNode() {
int nextDependentSchemaIndex = nextDependentSchema(schema, 0, instance.getJsonObject());
if (nextDependentSchemaIndex > -1) {
return new DependentSchemasIndexNode(instance, schema, keywordSchemaIndex(), validationContext(), rootInstance, 0, nextDependentSchemaIndex);
}
return null;
}
@Override
protected ValidationNode createNextChildNodeOfParent() {
return ((SchemaNode) parentNode).createKeywordNode(keywordSchemaIndex() + 1);
}
@Override
protected Boolean calculateValid() {
return null; //NOSONAR
}
@Override
protected JsonValue keyword() {
return DEPENDENT_SCHEMAS;
}
@Override
public Location location() {
if (location == null) {
location = new Location(
parentNode.location.instance,
parentNode.location.instanceProperty,
parentNode.location.keyword.add(DEPENDENT_SCHEMAS),
schema.getKeyword(Keywords.DEPENDENT_SCHEMAS));
}
return location;
}
private JsonArray dependentSchemasToBeApplied(JsonObject dependentSchemas, JsonObject jsonObject2) {
JsonArrayBuilder builder = JsonArray.EMPTY.builder();
for (int i = 0; i < dependentSchemas.size(); i++) {
JsonValue propertyName = dependentSchemas.keys().get(i);
builder = jsonObject2.get(propertyName.getString()) == null ? builder : builder.add(i);
}
return builder.build();
}
public static int nextDependentSchema(JsonSchema schema, int startIndex, JsonObject instance) {
for (int i = startIndex; i < schema.getDependentSchemas().keys().size(); i++) {
JsonValue fieldValue = instance.get(schema.getDependentSchemas().keys().get(i).getString());
if (fieldValue != null) {
return i;
}
}
return -1;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy