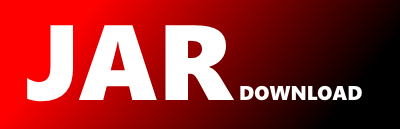
cz.mmsparams.api.websocket.model.mms.MmsDownloadOptionsModel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of MmsParamsAPI Show documentation
Show all versions of MmsParamsAPI Show documentation
Common library for MmsParams system
The newest version!
package cz.mmsparams.api.websocket.model.mms;
import java.io.Serializable;
import cz.mmsparams.api.utils.Preconditions;
import cz.mmsparams.api.websocket.WebSocketModelBase;
/**
* Class for configuring recipient device how to response to new MMS message in terms of downloading from MMSC
*/
public class MmsDownloadOptionsModel extends WebSocketModelBase implements Serializable
{
private boolean autoDownload;
private int downloadAfterSeconds;
/**
* Create recipient profile when MMS will be downloaded right after receiving
*
* @return Recipient MMS download profile
*/
public static MmsDownloadOptionsModel createAutoDownload()
{
MmsDownloadOptionsModel opt = new MmsDownloadOptionsModel();
opt.setAutoDownload(true);
opt.setDownloadAfterSeconds(0);
return opt;
}
/**
* Create recipient profile when MMS will be downloaded after certain delay
*
* @param downloadAfterSeconds Delay before downloading message in seconds
* @return Recipient MMS download profile
*/
public static MmsDownloadOptionsModel createManualDownload(int downloadAfterSeconds)
{
Preconditions.isGreaterZero(downloadAfterSeconds, "downloadAfterSeconds");
MmsDownloadOptionsModel opt = new MmsDownloadOptionsModel();
opt.setAutoDownload(false);
opt.setDownloadAfterSeconds(downloadAfterSeconds);
return opt;
}
/**
* Create recipient profile when MMS will never be downloaded
*
* @return Recipient MMS download profile
*/
public static MmsDownloadOptionsModel createNeverDownload()
{
MmsDownloadOptionsModel opt = new MmsDownloadOptionsModel();
opt.setAutoDownload(false);
opt.setDownloadAfterSeconds(-1);
return opt;
}
/**
* USE static create methods instead
*/
@Deprecated
public MmsDownloadOptionsModel()
{
}
public boolean isAutoDownload()
{
return autoDownload;
}
public void setAutoDownload(boolean autoDownload)
{
this.autoDownload = autoDownload;
}
public int getDownloadAfterSeconds()
{
return downloadAfterSeconds;
}
public void setDownloadAfterSeconds(int downloadAfterSeconds)
{
this.downloadAfterSeconds = downloadAfterSeconds;
}
@Override
public String toString()
{
return "MmsDownloadOptionsModel{" +
"autoDownload=" + autoDownload +
", downloadAfterSeconds=" + downloadAfterSeconds +
"} " + super.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy