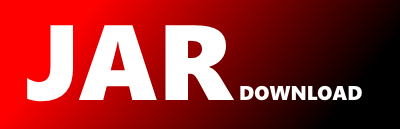
cz.nic.xml.epp.domain_1.ObjectFactory Maven / Gradle / Ivy
Show all versions of fred-client Show documentation
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.3.2
// See https://javaee.github.io/jaxb-v2/
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2021.12.21 at 12:01:05 PM CET
//
package cz.nic.xml.epp.domain_1;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.annotation.XmlElementDecl;
import javax.xml.bind.annotation.XmlRegistry;
import javax.xml.namespace.QName;
/**
* This object contains factory methods for each
* Java content interface and Java element interface
* generated in the cz.nic.xml.epp.domain_1 package.
* An ObjectFactory allows you to programatically
* construct new instances of the Java representation
* for XML content. The Java representation of XML
* content can consist of schema derived interfaces
* and classes representing the binding of schema
* type definitions, element declarations and model
* groups. Factory methods for each of these are
* provided in this class.
*
*/
@XmlRegistry
public class ObjectFactory {
private final static QName _Check_QNAME = new QName("http://www.nic.cz/xml/epp/domain-1.4", "check");
private final static QName _Create_QNAME = new QName("http://www.nic.cz/xml/epp/domain-1.4", "create");
private final static QName _Delete_QNAME = new QName("http://www.nic.cz/xml/epp/domain-1.4", "delete");
private final static QName _Info_QNAME = new QName("http://www.nic.cz/xml/epp/domain-1.4", "info");
private final static QName _Renew_QNAME = new QName("http://www.nic.cz/xml/epp/domain-1.4", "renew");
private final static QName _Transfer_QNAME = new QName("http://www.nic.cz/xml/epp/domain-1.4", "transfer");
private final static QName _Update_QNAME = new QName("http://www.nic.cz/xml/epp/domain-1.4", "update");
private final static QName _List_QNAME = new QName("http://www.nic.cz/xml/epp/domain-1.4", "list");
private final static QName _SendAuthInfo_QNAME = new QName("http://www.nic.cz/xml/epp/domain-1.4", "sendAuthInfo");
private final static QName _ChkData_QNAME = new QName("http://www.nic.cz/xml/epp/domain-1.4", "chkData");
private final static QName _CreData_QNAME = new QName("http://www.nic.cz/xml/epp/domain-1.4", "creData");
private final static QName _InfData_QNAME = new QName("http://www.nic.cz/xml/epp/domain-1.4", "infData");
private final static QName _RenData_QNAME = new QName("http://www.nic.cz/xml/epp/domain-1.4", "renData");
private final static QName _ListData_QNAME = new QName("http://www.nic.cz/xml/epp/domain-1.4", "listData");
private final static QName _TrnData_QNAME = new QName("http://www.nic.cz/xml/epp/domain-1.4", "trnData");
private final static QName _ImpendingExpData_QNAME = new QName("http://www.nic.cz/xml/epp/domain-1.4", "impendingExpData");
private final static QName _ExpData_QNAME = new QName("http://www.nic.cz/xml/epp/domain-1.4", "expData");
private final static QName _DnsOutageData_QNAME = new QName("http://www.nic.cz/xml/epp/domain-1.4", "dnsOutageData");
private final static QName _DelData_QNAME = new QName("http://www.nic.cz/xml/epp/domain-1.4", "delData");
private final static QName _UpdateData_QNAME = new QName("http://www.nic.cz/xml/epp/domain-1.4", "updateData");
/**
* Create a new ObjectFactory that can be used to create new instances of schema derived classes for package: cz.nic.xml.epp.domain_1
*
*/
public ObjectFactory() {
}
/**
* Create an instance of {@link MNameType }
*
*/
public MNameType createMNameType() {
return new MNameType();
}
/**
* Create an instance of {@link CreateType }
*
*/
public CreateType createCreateType() {
return new CreateType();
}
/**
* Create an instance of {@link SNameType }
*
*/
public SNameType createSNameType() {
return new SNameType();
}
/**
* Create an instance of {@link RenewType }
*
*/
public RenewType createRenewType() {
return new RenewType();
}
/**
* Create an instance of {@link TransferType }
*
*/
public TransferType createTransferType() {
return new TransferType();
}
/**
* Create an instance of {@link UpdateType }
*
*/
public UpdateType createUpdateType() {
return new UpdateType();
}
/**
* Create an instance of {@link SendAuthInfoType }
*
*/
public SendAuthInfoType createSendAuthInfoType() {
return new SendAuthInfoType();
}
/**
* Create an instance of {@link ChkDataType }
*
*/
public ChkDataType createChkDataType() {
return new ChkDataType();
}
/**
* Create an instance of {@link CreDataType }
*
*/
public CreDataType createCreDataType() {
return new CreDataType();
}
/**
* Create an instance of {@link InfDataType }
*
*/
public InfDataType createInfDataType() {
return new InfDataType();
}
/**
* Create an instance of {@link RenDataType }
*
*/
public RenDataType createRenDataType() {
return new RenDataType();
}
/**
* Create an instance of {@link ListDataType }
*
*/
public ListDataType createListDataType() {
return new ListDataType();
}
/**
* Create an instance of {@link HandleDateT }
*
*/
public HandleDateT createHandleDateT() {
return new HandleDateT();
}
/**
* Create an instance of {@link ImpendingExpDataT }
*
*/
public ImpendingExpDataT createImpendingExpDataT() {
return new ImpendingExpDataT();
}
/**
* Create an instance of {@link ExpDataT }
*
*/
public ExpDataT createExpDataT() {
return new ExpDataT();
}
/**
* Create an instance of {@link DnsOutageDataT }
*
*/
public DnsOutageDataT createDnsOutageDataT() {
return new DnsOutageDataT();
}
/**
* Create an instance of {@link DelDataT }
*
*/
public DelDataT createDelDataT() {
return new DelDataT();
}
/**
* Create an instance of {@link UpdateDataT }
*
*/
public UpdateDataT createUpdateDataT() {
return new UpdateDataT();
}
/**
* Create an instance of {@link PeriodType }
*
*/
public PeriodType createPeriodType() {
return new PeriodType();
}
/**
* Create an instance of {@link AddType }
*
*/
public AddType createAddType() {
return new AddType();
}
/**
* Create an instance of {@link RemType }
*
*/
public RemType createRemType() {
return new RemType();
}
/**
* Create an instance of {@link ChgType }
*
*/
public ChgType createChgType() {
return new ChgType();
}
/**
* Create an instance of {@link CheckType }
*
*/
public CheckType createCheckType() {
return new CheckType();
}
/**
* Create an instance of {@link CheckNameType }
*
*/
public CheckNameType createCheckNameType() {
return new CheckNameType();
}
/**
* Create an instance of {@link StatusType }
*
*/
public StatusType createStatusType() {
return new StatusType();
}
/**
* Create an instance of {@link OldData }
*
*/
public OldData createOldData() {
return new OldData();
}
/**
* Create an instance of {@link NewData }
*
*/
public NewData createNewData() {
return new NewData();
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link MNameType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link MNameType }{@code >}
*/
@XmlElementDecl(namespace = "http://www.nic.cz/xml/epp/domain-1.4", name = "check")
public JAXBElement createCheck(MNameType value) {
return new JAXBElement(_Check_QNAME, MNameType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link CreateType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link CreateType }{@code >}
*/
@XmlElementDecl(namespace = "http://www.nic.cz/xml/epp/domain-1.4", name = "create")
public JAXBElement createCreate(CreateType value) {
return new JAXBElement(_Create_QNAME, CreateType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link SNameType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link SNameType }{@code >}
*/
@XmlElementDecl(namespace = "http://www.nic.cz/xml/epp/domain-1.4", name = "delete")
public JAXBElement createDelete(SNameType value) {
return new JAXBElement(_Delete_QNAME, SNameType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link SNameType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link SNameType }{@code >}
*/
@XmlElementDecl(namespace = "http://www.nic.cz/xml/epp/domain-1.4", name = "info")
public JAXBElement createInfo(SNameType value) {
return new JAXBElement(_Info_QNAME, SNameType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link RenewType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link RenewType }{@code >}
*/
@XmlElementDecl(namespace = "http://www.nic.cz/xml/epp/domain-1.4", name = "renew")
public JAXBElement createRenew(RenewType value) {
return new JAXBElement(_Renew_QNAME, RenewType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link TransferType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link TransferType }{@code >}
*/
@XmlElementDecl(namespace = "http://www.nic.cz/xml/epp/domain-1.4", name = "transfer")
public JAXBElement createTransfer(TransferType value) {
return new JAXBElement(_Transfer_QNAME, TransferType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link UpdateType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link UpdateType }{@code >}
*/
@XmlElementDecl(namespace = "http://www.nic.cz/xml/epp/domain-1.4", name = "update")
public JAXBElement createUpdate(UpdateType value) {
return new JAXBElement(_Update_QNAME, UpdateType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Object }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link Object }{@code >}
*/
@XmlElementDecl(namespace = "http://www.nic.cz/xml/epp/domain-1.4", name = "list")
public JAXBElement