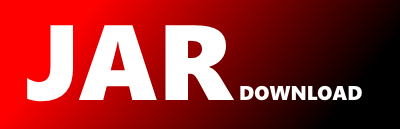
cz.mmsparams.api.utils.Preconditions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of MmsParamsAPI Show documentation
Show all versions of MmsParamsAPI Show documentation
Common library for MmsParams system
The newest version!
package cz.mmsparams.api.utils;
import java.util.List;
import java.util.Optional;
import cz.mmsparams.api.exceptions.TestInvalidException;
public class Preconditions
{
private Preconditions()
{
}
public static T checkNotNull(final T obj, final String msg)
{
if (obj == null)
throw new TestInvalidException(msg + " is null!");
return obj;
}
public static String checkNotNullOrEmpty(final String text, final String msg)
{
if (StringUtil.isEmptyOrNull(text))
throw new TestInvalidException(msg + " is null or empty!");
return text;
}
public static List checkNotNullOrEmpty(final List text, final String msg)
{
checkNotNull(text, msg);
for (String t : text)
if (StringUtil.isEmptyOrNull(t))
throw new TestInvalidException(msg);
return text;
}
public static long isGreater(final long value, final int minValue, final String msg)
{
if (value <= minValue)
throw new TestInvalidException(msg);
return value;
}
public static long isGreaterZero(final long value, final String msg)
{
return isGreater(value, 0, msg);
}
public static int isGreater(final int value, final int minValue, final String msg)
{
if (value <= minValue)
throw new TestInvalidException(msg);
return value;
}
public static int isGreaterZero(final int value, final String msg)
{
return isGreater(value, 0, msg);
}
public static int isGreaterZeroOrDefault(final int value, final String msg)
{
if (value <= 0 && value != -1)
{
throw new TestInvalidException(msg);
}
return value;
}
public static int isEqual(final int value, final int expected, final String msg)
{
if (value != expected)
throw new TestInvalidException(msg);
return value;
}
public static boolean checkTrue(boolean value, String msg)
{
if (!value)
throw new TestInvalidException(msg);
return value;
}
public static T checkOptionalFilled(final Optional optional, String msg)
{
if (!optional.isPresent())
throw new TestInvalidException(msg);
return optional.get();
}
public static String checkStringLenghtMax(String value, int maxLenght, String msg)
{
if (StringUtil.isEmptyOrNull(value))
return value;
if (value.length() >= maxLenght)
{
throw new TestInvalidException(msg);
}
return value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy