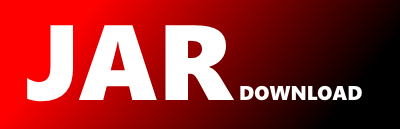
klass.model.meta.domain.ServiceData Maven / Gradle / Ivy
The newest version!
package klass.model.meta.domain;
import java.util.*;
import java.sql.Timestamp;
import java.sql.Date;
import java.math.BigDecimal;
import java.sql.Timestamp;
import java.util.*;
import java.io.*;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.gs.fw.common.mithra.*;
import com.gs.fw.common.mithra.attribute.*;
import com.gs.fw.common.mithra.util.*;
import com.gs.fw.common.mithra.notification.*;
import com.gs.fw.common.mithra.notification.listener.*;
import com.gs.fw.common.mithra.list.cursor.Cursor;
import com.gs.fw.common.mithra.bulkloader.*;
import com.gs.fw.common.mithra.finder.PrintablePreparedStatement;
import com.gs.fw.common.mithra.finder.RelatedFinder;
import com.gs.fw.common.mithra.cache.offheap.MithraOffHeapDataObject;
import com.gs.fw.common.mithra.cache.offheap.OffHeapDataStorage;
/**
* This file was automatically generated using Mithra 18.0.0. Please do not modify it.
* Add custom logic to its subclass instead.
*/
public class ServiceData
implements MithraDataObject
{
private Object[] _relationships;
private byte isNullBits0 = (byte) 15L;
private long authorizeCriteriaId;
private long conflictCriteriaId;
private int ordinal;
private String projectionName;
private long queryCriteriaId;
private String serviceGroupName;
private String serviceMultiplicity;
private String urlString;
private long validateCriteriaId;
private String verb;
public boolean isOrdinalNull()
{
return false;
}
public boolean isProjectionNameNull()
{
return this.getProjectionName() == null;
}
public final boolean isServiceGroupNameNull()
{
return this.getServiceGroupName() == null;
}
public boolean isServiceMultiplicityNull()
{
return this.getServiceMultiplicity() == null;
}
public final boolean isUrlStringNull()
{
return this.getUrlString() == null;
}
public final boolean isVerbNull()
{
return this.getVerb() == null;
}
public boolean isQueryCriteriaIdNull()
{
return (isNullBits0 & 1) != 0 ;
}
public boolean isAuthorizeCriteriaIdNull()
{
return (isNullBits0 & 1 << 1) != 0 ;
}
public boolean isValidateCriteriaIdNull()
{
return (isNullBits0 & 1 << 2) != 0 ;
}
public boolean isConflictCriteriaIdNull()
{
return (isNullBits0 & 1 << 3) != 0 ;
}
public void zSerializeFullData(ObjectOutput out) throws IOException
{
zWriteNullBits(out);
out.writeLong(this.authorizeCriteriaId);
out.writeLong(this.conflictCriteriaId);
out.writeInt(this.ordinal);
out.writeObject(this.projectionName);
out.writeLong(this.queryCriteriaId);
out.writeObject(this.serviceGroupName);
out.writeObject(this.serviceMultiplicity);
out.writeObject(this.urlString);
out.writeLong(this.validateCriteriaId);
out.writeObject(this.verb);
}
private void zWriteNullBits(ObjectOutput out) throws IOException
{
out.writeByte(this.isNullBits0);
}
public long getAuthorizeCriteriaId()
{
return this.authorizeCriteriaId;
}
public void setAuthorizeCriteriaId(long value)
{
this.authorizeCriteriaId = value;
isNullBits0 = (byte)((int)isNullBits0 & ~( 1 << 1));
}
public long getConflictCriteriaId()
{
return this.conflictCriteriaId;
}
public void setConflictCriteriaId(long value)
{
this.conflictCriteriaId = value;
isNullBits0 = (byte)((int)isNullBits0 & ~( 1 << 3));
}
public int getOrdinal()
{
return this.ordinal;
}
public void setOrdinal(int value)
{
this.ordinal = value;
}
public void setOrdinalNull()
{
throw new RuntimeException("should never be called");
}
public String getProjectionName()
{
return this.projectionName;
}
public int zGetProjectionNameAsInt()
{
return StringPool.getInstance().getOffHeapAddressWithoutAdding(projectionName);
}
public void setProjectionName(String value)
{
this.projectionName = StringPool.getInstance().getOrAddToCache(value, ServiceFinder.isFullCache());
}
public void setProjectionNameNull()
{
this.setProjectionName(null);
}
public long getQueryCriteriaId()
{
return this.queryCriteriaId;
}
public void setQueryCriteriaId(long value)
{
this.queryCriteriaId = value;
isNullBits0 = (byte)((int)isNullBits0 & ~( 1));
}
public String getServiceGroupName()
{
return this.serviceGroupName;
}
public int zGetServiceGroupNameAsInt()
{
return StringPool.getInstance().getOffHeapAddressWithoutAdding(serviceGroupName);
}
public void setServiceGroupName(String value)
{
this.serviceGroupName = StringPool.getInstance().getOrAddToCache(value, ServiceFinder.isFullCache());
}
public void setServiceGroupNameNull()
{
this.setServiceGroupName(null);
}
public String getServiceMultiplicity()
{
return this.serviceMultiplicity;
}
public int zGetServiceMultiplicityAsInt()
{
return StringPool.getInstance().getOffHeapAddressWithoutAdding(serviceMultiplicity);
}
public void setServiceMultiplicity(String value)
{
this.serviceMultiplicity = StringPool.getInstance().getOrAddToCache(value, ServiceFinder.isFullCache());
}
public void setServiceMultiplicityNull()
{
this.setServiceMultiplicity(null);
}
public String getUrlString()
{
return this.urlString;
}
public int zGetUrlStringAsInt()
{
return StringPool.getInstance().getOffHeapAddressWithoutAdding(urlString);
}
public void setUrlString(String value)
{
this.urlString = StringPool.getInstance().getOrAddToCache(value, ServiceFinder.isFullCache());
}
public void setUrlStringNull()
{
this.setUrlString(null);
}
public long getValidateCriteriaId()
{
return this.validateCriteriaId;
}
public void setValidateCriteriaId(long value)
{
this.validateCriteriaId = value;
isNullBits0 = (byte)((int)isNullBits0 & ~( 1 << 2));
}
public String getVerb()
{
return this.verb;
}
public int zGetVerbAsInt()
{
return StringPool.getInstance().getOffHeapAddressWithoutAdding(verb);
}
public void setVerb(String value)
{
this.verb = StringPool.getInstance().getOrAddToCache(value, ServiceFinder.isFullCache());
}
public void setVerbNull()
{
this.setVerb(null);
}
public void setQueryCriteriaIdNull()
{
isNullBits0 = (byte)((int)isNullBits0 | 1);
}
public void setAuthorizeCriteriaIdNull()
{
isNullBits0 = (byte)((int)isNullBits0 | 1 << 1);
}
public void setValidateCriteriaIdNull()
{
isNullBits0 = (byte)((int)isNullBits0 | 1 << 2);
}
public void setConflictCriteriaIdNull()
{
isNullBits0 = (byte)((int)isNullBits0 | 1 << 3);
}
public byte zGetIsNullBits0()
{
return this.isNullBits0;
}
public void zSetIsNullBits0(byte newValue)
{
this.isNullBits0 = newValue;
}
protected void copyInto(ServiceData copy, boolean withRelationships)
{
copy.isNullBits0 = this.isNullBits0;
copy.authorizeCriteriaId = this.authorizeCriteriaId;
copy.conflictCriteriaId = this.conflictCriteriaId;
copy.ordinal = this.ordinal;
copy.projectionName = this.projectionName;
copy.queryCriteriaId = this.queryCriteriaId;
copy.serviceGroupName = this.serviceGroupName;
copy.serviceMultiplicity = this.serviceMultiplicity;
copy.urlString = this.urlString;
copy.validateCriteriaId = this.validateCriteriaId;
copy.verb = this.verb;
if (withRelationships)
{
if (_relationships != null)
{
copy._relationships = new Object[7];
System.arraycopy(_relationships, 0, copy._relationships, 0, _relationships.length);
}
}
}
public void zDeserializeFullData(ObjectInput in) throws IOException, ClassNotFoundException
{
this.isNullBits0 = in.readByte();
this.authorizeCriteriaId = in.readLong();
this.conflictCriteriaId = in.readLong();
this.ordinal = in.readInt();
this.projectionName = StringPool.getInstance().getOrAddToCache((String)in.readObject(), ServiceFinder.isFullCache());
this.queryCriteriaId = in.readLong();
this.serviceGroupName = StringPool.getInstance().getOrAddToCache((String)in.readObject(), ServiceFinder.isFullCache());
this.serviceMultiplicity = StringPool.getInstance().getOrAddToCache((String)in.readObject(), ServiceFinder.isFullCache());
this.urlString = StringPool.getInstance().getOrAddToCache((String)in.readObject(), ServiceFinder.isFullCache());
this.validateCriteriaId = in.readLong();
this.verb = StringPool.getInstance().getOrAddToCache((String)in.readObject(), ServiceFinder.isFullCache());
}
public boolean hasSamePrimaryKeyIgnoringAsOfAttributes(MithraDataObject other)
{
if (this == other) return true;
final ServiceData otherData = (ServiceData) other;
if (!isServiceGroupNameNull() ? !getServiceGroupName().equals(otherData.getServiceGroupName()) : !otherData.isServiceGroupNameNull())
{
return false;
}
if (!isUrlStringNull() ? !getUrlString().equals(otherData.getUrlString()) : !otherData.isUrlStringNull())
{
return false;
}
if (!isVerbNull() ? !getVerb().equals(otherData.getVerb()) : !otherData.isVerbNull())
{
return false;
}
return true;
}
public void zSerializePrimaryKey(ObjectOutput out) throws IOException
{
out.writeObject(this.serviceGroupName);
out.writeObject(this.urlString);
out.writeObject(this.verb);
}
public void zDeserializePrimaryKey(ObjectInput in) throws IOException, ClassNotFoundException
{
this.serviceGroupName = StringPool.getInstance().getOrAddToCache((String)in.readObject(), ServiceFinder.isFullCache());
this.urlString = StringPool.getInstance().getOrAddToCache((String)in.readObject(), ServiceFinder.isFullCache());
this.verb = StringPool.getInstance().getOrAddToCache((String)in.readObject(), ServiceFinder.isFullCache());
}
public void clearRelationships()
{
_relationships = null;
clearAllDirectRefs();
}
public void clearAllDirectRefs()
{
}
public Object getProjection()
{
if (_relationships != null)
{
return _relationships[3];
}
return null;
}
public void setProjection(Object related)
{
if (_relationships == null)
{
_relationships = new Object[7];
}
_relationships[3] = related;
}
public Object getQueryCriteria()
{
if (_relationships != null)
{
return _relationships[4];
}
return null;
}
public void setQueryCriteria(Object related)
{
if (_relationships == null)
{
_relationships = new Object[7];
}
_relationships[4] = related;
}
public Object getAuthorizeCriteria()
{
if (_relationships != null)
{
return _relationships[0];
}
return null;
}
public void setAuthorizeCriteria(Object related)
{
if (_relationships == null)
{
_relationships = new Object[7];
}
_relationships[0] = related;
}
public Object getValidateCriteria()
{
if (_relationships != null)
{
return _relationships[6];
}
return null;
}
public void setValidateCriteria(Object related)
{
if (_relationships == null)
{
_relationships = new Object[7];
}
_relationships[6] = related;
}
public Object getConflictCriteria()
{
if (_relationships != null)
{
return _relationships[1];
}
return null;
}
public void setConflictCriteria(Object related)
{
if (_relationships == null)
{
_relationships = new Object[7];
}
_relationships[1] = related;
}
public Object getOrderBys()
{
if (_relationships != null)
{
return _relationships[2];
}
return null;
}
public void setOrderBys(Object related)
{
if (_relationships == null)
{
_relationships = new Object[7];
}
_relationships[2] = related;
}
public Object getUrl()
{
if (_relationships != null)
{
return _relationships[5];
}
return null;
}
public void setUrl(Object related)
{
if (_relationships == null)
{
_relationships = new Object[7];
}
_relationships[5] = related;
}
public void zSerializeRelationships(ObjectOutputStream out) throws IOException
{
if (_relationships == null)
{
out.writeInt(0);
return;
}
out.writeInt(_relationships.length);
for(int i=0;i<_relationships.length;i++)
{
out.writeObject(_relationships[i]);
}
}
public void zDeserializeRelationships(ObjectInputStream in) throws IOException, ClassNotFoundException
{
int total = in.readInt();
if(total > 0)
{
_relationships = new Object[total];
for(int i=0;i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy