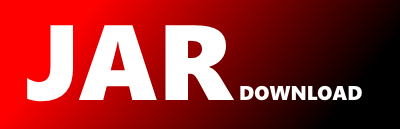
cz.encircled.joiner.query.JoinerQuery Maven / Gradle / Ivy
package cz.encircled.joiner.query;
import com.querydsl.core.types.EntityPath;
import com.querydsl.core.types.Expression;
import com.querydsl.core.types.Path;
import com.querydsl.core.types.Predicate;
import cz.encircled.joiner.query.join.JoinDescription;
import java.util.Collection;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Set;
/**
* Base interface which defines possible parameters of joiner query
* T - select from
* R - projection type
*
* @author Vlad on 04-Sep-16.
*/
public interface JoinerQuery extends JoinRoot {
EntityPath getFrom();
Expression getReturnProjection();
JoinerQueryBase where(Predicate where);
Predicate getWhere();
JoinerQueryBase distinct(boolean isDistinct);
boolean isDistinct();
JoinerQueryBase groupBy(Path> groupBy);
Path> getGroupBy();
JoinerQueryBase having(Predicate having);
Predicate getHaving();
/**
* Add join graphs to the query.
*
* @param names names of join graphs
* @return this
* @see cz.encircled.joiner.query.join.JoinGraphRegistry
*/
JoinerQueryBase joinGraphs(String... names);
/**
* Add join graphs to the query.
*
* @param names names of join graphs
* @return this
* @see cz.encircled.joiner.query.join.JoinGraphRegistry
*/
JoinerQueryBase joinGraphs(Enum... names);
/**
* Add join graphs to the query.
*
* @param names names of join graphs
* @return this
* @see cz.encircled.joiner.query.join.JoinGraphRegistry
*/
JoinerQueryBase joinGraphs(Collection> names);
Set
© 2015 - 2025 Weber Informatics LLC | Privacy Policy