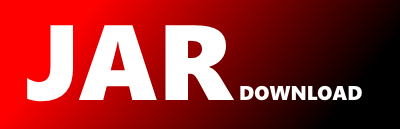
klass.model.meta.domain.ProjectionElementAbstract Maven / Gradle / Ivy
The newest version!
package klass.model.meta.domain;
import java.math.BigDecimal;
import java.sql.Timestamp;
import java.util.*;
import java.io.*;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.gs.fw.common.mithra.*;
import com.gs.fw.common.mithra.attribute.*;
import com.gs.fw.common.mithra.util.*;
import com.gs.fw.common.mithra.notification.*;
import com.gs.fw.common.mithra.notification.listener.*;
import com.gs.fw.common.mithra.list.cursor.Cursor;
import com.gs.fw.common.mithra.bulkloader.*;
import com.gs.fw.common.mithra.*;
import com.gs.fw.common.mithra.behavior.*;
import com.gs.fw.common.mithra.cache.Cache;
import com.gs.fw.common.mithra.extractor.*;
import com.gs.fw.common.mithra.finder.*;
import com.gs.fw.common.mithra.list.*;
import com.gs.fw.common.mithra.behavior.state.PersistenceState;
import com.gs.fw.common.mithra.attribute.update.*;
import com.gs.fw.common.mithra.transaction.MithraObjectPersister;
import java.util.Arrays;
import java.util.HashSet;
/**
* This file was automatically generated using Mithra 18.0.0. Please do not modify it.
* Add custom logic to its subclass instead.
*/
// Generated from templates/transactional/Abstract.jsp
public abstract class ProjectionElementAbstract extends com.gs.fw.common.mithra.superclassimpl.MithraTransactionalObjectImpl
implements
NamedElement
{
private static byte MEMORY_STATE = PersistenceState.IN_MEMORY;
private static byte PERSISTED_STATE = PersistenceState.PERSISTED;
private static final Logger logger = LoggerFactory.getLogger(ProjectionElement.class.getName());
private static final RelationshipHashStrategy forrootProjectionSubClass = new RootProjectionSubClassRhs();
private static final RelationshipHashStrategy forprojectionWithAssociationEndSubClass = new ProjectionWithAssociationEndSubClassRhs();
private static final RelationshipHashStrategy forprojectionDataTypePropertySubClass = new ProjectionDataTypePropertySubClassRhs();
private static final RelationshipHashStrategy forparent = new ParentRhs();
private static final class RootProjectionSubClassRhs implements RelationshipHashStrategy
{
public boolean equalsForRelationship(Object _srcObject, Object _srcData, Object _targetData, Timestamp _asOfDate0, Timestamp _asOfDate1)
{
ProjectionElementData _castedSrcData = (ProjectionElementData) _srcData;
RootProjectionData _castedTargetData = (RootProjectionData) _targetData;
if (_castedSrcData.getId() == _castedTargetData.getId())
{
return true;
}
return false;
}
public int computeHashCodeFromRelated(Object _srcObject, Object _srcData)
{
ProjectionElementData _castedSrcData = (ProjectionElementData) _srcData;
return HashUtil.hash(_castedSrcData.getId());
}
public int computeOffHeapHashCodeFromRelated(Object _srcObject, Object _srcData)
{
return computeHashCodeFromRelated(_srcObject, _srcData);
}
}
private static final class ProjectionWithAssociationEndSubClassRhs implements RelationshipHashStrategy
{
public boolean equalsForRelationship(Object _srcObject, Object _srcData, Object _targetData, Timestamp _asOfDate0, Timestamp _asOfDate1)
{
ProjectionElementData _castedSrcData = (ProjectionElementData) _srcData;
ProjectionWithAssociationEndData _castedTargetData = (ProjectionWithAssociationEndData) _targetData;
if (_castedSrcData.getId() == _castedTargetData.getId())
{
return true;
}
return false;
}
public int computeHashCodeFromRelated(Object _srcObject, Object _srcData)
{
ProjectionElementData _castedSrcData = (ProjectionElementData) _srcData;
return HashUtil.hash(_castedSrcData.getId());
}
public int computeOffHeapHashCodeFromRelated(Object _srcObject, Object _srcData)
{
return computeHashCodeFromRelated(_srcObject, _srcData);
}
}
private static final class ProjectionDataTypePropertySubClassRhs implements RelationshipHashStrategy
{
public boolean equalsForRelationship(Object _srcObject, Object _srcData, Object _targetData, Timestamp _asOfDate0, Timestamp _asOfDate1)
{
ProjectionElementData _castedSrcData = (ProjectionElementData) _srcData;
ProjectionDataTypePropertyData _castedTargetData = (ProjectionDataTypePropertyData) _targetData;
if (_castedSrcData.getId() == _castedTargetData.getId())
{
return true;
}
return false;
}
public int computeHashCodeFromRelated(Object _srcObject, Object _srcData)
{
ProjectionElementData _castedSrcData = (ProjectionElementData) _srcData;
return HashUtil.hash(_castedSrcData.getId());
}
public int computeOffHeapHashCodeFromRelated(Object _srcObject, Object _srcData)
{
return computeHashCodeFromRelated(_srcObject, _srcData);
}
}
private static final class ParentRhs implements RelationshipHashStrategy
{
public boolean equalsForRelationship(Object _srcObject, Object _srcData, Object _targetData, Timestamp _asOfDate0, Timestamp _asOfDate1)
{
ProjectionElementData _castedSrcData = (ProjectionElementData) _srcData;
ProjectionElementData _castedTargetData = (ProjectionElementData) _targetData;
if (!_castedSrcData.isParentIdNull() && _castedSrcData.getParentId() == _castedTargetData.getId())
{
return true;
}
return false;
}
public int computeHashCodeFromRelated(Object _srcObject, Object _srcData)
{
ProjectionElementData _castedSrcData = (ProjectionElementData) _srcData;
return HashUtil.hash(_castedSrcData.getParentId(), _castedSrcData.isParentIdNull());
}
public int computeOffHeapHashCodeFromRelated(Object _srcObject, Object _srcData)
{
return computeHashCodeFromRelated(_srcObject, _srcData);
}
}
public ProjectionElementAbstract()
{
this.persistenceState = MEMORY_STATE;
}
public ProjectionElement getDetachedCopy() throws MithraBusinessException
{
return (ProjectionElement) super.getDetachedCopy();
}
public ProjectionElement getNonPersistentCopy() throws MithraBusinessException
{
ProjectionElement result = (ProjectionElement) super.getNonPersistentCopy();
result.persistenceState = MEMORY_STATE;
return result;
}
public void insert() throws MithraBusinessException
{
TransactionalBehavior behavior = zGetTransactionalBehaviorForWriteWithWaitIfNecessary();
this.checkAndGeneratePrimaryKeys();
behavior.insert(this);
}
public ProjectionElement copyDetachedValuesToOriginalOrInsertIfNew()
{
return (ProjectionElement) this.zCopyDetachedValuesToOriginalOrInsertIfNew();
}
public ProjectionElement zFindOriginal()
{
ProjectionElementData data = (ProjectionElementData) this.currentData;
Operation op;
op = ProjectionElementFinder.id().eq(data.getId());
return ProjectionElementFinder.findOne(op);
}
public boolean isModifiedSinceDetachmentByDependentRelationships()
{
if(this.isModifiedSinceDetachment()) return true;
if(isRootProjectionSubClassModifiedSinceDetachment()) return true;
if(isProjectionWithAssociationEndSubClassModifiedSinceDetachment()) return true;
if(isProjectionDataTypePropertySubClassModifiedSinceDetachment()) return true;
return false;
}
private Logger getLogger()
{
return logger;
}
public MithraDataObject zAllocateData()
{
return new ProjectionElementData();
}
protected void zSetFromProjectionElementData( ProjectionElementData data )
{
super.zSetData(data);
this.persistenceState = PERSISTED_STATE;
}
public void setFromProjectionElementData( ProjectionElementData data )
{
super.zSetData(data);
}
public void zWriteDataClassName(ObjectOutput out) throws IOException
{
}
public final boolean isIdNull()
{
return ((ProjectionElementData) this.zSynchronizedGetData()).isIdNull();
}
public final long getId()
{
ProjectionElementData data = (ProjectionElementData) this.zSynchronizedGetData();
return data.getId();
}
public void setId(long newValue)
{
MithraDataObject d = zSetLong(ProjectionElementFinder.id(), newValue, true, false ,false);
if (d == null) return;
ProjectionElementData data = (ProjectionElementData) d;
TransactionalBehavior _behavior = zGetTransactionalBehaviorForWriteWithWaitIfNecessary();
if (!_behavior.isPersisted())
{
RootProjection rootProjectionSubClass =
(RootProjection ) data.getRootProjectionSubClass();
if (rootProjectionSubClass != null)
{
rootProjectionSubClass.setId(newValue);
}
ProjectionWithAssociationEnd projectionWithAssociationEndSubClass =
(ProjectionWithAssociationEnd ) data.getProjectionWithAssociationEndSubClass();
if (projectionWithAssociationEndSubClass != null)
{
projectionWithAssociationEndSubClass.setId(newValue);
}
ProjectionDataTypeProperty projectionDataTypePropertySubClass =
(ProjectionDataTypeProperty ) data.getProjectionDataTypePropertySubClass();
if (projectionDataTypePropertySubClass != null)
{
projectionDataTypePropertySubClass.setId(newValue);
}
}
}
public boolean isNameNull()
{
return ((ProjectionElementData) this.zSynchronizedGetData()).isNameNull();
}
public String getName()
{
ProjectionElementData data = (ProjectionElementData) this.zSynchronizedGetData();
return data.getName();
}
public void setName(String newValue)
{
if (newValue != null && newValue.length() > 256)
throw new MithraBusinessException("Attribute 'name' cannot exceed maximum length of 256: " + newValue);
zSetString(ProjectionElementFinder.name(), newValue, false, false );
}
public boolean isOrdinalNull()
{
return ((ProjectionElementData) this.zSynchronizedGetData()).isOrdinalNull();
}
public int getOrdinal()
{
ProjectionElementData data = (ProjectionElementData) this.zSynchronizedGetData();
return data.getOrdinal();
}
public void setOrdinal(int newValue)
{
zSetInteger(ProjectionElementFinder.ordinal(), newValue, false, false ,false);
}
public boolean isParentIdNull()
{
return ((ProjectionElementData) this.zSynchronizedGetData()).isParentIdNull();
}
public long getParentId()
{
ProjectionElementData data = (ProjectionElementData) this.zSynchronizedGetData();
if (data.isParentIdNull()) MithraNullPrimitiveException.throwNew("parentId", data);
return data.getParentId();
}
public void setParentId(long newValue)
{
zSetLong(ProjectionElementFinder.parentId(), newValue, false, false ,true);
}
protected void issuePrimitiveNullSetters(TransactionalBehavior behavior, MithraDataObject data)
{
zNullify(behavior, data, ProjectionElementFinder.parentId(), false);
}
public void setParentIdNull()
{
zNullify(ProjectionElementFinder.parentId(), false);
}
public void zPersistDetachedRelationships(MithraDataObject _data)
{
ProjectionElementData _newData = (ProjectionElementData) _data;
if (_newData.getRootProjectionSubClass() instanceof NulledRelation)
{
RootProjection rootProjectionSubClass =
this.getRootProjectionSubClass();
if (rootProjectionSubClass != null)
{
rootProjectionSubClass.cascadeDelete();
}
}
else
{
RootProjection rootProjectionSubClass =
(RootProjection) _newData.getRootProjectionSubClass();
if (rootProjectionSubClass != null)
{
RootProjection _existing =
this.getRootProjectionSubClass();
if (_existing == null)
{
rootProjectionSubClass.copyDetachedValuesToOriginalOrInsertIfNew();
}
else
{
_existing.zCopyAttributesFrom(rootProjectionSubClass.zGetTxDataForRead());
_existing.zPersistDetachedRelationships(rootProjectionSubClass.zGetTxDataForRead());
}
}
}
if (_newData.getProjectionWithAssociationEndSubClass() instanceof NulledRelation)
{
ProjectionWithAssociationEnd projectionWithAssociationEndSubClass =
this.getProjectionWithAssociationEndSubClass();
if (projectionWithAssociationEndSubClass != null)
{
projectionWithAssociationEndSubClass.cascadeDelete();
}
}
else
{
ProjectionWithAssociationEnd projectionWithAssociationEndSubClass =
(ProjectionWithAssociationEnd) _newData.getProjectionWithAssociationEndSubClass();
if (projectionWithAssociationEndSubClass != null)
{
ProjectionWithAssociationEnd _existing =
this.getProjectionWithAssociationEndSubClass();
if (_existing == null)
{
projectionWithAssociationEndSubClass.copyDetachedValuesToOriginalOrInsertIfNew();
}
else
{
_existing.zCopyAttributesFrom(projectionWithAssociationEndSubClass.zGetTxDataForRead());
_existing.zPersistDetachedRelationships(projectionWithAssociationEndSubClass.zGetTxDataForRead());
}
}
}
if (_newData.getProjectionDataTypePropertySubClass() instanceof NulledRelation)
{
ProjectionDataTypeProperty projectionDataTypePropertySubClass =
this.getProjectionDataTypePropertySubClass();
if (projectionDataTypePropertySubClass != null)
{
projectionDataTypePropertySubClass.cascadeDelete();
}
}
else
{
ProjectionDataTypeProperty projectionDataTypePropertySubClass =
(ProjectionDataTypeProperty) _newData.getProjectionDataTypePropertySubClass();
if (projectionDataTypePropertySubClass != null)
{
ProjectionDataTypeProperty _existing =
this.getProjectionDataTypePropertySubClass();
if (_existing == null)
{
projectionDataTypePropertySubClass.copyDetachedValuesToOriginalOrInsertIfNew();
}
else
{
_existing.zCopyAttributesFrom(projectionDataTypePropertySubClass.zGetTxDataForRead());
_existing.zPersistDetachedRelationships(projectionDataTypePropertySubClass.zGetTxDataForRead());
}
}
}
}
public void zSetTxDetachedDeleted()
{
TransactionalBehavior _behavior = zGetTransactionalBehaviorForWriteWithWaitIfNecessary();
if (_behavior.isDetached() && _behavior.isDeleted()) return;
ProjectionElementData _newData = (ProjectionElementData) _behavior.getCurrentDataForRead(this);
if (_newData.getRootProjectionSubClass() != null && !(_newData.getRootProjectionSubClass() instanceof NulledRelation))
{
((RootProjection)_newData.getRootProjectionSubClass()).zSetTxDetachedDeleted();
}
if (_newData.getProjectionWithAssociationEndSubClass() != null && !(_newData.getProjectionWithAssociationEndSubClass() instanceof NulledRelation))
{
((ProjectionWithAssociationEnd)_newData.getProjectionWithAssociationEndSubClass()).zSetTxDetachedDeleted();
}
if (_newData.getProjectionDataTypePropertySubClass() != null && !(_newData.getProjectionDataTypePropertySubClass() instanceof NulledRelation))
{
((ProjectionDataTypeProperty)_newData.getProjectionDataTypePropertySubClass()).zSetTxDetachedDeleted();
}
this.zSetTxPersistenceState(PersistenceState.DETACHED_DELETED);
}
public void zSetNonTxDetachedDeleted()
{
TransactionalBehavior _behavior = zGetTransactionalBehaviorForWriteWithWaitIfNecessary();
ProjectionElementData _newData = (ProjectionElementData) _behavior.getCurrentDataForRead(this);
if (_newData.getRootProjectionSubClass() != null && !(_newData.getRootProjectionSubClass() instanceof NulledRelation))
{
((RootProjection)_newData.getRootProjectionSubClass()).zSetNonTxDetachedDeleted();
}
if (_newData.getProjectionWithAssociationEndSubClass() != null && !(_newData.getProjectionWithAssociationEndSubClass() instanceof NulledRelation))
{
((ProjectionWithAssociationEnd)_newData.getProjectionWithAssociationEndSubClass()).zSetNonTxDetachedDeleted();
}
if (_newData.getProjectionDataTypePropertySubClass() != null && !(_newData.getProjectionDataTypePropertySubClass() instanceof NulledRelation))
{
((ProjectionDataTypeProperty)_newData.getProjectionDataTypePropertySubClass()).zSetNonTxDetachedDeleted();
}
this.zSetNonTxPersistenceState(PersistenceState.DETACHED_DELETED);
}
/**
* Relationship Expression:
this.id = ProjectionElement.parentId
* Order By: ordinal asc
.
* @see ProjectionElement#getParent() reverse relationship ProjectionElement.getParent()
* @return children
*/
public ProjectionElementList getChildren()
{
ProjectionElementList _result = null;
Operation _op = null;
TransactionalBehavior _behavior = zGetTransactionalBehaviorForReadWithWaitIfNecessary();
ProjectionElementData _data = (ProjectionElementData) _behavior.getCurrentDataForRead(this);
if (_behavior.isPersisted())
{
{
{
_op = ProjectionElementFinder.parentId().eq(_data.getId());
}
}
}
else if (_behavior.isDetached())
{
{
{
Operation detachedOp = ProjectionElementFinder.parentId().eq(_data.getId());
_result = new ProjectionElementList(detachedOp);
_result.zSetForRelationship();
}
_result.setOrderBy(ProjectionElementFinder.ordinal().ascendingOrderBy());
}
}
else if (_behavior.isInMemory())
{
_result = (ProjectionElementList) _data.getChildren();
if (_result == null)
{
{
_op = ProjectionElementFinder.parentId().eq(_data.getId());
}
}
}
if (_op != null)
{
_result = new ProjectionElementList(_op);
_result.zSetForRelationship();
_result.setOrderBy(ProjectionElementFinder.ordinal().ascendingOrderBy());
}
return _result;
}
public void setChildren(ProjectionElementList children)
{
ProjectionElementList _children = (ProjectionElementList) children;
TransactionalBehavior _behavior = zGetTransactionalBehaviorForWriteWithWaitIfNecessary();
ProjectionElementData _data = (ProjectionElementData) _behavior.getCurrentDataForWrite(this);
if (_behavior.isInMemory())
{
_data.setChildren(_children);
}
else if (_behavior.isPersisted())
{
_behavior.clearTempTransaction(this);
}
else throw new RuntimeException("not implemented");
}
/**
* Relationship Expression:
this.id = RootProjection.id
* @see RootProjection#getProjectionElementSuperClass() reverse relationship RootProjection.getProjectionElementSuperClass()
* @return The root projection sub class
*/
public RootProjection getRootProjectionSubClass()
{
RootProjection _result = null;
Operation _op = null;
TransactionalBehavior _behavior = zGetTransactionalBehaviorForReadWithWaitIfNecessary();
ProjectionElementData _data = (ProjectionElementData) _behavior.getCurrentDataForRead(this);
MithraObjectPortal _portal = null;
if (_behavior.isPersisted())
{
{
_portal = RootProjectionFinder.getMithraObjectPortal();
Object _related = _portal.getAsOneFromCache(this, _data, forrootProjectionSubClass, null, null);
if (!(_related instanceof NulledRelation)) _result = (RootProjection) _related;
if (_related == null)
{
_op = RootProjectionFinder.id().eq(_data.getId());
}
}
}
else if (_behavior.isDetached())
{
if (_data.getRootProjectionSubClass() instanceof NulledRelation)
{
return null;
}
_result = (RootProjection) _data.getRootProjectionSubClass();
if (_result == null)
{
{
Operation detachedOp = RootProjectionFinder.id().eq(_data.getId());
_result = RootProjectionFinder.zFindOneForRelationship(detachedOp);
if(_result != null)
{
_result = _result.getDetachedCopy();
}
}
_behavior = zGetTransactionalBehaviorForWriteWithWaitIfNecessary();
_data = (ProjectionElementData) _behavior.getCurrentDataForWrite(this);
_data.setRootProjectionSubClass(_result);
if (_result != null) _result.zSetParentContainerprojectionElementSuperClass(this);
}
}
else if (_behavior.isInMemory())
{
_result = (RootProjection) _data.getRootProjectionSubClass();
}
if (_op != null)
{
_result = RootProjectionFinder.zFindOneForRelationship(_op);
}
return _result;
}
public void setRootProjectionSubClass(RootProjection rootProjectionSubClass)
{
RootProjection _rootProjectionSubClass = (RootProjection) rootProjectionSubClass;
TransactionalBehavior _behavior = zGetTransactionalBehaviorForWriteWithWaitIfNecessary();
ProjectionElementData _data = (ProjectionElementData) _behavior.getCurrentDataForWrite(this);
if (_behavior.isInMemory())
{
Object _prev = _data.getRootProjectionSubClass();
_data.setRootProjectionSubClass(_rootProjectionSubClass);
if (_rootProjectionSubClass != null)
{
_rootProjectionSubClass.setId(_data.getId());
_rootProjectionSubClass.zSetParentContainerprojectionElementSuperClass(this);
}
else if (_behavior.isDetached())
{
_data.setRootProjectionSubClass(NulledRelation.create(_prev));
if (_prev != null && !(_prev instanceof NulledRelation)
&& (!((MithraTransactionalObject)_prev).isInMemoryAndNotInserted() || ((MithraTransactionalObject)_prev).zIsDetached()))
{
((MithraTransactionalObject)_prev).delete();
}
}
}
else if (_behavior.isPersisted())
{
_behavior.clearTempTransaction(this);
RootProjection _existing = this.getRootProjectionSubClass();
if (_rootProjectionSubClass != _existing)
{
if (_existing != null)
{
_existing.cascadeDelete();
}
if (_rootProjectionSubClass != null)
{
_rootProjectionSubClass.setId(_data.getId());
_rootProjectionSubClass.cascadeInsert();
}
}
}
else throw new RuntimeException("not implemented");
}
public boolean isRootProjectionSubClassModifiedSinceDetachment()
{
TransactionalBehavior _behavior = zGetTransactionalBehaviorForReadWithWaitIfNecessary();
ProjectionElementData _data = (ProjectionElementData) _behavior.getCurrentDataForRead(this);
if (_data.getRootProjectionSubClass() instanceof NulledRelation)
{
RootProjection _existing = this.getOriginalPersistentObject().getRootProjectionSubClass();
return _existing != null;
}
RootProjection rootProjectionSubClass =
(RootProjection) _data.getRootProjectionSubClass();
if( rootProjectionSubClass != null)
{
return rootProjectionSubClass.isModifiedSinceDetachment();
}
return false;
}
/**
* Relationship Expression:
this.id = ProjectionWithAssociationEnd.id
* @see ProjectionWithAssociationEnd#getProjectionElementSuperClass() reverse relationship ProjectionWithAssociationEnd.getProjectionElementSuperClass()
* @return The projection with association end sub class
*/
public ProjectionWithAssociationEnd getProjectionWithAssociationEndSubClass()
{
ProjectionWithAssociationEnd _result = null;
Operation _op = null;
TransactionalBehavior _behavior = zGetTransactionalBehaviorForReadWithWaitIfNecessary();
ProjectionElementData _data = (ProjectionElementData) _behavior.getCurrentDataForRead(this);
MithraObjectPortal _portal = null;
if (_behavior.isPersisted())
{
{
_portal = ProjectionWithAssociationEndFinder.getMithraObjectPortal();
Object _related = _portal.getAsOneFromCache(this, _data, forprojectionWithAssociationEndSubClass, null, null);
if (!(_related instanceof NulledRelation)) _result = (ProjectionWithAssociationEnd) _related;
if (_related == null)
{
_op = ProjectionWithAssociationEndFinder.id().eq(_data.getId());
}
}
}
else if (_behavior.isDetached())
{
if (_data.getProjectionWithAssociationEndSubClass() instanceof NulledRelation)
{
return null;
}
_result = (ProjectionWithAssociationEnd) _data.getProjectionWithAssociationEndSubClass();
if (_result == null)
{
{
Operation detachedOp = ProjectionWithAssociationEndFinder.id().eq(_data.getId());
_result = ProjectionWithAssociationEndFinder.zFindOneForRelationship(detachedOp);
if(_result != null)
{
_result = _result.getDetachedCopy();
}
}
_behavior = zGetTransactionalBehaviorForWriteWithWaitIfNecessary();
_data = (ProjectionElementData) _behavior.getCurrentDataForWrite(this);
_data.setProjectionWithAssociationEndSubClass(_result);
if (_result != null) _result.zSetParentContainerprojectionElementSuperClass(this);
}
}
else if (_behavior.isInMemory())
{
_result = (ProjectionWithAssociationEnd) _data.getProjectionWithAssociationEndSubClass();
}
if (_op != null)
{
_result = ProjectionWithAssociationEndFinder.zFindOneForRelationship(_op);
}
return _result;
}
public void setProjectionWithAssociationEndSubClass(ProjectionWithAssociationEnd projectionWithAssociationEndSubClass)
{
ProjectionWithAssociationEnd _projectionWithAssociationEndSubClass = (ProjectionWithAssociationEnd) projectionWithAssociationEndSubClass;
TransactionalBehavior _behavior = zGetTransactionalBehaviorForWriteWithWaitIfNecessary();
ProjectionElementData _data = (ProjectionElementData) _behavior.getCurrentDataForWrite(this);
if (_behavior.isInMemory())
{
Object _prev = _data.getProjectionWithAssociationEndSubClass();
_data.setProjectionWithAssociationEndSubClass(_projectionWithAssociationEndSubClass);
if (_projectionWithAssociationEndSubClass != null)
{
_projectionWithAssociationEndSubClass.setId(_data.getId());
_projectionWithAssociationEndSubClass.zSetParentContainerprojectionElementSuperClass(this);
}
else if (_behavior.isDetached())
{
_data.setProjectionWithAssociationEndSubClass(NulledRelation.create(_prev));
if (_prev != null && !(_prev instanceof NulledRelation)
&& (!((MithraTransactionalObject)_prev).isInMemoryAndNotInserted() || ((MithraTransactionalObject)_prev).zIsDetached()))
{
((MithraTransactionalObject)_prev).delete();
}
}
}
else if (_behavior.isPersisted())
{
_behavior.clearTempTransaction(this);
ProjectionWithAssociationEnd _existing = this.getProjectionWithAssociationEndSubClass();
if (_projectionWithAssociationEndSubClass != _existing)
{
if (_existing != null)
{
_existing.cascadeDelete();
}
if (_projectionWithAssociationEndSubClass != null)
{
_projectionWithAssociationEndSubClass.setId(_data.getId());
_projectionWithAssociationEndSubClass.cascadeInsert();
}
}
}
else throw new RuntimeException("not implemented");
}
public boolean isProjectionWithAssociationEndSubClassModifiedSinceDetachment()
{
TransactionalBehavior _behavior = zGetTransactionalBehaviorForReadWithWaitIfNecessary();
ProjectionElementData _data = (ProjectionElementData) _behavior.getCurrentDataForRead(this);
if (_data.getProjectionWithAssociationEndSubClass() instanceof NulledRelation)
{
ProjectionWithAssociationEnd _existing = this.getOriginalPersistentObject().getProjectionWithAssociationEndSubClass();
return _existing != null;
}
ProjectionWithAssociationEnd projectionWithAssociationEndSubClass =
(ProjectionWithAssociationEnd) _data.getProjectionWithAssociationEndSubClass();
if( projectionWithAssociationEndSubClass != null)
{
return projectionWithAssociationEndSubClass.isModifiedSinceDetachment();
}
return false;
}
/**
* Relationship Expression:
this.id = ProjectionDataTypeProperty.id
* @see ProjectionDataTypeProperty#getProjectionElementSuperClass() reverse relationship ProjectionDataTypeProperty.getProjectionElementSuperClass()
* @return The projection data type property sub class
*/
public ProjectionDataTypeProperty getProjectionDataTypePropertySubClass()
{
ProjectionDataTypeProperty _result = null;
Operation _op = null;
TransactionalBehavior _behavior = zGetTransactionalBehaviorForReadWithWaitIfNecessary();
ProjectionElementData _data = (ProjectionElementData) _behavior.getCurrentDataForRead(this);
MithraObjectPortal _portal = null;
if (_behavior.isPersisted())
{
{
_portal = ProjectionDataTypePropertyFinder.getMithraObjectPortal();
Object _related = _portal.getAsOneFromCache(this, _data, forprojectionDataTypePropertySubClass, null, null);
if (!(_related instanceof NulledRelation)) _result = (ProjectionDataTypeProperty) _related;
if (_related == null)
{
_op = ProjectionDataTypePropertyFinder.id().eq(_data.getId());
}
}
}
else if (_behavior.isDetached())
{
if (_data.getProjectionDataTypePropertySubClass() instanceof NulledRelation)
{
return null;
}
_result = (ProjectionDataTypeProperty) _data.getProjectionDataTypePropertySubClass();
if (_result == null)
{
{
Operation detachedOp = ProjectionDataTypePropertyFinder.id().eq(_data.getId());
_result = ProjectionDataTypePropertyFinder.zFindOneForRelationship(detachedOp);
if(_result != null)
{
_result = _result.getDetachedCopy();
}
}
_behavior = zGetTransactionalBehaviorForWriteWithWaitIfNecessary();
_data = (ProjectionElementData) _behavior.getCurrentDataForWrite(this);
_data.setProjectionDataTypePropertySubClass(_result);
if (_result != null) _result.zSetParentContainerprojectionElementSuperClass(this);
}
}
else if (_behavior.isInMemory())
{
_result = (ProjectionDataTypeProperty) _data.getProjectionDataTypePropertySubClass();
}
if (_op != null)
{
_result = ProjectionDataTypePropertyFinder.zFindOneForRelationship(_op);
}
return _result;
}
public void setProjectionDataTypePropertySubClass(ProjectionDataTypeProperty projectionDataTypePropertySubClass)
{
ProjectionDataTypeProperty _projectionDataTypePropertySubClass = (ProjectionDataTypeProperty) projectionDataTypePropertySubClass;
TransactionalBehavior _behavior = zGetTransactionalBehaviorForWriteWithWaitIfNecessary();
ProjectionElementData _data = (ProjectionElementData) _behavior.getCurrentDataForWrite(this);
if (_behavior.isInMemory())
{
Object _prev = _data.getProjectionDataTypePropertySubClass();
_data.setProjectionDataTypePropertySubClass(_projectionDataTypePropertySubClass);
if (_projectionDataTypePropertySubClass != null)
{
_projectionDataTypePropertySubClass.setId(_data.getId());
_projectionDataTypePropertySubClass.zSetParentContainerprojectionElementSuperClass(this);
}
else if (_behavior.isDetached())
{
_data.setProjectionDataTypePropertySubClass(NulledRelation.create(_prev));
if (_prev != null && !(_prev instanceof NulledRelation)
&& (!((MithraTransactionalObject)_prev).isInMemoryAndNotInserted() || ((MithraTransactionalObject)_prev).zIsDetached()))
{
((MithraTransactionalObject)_prev).delete();
}
}
}
else if (_behavior.isPersisted())
{
_behavior.clearTempTransaction(this);
ProjectionDataTypeProperty _existing = this.getProjectionDataTypePropertySubClass();
if (_projectionDataTypePropertySubClass != _existing)
{
if (_existing != null)
{
_existing.cascadeDelete();
}
if (_projectionDataTypePropertySubClass != null)
{
_projectionDataTypePropertySubClass.setId(_data.getId());
_projectionDataTypePropertySubClass.cascadeInsert();
}
}
}
else throw new RuntimeException("not implemented");
}
public boolean isProjectionDataTypePropertySubClassModifiedSinceDetachment()
{
TransactionalBehavior _behavior = zGetTransactionalBehaviorForReadWithWaitIfNecessary();
ProjectionElementData _data = (ProjectionElementData) _behavior.getCurrentDataForRead(this);
if (_data.getProjectionDataTypePropertySubClass() instanceof NulledRelation)
{
ProjectionDataTypeProperty _existing = this.getOriginalPersistentObject().getProjectionDataTypePropertySubClass();
return _existing != null;
}
ProjectionDataTypeProperty projectionDataTypePropertySubClass =
(ProjectionDataTypeProperty) _data.getProjectionDataTypePropertySubClass();
if( projectionDataTypePropertySubClass != null)
{
return projectionDataTypePropertySubClass.isModifiedSinceDetachment();
}
return false;
}
/**
* Relationship Expression:
ProjectionElement.id = this.parentId
* @see ProjectionElement#getChildren() reverse relationship ProjectionElement.getChildren()
* @return The parent
*/
public ProjectionElement getParent()
{
ProjectionElement _result = null;
Operation _op = null;
TransactionalBehavior _behavior = zGetTransactionalBehaviorForReadWithWaitIfNecessary();
ProjectionElementData _data = (ProjectionElementData) _behavior.getCurrentDataForRead(this);
MithraObjectPortal _portal = null;
if (_behavior.isPersisted())
{
if (!_data.isParentIdNull())
{
_portal = ProjectionElementFinder.getMithraObjectPortal();
Object _related = _portal.getAsOneFromCache(this, _data, forparent, null, null);
if (!(_related instanceof NulledRelation)) _result = (ProjectionElement) _related;
if (_related == null)
{
_op = ProjectionElementFinder.id().eq(_data.getParentId());
}
}
else
{
return null;
}
}
else if (_behavior.isDetached())
{
{
if (!_data.isParentIdNull())
{
Operation detachedOp = ProjectionElementFinder.id().eq(_data.getParentId());
_result = ProjectionElementFinder.zFindOneForRelationship(detachedOp);
}
}
}
else if (_behavior.isInMemory())
{
_result = (ProjectionElement) _data.getParent();
if (_result == null)
{
if (!_data.isParentIdNull())
{
_op = ProjectionElementFinder.id().eq(_data.getParentId());
}
}
}
if (_op != null)
{
_result = ProjectionElementFinder.zFindOneForRelationship(_op);
}
return _result;
}
public void setParent(ProjectionElement parent)
{
ProjectionElement _parent = (ProjectionElement) parent;
TransactionalBehavior _behavior = zGetTransactionalBehaviorForWriteWithWaitIfNecessary();
ProjectionElementData _data = (ProjectionElementData) _behavior.getCurrentDataForWrite(this);
if (_behavior.isInMemory())
{
_data.setParent(_parent);
if (_parent == null)
{
this.setParentIdNull();
}
else
{
this.setParentId(_parent.getId());
}
}
else if (_behavior.isPersisted())
{
_behavior.clearTempTransaction(this);
if (_parent == null)
{
this.setParentIdNull();
}
else
{
this.setParentId(
_parent.getId());
}
}
else throw new RuntimeException("not implemented");
}
protected void cascadeInsertImpl() throws MithraBusinessException
{
TransactionalBehavior _behavior = zGetTransactionalBehaviorForWriteWithWaitIfNecessary();
this.checkAndGeneratePrimaryKeys();
ProjectionElementData _data = (ProjectionElementData) _behavior.getCurrentDataForWrite(this);
RootProjection rootProjectionSubClass =
(RootProjection) _data.getRootProjectionSubClass();
ProjectionWithAssociationEnd projectionWithAssociationEndSubClass =
(ProjectionWithAssociationEnd) _data.getProjectionWithAssociationEndSubClass();
ProjectionDataTypeProperty projectionDataTypePropertySubClass =
(ProjectionDataTypeProperty) _data.getProjectionDataTypePropertySubClass();
_behavior.insert(this);
if (rootProjectionSubClass != null)
{
rootProjectionSubClass.cascadeInsert();
}
if (projectionWithAssociationEndSubClass != null)
{
projectionWithAssociationEndSubClass.cascadeInsert();
}
if (projectionDataTypePropertySubClass != null)
{
projectionDataTypePropertySubClass.cascadeInsert();
}
}
@Override
public Map< RelatedFinder, StatisticCounter > zAddNavigatedRelationshipsStats(RelatedFinder finder, Map< RelatedFinder, StatisticCounter > navigationStats)
{
TransactionalBehavior _behavior = zGetTransactionalBehaviorForWriteWithWaitIfNecessary();
_behavior.addNavigatedRelationshipsStats(this, finder, navigationStats);
return navigationStats;
}
@Override
public Map< RelatedFinder, StatisticCounter > zAddNavigatedRelationshipsStatsForUpdate(RelatedFinder parentFinderGeneric, Map< RelatedFinder, StatisticCounter > navigationStats)
{
ProjectionElementFinder.ProjectionElementRelatedFinder parentFinder = (ProjectionElementFinder.ProjectionElementRelatedFinder) parentFinderGeneric;
TransactionalBehavior _behavior = zGetTransactionalBehaviorForWriteWithWaitIfNecessary();
ProjectionElementData _newData = (ProjectionElementData) _behavior.getCurrentDataForWrite(this);
if (_newData.getRootProjectionSubClass() instanceof NulledRelation)
{
RelatedFinder dependentFinder = parentFinder.rootProjectionSubClass();
DeepRelationshipUtility.zAddToNavigationStats(dependentFinder, true, navigationStats);
DeepRelationshipUtility.zAddAllDependentNavigationsStatsForDelete(dependentFinder, navigationStats);
}
else
{
RootProjection rootProjectionSubClass =
(RootProjection) _newData.getRootProjectionSubClass();
RelatedFinder dependentFinder = parentFinder.rootProjectionSubClass();
DeepRelationshipUtility.zAddToNavigationStats(dependentFinder, rootProjectionSubClass != null, navigationStats);
if (rootProjectionSubClass != null)
{
_behavior.addNavigatedRelationshipsStats(rootProjectionSubClass, dependentFinder, navigationStats);
}
}
if (_newData.getProjectionWithAssociationEndSubClass() instanceof NulledRelation)
{
RelatedFinder dependentFinder = parentFinder.projectionWithAssociationEndSubClass();
DeepRelationshipUtility.zAddToNavigationStats(dependentFinder, true, navigationStats);
DeepRelationshipUtility.zAddAllDependentNavigationsStatsForDelete(dependentFinder, navigationStats);
}
else
{
ProjectionWithAssociationEnd projectionWithAssociationEndSubClass =
(ProjectionWithAssociationEnd) _newData.getProjectionWithAssociationEndSubClass();
RelatedFinder dependentFinder = parentFinder.projectionWithAssociationEndSubClass();
DeepRelationshipUtility.zAddToNavigationStats(dependentFinder, projectionWithAssociationEndSubClass != null, navigationStats);
if (projectionWithAssociationEndSubClass != null)
{
_behavior.addNavigatedRelationshipsStats(projectionWithAssociationEndSubClass, dependentFinder, navigationStats);
}
}
if (_newData.getProjectionDataTypePropertySubClass() instanceof NulledRelation)
{
RelatedFinder dependentFinder = parentFinder.projectionDataTypePropertySubClass();
DeepRelationshipUtility.zAddToNavigationStats(dependentFinder, true, navigationStats);
DeepRelationshipUtility.zAddAllDependentNavigationsStatsForDelete(dependentFinder, navigationStats);
}
else
{
ProjectionDataTypeProperty projectionDataTypePropertySubClass =
(ProjectionDataTypeProperty) _newData.getProjectionDataTypePropertySubClass();
RelatedFinder dependentFinder = parentFinder.projectionDataTypePropertySubClass();
DeepRelationshipUtility.zAddToNavigationStats(dependentFinder, projectionDataTypePropertySubClass != null, navigationStats);
if (projectionDataTypePropertySubClass != null)
{
_behavior.addNavigatedRelationshipsStats(projectionDataTypePropertySubClass, dependentFinder, navigationStats);
}
}
return navigationStats;
}
@Override
public Map< RelatedFinder, StatisticCounter > zAddNavigatedRelationshipsStatsForDelete(RelatedFinder parentFinder, Map< RelatedFinder, StatisticCounter > navigationStats)
{
DeepRelationshipUtility.zAddAllDependentNavigationsStatsForDelete(parentFinder, navigationStats);
return navigationStats;
}
@Override
public ProjectionElement zCascadeCopyThenInsert() throws MithraBusinessException
{
TransactionalBehavior _behavior = zGetTransactionalBehaviorForWriteWithWaitIfNecessary();
this.checkAndGeneratePrimaryKeys();
ProjectionElementData _data = (ProjectionElementData) _behavior.getCurrentDataForWrite(this);
RootProjection rootProjectionSubClass =
(RootProjection) _data.getRootProjectionSubClass();
ProjectionWithAssociationEnd projectionWithAssociationEndSubClass =
(ProjectionWithAssociationEnd) _data.getProjectionWithAssociationEndSubClass();
ProjectionDataTypeProperty projectionDataTypePropertySubClass =
(ProjectionDataTypeProperty) _data.getProjectionDataTypePropertySubClass();
ProjectionElement original = (ProjectionElement) _behavior.copyThenInsert(this);
if (rootProjectionSubClass != null)
{
rootProjectionSubClass.zCascadeCopyThenInsert();
}
if (projectionWithAssociationEndSubClass != null)
{
projectionWithAssociationEndSubClass.zCascadeCopyThenInsert();
}
if (projectionDataTypePropertySubClass != null)
{
projectionDataTypePropertySubClass.zCascadeCopyThenInsert();
}
return original;
}
protected void cascadeDeleteImpl() throws MithraBusinessException
{
{
RootProjection related = this.getRootProjectionSubClass();
if (related != null)
related.cascadeDelete();
}
{
ProjectionWithAssociationEnd related = this.getProjectionWithAssociationEndSubClass();
if (related != null)
related.cascadeDelete();
}
{
ProjectionDataTypeProperty related = this.getProjectionDataTypePropertySubClass();
if (related != null)
related.cascadeDelete();
}
this.delete();
}
public Cache zGetCache()
{
return ProjectionElementFinder.getMithraObjectPortal().getCache();
}
public MithraObjectPortal zGetPortal()
{
return ProjectionElementFinder.getMithraObjectPortal();
}
public ProjectionElement getOriginalPersistentObject()
{
return this.zFindOriginal();
}
protected boolean issueUpdatesForNonPrimaryKeys(TransactionalBehavior behavior, MithraDataObject data, MithraDataObject newData)
{
boolean changed = false;
changed |= zUpdateString(behavior, data, newData, ProjectionElementFinder.name(), false);
changed |= zUpdateInteger(behavior, data, newData, ProjectionElementFinder.ordinal(), false);
changed |= zUpdateLong(behavior, data, newData, ProjectionElementFinder.parentId(), false);
return changed;
}
protected boolean issueUpdatesForPrimaryKeys(TransactionalBehavior behavior, MithraDataObject data, MithraDataObject newData)
{
boolean changed = false;
changed |= zUpdateLong(behavior, data, newData, ProjectionElementFinder.id(), false);
return changed;
}
public long generateAndSetId()
{
long nextValue =(long) this.generateId();
this.setId(nextValue);
return nextValue;
}
public boolean zGetIsIdSet()
{
TransactionalBehavior behavior = zGetTransactionalBehaviorForReadWithWaitIfNecessary();
ProjectionElementData data = (ProjectionElementData) behavior.getCurrentDataForRead(this);
return data.zGetIsIdSet();
}
protected long generateId()
{
Object sourceAttribute = null;
ProjectionElementFinder.getMithraObjectPortal().getCache();
SimulatedSequencePrimaryKeyGenerator primaryKeyGenerator =
MithraPrimaryKeyGenerator.getInstance().getSimulatedSequencePrimaryKeyGeneratorForNoSourceAttribute("ProjectionElement", "io.liftwizard.reladomo.simseq.ObjectSequenceObjectFactory",sourceAttribute);
return (long)primaryKeyGenerator.getNextId(sourceAttribute);
}
private void checkAndGeneratePrimaryKeys()
{
TransactionalBehavior behavior = zGetTransactionalBehaviorForWriteWithWaitIfNecessary();
ProjectionElementData data = (ProjectionElementData) behavior.getCurrentDataForWrite(this);
if (!data.zGetIsIdSet())
{
data.setId(generateId());
RootProjection rootProjectionSubClass =
(RootProjection ) data.getRootProjectionSubClass();
if (rootProjectionSubClass != null)
{
rootProjectionSubClass.setId(data.getId());
}
ProjectionWithAssociationEnd projectionWithAssociationEndSubClass =
(ProjectionWithAssociationEnd ) data.getProjectionWithAssociationEndSubClass();
if (projectionWithAssociationEndSubClass != null)
{
projectionWithAssociationEndSubClass.setId(data.getId());
}
ProjectionDataTypeProperty projectionDataTypePropertySubClass =
(ProjectionDataTypeProperty ) data.getProjectionDataTypePropertySubClass();
if (projectionDataTypePropertySubClass != null)
{
projectionDataTypePropertySubClass.setId(data.getId());
}
}
}
public Object readResolve() throws ObjectStreamException
{
ProjectionElementAbstract result = (ProjectionElementAbstract) super.readResolve();
if (result.persistenceState == PersistenceState.PERSISTED)
{
result.persistenceState = PERSISTED_STATE;
}
else if (result.persistenceState == PersistenceState.IN_MEMORY)
{
result.persistenceState = MEMORY_STATE;
}
return result;
}
protected static void zConfigNonTx()
{
MEMORY_STATE = PersistenceState.IN_MEMORY_NON_TRANSACTIONAL;
PERSISTED_STATE = PersistenceState.PERSISTED_NON_TRANSACTIONAL;
}
protected static void zConfigFullTx()
{
MEMORY_STATE = PersistenceState.IN_MEMORY;
PERSISTED_STATE = PersistenceState.PERSISTED;
}
protected class RootProjectionSubClassAddHandlerInMemory implements DependentRelationshipAddHandler
{
public void addRelatedObject(MithraTransactionalObject relatedObject)
{
RootProjection item = (RootProjection) relatedObject;
item.setId(getId());
item.zSetParentContainerprojectionElementSuperClass(ProjectionElementAbstract.this);
}
}
protected class RootProjectionSubClassAddHandlerPersisted implements DependentRelationshipAddHandler
{
public void addRelatedObject(MithraTransactionalObject relatedObject)
{
RootProjection item = (RootProjection) relatedObject;
item.setId(getId());
item.cascadeInsert();
}
}
protected class ProjectionWithAssociationEndSubClassAddHandlerInMemory implements DependentRelationshipAddHandler
{
public void addRelatedObject(MithraTransactionalObject relatedObject)
{
ProjectionWithAssociationEnd item = (ProjectionWithAssociationEnd) relatedObject;
item.setId(getId());
item.zSetParentContainerprojectionElementSuperClass(ProjectionElementAbstract.this);
}
}
protected class ProjectionWithAssociationEndSubClassAddHandlerPersisted implements DependentRelationshipAddHandler
{
public void addRelatedObject(MithraTransactionalObject relatedObject)
{
ProjectionWithAssociationEnd item = (ProjectionWithAssociationEnd) relatedObject;
item.setId(getId());
item.cascadeInsert();
}
}
protected class ProjectionDataTypePropertySubClassAddHandlerInMemory implements DependentRelationshipAddHandler
{
public void addRelatedObject(MithraTransactionalObject relatedObject)
{
ProjectionDataTypeProperty item = (ProjectionDataTypeProperty) relatedObject;
item.setId(getId());
item.zSetParentContainerprojectionElementSuperClass(ProjectionElementAbstract.this);
}
}
protected class ProjectionDataTypePropertySubClassAddHandlerPersisted implements DependentRelationshipAddHandler
{
public void addRelatedObject(MithraTransactionalObject relatedObject)
{
ProjectionDataTypeProperty item = (ProjectionDataTypeProperty) relatedObject;
item.setId(getId());
item.cascadeInsert();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy