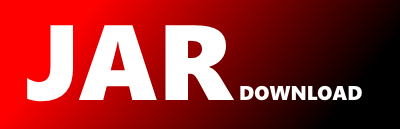
klass.model.meta.domain.ServiceAbstract Maven / Gradle / Ivy
The newest version!
package klass.model.meta.domain;
import java.math.BigDecimal;
import java.sql.Timestamp;
import java.util.*;
import java.io.*;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.gs.fw.common.mithra.*;
import com.gs.fw.common.mithra.attribute.*;
import com.gs.fw.common.mithra.util.*;
import com.gs.fw.common.mithra.notification.*;
import com.gs.fw.common.mithra.notification.listener.*;
import com.gs.fw.common.mithra.list.cursor.Cursor;
import com.gs.fw.common.mithra.bulkloader.*;
import com.gs.fw.common.mithra.*;
import com.gs.fw.common.mithra.behavior.*;
import com.gs.fw.common.mithra.cache.Cache;
import com.gs.fw.common.mithra.extractor.*;
import com.gs.fw.common.mithra.finder.*;
import com.gs.fw.common.mithra.list.*;
import com.gs.fw.common.mithra.behavior.state.PersistenceState;
import com.gs.fw.common.mithra.attribute.update.*;
import com.gs.fw.common.mithra.transaction.MithraObjectPersister;
import java.util.Arrays;
import java.util.HashSet;
/**
* This file was automatically generated using Mithra 18.0.0. Please do not modify it.
* Add custom logic to its subclass instead.
*/
// Generated from templates/transactional/Abstract.jsp
public abstract class ServiceAbstract extends com.gs.fw.common.mithra.superclassimpl.MithraTransactionalObjectImpl
implements
Element
{
private static byte MEMORY_STATE = PersistenceState.IN_MEMORY;
private static byte PERSISTED_STATE = PersistenceState.PERSISTED;
private static final Logger logger = LoggerFactory.getLogger(Service.class.getName());
private static final RelationshipHashStrategy forprojection = new ProjectionRhs();
private static final RelationshipHashStrategy forqueryCriteria = new QueryCriteriaRhs();
private static final RelationshipHashStrategy forauthorizeCriteria = new AuthorizeCriteriaRhs();
private static final RelationshipHashStrategy forvalidateCriteria = new ValidateCriteriaRhs();
private static final RelationshipHashStrategy forconflictCriteria = new ConflictCriteriaRhs();
private static final RelationshipHashStrategy forurl = new UrlRhs();
private static final class ProjectionRhs implements RelationshipHashStrategy
{
public boolean equalsForRelationship(Object _srcObject, Object _srcData, Object _targetData, Timestamp _asOfDate0, Timestamp _asOfDate1)
{
ServiceData _castedSrcData = (ServiceData) _srcData;
NamedProjectionData _castedTargetData = (NamedProjectionData) _targetData;
if (!_castedSrcData.isProjectionNameNull() && _castedSrcData.getProjectionName().equals(_castedTargetData.getName()))
{
return true;
}
return false;
}
public int computeHashCodeFromRelated(Object _srcObject, Object _srcData)
{
ServiceData _castedSrcData = (ServiceData) _srcData;
return HashUtil.hash(_castedSrcData.getProjectionName());
}
public int computeOffHeapHashCodeFromRelated(Object _srcObject, Object _srcData)
{
ServiceData _castedSrcData = (ServiceData) _srcData;
return HashUtil.offHeapHash(_castedSrcData.getProjectionName());
}
}
private static final class QueryCriteriaRhs implements RelationshipHashStrategy
{
public boolean equalsForRelationship(Object _srcObject, Object _srcData, Object _targetData, Timestamp _asOfDate0, Timestamp _asOfDate1)
{
ServiceData _castedSrcData = (ServiceData) _srcData;
CriteriaData _castedTargetData = (CriteriaData) _targetData;
if (!_castedSrcData.isQueryCriteriaIdNull() && _castedSrcData.getQueryCriteriaId() == _castedTargetData.getId())
{
return true;
}
return false;
}
public int computeHashCodeFromRelated(Object _srcObject, Object _srcData)
{
ServiceData _castedSrcData = (ServiceData) _srcData;
return HashUtil.hash(_castedSrcData.getQueryCriteriaId(), _castedSrcData.isQueryCriteriaIdNull());
}
public int computeOffHeapHashCodeFromRelated(Object _srcObject, Object _srcData)
{
return computeHashCodeFromRelated(_srcObject, _srcData);
}
}
private static final class AuthorizeCriteriaRhs implements RelationshipHashStrategy
{
public boolean equalsForRelationship(Object _srcObject, Object _srcData, Object _targetData, Timestamp _asOfDate0, Timestamp _asOfDate1)
{
ServiceData _castedSrcData = (ServiceData) _srcData;
CriteriaData _castedTargetData = (CriteriaData) _targetData;
if (!_castedSrcData.isAuthorizeCriteriaIdNull() && _castedSrcData.getAuthorizeCriteriaId() == _castedTargetData.getId())
{
return true;
}
return false;
}
public int computeHashCodeFromRelated(Object _srcObject, Object _srcData)
{
ServiceData _castedSrcData = (ServiceData) _srcData;
return HashUtil.hash(_castedSrcData.getAuthorizeCriteriaId(), _castedSrcData.isAuthorizeCriteriaIdNull());
}
public int computeOffHeapHashCodeFromRelated(Object _srcObject, Object _srcData)
{
return computeHashCodeFromRelated(_srcObject, _srcData);
}
}
private static final class ValidateCriteriaRhs implements RelationshipHashStrategy
{
public boolean equalsForRelationship(Object _srcObject, Object _srcData, Object _targetData, Timestamp _asOfDate0, Timestamp _asOfDate1)
{
ServiceData _castedSrcData = (ServiceData) _srcData;
CriteriaData _castedTargetData = (CriteriaData) _targetData;
if (!_castedSrcData.isValidateCriteriaIdNull() && _castedSrcData.getValidateCriteriaId() == _castedTargetData.getId())
{
return true;
}
return false;
}
public int computeHashCodeFromRelated(Object _srcObject, Object _srcData)
{
ServiceData _castedSrcData = (ServiceData) _srcData;
return HashUtil.hash(_castedSrcData.getValidateCriteriaId(), _castedSrcData.isValidateCriteriaIdNull());
}
public int computeOffHeapHashCodeFromRelated(Object _srcObject, Object _srcData)
{
return computeHashCodeFromRelated(_srcObject, _srcData);
}
}
private static final class ConflictCriteriaRhs implements RelationshipHashStrategy
{
public boolean equalsForRelationship(Object _srcObject, Object _srcData, Object _targetData, Timestamp _asOfDate0, Timestamp _asOfDate1)
{
ServiceData _castedSrcData = (ServiceData) _srcData;
CriteriaData _castedTargetData = (CriteriaData) _targetData;
if (!_castedSrcData.isConflictCriteriaIdNull() && _castedSrcData.getConflictCriteriaId() == _castedTargetData.getId())
{
return true;
}
return false;
}
public int computeHashCodeFromRelated(Object _srcObject, Object _srcData)
{
ServiceData _castedSrcData = (ServiceData) _srcData;
return HashUtil.hash(_castedSrcData.getConflictCriteriaId(), _castedSrcData.isConflictCriteriaIdNull());
}
public int computeOffHeapHashCodeFromRelated(Object _srcObject, Object _srcData)
{
return computeHashCodeFromRelated(_srcObject, _srcData);
}
}
private static final class UrlRhs implements RelationshipHashStrategy
{
public boolean equalsForRelationship(Object _srcObject, Object _srcData, Object _targetData, Timestamp _asOfDate0, Timestamp _asOfDate1)
{
ServiceData _castedSrcData = (ServiceData) _srcData;
UrlData _castedTargetData = (UrlData) _targetData;
if (_castedSrcData.getServiceGroupName()!= null && _castedSrcData.getServiceGroupName().equals(_castedTargetData.getServiceGroupName()) && _castedSrcData.getUrlString()!= null && _castedSrcData.getUrlString().equals(_castedTargetData.getUrl()))
{
return true;
}
return false;
}
public int computeHashCodeFromRelated(Object _srcObject, Object _srcData)
{
ServiceData _castedSrcData = (ServiceData) _srcData;
return HashUtil.combineHashes(HashUtil.hash(_castedSrcData.getServiceGroupName()),HashUtil.hash(_castedSrcData.getUrlString()));
}
public int computeOffHeapHashCodeFromRelated(Object _srcObject, Object _srcData)
{
ServiceData _castedSrcData = (ServiceData) _srcData;
return HashUtil.combineHashes(HashUtil.offHeapHash(_castedSrcData.getServiceGroupName()),HashUtil.offHeapHash(_castedSrcData.getUrlString()));
}
}
public ServiceAbstract()
{
this.persistenceState = MEMORY_STATE;
}
public Service getDetachedCopy() throws MithraBusinessException
{
return (Service) super.getDetachedCopy();
}
public Service getNonPersistentCopy() throws MithraBusinessException
{
Service result = (Service) super.getNonPersistentCopy();
result.persistenceState = MEMORY_STATE;
return result;
}
public Service copyDetachedValuesToOriginalOrInsertIfNew()
{
return (Service) this.zCopyDetachedValuesToOriginalOrInsertIfNew();
}
public Service zFindOriginal()
{
ServiceData data = (ServiceData) this.currentData;
Operation op;
op = ServiceFinder.serviceGroupName().eq(data.getServiceGroupName());
op = op.and(ServiceFinder.urlString().eq(data.getUrlString()));
op = op.and(ServiceFinder.verb().eq(data.getVerb()));
return ServiceFinder.findOne(op);
}
public boolean isModifiedSinceDetachmentByDependentRelationships()
{
if(this.isModifiedSinceDetachment()) return true;
if(isOrderBysModifiedSinceDetachment()) return true;
return false;
}
private Logger getLogger()
{
return logger;
}
public MithraDataObject zAllocateData()
{
return new ServiceData();
}
protected void zSetFromServiceData( ServiceData data )
{
super.zSetData(data);
this.persistenceState = PERSISTED_STATE;
}
public void setFromServiceData( ServiceData data )
{
super.zSetData(data);
}
public void zWriteDataClassName(ObjectOutput out) throws IOException
{
}
public boolean isAuthorizeCriteriaIdNull()
{
return ((ServiceData) this.zSynchronizedGetData()).isAuthorizeCriteriaIdNull();
}
public long getAuthorizeCriteriaId()
{
ServiceData data = (ServiceData) this.zSynchronizedGetData();
if (data.isAuthorizeCriteriaIdNull()) MithraNullPrimitiveException.throwNew("authorizeCriteriaId", data);
return data.getAuthorizeCriteriaId();
}
public void setAuthorizeCriteriaId(long newValue)
{
zSetLong(ServiceFinder.authorizeCriteriaId(), newValue, false, false ,true);
}
public boolean isConflictCriteriaIdNull()
{
return ((ServiceData) this.zSynchronizedGetData()).isConflictCriteriaIdNull();
}
public long getConflictCriteriaId()
{
ServiceData data = (ServiceData) this.zSynchronizedGetData();
if (data.isConflictCriteriaIdNull()) MithraNullPrimitiveException.throwNew("conflictCriteriaId", data);
return data.getConflictCriteriaId();
}
public void setConflictCriteriaId(long newValue)
{
zSetLong(ServiceFinder.conflictCriteriaId(), newValue, false, false ,true);
}
public boolean isOrdinalNull()
{
return ((ServiceData) this.zSynchronizedGetData()).isOrdinalNull();
}
public int getOrdinal()
{
ServiceData data = (ServiceData) this.zSynchronizedGetData();
return data.getOrdinal();
}
public void setOrdinal(int newValue)
{
zSetInteger(ServiceFinder.ordinal(), newValue, false, false ,false);
}
public boolean isProjectionNameNull()
{
return ((ServiceData) this.zSynchronizedGetData()).isProjectionNameNull();
}
public String getProjectionName()
{
ServiceData data = (ServiceData) this.zSynchronizedGetData();
return data.getProjectionName();
}
public void setProjectionName(String newValue)
{
if (newValue != null && newValue.length() > 256)
throw new MithraBusinessException("Attribute 'projectionName' cannot exceed maximum length of 256: " + newValue);
zSetString(ServiceFinder.projectionName(), newValue, false, false );
}
public boolean isQueryCriteriaIdNull()
{
return ((ServiceData) this.zSynchronizedGetData()).isQueryCriteriaIdNull();
}
public long getQueryCriteriaId()
{
ServiceData data = (ServiceData) this.zSynchronizedGetData();
if (data.isQueryCriteriaIdNull()) MithraNullPrimitiveException.throwNew("queryCriteriaId", data);
return data.getQueryCriteriaId();
}
public void setQueryCriteriaId(long newValue)
{
zSetLong(ServiceFinder.queryCriteriaId(), newValue, false, false ,true);
}
public final boolean isServiceGroupNameNull()
{
return ((ServiceData) this.zSynchronizedGetData()).isServiceGroupNameNull();
}
public final String getServiceGroupName()
{
ServiceData data = (ServiceData) this.zSynchronizedGetData();
return data.getServiceGroupName();
}
public void setServiceGroupName(String newValue)
{
if (newValue != null && newValue.length() > 256)
throw new MithraBusinessException("Attribute 'serviceGroupName' cannot exceed maximum length of 256: " + newValue);
MithraDataObject d = zSetString(ServiceFinder.serviceGroupName(), newValue, true, false );
if (d == null) return;
ServiceData data = (ServiceData) d;
TransactionalBehavior _behavior = zGetTransactionalBehaviorForWriteWithWaitIfNecessary();
if (!_behavior.isPersisted())
{
ServiceOrderByList orderBys =
(ServiceOrderByList ) data.getOrderBys();
if (orderBys != null)
{
orderBys.setServiceGroupName(newValue);
}
}
}
public boolean isServiceMultiplicityNull()
{
return ((ServiceData) this.zSynchronizedGetData()).isServiceMultiplicityNull();
}
public String getServiceMultiplicity()
{
ServiceData data = (ServiceData) this.zSynchronizedGetData();
return data.getServiceMultiplicity();
}
public void setServiceMultiplicity(String newValue)
{
if (newValue != null && newValue.length() > 256)
throw new MithraBusinessException("Attribute 'serviceMultiplicity' cannot exceed maximum length of 256: " + newValue);
zSetString(ServiceFinder.serviceMultiplicity(), newValue, false, false );
}
public final boolean isUrlStringNull()
{
return ((ServiceData) this.zSynchronizedGetData()).isUrlStringNull();
}
public final String getUrlString()
{
ServiceData data = (ServiceData) this.zSynchronizedGetData();
return data.getUrlString();
}
public void setUrlString(String newValue)
{
if (newValue != null && newValue.length() > 8192)
throw new MithraBusinessException("Attribute 'urlString' cannot exceed maximum length of 8192: " + newValue);
MithraDataObject d = zSetString(ServiceFinder.urlString(), newValue, true, false );
if (d == null) return;
ServiceData data = (ServiceData) d;
TransactionalBehavior _behavior = zGetTransactionalBehaviorForWriteWithWaitIfNecessary();
if (!_behavior.isPersisted())
{
ServiceOrderByList orderBys =
(ServiceOrderByList ) data.getOrderBys();
if (orderBys != null)
{
orderBys.setServiceUrlString(newValue);
}
}
}
public boolean isValidateCriteriaIdNull()
{
return ((ServiceData) this.zSynchronizedGetData()).isValidateCriteriaIdNull();
}
public long getValidateCriteriaId()
{
ServiceData data = (ServiceData) this.zSynchronizedGetData();
if (data.isValidateCriteriaIdNull()) MithraNullPrimitiveException.throwNew("validateCriteriaId", data);
return data.getValidateCriteriaId();
}
public void setValidateCriteriaId(long newValue)
{
zSetLong(ServiceFinder.validateCriteriaId(), newValue, false, false ,true);
}
public final boolean isVerbNull()
{
return ((ServiceData) this.zSynchronizedGetData()).isVerbNull();
}
public final String getVerb()
{
ServiceData data = (ServiceData) this.zSynchronizedGetData();
return data.getVerb();
}
public void setVerb(String newValue)
{
if (newValue != null && newValue.length() > 256)
throw new MithraBusinessException("Attribute 'verb' cannot exceed maximum length of 256: " + newValue);
MithraDataObject d = zSetString(ServiceFinder.verb(), newValue, true, false );
if (d == null) return;
ServiceData data = (ServiceData) d;
TransactionalBehavior _behavior = zGetTransactionalBehaviorForWriteWithWaitIfNecessary();
if (!_behavior.isPersisted())
{
ServiceOrderByList orderBys =
(ServiceOrderByList ) data.getOrderBys();
if (orderBys != null)
{
orderBys.setServiceVerb(newValue);
}
}
}
protected void issuePrimitiveNullSetters(TransactionalBehavior behavior, MithraDataObject data)
{
zNullify(behavior, data, ServiceFinder.queryCriteriaId(), false);
zNullify(behavior, data, ServiceFinder.authorizeCriteriaId(), false);
zNullify(behavior, data, ServiceFinder.validateCriteriaId(), false);
zNullify(behavior, data, ServiceFinder.conflictCriteriaId(), false);
}
public void setQueryCriteriaIdNull()
{
zNullify(ServiceFinder.queryCriteriaId(), false);
}
public void setAuthorizeCriteriaIdNull()
{
zNullify(ServiceFinder.authorizeCriteriaId(), false);
}
public void setValidateCriteriaIdNull()
{
zNullify(ServiceFinder.validateCriteriaId(), false);
}
public void setConflictCriteriaIdNull()
{
zNullify(ServiceFinder.conflictCriteriaId(), false);
}
public void zPersistDetachedRelationships(MithraDataObject _data)
{
ServiceData _newData = (ServiceData) _data;
{
ServiceOrderByList orderBys =
(ServiceOrderByList) _newData.getOrderBys();
if (orderBys != null)
{
orderBys.copyDetachedValuesToOriginalOrInsertIfNewOrDeleteIfRemoved();
}
}
}
public void zSetTxDetachedDeleted()
{
TransactionalBehavior _behavior = zGetTransactionalBehaviorForWriteWithWaitIfNecessary();
if (_behavior.isDetached() && _behavior.isDeleted()) return;
ServiceData _newData = (ServiceData) _behavior.getCurrentDataForRead(this);
if (_newData.getOrderBys() != null && !(_newData.getOrderBys() instanceof NulledRelation))
{
((ServiceOrderByList)_newData.getOrderBys()).zSetTxDetachedDeleted();
}
this.zSetTxPersistenceState(PersistenceState.DETACHED_DELETED);
}
public void zSetNonTxDetachedDeleted()
{
TransactionalBehavior _behavior = zGetTransactionalBehaviorForWriteWithWaitIfNecessary();
ServiceData _newData = (ServiceData) _behavior.getCurrentDataForRead(this);
if (_newData.getOrderBys() != null && !(_newData.getOrderBys() instanceof NulledRelation))
{
((ServiceOrderByList)_newData.getOrderBys()).zSetNonTxDetachedDeleted();
}
this.zSetNonTxPersistenceState(PersistenceState.DETACHED_DELETED);
}
/**
* Relationship Expression:
this.projectionName = NamedProjection.name
* @see NamedProjection#getServices() reverse relationship NamedProjection.getServices()
* @return The projection
*/
public NamedProjection getProjection()
{
NamedProjection _result = null;
Operation _op = null;
TransactionalBehavior _behavior = zGetTransactionalBehaviorForReadWithWaitIfNecessary();
ServiceData _data = (ServiceData) _behavior.getCurrentDataForRead(this);
MithraObjectPortal _portal = null;
if (_behavior.isPersisted())
{
if (!_data.isProjectionNameNull())
{
_portal = NamedProjectionFinder.getMithraObjectPortal();
Object _related = _portal.getAsOneFromCache(this, _data, forprojection, null, null);
if (!(_related instanceof NulledRelation)) _result = (NamedProjection) _related;
if (_related == null)
{
_op = NamedProjectionFinder.name().eq(_data.getProjectionName());
}
}
else
{
return null;
}
}
else if (_behavior.isDetached())
{
{
if (!_data.isProjectionNameNull())
{
Operation detachedOp = NamedProjectionFinder.name().eq(_data.getProjectionName());
_result = NamedProjectionFinder.zFindOneForRelationship(detachedOp);
}
}
}
else if (_behavior.isInMemory())
{
_result = (NamedProjection) _data.getProjection();
if (_result == null)
{
if (!_data.isProjectionNameNull())
{
_op = NamedProjectionFinder.name().eq(_data.getProjectionName());
}
}
}
if (_op != null)
{
_result = NamedProjectionFinder.zFindOneForRelationship(_op);
}
return _result;
}
public void setProjection(NamedProjection projection)
{
NamedProjection _projection = (NamedProjection) projection;
TransactionalBehavior _behavior = zGetTransactionalBehaviorForWriteWithWaitIfNecessary();
ServiceData _data = (ServiceData) _behavior.getCurrentDataForWrite(this);
if (_behavior.isInMemory())
{
_data.setProjection(_projection);
if (_projection == null)
{
this.setProjectionName(null);
}
else
{
this.setProjectionName(_projection.getName());
}
}
else if (_behavior.isPersisted())
{
_behavior.clearTempTransaction(this);
if (_projection == null)
{
this.setProjectionName(null);
}
else
{
this.setProjectionName(
_projection.getName());
}
}
else throw new RuntimeException("not implemented");
}
/**
* Relationship Expression:
this.queryCriteriaId = Criteria.id
* @see Criteria#getQueryService() reverse relationship Criteria.getQueryService()
* @return The query criteria
*/
public Criteria getQueryCriteria()
{
Criteria _result = null;
Operation _op = null;
TransactionalBehavior _behavior = zGetTransactionalBehaviorForReadWithWaitIfNecessary();
ServiceData _data = (ServiceData) _behavior.getCurrentDataForRead(this);
MithraObjectPortal _portal = null;
if (_behavior.isPersisted())
{
if (!_data.isQueryCriteriaIdNull())
{
_portal = CriteriaFinder.getMithraObjectPortal();
Object _related = _portal.getAsOneFromCache(this, _data, forqueryCriteria, null, null);
if (!(_related instanceof NulledRelation)) _result = (Criteria) _related;
if (_related == null)
{
_op = CriteriaFinder.id().eq(_data.getQueryCriteriaId());
}
}
else
{
return null;
}
}
else if (_behavior.isDetached())
{
{
if (!_data.isQueryCriteriaIdNull())
{
Operation detachedOp = CriteriaFinder.id().eq(_data.getQueryCriteriaId());
_result = CriteriaFinder.zFindOneForRelationship(detachedOp);
}
}
}
else if (_behavior.isInMemory())
{
_result = (Criteria) _data.getQueryCriteria();
if (_result == null)
{
if (!_data.isQueryCriteriaIdNull())
{
_op = CriteriaFinder.id().eq(_data.getQueryCriteriaId());
}
}
}
if (_op != null)
{
_result = CriteriaFinder.zFindOneForRelationship(_op);
}
return _result;
}
public void setQueryCriteria(Criteria queryCriteria)
{
Criteria _queryCriteria = (Criteria) queryCriteria;
TransactionalBehavior _behavior = zGetTransactionalBehaviorForWriteWithWaitIfNecessary();
ServiceData _data = (ServiceData) _behavior.getCurrentDataForWrite(this);
if (_behavior.isInMemory())
{
_data.setQueryCriteria(_queryCriteria);
if (_queryCriteria == null)
{
this.setQueryCriteriaIdNull();
}
else
{
this.setQueryCriteriaId(_queryCriteria.getId());
}
}
else if (_behavior.isPersisted())
{
_behavior.clearTempTransaction(this);
if (_queryCriteria == null)
{
this.setQueryCriteriaIdNull();
}
else
{
this.setQueryCriteriaId(
_queryCriteria.getId());
}
}
else throw new RuntimeException("not implemented");
}
/**
* Relationship Expression:
this.authorizeCriteriaId = Criteria.id
* @see Criteria#getAuthorizeService() reverse relationship Criteria.getAuthorizeService()
* @return The authorize criteria
*/
public Criteria getAuthorizeCriteria()
{
Criteria _result = null;
Operation _op = null;
TransactionalBehavior _behavior = zGetTransactionalBehaviorForReadWithWaitIfNecessary();
ServiceData _data = (ServiceData) _behavior.getCurrentDataForRead(this);
MithraObjectPortal _portal = null;
if (_behavior.isPersisted())
{
if (!_data.isAuthorizeCriteriaIdNull())
{
_portal = CriteriaFinder.getMithraObjectPortal();
Object _related = _portal.getAsOneFromCache(this, _data, forauthorizeCriteria, null, null);
if (!(_related instanceof NulledRelation)) _result = (Criteria) _related;
if (_related == null)
{
_op = CriteriaFinder.id().eq(_data.getAuthorizeCriteriaId());
}
}
else
{
return null;
}
}
else if (_behavior.isDetached())
{
{
if (!_data.isAuthorizeCriteriaIdNull())
{
Operation detachedOp = CriteriaFinder.id().eq(_data.getAuthorizeCriteriaId());
_result = CriteriaFinder.zFindOneForRelationship(detachedOp);
}
}
}
else if (_behavior.isInMemory())
{
_result = (Criteria) _data.getAuthorizeCriteria();
if (_result == null)
{
if (!_data.isAuthorizeCriteriaIdNull())
{
_op = CriteriaFinder.id().eq(_data.getAuthorizeCriteriaId());
}
}
}
if (_op != null)
{
_result = CriteriaFinder.zFindOneForRelationship(_op);
}
return _result;
}
public void setAuthorizeCriteria(Criteria authorizeCriteria)
{
Criteria _authorizeCriteria = (Criteria) authorizeCriteria;
TransactionalBehavior _behavior = zGetTransactionalBehaviorForWriteWithWaitIfNecessary();
ServiceData _data = (ServiceData) _behavior.getCurrentDataForWrite(this);
if (_behavior.isInMemory())
{
_data.setAuthorizeCriteria(_authorizeCriteria);
if (_authorizeCriteria == null)
{
this.setAuthorizeCriteriaIdNull();
}
else
{
this.setAuthorizeCriteriaId(_authorizeCriteria.getId());
}
}
else if (_behavior.isPersisted())
{
_behavior.clearTempTransaction(this);
if (_authorizeCriteria == null)
{
this.setAuthorizeCriteriaIdNull();
}
else
{
this.setAuthorizeCriteriaId(
_authorizeCriteria.getId());
}
}
else throw new RuntimeException("not implemented");
}
/**
* Relationship Expression:
this.validateCriteriaId = Criteria.id
* @see Criteria#getValidateService() reverse relationship Criteria.getValidateService()
* @return The validate criteria
*/
public Criteria getValidateCriteria()
{
Criteria _result = null;
Operation _op = null;
TransactionalBehavior _behavior = zGetTransactionalBehaviorForReadWithWaitIfNecessary();
ServiceData _data = (ServiceData) _behavior.getCurrentDataForRead(this);
MithraObjectPortal _portal = null;
if (_behavior.isPersisted())
{
if (!_data.isValidateCriteriaIdNull())
{
_portal = CriteriaFinder.getMithraObjectPortal();
Object _related = _portal.getAsOneFromCache(this, _data, forvalidateCriteria, null, null);
if (!(_related instanceof NulledRelation)) _result = (Criteria) _related;
if (_related == null)
{
_op = CriteriaFinder.id().eq(_data.getValidateCriteriaId());
}
}
else
{
return null;
}
}
else if (_behavior.isDetached())
{
{
if (!_data.isValidateCriteriaIdNull())
{
Operation detachedOp = CriteriaFinder.id().eq(_data.getValidateCriteriaId());
_result = CriteriaFinder.zFindOneForRelationship(detachedOp);
}
}
}
else if (_behavior.isInMemory())
{
_result = (Criteria) _data.getValidateCriteria();
if (_result == null)
{
if (!_data.isValidateCriteriaIdNull())
{
_op = CriteriaFinder.id().eq(_data.getValidateCriteriaId());
}
}
}
if (_op != null)
{
_result = CriteriaFinder.zFindOneForRelationship(_op);
}
return _result;
}
public void setValidateCriteria(Criteria validateCriteria)
{
Criteria _validateCriteria = (Criteria) validateCriteria;
TransactionalBehavior _behavior = zGetTransactionalBehaviorForWriteWithWaitIfNecessary();
ServiceData _data = (ServiceData) _behavior.getCurrentDataForWrite(this);
if (_behavior.isInMemory())
{
_data.setValidateCriteria(_validateCriteria);
if (_validateCriteria == null)
{
this.setValidateCriteriaIdNull();
}
else
{
this.setValidateCriteriaId(_validateCriteria.getId());
}
}
else if (_behavior.isPersisted())
{
_behavior.clearTempTransaction(this);
if (_validateCriteria == null)
{
this.setValidateCriteriaIdNull();
}
else
{
this.setValidateCriteriaId(
_validateCriteria.getId());
}
}
else throw new RuntimeException("not implemented");
}
/**
* Relationship Expression:
this.conflictCriteriaId = Criteria.id
* @see Criteria#getConflictService() reverse relationship Criteria.getConflictService()
* @return The conflict criteria
*/
public Criteria getConflictCriteria()
{
Criteria _result = null;
Operation _op = null;
TransactionalBehavior _behavior = zGetTransactionalBehaviorForReadWithWaitIfNecessary();
ServiceData _data = (ServiceData) _behavior.getCurrentDataForRead(this);
MithraObjectPortal _portal = null;
if (_behavior.isPersisted())
{
if (!_data.isConflictCriteriaIdNull())
{
_portal = CriteriaFinder.getMithraObjectPortal();
Object _related = _portal.getAsOneFromCache(this, _data, forconflictCriteria, null, null);
if (!(_related instanceof NulledRelation)) _result = (Criteria) _related;
if (_related == null)
{
_op = CriteriaFinder.id().eq(_data.getConflictCriteriaId());
}
}
else
{
return null;
}
}
else if (_behavior.isDetached())
{
{
if (!_data.isConflictCriteriaIdNull())
{
Operation detachedOp = CriteriaFinder.id().eq(_data.getConflictCriteriaId());
_result = CriteriaFinder.zFindOneForRelationship(detachedOp);
}
}
}
else if (_behavior.isInMemory())
{
_result = (Criteria) _data.getConflictCriteria();
if (_result == null)
{
if (!_data.isConflictCriteriaIdNull())
{
_op = CriteriaFinder.id().eq(_data.getConflictCriteriaId());
}
}
}
if (_op != null)
{
_result = CriteriaFinder.zFindOneForRelationship(_op);
}
return _result;
}
public void setConflictCriteria(Criteria conflictCriteria)
{
Criteria _conflictCriteria = (Criteria) conflictCriteria;
TransactionalBehavior _behavior = zGetTransactionalBehaviorForWriteWithWaitIfNecessary();
ServiceData _data = (ServiceData) _behavior.getCurrentDataForWrite(this);
if (_behavior.isInMemory())
{
_data.setConflictCriteria(_conflictCriteria);
if (_conflictCriteria == null)
{
this.setConflictCriteriaIdNull();
}
else
{
this.setConflictCriteriaId(_conflictCriteria.getId());
}
}
else if (_behavior.isPersisted())
{
_behavior.clearTempTransaction(this);
if (_conflictCriteria == null)
{
this.setConflictCriteriaIdNull();
}
else
{
this.setConflictCriteriaId(
_conflictCriteria.getId());
}
}
else throw new RuntimeException("not implemented");
}
/**
* Relationship Expression:
this.serviceGroupName = ServiceOrderBy.serviceGroupName and this.urlString = ServiceOrderBy.serviceUrlString and this.verb = ServiceOrderBy.serviceVerb
* Order By: ordinal asc
.
* @see ServiceOrderBy#getService() reverse relationship ServiceOrderBy.getService()
* @return order bys
*/
public ServiceOrderByList getOrderBys()
{
ServiceOrderByList _result = null;
Operation _op = null;
TransactionalBehavior _behavior = zGetTransactionalBehaviorForReadWithWaitIfNecessary();
ServiceData _data = (ServiceData) _behavior.getCurrentDataForRead(this);
if (_behavior.isPersisted())
{
{
{
_op = new RelationshipMultiEqualityOperation(ServiceFinder.orderBys().zGetRelationshipMultiExtractor(), this);
}
}
}
else if (_behavior.isDetached())
{
_result = (ServiceOrderByList) _data.getOrderBys();
if (_result == null)
{
{
Operation detachedOp = new RelationshipMultiEqualityOperation(ServiceFinder.orderBys().zGetRelationshipMultiExtractor(), this);
_result = new ServiceOrderByList(detachedOp);
_result.zSetForRelationship();
if(_result != null)
{
_result = _result.getDetachedCopy();
}
_result.zSetAddHandler(new OrderBysAddHandlerInMemory());
}
_result.setOrderBy(ServiceOrderByFinder.ordinal().ascendingOrderBy());
_behavior = zGetTransactionalBehaviorForWriteWithWaitIfNecessary();
_data = (ServiceData) _behavior.getCurrentDataForWrite(this);
_data.setOrderBys(_result);
if (_result != null) _result.zSetParentContainerservice(this);
}
}
else if (_behavior.isInMemory())
{
_result = (ServiceOrderByList) _data.getOrderBys();
if (_result == null)
{
_result = new ServiceOrderByList();
_result.zSetAddHandler(new OrderBysAddHandlerInMemory());
_behavior = zGetTransactionalBehaviorForWriteWithWaitIfNecessary();
_data = (ServiceData) _behavior.getCurrentDataForWrite(this);
_data.setOrderBys(_result);
}
}
if (_op != null)
{
_result = new ServiceOrderByList(_op);
_result.zSetForRelationship();
_result.zSetRemoveHandler(DeleteOnRemoveHandler.getInstance());
_result.zSetAddHandler(new OrderBysAddHandlerPersisted());
_result.setOrderBy(ServiceOrderByFinder.ordinal().ascendingOrderBy());
}
return _result;
}
public void setOrderBys(ServiceOrderByList orderBys)
{
ServiceOrderByList _orderBys = (ServiceOrderByList) orderBys;
TransactionalBehavior _behavior = zGetTransactionalBehaviorForWriteWithWaitIfNecessary();
ServiceData _data = (ServiceData) _behavior.getCurrentDataForWrite(this);
if (_behavior.isInMemory())
{
if (_behavior.isDetached() && _orderBys != null)
{
_orderBys.zMakeDetached(new RelationshipMultiEqualityOperation(ServiceFinder.orderBys().zGetRelationshipMultiExtractor(), this),
_data.getOrderBys());
}
_data.setOrderBys(_orderBys);
if (_orderBys != null)
{
_orderBys.setServiceGroupName(_data.getServiceGroupName());
_orderBys.setServiceUrlString(_data.getUrlString());
_orderBys.setServiceVerb(_data.getVerb());
_orderBys.zSetParentContainerservice(this);
_orderBys.zSetAddHandler(new OrderBysAddHandlerInMemory());
}
else if (_behavior.isDetached())
{
throw new MithraBusinessException("to-many relationships cannot be set to null. Use the clear() method on the list instead.");
}
}
else if (_behavior.isPersisted())
{
_behavior.clearTempTransaction(this);
_orderBys.zSetAddHandler(new OrderBysAddHandlerPersisted());
ServiceOrderByList orderBysToDelete = new ServiceOrderByList();
orderBysToDelete.addAll(this.getOrderBys());
for(int i=0;i < _orderBys.size(); i++)
{
ServiceOrderBy item = _orderBys.getServiceOrderByAt(i);
if (!orderBysToDelete.remove(item))
{
item.setServiceGroupName(_data.getServiceGroupName());
item.setServiceUrlString(_data.getUrlString());
item.setServiceVerb(_data.getVerb());
item.cascadeInsert();
}
}
orderBysToDelete.cascadeDeleteAll();
}
else throw new RuntimeException("not implemented");
}
public boolean isOrderBysModifiedSinceDetachment()
{
TransactionalBehavior _behavior = zGetTransactionalBehaviorForReadWithWaitIfNecessary();
ServiceData _data = (ServiceData) _behavior.getCurrentDataForRead(this);
ServiceOrderByList orderBys =
(ServiceOrderByList) _data.getOrderBys();
if( orderBys != null)
{
return orderBys.isModifiedSinceDetachment();
}
return false;
}
/**
* Relationship Expression:
(Url.serviceGroupName = this.serviceGroupName and Url.url = this.urlString )
* @see Url#getServices() reverse relationship Url.getServices()
* @return The url
*/
public Url getUrl()
{
Url _result = null;
Operation _op = null;
TransactionalBehavior _behavior = zGetTransactionalBehaviorForReadWithWaitIfNecessary();
ServiceData _data = (ServiceData) _behavior.getCurrentDataForRead(this);
MithraObjectPortal _portal = null;
if (_behavior.isPersisted())
{
{
_portal = UrlFinder.getMithraObjectPortal();
Object _related = _portal.getAsOneFromCache(this, _data, forurl, null, null);
if (!(_related instanceof NulledRelation)) _result = (Url) _related;
if (_related == null)
{
_op = new RelationshipMultiEqualityOperation(ServiceFinder.url().zGetRelationshipMultiExtractor(), this);
}
}
}
else if (_behavior.isDetached())
{
if (_data.getUrl() instanceof NulledRelation)
{
return null;
}
_result = (Url) _data.getUrl();
if (_result == null)
{
{
Operation detachedOp = new RelationshipMultiEqualityOperation(ServiceFinder.url().zGetRelationshipMultiExtractor(), this);
_result = UrlFinder.zFindOneForRelationship(detachedOp);
if(_result != null)
{
_result = _result.getDetachedCopy();
}
}
_data = (ServiceData) _behavior.getCurrentDataForWrite(this);
_data.setUrl(_result);
}
}
else if (_behavior.isInMemory())
{
_result = (Url) _data.getUrl();
if (_result == null)
{
{
_op = new RelationshipMultiEqualityOperation(ServiceFinder.url().zGetRelationshipMultiExtractor(), this);
}
}
}
if (_op != null)
{
_result = UrlFinder.zFindOneForRelationship(_op);
}
return _result;
}
public void setUrl(Url url)
{
Url _url = (Url) url;
TransactionalBehavior _behavior = zGetTransactionalBehaviorForWriteWithWaitIfNecessary();
ServiceData _data = (ServiceData) _behavior.getCurrentDataForWrite(this);
if (_behavior.isInMemory())
{
Object _prev = _data.getUrl();
if (_behavior.isDetached() && _prev != null)
{
((DelegatingList)((Url)_prev).getServices()).zMarkMoved( (Service) this);
}
_data.setUrl(_url);
_url.getServices().add( (Service) this);
}
else if (_behavior.isPersisted())
{
_behavior.clearTempTransaction(this);
if (_url == null)
{
this.setServiceGroupName(null);
this.setUrlString(null);
}
else
{
this.setServiceGroupName(
_url.getServiceGroupName());
this.setUrlString(
_url.getUrl());
}
}
else throw new RuntimeException("not implemented");
}
public void zSetParentContainerurl(UrlAbstract parent)
{
TransactionalBehavior _behavior = zGetTransactionalBehaviorForWriteWithWaitIfNecessary();
ServiceData _data = (ServiceData) _behavior.getCurrentDataForWrite(this);
_behavior.clearTempTransaction(this);
if (_behavior.isInMemory())
{
_data.setUrl(parent);
}
}
protected void cascadeInsertImpl() throws MithraBusinessException
{
TransactionalBehavior _behavior = zGetTransactionalBehaviorForWriteWithWaitIfNecessary();
ServiceData _data = (ServiceData) _behavior.getCurrentDataForWrite(this);
ServiceOrderByList orderBys =
(ServiceOrderByList) _data.getOrderBys();
_behavior.insert(this);
if (orderBys != null)
{
orderBys.cascadeInsertAll();
}
}
@Override
public Map< RelatedFinder, StatisticCounter > zAddNavigatedRelationshipsStats(RelatedFinder finder, Map< RelatedFinder, StatisticCounter > navigationStats)
{
TransactionalBehavior _behavior = zGetTransactionalBehaviorForWriteWithWaitIfNecessary();
_behavior.addNavigatedRelationshipsStats(this, finder, navigationStats);
return navigationStats;
}
@Override
public Map< RelatedFinder, StatisticCounter > zAddNavigatedRelationshipsStatsForUpdate(RelatedFinder parentFinderGeneric, Map< RelatedFinder, StatisticCounter > navigationStats)
{
ServiceFinder.ServiceRelatedFinder parentFinder = (ServiceFinder.ServiceRelatedFinder) parentFinderGeneric;
TransactionalBehavior _behavior = zGetTransactionalBehaviorForWriteWithWaitIfNecessary();
ServiceData _newData = (ServiceData) _behavior.getCurrentDataForWrite(this);
{
ServiceOrderByList orderBys =
(ServiceOrderByList) _newData.getOrderBys();
RelatedFinder dependentFinder = parentFinder.orderBys();
DeepRelationshipUtility.zAddToNavigationStats(dependentFinder, orderBys != null, navigationStats);
if (orderBys != null)
{
orderBys.zCascadeAddNavigatedRelationshipsStats(dependentFinder, navigationStats);
}
}
return navigationStats;
}
@Override
public Map< RelatedFinder, StatisticCounter > zAddNavigatedRelationshipsStatsForDelete(RelatedFinder parentFinder, Map< RelatedFinder, StatisticCounter > navigationStats)
{
DeepRelationshipUtility.zAddAllDependentNavigationsStatsForDelete(parentFinder, navigationStats);
return navigationStats;
}
@Override
public Service zCascadeCopyThenInsert() throws MithraBusinessException
{
TransactionalBehavior _behavior = zGetTransactionalBehaviorForWriteWithWaitIfNecessary();
ServiceData _data = (ServiceData) _behavior.getCurrentDataForWrite(this);
ServiceOrderByList orderBys =
(ServiceOrderByList) _data.getOrderBys();
Service original = (Service) _behavior.copyThenInsert(this);
if (orderBys != null)
{
orderBys.zCascadeCopyThenInsertAll();
}
return original;
}
protected void cascadeDeleteImpl() throws MithraBusinessException
{
this.getOrderBys().cascadeDeleteAll();
this.delete();
}
public Cache zGetCache()
{
return ServiceFinder.getMithraObjectPortal().getCache();
}
public MithraObjectPortal zGetPortal()
{
return ServiceFinder.getMithraObjectPortal();
}
public Service getOriginalPersistentObject()
{
return this.zFindOriginal();
}
protected boolean issueUpdatesForNonPrimaryKeys(TransactionalBehavior behavior, MithraDataObject data, MithraDataObject newData)
{
boolean changed = false;
changed |= zUpdateLong(behavior, data, newData, ServiceFinder.authorizeCriteriaId(), false);
changed |= zUpdateLong(behavior, data, newData, ServiceFinder.conflictCriteriaId(), false);
changed |= zUpdateInteger(behavior, data, newData, ServiceFinder.ordinal(), false);
changed |= zUpdateString(behavior, data, newData, ServiceFinder.projectionName(), false);
changed |= zUpdateLong(behavior, data, newData, ServiceFinder.queryCriteriaId(), false);
changed |= zUpdateString(behavior, data, newData, ServiceFinder.serviceMultiplicity(), false);
changed |= zUpdateLong(behavior, data, newData, ServiceFinder.validateCriteriaId(), false);
return changed;
}
protected boolean issueUpdatesForPrimaryKeys(TransactionalBehavior behavior, MithraDataObject data, MithraDataObject newData)
{
boolean changed = false;
changed |= zUpdateString(behavior, data, newData, ServiceFinder.serviceGroupName(), false);
changed |= zUpdateString(behavior, data, newData, ServiceFinder.urlString(), false);
changed |= zUpdateString(behavior, data, newData, ServiceFinder.verb(), false);
return changed;
}
public Object readResolve() throws ObjectStreamException
{
ServiceAbstract result = (ServiceAbstract) super.readResolve();
if (result.persistenceState == PersistenceState.PERSISTED)
{
result.persistenceState = PERSISTED_STATE;
}
else if (result.persistenceState == PersistenceState.IN_MEMORY)
{
result.persistenceState = MEMORY_STATE;
}
return result;
}
protected static void zConfigNonTx()
{
MEMORY_STATE = PersistenceState.IN_MEMORY_NON_TRANSACTIONAL;
PERSISTED_STATE = PersistenceState.PERSISTED_NON_TRANSACTIONAL;
}
protected static void zConfigFullTx()
{
MEMORY_STATE = PersistenceState.IN_MEMORY;
PERSISTED_STATE = PersistenceState.PERSISTED;
}
protected class OrderBysAddHandlerInMemory implements DependentRelationshipAddHandler
{
public void addRelatedObject(MithraTransactionalObject relatedObject)
{
ServiceOrderBy item = (ServiceOrderBy) relatedObject;
item.setServiceGroupName(getServiceGroupName());
item.setServiceUrlString(getUrlString());
item.setServiceVerb(getVerb());
item.zSetParentContainerservice(ServiceAbstract.this);
}
}
protected class OrderBysAddHandlerPersisted implements DependentRelationshipAddHandler
{
public void addRelatedObject(MithraTransactionalObject relatedObject)
{
ServiceOrderBy item = (ServiceOrderBy) relatedObject;
item.setServiceGroupName(getServiceGroupName());
item.setServiceUrlString(getUrlString());
item.setServiceVerb(getVerb());
item.cascadeInsert();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy