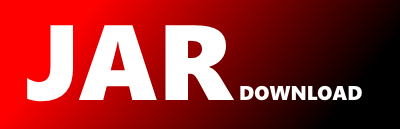
cz.mmsparams.api.websocket.model.mms.pdus.NotificationIndModel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of MmsParamsAPI Show documentation
Show all versions of MmsParamsAPI Show documentation
Common library for MmsParams system
The newest version!
package cz.mmsparams.api.websocket.model.mms.pdus;
import java.io.Serializable;
import java.util.Arrays;
import cz.mmsparams.api.websocket.WebSocketModelBase;
/**
* Class representing MM1_notification.REQ PDU
*/
public class NotificationIndModel extends WebSocketModelBase implements Serializable
{
private String id;
private String transactionId;
private int contentClass;
private String contentLocation;
private Long expiry;
private String messageClass;
private Long msgSize;
private int deliveryReport;
private String subject;
private int mmsVersion;
private String from;
private int stored;
private byte[] applicId;
private byte[] auxApplicId;
private int distributionIndicator;
private int priority;
private int recommendedRetrievalMode;
private int recommendedRetrievalModeText;
private byte[] replaceId;
private int replyCharging;
private int replyChargingDeadline;
private byte[] replyChargingId;
private long replyChargingSize;
public NotificationIndModel()
{
}
public NotificationIndModel(String id, String transactionId, int contentClass, String contentLocation, Long expiry, String messageClass, Long msgSize, int deliveryReport, String subject, int mmsVersion, String from)
{
this.id = id;
this.transactionId = transactionId;
this.contentClass = contentClass;
this.contentLocation = contentLocation;
this.expiry = expiry;
this.messageClass = messageClass;
this.msgSize = msgSize;
this.deliveryReport = deliveryReport;
this.subject = subject;
this.mmsVersion = mmsVersion;
this.from = from;
}
public String getId()
{
return id;
}
public void setId(String id)
{
this.id = id;
}
public String getTransactionId()
{
return transactionId;
}
public void setTransactionId(String transactionId)
{
this.transactionId = transactionId;
}
public int getContentClass()
{
return contentClass;
}
public void setContentClass(int contentClass)
{
this.contentClass = contentClass;
}
public String getContentLocation()
{
return contentLocation;
}
public void setContentLocation(String contentLocation)
{
this.contentLocation = contentLocation;
}
public Long getExpiry()
{
return expiry;
}
public void setExpiry(Long expiry)
{
this.expiry = expiry;
}
public String getMessageClass()
{
return messageClass;
}
public void setMessageClass(String messageClass)
{
this.messageClass = messageClass;
}
public Long getMsgSize()
{
return msgSize;
}
public void setMsgSize(Long msgSize)
{
this.msgSize = msgSize;
}
public int getDeliveryReport()
{
return deliveryReport;
}
public void setDeliveryReport(int deliveryReport)
{
this.deliveryReport = deliveryReport;
}
public String getSubject()
{
return subject;
}
public void setSubject(String subject)
{
this.subject = subject;
}
public int getMmsVersion()
{
return mmsVersion;
}
public void setMmsVersion(int mmsVersion)
{
this.mmsVersion = mmsVersion;
}
public String getFrom()
{
return from;
}
public void setFrom(String from)
{
this.from = from;
}
public void setApplicId(byte[] applicId)
{
this.applicId = applicId;
}
public void setAuxApplicId(byte[] auxApplicId)
{
this.auxApplicId = auxApplicId;
}
public void setDistributionIndicator(int distributionIndicator)
{
this.distributionIndicator = distributionIndicator;
}
public void setPriority(int priority)
{
this.priority = priority;
}
public void setRecommendedRetrievalMode(int recommendedRetrievalMode)
{
this.recommendedRetrievalMode = recommendedRetrievalMode;
}
public void setRecommendedRetrievalModeText(int recommendedRetrievalModeText)
{
this.recommendedRetrievalModeText = recommendedRetrievalModeText;
}
public void setReplaceId(byte[] replaceId)
{
this.replaceId = replaceId;
}
public void setReplyCharging(int replyCharging)
{
this.replyCharging = replyCharging;
}
public void setReplyChargingDeadline(int replyChargingDeadline)
{
this.replyChargingDeadline = replyChargingDeadline;
}
public void setReplyChargingId(byte[] replyChargingId)
{
this.replyChargingId = replyChargingId;
}
public void setReplyChargingSize(long replyChargingSize)
{
this.replyChargingSize = replyChargingSize;
}
public void setStored(int stored)
{
this.stored = stored;
}
public int getStored()
{
return stored;
}
public byte[] getApplicId()
{
return applicId;
}
public byte[] getAuxApplicId()
{
return auxApplicId;
}
public int getDistributionIndicator()
{
return distributionIndicator;
}
public int getPriority()
{
return priority;
}
public int getRecommendedRetrievalMode()
{
return recommendedRetrievalMode;
}
public int getRecommendedRetrievalModeText()
{
return recommendedRetrievalModeText;
}
public byte[] getReplaceId()
{
return replaceId;
}
public int getReplyCharging()
{
return replyCharging;
}
public int getReplyChargingDeadline()
{
return replyChargingDeadline;
}
public byte[] getReplyChargingId()
{
return replyChargingId;
}
public long getReplyChargingSize()
{
return replyChargingSize;
}
@Override
public String toString()
{
return "NotificationIndModel{" +
"id='" + id + '\'' +
", transactionId='" + transactionId + '\'' +
", contentClass=" + contentClass +
", contentLocation='" + contentLocation + '\'' +
", expiry=" + expiry +
", messageClass='" + messageClass + '\'' +
", msgSize=" + msgSize +
", deliveryReport=" + deliveryReport +
", subject='" + subject + '\'' +
", mmsVersion=" + mmsVersion +
", from='" + from + '\'' +
", stored=" + stored +
", applicId=" + Arrays.toString(applicId) +
", auxApplicId=" + Arrays.toString(auxApplicId) +
", distributionIndicator=" + distributionIndicator +
", priority=" + priority +
", recommendedRetrievalMode=" + recommendedRetrievalMode +
", recommendedRetrievalModeText=" + recommendedRetrievalModeText +
", replaceId=" + Arrays.toString(replaceId) +
", replyCharging=" + replyCharging +
", replyChargingDeadline=" + replyChargingDeadline +
", replyChargingId=" + Arrays.toString(replyChargingId) +
", replyChargingSize=" + replyChargingSize +
'}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy