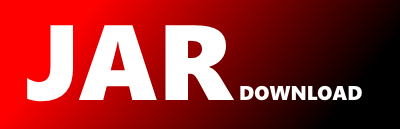
cz.mmsparams.api.websocket.model.mms.pdus.ReadOrigIndModel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of MmsParamsAPI Show documentation
Show all versions of MmsParamsAPI Show documentation
Common library for MmsParams system
The newest version!
package cz.mmsparams.api.websocket.model.mms.pdus;
import java.io.Serializable;
import java.util.Arrays;
import cz.mmsparams.api.websocket.WebSocketModelBase;
/**
* Class representing MM1_read_reply_originator.REQ PDU
*/
public class ReadOrigIndModel extends WebSocketModelBase implements Serializable
{
private String id;
private Long date;
private String messageId;
private int readStatus;
private int mmsVersion;
private String from;
private String[] to;
private byte[] replyApplicId;
private byte[] applicId;
private byte[] auxApplicId;
public ReadOrigIndModel()
{
}
public ReadOrigIndModel(String id, Long date, String messageId, int readStatus, int mmsVersion, String from, String[] to)
{
this.id = id;
this.date = date;
this.messageId = messageId;
this.readStatus = readStatus;
this.mmsVersion = mmsVersion;
this.from = from;
this.to = to;
}
public String getId()
{
return id;
}
public void setId(String id)
{
this.id = id;
}
public Long getDate()
{
return date;
}
public void setDate(Long date)
{
this.date = date;
}
public String getMessageId()
{
return messageId;
}
public void setMessageId(String messageId)
{
this.messageId = messageId;
}
public int getReadStatus()
{
return readStatus;
}
public void setReadStatus(int readStatus)
{
this.readStatus = readStatus;
}
public int getMmsVersion()
{
return mmsVersion;
}
public void setMmsVersion(int mmsVersion)
{
this.mmsVersion = mmsVersion;
}
public String getFrom()
{
return from;
}
public void setFrom(String from)
{
this.from = from;
}
public String[] getTo()
{
return to;
}
public void setTo(String[] to)
{
this.to = to;
}
public void setApplicId(byte[] applicId)
{
this.applicId = applicId;
}
public void setAuxApplicId(byte[] auxApplicId)
{
this.auxApplicId = auxApplicId;
}
public void setReplyApplicId(byte[] replyApplicId)
{
this.replyApplicId = replyApplicId;
}
public byte[] getReplyApplicId()
{
return replyApplicId;
}
public byte[] getApplicId()
{
return applicId;
}
public byte[] getAuxApplicId()
{
return auxApplicId;
}
@Override
public String toString()
{
return "ReadOrigIndModel{" +
"id='" + id + '\'' +
", date=" + date +
", messageId='" + messageId + '\'' +
", readStatus=" + readStatus +
", mmsVersion=" + mmsVersion +
", from='" + from + '\'' +
", to=" + Arrays.toString(to) +
", replyApplicId=" + Arrays.toString(replyApplicId) +
", applicId=" + Arrays.toString(applicId) +
", auxApplicId=" + Arrays.toString(auxApplicId) +
'}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy