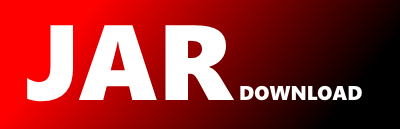
cz.geek.fio.ObjectFactory Maven / Gradle / Ivy
//
// This file was generated by the Eclipse Implementation of JAXB, v4.0.5
// See https://eclipse-ee4j.github.io/jaxb-ri
// Any modifications to this file will be lost upon recompilation of the source schema.
//
package cz.geek.fio;
import jakarta.xml.bind.annotation.XmlRegistry;
/**
* This object contains factory methods for each
* Java content interface and Java element interface
* generated in the cz.geek.fio package.
* An ObjectFactory allows you to programmatically
* construct new instances of the Java representation
* for XML content. The Java representation of XML
* content can consist of schema derived interfaces
* and classes representing the binding of schema
* type definitions, element declarations and model
* groups. Factory methods for each of these are
* provided in this class.
*
*/
@XmlRegistry
public class ObjectFactory {
/**
* Create a new ObjectFactory that can be used to create new instances of schema derived classes for package: cz.geek.fio
*
*/
public ObjectFactory() {
}
/**
* Create an instance of {@link AccountStatement }
*
* @return
* the new instance of {@link AccountStatement }
*/
public AccountStatement createAccountStatement() {
return new AccountStatement();
}
/**
* Create an instance of {@link Info }
*
* @return
* the new instance of {@link Info }
*/
public Info createInfo() {
return new Info();
}
/**
* Create an instance of {@link TransactionList }
*
* @return
* the new instance of {@link TransactionList }
*/
public TransactionList createTransactionList() {
return new TransactionList();
}
/**
* Create an instance of {@link Transaction }
*
* @return
* the new instance of {@link Transaction }
*/
public Transaction createTransaction() {
return new Transaction();
}
/**
* Create an instance of {@link Column22 }
*
* @return
* the new instance of {@link Column22 }
*/
public Column22 createColumn22() {
return new Column22();
}
/**
* Create an instance of {@link Column0 }
*
* @return
* the new instance of {@link Column0 }
*/
public Column0 createColumn0() {
return new Column0();
}
/**
* Create an instance of {@link Column1 }
*
* @return
* the new instance of {@link Column1 }
*/
public Column1 createColumn1() {
return new Column1();
}
/**
* Create an instance of {@link Column14 }
*
* @return
* the new instance of {@link Column14 }
*/
public Column14 createColumn14() {
return new Column14();
}
/**
* Create an instance of {@link Column2 }
*
* @return
* the new instance of {@link Column2 }
*/
public Column2 createColumn2() {
return new Column2();
}
/**
* Create an instance of {@link Column10 }
*
* @return
* the new instance of {@link Column10 }
*/
public Column10 createColumn10() {
return new Column10();
}
/**
* Create an instance of {@link Column3 }
*
* @return
* the new instance of {@link Column3 }
*/
public Column3 createColumn3() {
return new Column3();
}
/**
* Create an instance of {@link Column12 }
*
* @return
* the new instance of {@link Column12 }
*/
public Column12 createColumn12() {
return new Column12();
}
/**
* Create an instance of {@link Column4 }
*
* @return
* the new instance of {@link Column4 }
*/
public Column4 createColumn4() {
return new Column4();
}
/**
* Create an instance of {@link Column5 }
*
* @return
* the new instance of {@link Column5 }
*/
public Column5 createColumn5() {
return new Column5();
}
/**
* Create an instance of {@link Column6 }
*
* @return
* the new instance of {@link Column6 }
*/
public Column6 createColumn6() {
return new Column6();
}
/**
* Create an instance of {@link Column7 }
*
* @return
* the new instance of {@link Column7 }
*/
public Column7 createColumn7() {
return new Column7();
}
/**
* Create an instance of {@link Column16 }
*
* @return
* the new instance of {@link Column16 }
*/
public Column16 createColumn16() {
return new Column16();
}
/**
* Create an instance of {@link Column8 }
*
* @return
* the new instance of {@link Column8 }
*/
public Column8 createColumn8() {
return new Column8();
}
/**
* Create an instance of {@link Column9 }
*
* @return
* the new instance of {@link Column9 }
*/
public Column9 createColumn9() {
return new Column9();
}
/**
* Create an instance of {@link Column18 }
*
* @return
* the new instance of {@link Column18 }
*/
public Column18 createColumn18() {
return new Column18();
}
/**
* Create an instance of {@link Column25 }
*
* @return
* the new instance of {@link Column25 }
*/
public Column25 createColumn25() {
return new Column25();
}
/**
* Create an instance of {@link Column26 }
*
* @return
* the new instance of {@link Column26 }
*/
public Column26 createColumn26() {
return new Column26();
}
/**
* Create an instance of {@link Column17 }
*
* @return
* the new instance of {@link Column17 }
*/
public Column17 createColumn17() {
return new Column17();
}
}