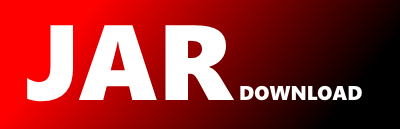
klass.model.meta.domain.ParameterizedPropertyFinder Maven / Gradle / Ivy
The newest version!
package klass.model.meta.domain;
import java.math.BigDecimal;
import java.sql.Timestamp;
import java.util.*;
import java.io.*;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.gs.fw.common.mithra.*;
import com.gs.fw.common.mithra.attribute.*;
import com.gs.fw.common.mithra.util.*;
import com.gs.fw.common.mithra.notification.*;
import com.gs.fw.common.mithra.notification.listener.*;
import com.gs.fw.common.mithra.list.cursor.Cursor;
import com.gs.fw.common.mithra.bulkloader.*;
import com.gs.fw.common.mithra.*;
import com.gs.fw.common.mithra.attribute.*;
import com.gs.fw.common.mithra.attribute.calculator.procedure.ObjectProcedure;
import com.gs.fw.common.mithra.behavior.txparticipation.*;
import com.gs.fw.common.mithra.cache.Cache;
import com.gs.fw.common.mithra.cache.bean.*;
import com.gs.fw.common.mithra.extractor.*;
import com.gs.fw.common.mithra.finder.*;
import com.gs.fw.common.mithra.finder.booleanop.*;
import com.gs.fw.common.mithra.finder.integer.*;
import com.gs.fw.common.mithra.finder.longop.*;
import com.gs.fw.common.mithra.finder.orderby.OrderBy;
import com.gs.fw.common.mithra.finder.string.*;
import com.gs.fw.common.mithra.extractor.NormalAndListValueSelector;
import com.gs.fw.common.mithra.list.NulledRelation;
import com.gs.fw.common.mithra.querycache.CachedQuery;
import com.gs.fw.common.mithra.querycache.QueryCache;
import com.gs.fw.common.mithra.portal.*;
import com.gs.fw.common.mithra.remote.*;
import com.gs.fw.common.mithra.transaction.MithraObjectPersister;
import com.gs.fw.common.mithra.util.TimestampPool;
import org.eclipse.collections.impl.map.mutable.UnifiedMap;
import java.io.Serializable;
/**
* This file was automatically generated using Mithra 18.0.0. Please do not modify it.
* Add custom logic to its subclass instead.
*/
public class ParameterizedPropertyFinder
{
private static final String IMPL_CLASS_NAME_WITH_SLASHES = "klass/model/meta/domain/ParameterizedProperty";
private static final String BUSINESS_CLASS_NAME_WITH_DOTS = "klass.model.meta.domain.ParameterizedProperty";
private static final FinderMethodMap finderMethodMap;
private static boolean isFullCache;
private static boolean isOffHeap;
private static volatile MithraObjectPortal objectPortal = new UninitializedPortal("klass.model.meta.domain.ParameterizedProperty");
private static final ParameterizedPropertySingleFinder finder = new ParameterizedPropertySingleFinder();
private static ConstantStringSet[] constantStringSets = new ConstantStringSet[0];
private static ConstantIntSet[] constantIntSets = new ConstantIntSet[0];
private static ConstantShortSet[] constantShortSets = new ConstantShortSet[0];
static
{
finderMethodMap = new FinderMethodMap(ParameterizedPropertyFinder.ParameterizedPropertyRelatedFinder.class);
finderMethodMap.addNormalAttributeName("owningClassName");
finderMethodMap.addNormalAttributeName("name");
finderMethodMap.addNormalAttributeName("resultTypeName");
finderMethodMap.addNormalAttributeName("ordinal");
finderMethodMap.addNormalAttributeName("multiplicity");
finderMethodMap.addRelationshipName("resultType");
finderMethodMap.addRelationshipName("parameterizedPropertyOrderings");
finderMethodMap.addRelationshipName("parameters");
finderMethodMap.addRelationshipName("owningClass");
}
public static Attribute[] allPersistentAttributes()
{
return finder.getPersistentAttributes();
}
public static List allRelatedFinders()
{
return finder.getRelationshipFinders();
}
public static List allDependentRelatedFinders()
{
return finder.getDependentRelationshipFinders();
}
public static ConstantStringSet zGetConstantStringSet(int index)
{
return constantStringSets[index];
}
public static ConstantIntSet zGetConstantIntSet(int index)
{
return constantIntSets[index];
}
public static ConstantShortSet zGetConstantShortSet(int index)
{
return constantShortSets[index];
}
public static Mapper zGetConstantJoin(int index)
{
return getConstantJoinPool()[index];
}
private static Mapper[] constantJoinPool;
private static Mapper[] getConstantJoinPool()
{
if (constantJoinPool == null)
{
Mapper[] result = new Mapper[10];
result[0] = ParameterizedPropertyFinder.resultTypeName().constructEqualityMapper(KlassFinder.name());
result[1] = KlassFinder.name().constructEqualityMapper(ParameterizedPropertyFinder.resultTypeName());
result[2] = ParameterizedPropertyFinder.owningClassName().constructEqualityMapper(ParameterizedPropertyOrderingFinder.owningClassName());
result[3] = ParameterizedPropertyOrderingFinder.owningClassName().constructEqualityMapper(ParameterizedPropertyFinder.owningClassName());
result[4] = ParameterizedPropertyFinder.name().constructEqualityMapper(ParameterizedPropertyOrderingFinder.name());
result[5] = ParameterizedPropertyOrderingFinder.name().constructEqualityMapper(ParameterizedPropertyFinder.name());
result[6] = ParameterizedPropertyFinder.owningClassName().constructEqualityMapper(ParameterizedPropertyParameterFinder.classifierName());
result[7] = ParameterizedPropertyParameterFinder.classifierName().constructEqualityMapper(ParameterizedPropertyFinder.owningClassName());
result[8] = ParameterizedPropertyFinder.name().constructEqualityMapper(ParameterizedPropertyParameterFinder.parameterizedPropertyName());
result[9] = ParameterizedPropertyParameterFinder.parameterizedPropertyName().constructEqualityMapper(ParameterizedPropertyFinder.name());
constantJoinPool = result;
}
return constantJoinPool;
}
public static SourceAttributeType getSourceAttributeType()
{
return null;
}
public static ParameterizedProperty findOne(com.gs.fw.finder.Operation operation)
{
return findOne(operation, false);
}
public static ParameterizedProperty findOneBypassCache(com.gs.fw.finder.Operation operation)
{
return findOne(operation, true);
}
public static ParameterizedPropertyList findMany(com.gs.fw.finder.Operation operation)
{
return (ParameterizedPropertyList) finder.findMany(operation);
}
public static ParameterizedPropertyList findManyBypassCache(com.gs.fw.finder.Operation operation)
{
return (ParameterizedPropertyList) finder.findManyBypassCache(operation);
}
private static ParameterizedProperty findOne(com.gs.fw.finder.Operation operation, boolean bypassCache)
{
List found = getMithraObjectPortal().find((Operation) operation, bypassCache);
return (ParameterizedProperty) FinderUtils.findOne(found);
}
public static ParameterizedProperty findByPrimaryKey(String owningClassName, String name)
{
return finder.findByPrimaryKey(owningClassName, name);
}
private static final RelationshipHashStrategy forPrimaryKey = new PrimaryKeyRhs();
private static final class PrimaryKeyRhs implements RelationshipHashStrategy
{
public boolean equalsForRelationship(Object _srcObject, Object _srcData, Object _targetData, Timestamp _asOfDate0, Timestamp _asOfDate1)
{
I3O3L3 _bean = (I3O3L3) _srcData;
ParameterizedPropertyData _castedTargetData = (ParameterizedPropertyData) _targetData;
if (_bean.getO1AsString().equals(_castedTargetData.getOwningClassName()) && _bean.getO2AsString().equals(_castedTargetData.getName()))
{
return true;
}
return false;
}
public int computeHashCodeFromRelated(Object _srcObject, Object _srcData)
{
I3O3L3 _bean = (I3O3L3) _srcData;
return HashUtil.combineHashes(HashUtil.hash(_bean.getO1AsString()),HashUtil.hash(_bean.getO2AsString()));
}
public int computeOffHeapHashCodeFromRelated(Object _srcObject, Object _srcData)
{
I3O3L3 _bean = (I3O3L3) _srcData;
return HashUtil.combineHashes(HashUtil.offHeapHash(_bean.getO1AsString()),HashUtil.offHeapHash(_bean.getO2AsString()));
}
}
public static ParameterizedProperty zFindOneForRelationship(Operation operation)
{
List found = getMithraObjectPortal().findAsCachedQuery(operation, null, false, true, 0).getResult();
return (ParameterizedProperty) FinderUtils.findOne(found);
}
public static MithraObjectPortal getMithraObjectPortal()
{
return objectPortal.getInitializedPortal();
}
public static void clearQueryCache()
{
objectPortal.clearQueryCache();
}
public static void reloadCache()
{
objectPortal.reloadCache();
}
public static class ParameterizedPropertyRelatedFinder extends AbstractRelatedFinder
implements NamedElementFinder
{
private List relationshipFinders;
private List dependentRelationshipFinders;
private StringAttribute owningClassName;
private StringAttribute name;
private StringAttribute resultTypeName;
private IntegerAttribute ordinal;
private StringAttribute multiplicity;
private ParameterizedPropertyResultTypeFinderSubclass resultType;
private ParameterizedPropertyParameterizedPropertyOrderingsFinderSubclass parameterizedPropertyOrderings;
private ParameterizedPropertyParametersFinderSubclass parameters;
private ParameterizedPropertyOwningClassFinderSubclass owningClass;
public ParameterizedPropertyRelatedFinder()
{
super();
}
public ParameterizedPropertyRelatedFinder(Mapper mapper)
{
super(mapper);
}
public String getFinderClassName()
{
return "klass.model.meta.domain.ParameterizedPropertyFinder";
}
public RelatedFinder getRelationshipFinderByName(String relationshipName)
{
return ParameterizedPropertyFinder.finderMethodMap.getRelationshipFinderByName(relationshipName, this);
}
public Attribute getAttributeByName(String attributeName)
{
return ParameterizedPropertyFinder.finderMethodMap.getAttributeByName(attributeName, this);
}
public com.gs.fw.common.mithra.extractor.Function getAttributeOrRelationshipSelector(String attributeName)
{
return ParameterizedPropertyFinder.finderMethodMap.getAttributeOrRelationshipSelectorFunction(attributeName, this);
}
public Attribute[] getPersistentAttributes()
{
Attribute[] attributes = new Attribute[5];
attributes[0] = this.owningClassName();
attributes[1] = this.name();
attributes[2] = this.resultTypeName();
attributes[3] = this.ordinal();
attributes[4] = this.multiplicity();
return attributes;
}
public List getRelationshipFinders()
{
if (relationshipFinders == null)
{
List relatedFinders = new ArrayList(4);
relatedFinders.add(this.resultType());
relatedFinders.add(this.parameterizedPropertyOrderings());
relatedFinders.add(this.parameters());
relatedFinders.add(this.owningClass());
relationshipFinders = Collections.unmodifiableList(relatedFinders);
}
return relationshipFinders;
}
public List getDependentRelationshipFinders()
{
if (dependentRelationshipFinders == null)
{
List dependentRelatedFinders = new ArrayList(1);
dependentRelatedFinders.add(this.parameterizedPropertyOrderings());
dependentRelationshipFinders = Collections.unmodifiableList(dependentRelatedFinders);
}
return dependentRelationshipFinders;
}
public ParameterizedProperty findOne(com.gs.fw.finder.Operation operation)
{
return ParameterizedPropertyFinder.findOne(operation, false);
}
public ParameterizedProperty findOneBypassCache(com.gs.fw.finder.Operation operation)
{
return ParameterizedPropertyFinder.findOne(operation, true);
}
public MithraList extends ParameterizedProperty> findMany(com.gs.fw.finder.Operation operation)
{
return new ParameterizedPropertyList((Operation) operation);
}
public MithraList extends ParameterizedProperty> findManyBypassCache(com.gs.fw.finder.Operation operation)
{
ParameterizedPropertyList result = (ParameterizedPropertyList) this.findMany(operation);
result.setBypassCache(true);
return result;
}
public MithraList extends ParameterizedProperty> constructEmptyList()
{
return new ParameterizedPropertyList();
}
public int getSerialVersionId()
{
return 1138466370;
}
public boolean isPure()
{
return false;
}
public boolean isTemporary()
{
return false;
}
public int getHierarchyDepth()
{
return 0;
}
public StringAttribute owningClassName()
{
StringAttribute result = this.owningClassName;
if (result == null)
{
result = mapper == null ? SingleColumnStringAttribute.generate("\"OWNING_CLASS_NAME\"","","owningClassName",BUSINESS_CLASS_NAME_WITH_DOTS,IMPL_CLASS_NAME_WITH_SLASHES,false,false,this,null,true,false,-1,-1,-1,256,false, false) :
new MappedStringAttribute(ParameterizedPropertyFinder.owningClassName(), this.mapper, this.zGetValueSelector());
result.zSetOwningRelationship("owningClass");
result.zSetOwningReverseRelationship("klass.model.meta.domain", "Klass", "parameterizedProperties");
this.owningClassName = result;
}
return result;
}
public StringAttribute name()
{
StringAttribute result = this.name;
if (result == null)
{
result = mapper == null ? SingleColumnStringAttribute.generate("\"NAME\"","","name",BUSINESS_CLASS_NAME_WITH_DOTS,IMPL_CLASS_NAME_WITH_SLASHES,false,false,this,null,true,false,-1,-1,-1,256,false, false) :
new MappedStringAttribute(ParameterizedPropertyFinder.name(), this.mapper, this.zGetValueSelector());
this.name = result;
}
return result;
}
public StringAttribute resultTypeName()
{
StringAttribute result = this.resultTypeName;
if (result == null)
{
result = mapper == null ? SingleColumnStringAttribute.generate("\"RESULT_TYPE_NAME\"","","resultTypeName",BUSINESS_CLASS_NAME_WITH_DOTS,IMPL_CLASS_NAME_WITH_SLASHES,false,false,this,null,true,false,-1,-1,-1,256,false, false) :
new MappedStringAttribute(ParameterizedPropertyFinder.resultTypeName(), this.mapper, this.zGetValueSelector());
result.zSetOwningRelationship("resultType");
result.zSetOwningReverseRelationship("klass.model.meta.domain", "Klass", "parameterizedPropertiesResultTypeOf");
this.resultTypeName = result;
}
return result;
}
public IntegerAttribute ordinal()
{
IntegerAttribute result = this.ordinal;
if (result == null)
{
result = mapper == null ? SingleColumnIntegerAttribute.generate("\"ORDINAL\"","","ordinal",BUSINESS_CLASS_NAME_WITH_DOTS,IMPL_CLASS_NAME_WITH_SLASHES,false,false,this,null,true,false,false,-1,-1,-1,false, false) :
new MappedIntegerAttribute(ParameterizedPropertyFinder.ordinal(), this.mapper, this.zGetValueSelector());
this.ordinal = result;
}
return result;
}
public StringAttribute multiplicity()
{
StringAttribute result = this.multiplicity;
if (result == null)
{
result = mapper == null ? SingleColumnStringAttribute.generate("\"MULTIPLICITY\"","","multiplicity",BUSINESS_CLASS_NAME_WITH_DOTS,IMPL_CLASS_NAME_WITH_SLASHES,false,false,this,null,true,false,-1,-1,-1,256,false, false) :
new MappedStringAttribute(ParameterizedPropertyFinder.multiplicity(), this.mapper, this.zGetValueSelector());
this.multiplicity = result;
}
return result;
}
public KlassFinder.KlassSingleFinderForRelatedClasses resultType()
{
ParameterizedPropertyResultTypeFinderSubclass result = this.resultType;
if (result == null)
{
Mapper newMapper = combineWithMapperIfExists(ParameterizedPropertyFinder.zGetParameterizedPropertyResultTypeReverseMapper());
newMapper.setToMany(false);
result = new ParameterizedPropertyResultTypeFinderSubclass(newMapper , this.zGetValueSelector() );
this.resultType = result;
}
return result;
}
public ParameterizedPropertyOrderingFinder.ParameterizedPropertyOrderingCollectionFinderForRelatedClasses parameterizedPropertyOrderings()
{
ParameterizedPropertyParameterizedPropertyOrderingsFinderSubclass result = this.parameterizedPropertyOrderings;
if (result == null)
{
Mapper newMapper = combineWithMapperIfExists(ParameterizedPropertyFinder.zGetParameterizedPropertyParameterizedPropertyOrderingsReverseMapper());
newMapper.setToMany(true);
result = new ParameterizedPropertyParameterizedPropertyOrderingsFinderSubclass(newMapper , this.zGetValueSelector() );
this.parameterizedPropertyOrderings = result;
}
return result;
}
public ParameterizedPropertyParameterFinder.ParameterizedPropertyParameterCollectionFinderForRelatedClasses parameters()
{
ParameterizedPropertyParametersFinderSubclass result = this.parameters;
if (result == null)
{
Mapper newMapper = combineWithMapperIfExists(ParameterizedPropertyFinder.zGetParameterizedPropertyParametersReverseMapper());
newMapper.setToMany(true);
result = new ParameterizedPropertyParametersFinderSubclass(newMapper , this.zGetValueSelector() );
this.parameters = result;
}
return result;
}
public KlassFinder.KlassSingleFinderForRelatedClasses owningClass()
{
ParameterizedPropertyOwningClassFinderSubclass result = this.owningClass;
if (result == null)
{
Mapper newMapper = combineWithMapperIfExists(KlassFinder.zGetKlassParameterizedPropertiesMapper());
newMapper.setToMany(false);
result = new ParameterizedPropertyOwningClassFinderSubclass(newMapper , this.zGetValueSelector() );
this.owningClass = result;
}
return result;
}
public Attribute getSourceAttribute()
{
return null;
}
private Mapper combineWithMapperIfExists(Mapper newMapper)
{
if (this.mapper != null)
{
return new LinkedMapper(this.mapper, newMapper);
}
return newMapper;
}
public Attribute[] getPrimaryKeyAttributes()
{
return ParameterizedPropertyFinder.getPrimaryKeyAttributes();
}
public VersionAttribute getVersionAttribute()
{
return null;
}
public MithraObjectPortal getMithraObjectPortal()
{
return ParameterizedPropertyFinder.getMithraObjectPortal();
}
}
public static class ParameterizedPropertyCollectionFinder extends ParameterizedPropertyRelatedFinder
{
public ParameterizedPropertyCollectionFinder(Mapper mapper)
{
super(mapper);
}
}
public static abstract class ParameterizedPropertyCollectionFinderForRelatedClasses
extends ParameterizedPropertyCollectionFinder
implements DeepRelationshipAttribute
{
public ParameterizedPropertyCollectionFinderForRelatedClasses(Mapper mapper)
{
super(mapper);
}
protected NormalAndListValueSelector zGetValueSelector()
{
return this;
}
}
public static class ParameterizedPropertySingleFinder extends ParameterizedPropertyRelatedFinder
implements ToOneFinder
{
public ParameterizedPropertySingleFinder(Mapper mapper)
{
super(mapper);
}
public ParameterizedPropertySingleFinder()
{
super(null);
}
public Operation eq(ParameterizedProperty other)
{
return this.owningClassName().eq(other.getOwningClassName())
.and(this.name().eq(other.getName()))
;
}
// this implementation uses private API. Do NOT copy to application code. Application code must use normal operations for lookups.
public ParameterizedProperty findByPrimaryKey(String owningClassName, String name)
{
ParameterizedProperty _result = null;
Operation _op = null;
Object _related = null;
if (owningClassName != null && name != null)
{
I3O3L3 _bean = I3O3L3.POOL.getOrConstruct();
_bean.setO1(owningClassName);
_bean.setO2(name);
MithraObjectPortal _portal = this.getMithraObjectPortal();
_related = _portal.getAsOneFromCacheForFind(_bean, _bean, forPrimaryKey, null, null);
_bean.release();
}
if (!(_related instanceof NulledRelation)) _result = (ParameterizedProperty) _related;
if (_related == null)
{
_op = this.owningClassName().eq(owningClassName).and(this.name().eq(name));
}
if (_op != null)
{
_result = this.findOne(_op);
}
return _result;
}
}
public static abstract class ParameterizedPropertySingleFinderForRelatedClasses extends ParameterizedPropertySingleFinder implements DeepRelationshipAttribute
{
public ParameterizedPropertySingleFinderForRelatedClasses(Mapper mapper)
{
super(mapper);
}
protected NormalAndListValueSelector zGetValueSelector()
{
return this;
}
}
private static Mapper resultTypeReverseMapper = null;
public static Mapper zGetParameterizedPropertyResultTypeReverseMapper()
{
if (resultTypeReverseMapper == null)
{
resultTypeReverseMapper = zConstructParameterizedPropertyResultTypeReverseMapper();
}
return resultTypeReverseMapper;
}
private static Mapper resultTypeMapper = null;
public static Mapper zGetParameterizedPropertyResultTypeMapper()
{
if (resultTypeMapper == null)
{
resultTypeMapper = zConstructParameterizedPropertyResultTypeMapper();
}
return resultTypeMapper;
}
private static Mapper resultTypePureReverseMapper = null;
public static Mapper zGetParameterizedPropertyResultTypePureReverseMapper()
{
if (resultTypePureReverseMapper == null)
{
resultTypePureReverseMapper = zConstructParameterizedPropertyResultTypePureReverseMapper();
}
return resultTypePureReverseMapper;
}
private static Mapper zConstructParameterizedPropertyResultTypePureReverseMapper()
{
Mapper resultTypeMapper = ParameterizedPropertyFinder.zGetConstantJoin(0);
resultTypeMapper.setName("resultType");
return resultTypeMapper;
}
private static Mapper zConstructParameterizedPropertyResultTypeReverseMapper()
{
Mapper resultTypeMapper = ParameterizedPropertyFinder.zGetConstantJoin(0);
resultTypeMapper.setName("resultType");
return resultTypeMapper;
}
private static Mapper zConstructParameterizedPropertyResultTypeMapper()
{
Mapper resultTypeMapper = ParameterizedPropertyFinder.zGetConstantJoin(1);
resultTypeMapper.setName("parameterizedPropertiesResultTypeOf");
return resultTypeMapper;
}
private static Mapper parameterizedPropertyOrderingsReverseMapper = null;
public static Mapper zGetParameterizedPropertyParameterizedPropertyOrderingsReverseMapper()
{
if (parameterizedPropertyOrderingsReverseMapper == null)
{
parameterizedPropertyOrderingsReverseMapper = zConstructParameterizedPropertyParameterizedPropertyOrderingsReverseMapper();
}
return parameterizedPropertyOrderingsReverseMapper;
}
private static Mapper parameterizedPropertyOrderingsMapper = null;
public static Mapper zGetParameterizedPropertyParameterizedPropertyOrderingsMapper()
{
if (parameterizedPropertyOrderingsMapper == null)
{
parameterizedPropertyOrderingsMapper = zConstructParameterizedPropertyParameterizedPropertyOrderingsMapper();
}
return parameterizedPropertyOrderingsMapper;
}
private static Mapper parameterizedPropertyOrderingsPureReverseMapper = null;
public static Mapper zGetParameterizedPropertyParameterizedPropertyOrderingsPureReverseMapper()
{
if (parameterizedPropertyOrderingsPureReverseMapper == null)
{
parameterizedPropertyOrderingsPureReverseMapper = zConstructParameterizedPropertyParameterizedPropertyOrderingsPureReverseMapper();
}
return parameterizedPropertyOrderingsPureReverseMapper;
}
private static Mapper zConstructParameterizedPropertyParameterizedPropertyOrderingsPureReverseMapper()
{
InternalList parameterizedPropertyOrderingsMapperMapperList = new InternalList(2);
parameterizedPropertyOrderingsMapperMapperList.add(ParameterizedPropertyFinder.zGetConstantJoin(2));
parameterizedPropertyOrderingsMapperMapperList.add(ParameterizedPropertyFinder.zGetConstantJoin(4));
Mapper parameterizedPropertyOrderingsMapper = new MultiEqualityMapper(parameterizedPropertyOrderingsMapperMapperList);
parameterizedPropertyOrderingsMapper.setName("parameterizedPropertyOrderings");
return parameterizedPropertyOrderingsMapper;
}
private static Mapper zConstructParameterizedPropertyParameterizedPropertyOrderingsReverseMapper()
{
InternalList parameterizedPropertyOrderingsMapperMapperList = new InternalList(2);
parameterizedPropertyOrderingsMapperMapperList.add(ParameterizedPropertyFinder.zGetConstantJoin(2));
parameterizedPropertyOrderingsMapperMapperList.add(ParameterizedPropertyFinder.zGetConstantJoin(4));
Mapper parameterizedPropertyOrderingsMapper = new MultiEqualityMapper(parameterizedPropertyOrderingsMapperMapperList);
parameterizedPropertyOrderingsMapper.setName("parameterizedPropertyOrderings");
return parameterizedPropertyOrderingsMapper;
}
private static Mapper zConstructParameterizedPropertyParameterizedPropertyOrderingsMapper()
{
InternalList parameterizedPropertyOrderingsMapperMapperList = new InternalList(2);
parameterizedPropertyOrderingsMapperMapperList.add(ParameterizedPropertyFinder.zGetConstantJoin(3));
parameterizedPropertyOrderingsMapperMapperList.add(ParameterizedPropertyFinder.zGetConstantJoin(5));
Mapper parameterizedPropertyOrderingsMapper = new MultiEqualityMapper(parameterizedPropertyOrderingsMapperMapperList);
parameterizedPropertyOrderingsMapper.setName("parameterizedProperty");
return parameterizedPropertyOrderingsMapper;
}
private static Mapper parametersReverseMapper = null;
public static Mapper zGetParameterizedPropertyParametersReverseMapper()
{
if (parametersReverseMapper == null)
{
parametersReverseMapper = zConstructParameterizedPropertyParametersReverseMapper();
}
return parametersReverseMapper;
}
private static Mapper parametersMapper = null;
public static Mapper zGetParameterizedPropertyParametersMapper()
{
if (parametersMapper == null)
{
parametersMapper = zConstructParameterizedPropertyParametersMapper();
}
return parametersMapper;
}
private static Mapper parametersPureReverseMapper = null;
public static Mapper zGetParameterizedPropertyParametersPureReverseMapper()
{
if (parametersPureReverseMapper == null)
{
parametersPureReverseMapper = zConstructParameterizedPropertyParametersPureReverseMapper();
}
return parametersPureReverseMapper;
}
private static Mapper zConstructParameterizedPropertyParametersPureReverseMapper()
{
InternalList parametersMapperMapperList = new InternalList(2);
parametersMapperMapperList.add(ParameterizedPropertyFinder.zGetConstantJoin(6));
parametersMapperMapperList.add(ParameterizedPropertyFinder.zGetConstantJoin(8));
Mapper parametersMapper = new MultiEqualityMapper(parametersMapperMapperList);
parametersMapper.setName("parameters");
return parametersMapper;
}
private static Mapper zConstructParameterizedPropertyParametersReverseMapper()
{
InternalList parametersMapperMapperList = new InternalList(2);
parametersMapperMapperList.add(ParameterizedPropertyFinder.zGetConstantJoin(6));
parametersMapperMapperList.add(ParameterizedPropertyFinder.zGetConstantJoin(8));
Mapper parametersMapper = new MultiEqualityMapper(parametersMapperMapperList);
parametersMapper.setName("parameters");
return parametersMapper;
}
private static Mapper zConstructParameterizedPropertyParametersMapper()
{
InternalList parametersMapperMapperList = new InternalList(2);
parametersMapperMapperList.add(ParameterizedPropertyFinder.zGetConstantJoin(7));
parametersMapperMapperList.add(ParameterizedPropertyFinder.zGetConstantJoin(9));
Mapper parametersMapper = new MultiEqualityMapper(parametersMapperMapperList);
parametersMapper.setName("parameterizedProperty");
return parametersMapper;
}
/** maps to \"PARAMETERIZED_PROPERTY\".\"OWNING_CLASS_NAME\" **/
public static StringAttribute owningClassName()
{
return finder.owningClassName();
}
/** maps to \"PARAMETERIZED_PROPERTY\".\"NAME\" **/
public static StringAttribute name()
{
return finder.name();
}
/** maps to \"PARAMETERIZED_PROPERTY\".\"RESULT_TYPE_NAME\" **/
public static StringAttribute resultTypeName()
{
return finder.resultTypeName();
}
/** maps to \"PARAMETERIZED_PROPERTY\".\"ORDINAL\" **/
public static IntegerAttribute ordinal()
{
return finder.ordinal();
}
/** maps to \"PARAMETERIZED_PROPERTY\".\"MULTIPLICITY\" **/
public static StringAttribute multiplicity()
{
return finder.multiplicity();
}
public static KlassFinder.KlassSingleFinderForRelatedClasses resultType()
{
return finder.resultType();
}
public static class ParameterizedPropertyResultTypeFinderSubclass extends KlassFinder.KlassSingleFinderForRelatedClasses
{
public ParameterizedPropertyResultTypeFinderSubclass(Mapper mapper, NormalAndListValueSelector parentSelector )
{
super(mapper);
this._parentSelector = (AbstractRelatedFinder) parentSelector;
this._orderBy = null;
this._type = SIMPLE_TO_ONE;
this._name = "resultType";
}
public DeepRelationshipAttribute copy()
{
return new ParameterizedPropertyResultTypeFinderSubclass(zGetMapper(), (NormalAndListValueSelector) this._parentSelector
);
}
protected Klass plainValueOf(ParameterizedProperty _obj)
{
return _obj.getResultType();
}
protected KlassList plainListValueOf(Object _obj)
{
return ((ParameterizedPropertyList)_obj).getResultTypes();
}
}
public static ParameterizedPropertyOrderingFinder.ParameterizedPropertyOrderingCollectionFinderForRelatedClasses parameterizedPropertyOrderings()
{
return finder.parameterizedPropertyOrderings();
}
public static class ParameterizedPropertyParameterizedPropertyOrderingsFinderSubclass extends ParameterizedPropertyOrderingFinder.ParameterizedPropertyOrderingCollectionFinderForRelatedClasses
{
public ParameterizedPropertyParameterizedPropertyOrderingsFinderSubclass(Mapper mapper, NormalAndListValueSelector parentSelector )
{
super(mapper);
this._parentSelector = (AbstractRelatedFinder) parentSelector;
this._orderBy = ParameterizedPropertyOrderingFinder.ordinal().ascendingOrderBy();
this._type = SIMPLE_TO_MANY;
this._name = "parameterizedPropertyOrderings";
this.zSetRelationshipMultiExtractor(RelationshipMultiExtractor.withLeftAttributes(
ParameterizedPropertyOrderingFinder.owningClassName(),
ParameterizedPropertyOrderingFinder.name()).withExtractors(
ParameterizedPropertyFinder.owningClassName(),
ParameterizedPropertyFinder.name()));
}
public DeepRelationshipAttribute copy()
{
return new ParameterizedPropertyParameterizedPropertyOrderingsFinderSubclass(zGetMapper(), (NormalAndListValueSelector) this._parentSelector
);
}
public boolean isModifiedSinceDetachment(MithraTransactionalObject _obj)
{
return ((ParameterizedProperty) _obj).isParameterizedPropertyOrderingsModifiedSinceDetachment();
}
protected ParameterizedPropertyOrderingList plainValueOf(ParameterizedProperty _obj)
{
return _obj.getParameterizedPropertyOrderings();
}
protected ParameterizedPropertyOrderingList plainListValueOf(Object _obj)
{
return ((ParameterizedPropertyList)_obj).getParameterizedPropertyOrderings();
}
}
public static ParameterizedPropertyParameterFinder.ParameterizedPropertyParameterCollectionFinderForRelatedClasses parameters()
{
return finder.parameters();
}
public static class ParameterizedPropertyParametersFinderSubclass extends ParameterizedPropertyParameterFinder.ParameterizedPropertyParameterCollectionFinderForRelatedClasses
{
public ParameterizedPropertyParametersFinderSubclass(Mapper mapper, NormalAndListValueSelector parentSelector )
{
super(mapper);
this._parentSelector = (AbstractRelatedFinder) parentSelector;
this._orderBy = ParameterizedPropertyParameterFinder.ordinal().ascendingOrderBy();
this._type = SIMPLE_TO_MANY;
this._name = "parameters";
this.zSetRelationshipMultiExtractor(RelationshipMultiExtractor.withLeftAttributes(
ParameterizedPropertyParameterFinder.classifierName(),
ParameterizedPropertyParameterFinder.parameterizedPropertyName()).withExtractors(
ParameterizedPropertyFinder.owningClassName(),
ParameterizedPropertyFinder.name()));
}
public DeepRelationshipAttribute copy()
{
return new ParameterizedPropertyParametersFinderSubclass(zGetMapper(), (NormalAndListValueSelector) this._parentSelector
);
}
protected ParameterizedPropertyParameterList plainValueOf(ParameterizedProperty _obj)
{
return _obj.getParameters();
}
protected ParameterizedPropertyParameterList plainListValueOf(Object _obj)
{
return ((ParameterizedPropertyList)_obj).getParameters();
}
}
public static KlassFinder.KlassSingleFinderForRelatedClasses owningClass()
{
return finder.owningClass();
}
public static class ParameterizedPropertyOwningClassFinderSubclass extends KlassFinder.KlassSingleFinderForRelatedClasses
{
public ParameterizedPropertyOwningClassFinderSubclass(Mapper mapper, NormalAndListValueSelector parentSelector )
{
super(mapper);
this._parentSelector = (AbstractRelatedFinder) parentSelector;
this._orderBy = null;
this._type = SIMPLE_TO_ONE;
this._name = "owningClass";
}
public DeepRelationshipAttribute copy()
{
return new ParameterizedPropertyOwningClassFinderSubclass(zGetMapper(), (NormalAndListValueSelector) this._parentSelector
);
}
protected Klass plainValueOf(ParameterizedProperty _obj)
{
return _obj.getOwningClass();
}
protected KlassList plainListValueOf(Object _obj)
{
return ((ParameterizedPropertyList)_obj).getOwningClass();
}
}
public static Operation eq(ParameterizedProperty other)
{
return finder.eq(other);
}
public static Operation all()
{
return new All(owningClassName());
}
public static ParameterizedPropertySingleFinder getFinderInstance()
{
return finder;
}
public static Attribute[] getPrimaryKeyAttributes()
{
return new Attribute[]
{
owningClassName()
, name()
}
;
}
public static Attribute[] getImmutableAttributes()
{
return new Attribute[]
{
owningClassName()
, owningClassName()
, name()
}
;
}
public static AsOfAttribute[] getAsOfAttributes()
{
return null;
}
protected static void initializeIndicies(Cache cache)
{
cache.addIndex("0 Index", new Attribute[]
{
owningClassName()
}
);
cache.addIndex("1 Index", new Attribute[]
{
resultTypeName()
}
);
}
protected static void initializePortal(MithraObjectDeserializer objectFactory, Cache cache,
MithraConfigurationManager.Config config)
{
initializeIndicies(cache);
isFullCache = cache.isFullCache();
isOffHeap = cache.isOffHeap();
MithraObjectPortal portal;
if (config.isParticipatingInTx())
{
portal = new MithraTransactionalPortal(objectFactory, cache, getFinderInstance(),
config.getRelationshipCacheSize(), config.getMinQueriesToKeep(), null,
null, null, 0,
(MithraObjectPersister) objectFactory);
}
else
{
portal = new MithraReadOnlyPortal(objectFactory, cache, getFinderInstance(),
config.getRelationshipCacheSize(), config.getMinQueriesToKeep(), null,
null, null, 0,
(MithraObjectPersister) objectFactory);
}
portal.setParentFinders(new RelatedFinder[]
{
KlassFinder.getFinderInstance(),
}
);
config.initializePortal(portal);
objectPortal.destroy();
objectPortal = portal;
}
protected static void initializeClientPortal(MithraObjectDeserializer objectFactory, Cache cache,
MithraConfigurationManager.Config config)
{
initializeIndicies(cache);
isFullCache = cache.isFullCache();
isOffHeap = cache.isOffHeap();
MithraObjectPortal portal;
if (config.isParticipatingInTx())
{
portal = new MithraTransactionalPortal(objectFactory, cache, getFinderInstance(),
config.getRelationshipCacheSize(), config.getMinQueriesToKeep(),
null, null,
null, 0,
new RemoteMithraObjectPersister(config.getRemoteMithraService(), getFinderInstance(), false));
}
else
{
portal = new MithraReadOnlyPortal(objectFactory, cache, getFinderInstance(),
config.getRelationshipCacheSize(), config.getMinQueriesToKeep(),
null, null,
null, 0,
new RemoteMithraObjectPersister(config.getRemoteMithraService(), getFinderInstance(), false));
}
portal.setParentFinders(new RelatedFinder[]
{
KlassFinder.getFinderInstance(),
}
);
config.initializePortal(portal);
objectPortal.destroy();
objectPortal = portal;
}
public static boolean isFullCache()
{
return isFullCache;
}
public static boolean isOffHeap()
{
return isOffHeap;
}
public static Attribute getAttributeByName(String attributeName)
{
return finder.getAttributeByName(attributeName);
}
public static com.gs.fw.common.mithra.extractor.Function getAttributeOrRelationshipSelector(String attributeName)
{
return finder.getAttributeOrRelationshipSelector(attributeName);
}
public static RelatedFinder getRelatedFinderByName(String relationshipName)
{
return finder.getRelationshipFinderByName(relationshipName);
}
public static DoubleAttribute[] zGetDoubleAttributes()
{
DoubleAttribute[] result = new DoubleAttribute[0];
return result;
}
public static BigDecimalAttribute[] zGetBigDecimalAttributes()
{
BigDecimalAttribute[] result = new BigDecimalAttribute[0];
return result;
}
public static void zResetPortal()
{
objectPortal.destroy();
objectPortal = new UninitializedPortal("klass.model.meta.domain.ParameterizedProperty");
isFullCache = false;
isOffHeap = false;
}
public static void setTransactionModeFullTransactionParticipation(MithraTransaction tx)
{
tx.setTxParticipationMode(objectPortal, FullTransactionalParticipationMode.getInstance());
}
public static void setTransactionModeReadCacheUpdateCausesRefreshAndLock(MithraTransaction tx)
{
tx.setTxParticipationMode(objectPortal, ReadCacheUpdateCausesRefreshAndLockTxParticipationMode.getInstance());
}
public static void registerForNotification(MithraApplicationClassLevelNotificationListener listener)
{
getMithraObjectPortal().registerForApplicationClassLevelNotification(listener);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy