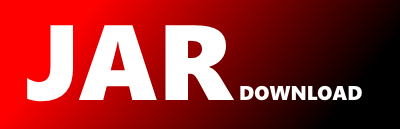
cool.linco.common.convert.ObjectMapUtils Maven / Gradle / Ivy
The newest version!
package cool.linco.common.convert;
import java.lang.reflect.Field;
import java.lang.reflect.Modifier;
import java.util.*;
import java.util.stream.Collectors;
import java.util.stream.Stream;
public class ObjectMapUtils {
public static Map objectToMap(Object obj){
Map map = new HashMap();
try {
for (Field field : getAllFields(obj.getClass())) {
field.setAccessible(true);
String fieldName = field.getName();
map.put(fieldName, field.get(obj));
}
} catch (IllegalAccessException e) {
throw new RuntimeException(e);
}
return map;
}
public static Map makeKeyValueFromCollection(String keyField, String valueField, Collection source){
Map mapValues = new HashMap<>();
for (Object item: source){
Map mapValue = ObjectMapUtils.objectToMap(item, Stream.of(keyField, valueField).collect(Collectors.toSet()));
if (mapValue.containsKey(keyField) && mapValue.containsKey(valueField)){
mapValues.put(String.valueOf(mapValue.get(keyField)), mapValue.get(valueField));
}
}
return mapValues;
}
public static Map objectToMap(Object obj, Set choose){
Map map = new HashMap<>();
try {
for (Field field : getAllFields(obj.getClass())) {
String fieldName = field.getName();
if (choose.contains(fieldName)) {
field.setAccessible(true);
map.put(fieldName, field.get(obj));
}
}
} catch (IllegalAccessException e) {
throw new RuntimeException(e);
}
return map;
}
private static Field[] getAllFields(Class> clazz){
List fieldList = new ArrayList<>();
while (clazz != Object.class){
Field[] fields = clazz.getDeclaredFields();
// 排除静态字段和final字段
Arrays.stream(fields).filter(field -> !Modifier.isStatic(field.getModifiers()) && !Modifier.isFinal(field.getModifiers()))
.forEach(fieldList::add);
clazz = clazz.getSuperclass();
}
Field[] fields = new Field[fieldList.size()];
fieldList.toArray(fields);
return fields;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy