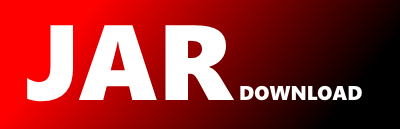
cz.active24.client.fred.data.create.nsset.NssetCreateRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fred-client Show documentation
Show all versions of fred-client Show documentation
A Java EPP client for FRED (Free Registry for ENUM and Domains)
package cz.active24.client.fred.data.create.nsset;
import cz.active24.client.fred.data.EppRequest;
import cz.active24.client.fred.data.common.nsset.NameserverData;
import cz.active24.client.fred.data.create.CreateRequest;
import cz.active24.client.fred.eppclient.objectstrategy.ServerObjectType;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.List;
/**
* A nsset create command is used to register a new nsset.
*
*
* - {@link NssetCreateRequest#id} - the nsset handle
* - {@link NssetCreateRequest#ns} - a nameserver, see {@link NameserverData}
* - {@link NssetCreateRequest#tech} - a handle of a contact that will be assigned as a technical contact
* - {@link NssetCreateRequest#authInfo} - authorization information (transfer password); if omitted, the password will be generated by the server
* - {@link NssetCreateRequest#reportLevel} - the default highest level of technical checks to be performed
*
*
* @see FRED documentation
*/
public class NssetCreateRequest extends EppRequest implements Serializable, CreateRequest {
private String id;
private List ns;
private List tech;
private String authInfo;
private Short reportLevel;
public NssetCreateRequest(String nssetId, List nameservers, List technicalContacts) {
setServerObjectType(ServerObjectType.NSSET);
this.setId(nssetId);
this.setNs(nameservers);
this.setTech(technicalContacts == null ? new ArrayList() : technicalContacts);
}
public String getId() {
return id;
}
protected void setId(String id) {
this.id = id;
}
public List getNs() {
return ns;
}
protected void setNs(List ns) {
this.ns = ns;
}
public List getTech() {
return tech;
}
protected void setTech(List tech) {
this.tech = tech;
}
public String getAuthInfo() {
return authInfo;
}
public void setAuthInfo(String authInfo) {
this.authInfo = authInfo;
}
public Short getReportLevel() {
return reportLevel;
}
public void setReportLevel(Short reportLevel) {
this.reportLevel = reportLevel;
}
@Override
public String toString() {
final StringBuffer sb = new StringBuffer("NssetCreateRequest{");
sb.append("id='").append(id).append('\'');
sb.append(", ns=").append(ns);
sb.append(", tech=").append(tech);
sb.append(", authInfo='").append(authInfo).append('\'');
sb.append(", reportLevel=").append(reportLevel);
sb.append('}');
return sb.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy