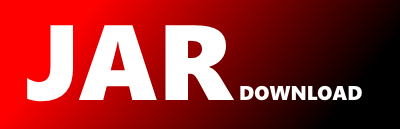
cz.mmsparams.api.websocket.model.mms.MmsReadReportOptionsModel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of MmsParamsAPI Show documentation
Show all versions of MmsParamsAPI Show documentation
Common library for MmsParams system
The newest version!
package cz.mmsparams.api.websocket.model.mms;
import java.io.Serializable;
import javax.annotation.Nonnull;
import cz.mmsparams.api.constants.GenericConstants;
import cz.mmsparams.api.enums.ReadStatus;
import cz.mmsparams.api.utils.Preconditions;
import cz.mmsparams.api.websocket.WebSocketModelBase;
/**
* Class for configuring recipient device how to response to new MMS message in terms of sending read report
*/
public class MmsReadReportOptionsModel extends WebSocketModelBase implements Serializable
{
private boolean sendReadReport;
private int sendAfterSeconds;
private ReadStatus readStatus;
/**
* Create recipient profile when MMS delivery report will never be sent
*
* @return Recipient MMS read report profile
*/
@Nonnull
public static MmsReadReportOptionsModel createNoReadReport()
{
MmsReadReportOptionsModel opt = new MmsReadReportOptionsModel();
opt.setSendReadReport(false);
opt.setSendAfterSeconds(0);
opt.setReadStatus(ReadStatus.RAED);
return opt;
}
/**
* Create recipient profile when MMS read report will be sent after certain detail with specific ReadStatus
*
* @param sendAfterSeconds Delay before sending read report in seconds
* @param readStatus Read status to be set to ReadReport PDU
* @return Recipient MMS read report profile
*/
@Nonnull
public static MmsReadReportOptionsModel createNoReadReport(int sendAfterSeconds, ReadStatus readStatus)
{
Preconditions.isGreaterZero(sendAfterSeconds, GenericConstants.SEND_AFTER_SECONDS);
Preconditions.checkNotNull(readStatus, GenericConstants.READ_STATUS);
MmsReadReportOptionsModel opt = new MmsReadReportOptionsModel();
opt.setSendReadReport(true);
opt.setSendAfterSeconds(sendAfterSeconds);
opt.setReadStatus(readStatus);
return opt;
}
/**
* USE static create methods instead
*/
@Deprecated
public MmsReadReportOptionsModel()
{
}
public boolean isSendReadReport()
{
return sendReadReport;
}
public void setSendReadReport(boolean sendReadReport)
{
this.sendReadReport = sendReadReport;
}
public ReadStatus getReadStatus()
{
return readStatus;
}
public void setReadStatus(ReadStatus readStatus)
{
this.readStatus = readStatus;
}
public int getSendAfterSeconds()
{
return sendAfterSeconds;
}
public void setSendAfterSeconds(int sendAfterSeconds)
{
this.sendAfterSeconds = sendAfterSeconds;
}
@Override
public String toString()
{
return "MmsReadReportOptionsModel{" +
"sendReadReport=" + sendReadReport +
", sendAfterSeconds=" + sendAfterSeconds +
", readStatus=" + readStatus +
"} " + super.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy