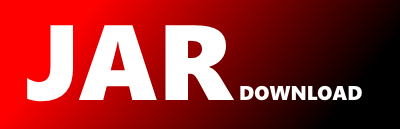
cz.mmsparams.api.websocket.model.mms.MmsRecipientPhoneProfile Maven / Gradle / Ivy
package cz.mmsparams.api.websocket.model.mms;
import java.io.Serializable;
import cz.mmsparams.api.websocket.WebSocketModelBase;
/**
* Class for configuring recipient device how to response
* to new MMS message in terms of downloading from MMSC and sending ReadReport
*/
public class MmsRecipientPhoneProfile extends WebSocketModelBase implements Serializable
{
private MmsDownloadOptionsModel mmsDownloadOptionsModel;
private MmsReadReportOptionsModel mmsReadReportOptionsModel;
private boolean allowDeliveryReport;
/**
* Create default MMS recipient profile,
* when message will be downloaded immediately and read report will not be sent
*
* @return created MMS recipient profile
*/
public static MmsRecipientPhoneProfile createDefault()
{
MmsRecipientPhoneProfile profile = new MmsRecipientPhoneProfile();
profile.setMmsDownloadOptionsModel(MmsDownloadOptionsModel.createAutoDownload());
profile.setMmsReadReportOptionsModel(MmsReadReportOptionsModel.createNoReadReport());
profile.setAllowDeliveryReport(true);
return profile;
}
/**
* Create MMS recipient profile
* @param downloadOptions options, when to download received MMS message
* @param readReportOptions option whether send MMS Read Report
* @param allowDeliveryReport option whether allow delivery report (it is set to PDU)
* @return created MMS recipient profile
*/
public static MmsRecipientPhoneProfile create(MmsDownloadOptionsModel downloadOptions,
MmsReadReportOptionsModel readReportOptions,
boolean allowDeliveryReport)
{
MmsRecipientPhoneProfile profile = new MmsRecipientPhoneProfile();
profile.setMmsDownloadOptionsModel(downloadOptions);
profile.setMmsReadReportOptionsModel(readReportOptions);
profile.setAllowDeliveryReport(allowDeliveryReport);
return profile;
}
/**
* USE static create methods instead
*/
public MmsRecipientPhoneProfile()
{
}
public MmsDownloadOptionsModel getMmsDownloadOptionsModel()
{
return mmsDownloadOptionsModel;
}
public void setMmsDownloadOptionsModel(MmsDownloadOptionsModel mmsDownloadOptionsModel)
{
this.mmsDownloadOptionsModel = mmsDownloadOptionsModel;
}
public boolean isAllowDeliveryReport()
{
return allowDeliveryReport;
}
public void setAllowDeliveryReport(boolean allowDeliveryReport)
{
this.allowDeliveryReport = allowDeliveryReport;
}
public MmsReadReportOptionsModel getMmsReadReportOptionsModel()
{
return mmsReadReportOptionsModel;
}
public void setMmsReadReportOptionsModel(MmsReadReportOptionsModel mmsReadReportOptionsModel)
{
this.mmsReadReportOptionsModel = mmsReadReportOptionsModel;
}
@Override
public String toString()
{
return "MmsRecipientPhoneProfile{" +
"mmsDownloadOptionsModel=" + mmsDownloadOptionsModel +
", mmsReadReportOptionsModel=" + mmsReadReportOptionsModel +
", allowDeliveryReport=" + allowDeliveryReport +
"} " + super.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy