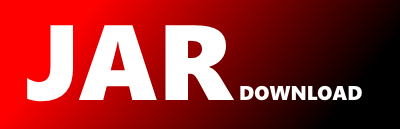
cz.mmsparams.api.websocket.model.mms.MmsSendModel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of MmsParamsAPI Show documentation
Show all versions of MmsParamsAPI Show documentation
Common library for MmsParams system
The newest version!
package cz.mmsparams.api.websocket.model.mms;
import java.io.Serializable;
import java.util.ArrayList;
import cz.mmsparams.api.constants.GenericConstants;
import cz.mmsparams.api.enums.MessageClass;
import cz.mmsparams.api.enums.Priority;
import cz.mmsparams.api.utils.MmsUtils;
import cz.mmsparams.api.utils.Preconditions;
import cz.mmsparams.api.websocket.WebSocketModelBase;
public class MmsSendModel extends WebSocketModelBase implements Serializable
{
private ArrayList to;
private ArrayList bcc;
private ArrayList cc;
private String text;
private ArrayList mmsAttachmentSendModel;
private boolean deliveryReport;
private boolean readReport;
private Long expiry; // seconds
private Long deliveryTime; // seconds
private Priority priority;
private MessageClass msgClass;
private boolean senderVisible;
public MmsSendModel()
{
MmsUtils.setDefaultTestProfile(this);
}
/**
* Create default MMS message. Read Report is false.
*
* @param numberTo recipient number (To)
* @param mmsText MMS message text
* @param deliveryReport require delivery report
* @return new MMS message
*/
public static MmsSendModel createDefault(final String numberTo, final String mmsText, final boolean deliveryReport)
{
Preconditions.checkNotNullOrEmpty(numberTo, GenericConstants.TO);
MmsSendModel mms = new MmsSendModel();
mms.addTo(numberTo);
mms.setText(mmsText);
MmsUtils.setDefaultTestProfile(mms);
mms.setDeliveryReport(deliveryReport);
mms.setReadReport(false);
return mms;
}
/**
* Get list of TO recipients
*
* @return recipients to
*/
public ArrayList getTo()
{
return to;
}
/**
* Set list of To recipients
*
* @param to recipients to
*/
public void setTo(ArrayList to)
{
this.to = to;
}
/**
* Get list of Bcc recipients
*
* @return recipients Bcc
*/
public ArrayList getBcc()
{
return bcc;
}
public void setBcc(ArrayList bcc)
{
this.bcc = bcc;
}
/**
* Get list of Cc recipients
*
* @return recipients Cc
*/
public ArrayList getCc()
{
return cc;
}
public void setCc(ArrayList cc)
{
this.cc = cc;
}
/**
* Get value whether delivery report is required
*
* @return true if require delivery report
*/
public boolean isDeliveryReport()
{
return deliveryReport;
}
/**
* Get value whether read report is required
*
* @return true if require read report
*/
public boolean isReadReport()
{
return readReport;
}
/**
* Get MMS text
*
* @return MMS text
*/
public String getText()
{
return text;
}
public void setText(String text)
{
this.text = text;
}
/**
* Get list of MMS attachments
*
* @return list of attachments
*/
public ArrayList getMmsAttachmentSendModel()
{
return mmsAttachmentSendModel;
}
public void setMmsAttachmentSendModel(ArrayList mmsAttachmentSendModel)
{
this.mmsAttachmentSendModel = mmsAttachmentSendModel;
}
/**
* Set whether delivery report is required
*
* @param deliveryReport require delivery report
*/
public void setDeliveryReport(boolean deliveryReport)
{
this.deliveryReport = deliveryReport;
}
/**
* Set whether read report is required
*
* @param readReport require read report
*/
public void setReadReport(boolean readReport)
{
this.readReport = readReport;
}
/**
* Get MMS expiry time in seconds
*
* @return message expiry
*/
public Long getExpiry()
{
return expiry;
}
/**
* Set MMS expiry time in seconds
*
* @param expiry seconds
*/
public void setExpiry(Long expiry)
{
this.expiry = expiry;
}
/**
* Get MMS earlies delivery time in seconds
*
* @return message delivery time
*/
public Long getDeliveryTime()
{
return deliveryTime;
}
/**
* Set MMS earlies delivery time in seconds
*
* @param deliveryTime seconds
*/
public void setDeliveryTime(Long deliveryTime)
{
this.deliveryTime = deliveryTime;
}
/**
* Get MMS priority
*
* @return message priority
*/
public Priority getPriority()
{
return priority;
}
/**
* Set MMS priority
*
* @param priority priority of MMS
*/
public void setPriority(Priority priority)
{
this.priority = priority;
}
/**
* Get MMS class
*
* @return message class
*/
public MessageClass getMsgClass()
{
return msgClass;
}
/**
* Set MMS class
*
* @param msgClass message class to set
*/
public void setMsgClass(MessageClass msgClass)
{
this.msgClass = msgClass;
}
/**
* Get MMS sender visibility (hide sender)
*
* @return true if sender is visible
*/
public boolean isSenderVisible()
{
return senderVisible;
}
/**
* Set MMS sender visibility
*
* @param senderVisible sender visibility value (true if sender is visible)
*/
public void setSenderVisible(boolean senderVisible)
{
this.senderVisible = senderVisible;
}
/**
* Add attachment to MMS message
*
* @param attachment attachment to add
*/
public void addAttachment(final MmsAttachmentSendModel attachment)
{
if (mmsAttachmentSendModel == null)
{
mmsAttachmentSendModel = new ArrayList<>();
}
mmsAttachmentSendModel.add(attachment);
}
/**
* Add TO recipient
*
* @param recipientTo number
*/
public void addTo(final String recipientTo)
{
if (to == null)
{
to = new ArrayList<>();
}
to.add(recipientTo);
}
/**
* Add Bcc recipient
*
* @param recipientBcc number
*/
public void addBcc(final String recipientBcc)
{
if (bcc == null)
{
bcc = new ArrayList<>();
}
bcc.add(recipientBcc);
}
/**
* Add Cc recipient
*
* @param recipientCc number
*/
public void addCc(final String recipientCc)
{
if (cc == null)
{
cc = new ArrayList<>();
}
cc.add(recipientCc);
}
@Override
public String toString()
{
return "MmsSendModel{" +
"to=" + to +
", bcc=" + bcc +
", cc=" + cc +
", text='" + text + '\'' +
", mmsAttachmentSendModel=" + mmsAttachmentSendModel +
", deliveryReport=" + deliveryReport +
", readReport=" + readReport +
", expiry=" + expiry +
", deliveryTime=" + deliveryTime +
", priority=" + priority +
", msgClass=" + msgClass +
", senderVisible=" + senderVisible +
"} " + super.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy