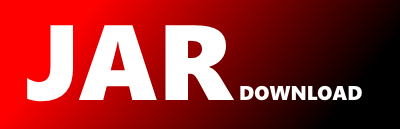
cool.scx.logging.spi.jdk.ScxJDKLogger Maven / Gradle / Ivy
package cool.scx.logging.spi.jdk;
import cool.scx.logging.ScxLogger;
import cool.scx.logging.ScxLoggerFactory;
import java.util.MissingResourceException;
import java.util.ResourceBundle;
public class ScxJDKLogger implements System.Logger {
private final ScxLogger scxLogger;
public ScxJDKLogger(String name) {
this.scxLogger = ScxLoggerFactory.getLogger(name);
}
// Copied from org.slf4j.helpers.NormalizedParameters.trimmedCopy
private static Object[] trimmedCopy(final Object[] argArray) {
if (argArray == null || argArray.length == 0) {
throw new IllegalStateException("non-sensical empty or null argument array");
}
final int trimmedLen = argArray.length - 1;
Object[] trimmed = new Object[trimmedLen];
if (trimmedLen > 0) {
System.arraycopy(argArray, 0, trimmed, 0, trimmedLen);
}
return trimmed;
}
// Copied from org.slf4j.helpers.NormalizedParameters.getThrowableCandidate
public static Throwable getThrowableCandidate(final Object[] argArray) {
if (argArray == null || argArray.length == 0) {
return null;
}
final Object lastEntry = argArray[argArray.length - 1];
if (lastEntry instanceof Throwable) {
return (Throwable) lastEntry;
}
return null;
}
// Copied from jdk.internal.logger.SimpleConsoleLogger.getString
private static String getString(ResourceBundle bundle, String key) {
if (bundle == null || key == null) {
return key;
}
try {
return bundle.getString(key);
} catch (MissingResourceException x) {
// Emulate what java.util.logging Formatters do
// We don't want unchecked exception to propagate up to
// the caller's code.
return key;
}
}
// Copied from jdk.internal.logger.SimpleConsoleLogger.Formatting.formatMessage
private static String formatMessage(String format, Object... parameters) {
// Do the formatting.
try {
if (parameters == null || parameters.length == 0) {
// No parameters. Just return format string.
return format;
}
// Is it a java.text style format?
// Ideally we could match with
// Pattern.compile("\\{\\d").matcher(format).find())
// However the cost is 14% higher, so we cheaply check for
//
boolean isJavaTestFormat = false;
final int len = format.length();
for (int i = 0; i < len - 2; i++) {
final char c = format.charAt(i);
if (c == '{') {
final int d = format.charAt(i + 1);
if (d >= '0' && d <= '9') {
isJavaTestFormat = true;
break;
}
}
}
if (isJavaTestFormat) {
return java.text.MessageFormat.format(format, parameters);
}
return format;
} catch (Exception ex) {
// Formatting failed: use format string.
return format;
}
}
@Override
public String getName() {
return this.scxLogger.name();
}
@Override
public boolean isLoggable(Level level) {
return level.getSeverity() >= this.scxLogger.level().getSeverity();
}
@Override
public void log(Level level, ResourceBundle bundle, String msg, Throwable thrown) {
if (isLoggable(level)) {
if (bundle != null) {
msg = getString(bundle, msg);
}
this.scxLogger.log(level, msg, thrown);
}
}
@Override
public void log(Level level, ResourceBundle bundle, String format, Object... params) {
if (isLoggable(level)) {
if (bundle != null) {
format = getString(bundle, format);
}
var throwableCandidate = getThrowableCandidate(params);
if (throwableCandidate != null) {
params = trimmedCopy(params);
}
this.scxLogger.log(level, formatMessage(format, params), throwableCandidate);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy