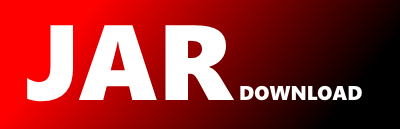
cz.active24.client.fred.data.info.keyset.KeysetInfoResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fred-client Show documentation
Show all versions of fred-client Show documentation
A Java EPP client for FRED (Free Registry for ENUM and Domains)
package cz.active24.client.fred.data.info.keyset;
import cz.active24.client.fred.data.common.keyset.DnsKeyData;
import cz.active24.client.fred.data.EppResponse;
import cz.active24.client.fred.data.info.InfoResponse;
import cz.active24.client.fred.eppclient.objectstrategy.ServerObjectType;
import java.io.Serializable;
import java.util.Date;
import java.util.List;
/**
* Keyset info response.
*
*
* - {@link KeysetInfoResponse#id} – a keyset handle
* - {@link KeysetInfoResponse#roid} – the keyset repository identifier
* - {@link KeysetInfoResponse#status} – the state name, see {@link KeysetStatusValueType}
* - {@link KeysetInfoResponse#clID} – the designated registrar’s handle
* - {@link KeysetInfoResponse#crID} – the handle of the registrar who created this keyset
* - {@link KeysetInfoResponse#crDate} – the timestamp of creation
* - {@link KeysetInfoResponse#upID} – the handle of the registrar who was the last to update this keyset
* - {@link KeysetInfoResponse#upDate} – the timestamp of the last update
* - {@link KeysetInfoResponse#trDate} – the timestamp of the last transfer
* - {@link KeysetInfoResponse#authInfo} – authorization information (transfer password)
* - {@link KeysetInfoResponse#dnskey} – a DNS key
* - {@link KeysetInfoResponse#tech} – a technical contact handle
*
*
* @see FRED documentation
*/
public class KeysetInfoResponse extends EppResponse implements Serializable, InfoResponse {
private String id;
private String roid;
private List status;
private String clID;
private String crID;
private Date crDate;
private String upID;
private Date upDate;
private Date trDate;
private String authInfo;
private List dnskey;
private List tech;
public KeysetInfoResponse() {
setServerObjectType(ServerObjectType.KEYSET);
}
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getRoid() {
return roid;
}
public void setRoid(String roid) {
this.roid = roid;
}
public List getStatus() {
return status;
}
public void setStatus(List status) {
this.status = status;
}
public String getClID() {
return clID;
}
public void setClID(String clID) {
this.clID = clID;
}
public String getCrID() {
return crID;
}
public void setCrID(String crID) {
this.crID = crID;
}
public Date getCrDate() {
return crDate;
}
public void setCrDate(Date crDate) {
this.crDate = crDate;
}
public String getUpID() {
return upID;
}
public void setUpID(String upID) {
this.upID = upID;
}
public Date getUpDate() {
return upDate;
}
public void setUpDate(Date upDate) {
this.upDate = upDate;
}
public Date getTrDate() {
return trDate;
}
public void setTrDate(Date trDate) {
this.trDate = trDate;
}
public String getAuthInfo() {
return authInfo;
}
public void setAuthInfo(String authInfo) {
this.authInfo = authInfo;
}
public List getDnskey() {
return dnskey;
}
public void setDnskey(List dnskey) {
this.dnskey = dnskey;
}
public List getTech() {
return tech;
}
public void setTech(List tech) {
this.tech = tech;
}
@Override
public String toString() {
final StringBuffer sb = new StringBuffer("KeysetInfoResponse{");
sb.append("id='").append(id).append('\'');
sb.append(", roid='").append(roid).append('\'');
sb.append(", status=").append(status);
sb.append(", clID='").append(clID).append('\'');
sb.append(", crID='").append(crID).append('\'');
sb.append(", crDate=").append(crDate);
sb.append(", upID='").append(upID).append('\'');
sb.append(", upDate=").append(upDate);
sb.append(", trDate=").append(trDate);
sb.append(", authInfo='").append(authInfo).append('\'');
sb.append(", dnskey=").append(dnskey);
sb.append(", tech=").append(tech);
sb.append(", clientTransactionId='").append(getClientTransactionId()).append('\'');
sb.append(", serverTransactionId='").append(getServerTransactionId()).append('\'');
sb.append(", result=").append(getResult());
sb.append(", serverObjectType=").append(getServerObjectType());
sb.append('}');
return sb.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy