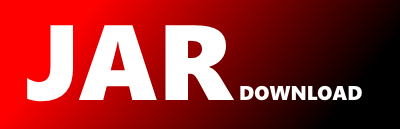
klass.model.meta.domain.VariableReferenceFinder Maven / Gradle / Ivy
The newest version!
package klass.model.meta.domain;
import java.math.BigDecimal;
import java.sql.Timestamp;
import java.util.*;
import java.io.*;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.gs.fw.common.mithra.*;
import com.gs.fw.common.mithra.attribute.*;
import com.gs.fw.common.mithra.util.*;
import com.gs.fw.common.mithra.notification.*;
import com.gs.fw.common.mithra.notification.listener.*;
import com.gs.fw.common.mithra.list.cursor.Cursor;
import com.gs.fw.common.mithra.bulkloader.*;
import com.gs.fw.common.mithra.*;
import com.gs.fw.common.mithra.attribute.*;
import com.gs.fw.common.mithra.attribute.calculator.procedure.ObjectProcedure;
import com.gs.fw.common.mithra.behavior.txparticipation.*;
import com.gs.fw.common.mithra.cache.Cache;
import com.gs.fw.common.mithra.cache.bean.*;
import com.gs.fw.common.mithra.extractor.*;
import com.gs.fw.common.mithra.finder.*;
import com.gs.fw.common.mithra.finder.booleanop.*;
import com.gs.fw.common.mithra.finder.integer.*;
import com.gs.fw.common.mithra.finder.longop.*;
import com.gs.fw.common.mithra.finder.orderby.OrderBy;
import com.gs.fw.common.mithra.finder.string.*;
import com.gs.fw.common.mithra.extractor.NormalAndListValueSelector;
import com.gs.fw.common.mithra.list.NulledRelation;
import com.gs.fw.common.mithra.querycache.CachedQuery;
import com.gs.fw.common.mithra.querycache.QueryCache;
import com.gs.fw.common.mithra.portal.*;
import com.gs.fw.common.mithra.remote.*;
import com.gs.fw.common.mithra.transaction.MithraObjectPersister;
import com.gs.fw.common.mithra.util.TimestampPool;
import org.eclipse.collections.impl.map.mutable.UnifiedMap;
import java.io.Serializable;
/**
* This file was automatically generated using Mithra 18.0.0. Please do not modify it.
* Add custom logic to its subclass instead.
*/
public class VariableReferenceFinder
{
private static final String IMPL_CLASS_NAME_WITH_SLASHES = "klass/model/meta/domain/VariableReference";
private static final String BUSINESS_CLASS_NAME_WITH_DOTS = "klass.model.meta.domain.VariableReference";
private static final FinderMethodMap finderMethodMap;
private static boolean isFullCache;
private static boolean isOffHeap;
private static volatile MithraObjectPortal objectPortal = new UninitializedPortal("klass.model.meta.domain.VariableReference");
private static final VariableReferenceSingleFinder finder = new VariableReferenceSingleFinder();
private static ConstantStringSet[] constantStringSets = new ConstantStringSet[0];
private static ConstantIntSet[] constantIntSets = new ConstantIntSet[0];
private static ConstantShortSet[] constantShortSets = new ConstantShortSet[0];
static
{
finderMethodMap = new FinderMethodMap(VariableReferenceFinder.VariableReferenceRelatedFinder.class);
finderMethodMap.addNormalAttributeName("id");
finderMethodMap.addNormalAttributeName("parameterId");
finderMethodMap.addRelationshipName("parameter");
finderMethodMap.addRelationshipName("expressionValueSuperClass");
}
public static Attribute[] allPersistentAttributes()
{
return finder.getPersistentAttributes();
}
public static List allRelatedFinders()
{
return finder.getRelationshipFinders();
}
public static List allDependentRelatedFinders()
{
return finder.getDependentRelationshipFinders();
}
public static ConstantStringSet zGetConstantStringSet(int index)
{
return constantStringSets[index];
}
public static ConstantIntSet zGetConstantIntSet(int index)
{
return constantIntSets[index];
}
public static ConstantShortSet zGetConstantShortSet(int index)
{
return constantShortSets[index];
}
public static Mapper zGetConstantJoin(int index)
{
return getConstantJoinPool()[index];
}
private static Mapper[] constantJoinPool;
private static Mapper[] getConstantJoinPool()
{
if (constantJoinPool == null)
{
Mapper[] result = new Mapper[2];
result[0] = VariableReferenceFinder.parameterId().constructEqualityMapper(ParameterFinder.id());
result[1] = ParameterFinder.id().constructEqualityMapper(VariableReferenceFinder.parameterId());
constantJoinPool = result;
}
return constantJoinPool;
}
public static SourceAttributeType getSourceAttributeType()
{
return null;
}
public static VariableReference findOne(com.gs.fw.finder.Operation operation)
{
return findOne(operation, false);
}
public static VariableReference findOneBypassCache(com.gs.fw.finder.Operation operation)
{
return findOne(operation, true);
}
public static VariableReferenceList findMany(com.gs.fw.finder.Operation operation)
{
return (VariableReferenceList) finder.findMany(operation);
}
public static VariableReferenceList findManyBypassCache(com.gs.fw.finder.Operation operation)
{
return (VariableReferenceList) finder.findManyBypassCache(operation);
}
private static VariableReference findOne(com.gs.fw.finder.Operation operation, boolean bypassCache)
{
List found = getMithraObjectPortal().find((Operation) operation, bypassCache);
return (VariableReference) FinderUtils.findOne(found);
}
public static VariableReference findByPrimaryKey(long id)
{
return finder.findByPrimaryKey(id);
}
private static final RelationshipHashStrategy forPrimaryKey = new PrimaryKeyRhs();
private static final class PrimaryKeyRhs implements RelationshipHashStrategy
{
public boolean equalsForRelationship(Object _srcObject, Object _srcData, Object _targetData, Timestamp _asOfDate0, Timestamp _asOfDate1)
{
I3O3L3 _bean = (I3O3L3) _srcData;
VariableReferenceData _castedTargetData = (VariableReferenceData) _targetData;
if (_bean.getL1AsLong() == _castedTargetData.getId())
{
return true;
}
return false;
}
public int computeHashCodeFromRelated(Object _srcObject, Object _srcData)
{
I3O3L3 _bean = (I3O3L3) _srcData;
return HashUtil.hash(_bean.getL1AsLong());
}
public int computeOffHeapHashCodeFromRelated(Object _srcObject, Object _srcData)
{
I3O3L3 _bean = (I3O3L3) _srcData;
return HashUtil.hash(_bean.getL1AsLong());
}
}
public static VariableReference zFindOneForRelationship(Operation operation)
{
List found = getMithraObjectPortal().findAsCachedQuery(operation, null, false, true, 0).getResult();
return (VariableReference) FinderUtils.findOne(found);
}
public static MithraObjectPortal getMithraObjectPortal()
{
return objectPortal.getInitializedPortal();
}
public static void clearQueryCache()
{
objectPortal.clearQueryCache();
}
public static void reloadCache()
{
objectPortal.reloadCache();
}
public static class VariableReferenceRelatedFinder extends AbstractRelatedFinder
{
private List relationshipFinders;
private List dependentRelationshipFinders;
private LongAttribute id;
private LongAttribute parameterId;
private VariableReferenceParameterFinderSubclass parameter;
private VariableReferenceExpressionValueSuperClassFinderSubclass expressionValueSuperClass;
public VariableReferenceRelatedFinder()
{
super();
}
public VariableReferenceRelatedFinder(Mapper mapper)
{
super(mapper);
}
public String getFinderClassName()
{
return "klass.model.meta.domain.VariableReferenceFinder";
}
public RelatedFinder getRelationshipFinderByName(String relationshipName)
{
return VariableReferenceFinder.finderMethodMap.getRelationshipFinderByName(relationshipName, this);
}
public Attribute getAttributeByName(String attributeName)
{
return VariableReferenceFinder.finderMethodMap.getAttributeByName(attributeName, this);
}
public com.gs.fw.common.mithra.extractor.Function getAttributeOrRelationshipSelector(String attributeName)
{
return VariableReferenceFinder.finderMethodMap.getAttributeOrRelationshipSelectorFunction(attributeName, this);
}
public Attribute[] getPersistentAttributes()
{
Attribute[] attributes = new Attribute[2];
attributes[0] = this.id();
attributes[1] = this.parameterId();
return attributes;
}
public List getRelationshipFinders()
{
if (relationshipFinders == null)
{
List relatedFinders = new ArrayList(2);
relatedFinders.add(this.parameter());
relatedFinders.add(this.expressionValueSuperClass());
relationshipFinders = Collections.unmodifiableList(relatedFinders);
}
return relationshipFinders;
}
public List getDependentRelationshipFinders()
{
if (dependentRelationshipFinders == null)
{
List dependentRelatedFinders = new ArrayList(0);
dependentRelationshipFinders = Collections.unmodifiableList(dependentRelatedFinders);
}
return dependentRelationshipFinders;
}
public VariableReference findOne(com.gs.fw.finder.Operation operation)
{
return VariableReferenceFinder.findOne(operation, false);
}
public VariableReference findOneBypassCache(com.gs.fw.finder.Operation operation)
{
return VariableReferenceFinder.findOne(operation, true);
}
public MithraList extends VariableReference> findMany(com.gs.fw.finder.Operation operation)
{
return new VariableReferenceList((Operation) operation);
}
public MithraList extends VariableReference> findManyBypassCache(com.gs.fw.finder.Operation operation)
{
VariableReferenceList result = (VariableReferenceList) this.findMany(operation);
result.setBypassCache(true);
return result;
}
public MithraList extends VariableReference> constructEmptyList()
{
return new VariableReferenceList();
}
public int getSerialVersionId()
{
return -372866896;
}
public boolean isPure()
{
return false;
}
public boolean isTemporary()
{
return false;
}
public int getHierarchyDepth()
{
return 0;
}
public LongAttribute id()
{
LongAttribute result = this.id;
if (result == null)
{
result = mapper == null ? SingleColumnLongAttribute.generate("\"ID\"","","id",BUSINESS_CLASS_NAME_WITH_DOTS,IMPL_CLASS_NAME_WITH_SLASHES,false,false,this,null,true,false,false,-1,-1,-1,false, false) :
new MappedLongAttribute(VariableReferenceFinder.id(), this.mapper, this.zGetValueSelector());
result.zSetOwningRelationship("expressionValueSuperClass");
result.zSetOwningReverseRelationship("klass.model.meta.domain", "ExpressionValue", "variableReferenceSubClass");
this.id = result;
}
return result;
}
public LongAttribute parameterId()
{
LongAttribute result = this.parameterId;
if (result == null)
{
result = mapper == null ? SingleColumnLongAttribute.generate("\"PARAMETER_ID\"","","parameterId",BUSINESS_CLASS_NAME_WITH_DOTS,IMPL_CLASS_NAME_WITH_SLASHES,false,false,this,null,true,false,false,-1,-1,-1,false, false) :
new MappedLongAttribute(VariableReferenceFinder.parameterId(), this.mapper, this.zGetValueSelector());
result.zSetOwningRelationship("parameter");
result.zSetOwningReverseRelationship("klass.model.meta.domain", "Parameter", "variableReferences");
this.parameterId = result;
}
return result;
}
public ParameterFinder.ParameterSingleFinderForRelatedClasses parameter()
{
VariableReferenceParameterFinderSubclass result = this.parameter;
if (result == null)
{
Mapper newMapper = combineWithMapperIfExists(VariableReferenceFinder.zGetVariableReferenceParameterReverseMapper());
newMapper.setToMany(false);
result = new VariableReferenceParameterFinderSubclass(newMapper , this.zGetValueSelector() );
this.parameter = result;
}
return result;
}
public ExpressionValueFinder.ExpressionValueSingleFinderForRelatedClasses expressionValueSuperClass()
{
VariableReferenceExpressionValueSuperClassFinderSubclass result = this.expressionValueSuperClass;
if (result == null)
{
Mapper newMapper = combineWithMapperIfExists(ExpressionValueFinder.zGetExpressionValueVariableReferenceSubClassMapper());
newMapper.setToMany(false);
result = new VariableReferenceExpressionValueSuperClassFinderSubclass(newMapper , this.zGetValueSelector() );
this.expressionValueSuperClass = result;
}
return result;
}
public Attribute getSourceAttribute()
{
return null;
}
private Mapper combineWithMapperIfExists(Mapper newMapper)
{
if (this.mapper != null)
{
return new LinkedMapper(this.mapper, newMapper);
}
return newMapper;
}
public Attribute[] getPrimaryKeyAttributes()
{
return VariableReferenceFinder.getPrimaryKeyAttributes();
}
public VersionAttribute getVersionAttribute()
{
return null;
}
public MithraObjectPortal getMithraObjectPortal()
{
return VariableReferenceFinder.getMithraObjectPortal();
}
}
public static class VariableReferenceCollectionFinder extends VariableReferenceRelatedFinder
{
public VariableReferenceCollectionFinder(Mapper mapper)
{
super(mapper);
}
}
public static abstract class VariableReferenceCollectionFinderForRelatedClasses
extends VariableReferenceCollectionFinder
implements DeepRelationshipAttribute
{
public VariableReferenceCollectionFinderForRelatedClasses(Mapper mapper)
{
super(mapper);
}
protected NormalAndListValueSelector zGetValueSelector()
{
return this;
}
}
public static class VariableReferenceSingleFinder extends VariableReferenceRelatedFinder
implements ToOneFinder
{
public VariableReferenceSingleFinder(Mapper mapper)
{
super(mapper);
}
public VariableReferenceSingleFinder()
{
super(null);
}
public Operation eq(VariableReference other)
{
return this.id().eq(other.getId())
;
}
// this implementation uses private API. Do NOT copy to application code. Application code must use normal operations for lookups.
public VariableReference findByPrimaryKey(long id)
{
VariableReference _result = null;
Operation _op = null;
Object _related = null;
{
I3O3L3 _bean = I3O3L3.POOL.getOrConstruct();
_bean.setL1AsLong(id);
MithraObjectPortal _portal = this.getMithraObjectPortal();
_related = _portal.getAsOneFromCacheForFind(_bean, _bean, forPrimaryKey, null, null);
_bean.release();
}
if (!(_related instanceof NulledRelation)) _result = (VariableReference) _related;
if (_related == null)
{
_op = this.id().eq(id);
}
if (_op != null)
{
_result = this.findOne(_op);
}
return _result;
}
}
public static abstract class VariableReferenceSingleFinderForRelatedClasses extends VariableReferenceSingleFinder implements DeepRelationshipAttribute
{
public VariableReferenceSingleFinderForRelatedClasses(Mapper mapper)
{
super(mapper);
}
protected NormalAndListValueSelector zGetValueSelector()
{
return this;
}
}
private static Mapper parameterReverseMapper = null;
public static Mapper zGetVariableReferenceParameterReverseMapper()
{
if (parameterReverseMapper == null)
{
parameterReverseMapper = zConstructVariableReferenceParameterReverseMapper();
}
return parameterReverseMapper;
}
private static Mapper parameterMapper = null;
public static Mapper zGetVariableReferenceParameterMapper()
{
if (parameterMapper == null)
{
parameterMapper = zConstructVariableReferenceParameterMapper();
}
return parameterMapper;
}
private static Mapper parameterPureReverseMapper = null;
public static Mapper zGetVariableReferenceParameterPureReverseMapper()
{
if (parameterPureReverseMapper == null)
{
parameterPureReverseMapper = zConstructVariableReferenceParameterPureReverseMapper();
}
return parameterPureReverseMapper;
}
private static Mapper zConstructVariableReferenceParameterPureReverseMapper()
{
Mapper parameterMapper = VariableReferenceFinder.zGetConstantJoin(0);
parameterMapper.setName("parameter");
return parameterMapper;
}
private static Mapper zConstructVariableReferenceParameterReverseMapper()
{
Mapper parameterMapper = VariableReferenceFinder.zGetConstantJoin(0);
parameterMapper.setName("parameter");
return parameterMapper;
}
private static Mapper zConstructVariableReferenceParameterMapper()
{
Mapper parameterMapper = VariableReferenceFinder.zGetConstantJoin(1);
parameterMapper.setName("variableReferences");
return parameterMapper;
}
/** maps to \"VARIABLE_REFERENCE\".\"ID\" **/
public static LongAttribute id()
{
return finder.id();
}
/** maps to \"VARIABLE_REFERENCE\".\"PARAMETER_ID\" **/
public static LongAttribute parameterId()
{
return finder.parameterId();
}
public static ParameterFinder.ParameterSingleFinderForRelatedClasses parameter()
{
return finder.parameter();
}
public static class VariableReferenceParameterFinderSubclass extends ParameterFinder.ParameterSingleFinderForRelatedClasses
{
public VariableReferenceParameterFinderSubclass(Mapper mapper, NormalAndListValueSelector parentSelector )
{
super(mapper);
this._parentSelector = (AbstractRelatedFinder) parentSelector;
this._orderBy = null;
this._type = SIMPLE_TO_ONE;
this._name = "parameter";
}
public DeepRelationshipAttribute copy()
{
return new VariableReferenceParameterFinderSubclass(zGetMapper(), (NormalAndListValueSelector) this._parentSelector
);
}
protected Parameter plainValueOf(VariableReference _obj)
{
return _obj.getParameter();
}
protected ParameterList plainListValueOf(Object _obj)
{
return ((VariableReferenceList)_obj).getParameters();
}
}
public static ExpressionValueFinder.ExpressionValueSingleFinderForRelatedClasses expressionValueSuperClass()
{
return finder.expressionValueSuperClass();
}
public static class VariableReferenceExpressionValueSuperClassFinderSubclass extends ExpressionValueFinder.ExpressionValueSingleFinderForRelatedClasses
{
public VariableReferenceExpressionValueSuperClassFinderSubclass(Mapper mapper, NormalAndListValueSelector parentSelector )
{
super(mapper);
this._parentSelector = (AbstractRelatedFinder) parentSelector;
this._orderBy = null;
this._type = SIMPLE_TO_ONE;
this._name = "expressionValueSuperClass";
}
public DeepRelationshipAttribute copy()
{
return new VariableReferenceExpressionValueSuperClassFinderSubclass(zGetMapper(), (NormalAndListValueSelector) this._parentSelector
);
}
protected ExpressionValue plainValueOf(VariableReference _obj)
{
return _obj.getExpressionValueSuperClass();
}
protected ExpressionValueList plainListValueOf(Object _obj)
{
return ((VariableReferenceList)_obj).getExpressionValueSuperClass();
}
}
public static Operation eq(VariableReference other)
{
return finder.eq(other);
}
public static Operation all()
{
return new All(id());
}
public static VariableReferenceSingleFinder getFinderInstance()
{
return finder;
}
public static Attribute[] getPrimaryKeyAttributes()
{
return new Attribute[]
{
id()
}
;
}
public static Attribute[] getImmutableAttributes()
{
return new Attribute[]
{
id()
, id()
}
;
}
public static AsOfAttribute[] getAsOfAttributes()
{
return null;
}
protected static void initializeIndicies(Cache cache)
{
cache.addIndex("0 Index", new Attribute[]
{
parameterId()
}
);
}
protected static void initializePortal(MithraObjectDeserializer objectFactory, Cache cache,
MithraConfigurationManager.Config config)
{
initializeIndicies(cache);
isFullCache = cache.isFullCache();
isOffHeap = cache.isOffHeap();
MithraObjectPortal portal;
if (config.isParticipatingInTx())
{
portal = new MithraTransactionalPortal(objectFactory, cache, getFinderInstance(),
config.getRelationshipCacheSize(), config.getMinQueriesToKeep(), null,
null, null, 0,
(MithraObjectPersister) objectFactory);
}
else
{
portal = new MithraReadOnlyPortal(objectFactory, cache, getFinderInstance(),
config.getRelationshipCacheSize(), config.getMinQueriesToKeep(), null,
null, null, 0,
(MithraObjectPersister) objectFactory);
}
portal.setParentFinders(new RelatedFinder[]
{
ExpressionValueFinder.getFinderInstance(),ParameterFinder.getFinderInstance(),
}
);
config.initializePortal(portal);
objectPortal.destroy();
objectPortal = portal;
}
protected static void initializeClientPortal(MithraObjectDeserializer objectFactory, Cache cache,
MithraConfigurationManager.Config config)
{
initializeIndicies(cache);
isFullCache = cache.isFullCache();
isOffHeap = cache.isOffHeap();
MithraObjectPortal portal;
if (config.isParticipatingInTx())
{
portal = new MithraTransactionalPortal(objectFactory, cache, getFinderInstance(),
config.getRelationshipCacheSize(), config.getMinQueriesToKeep(),
null, null,
null, 0,
new RemoteMithraObjectPersister(config.getRemoteMithraService(), getFinderInstance(), false));
}
else
{
portal = new MithraReadOnlyPortal(objectFactory, cache, getFinderInstance(),
config.getRelationshipCacheSize(), config.getMinQueriesToKeep(),
null, null,
null, 0,
new RemoteMithraObjectPersister(config.getRemoteMithraService(), getFinderInstance(), false));
}
portal.setParentFinders(new RelatedFinder[]
{
ExpressionValueFinder.getFinderInstance(),ParameterFinder.getFinderInstance(),
}
);
config.initializePortal(portal);
objectPortal.destroy();
objectPortal = portal;
}
public static boolean isFullCache()
{
return isFullCache;
}
public static boolean isOffHeap()
{
return isOffHeap;
}
public static Attribute getAttributeByName(String attributeName)
{
return finder.getAttributeByName(attributeName);
}
public static com.gs.fw.common.mithra.extractor.Function getAttributeOrRelationshipSelector(String attributeName)
{
return finder.getAttributeOrRelationshipSelector(attributeName);
}
public static RelatedFinder getRelatedFinderByName(String relationshipName)
{
return finder.getRelationshipFinderByName(relationshipName);
}
public static DoubleAttribute[] zGetDoubleAttributes()
{
DoubleAttribute[] result = new DoubleAttribute[0];
return result;
}
public static BigDecimalAttribute[] zGetBigDecimalAttributes()
{
BigDecimalAttribute[] result = new BigDecimalAttribute[0];
return result;
}
public static void zResetPortal()
{
objectPortal.destroy();
objectPortal = new UninitializedPortal("klass.model.meta.domain.VariableReference");
isFullCache = false;
isOffHeap = false;
}
public static void setTransactionModeFullTransactionParticipation(MithraTransaction tx)
{
tx.setTxParticipationMode(objectPortal, FullTransactionalParticipationMode.getInstance());
}
public static void setTransactionModeReadCacheUpdateCausesRefreshAndLock(MithraTransaction tx)
{
tx.setTxParticipationMode(objectPortal, ReadCacheUpdateCausesRefreshAndLockTxParticipationMode.getInstance());
}
public static void registerForNotification(MithraApplicationClassLevelNotificationListener listener)
{
getMithraObjectPortal().registerForApplicationClassLevelNotification(listener);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy