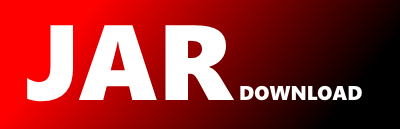
cz.mmsparams.api.websocket.model.phone.PhoneInfoModel Maven / Gradle / Ivy
package cz.mmsparams.api.websocket.model.phone;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Objects;
import cz.mmsparams.api.websocket.WebSocketModelBase;
public class PhoneInfoModel extends WebSocketModelBase implements Serializable
{
private String serial;
private String model;
private String id;
private String manufacturer;
private String brand;
private String user;
private String type;
private String versionIncremental;
private String versionSdk;
private String board;
private String host;
private String fingerprint;
private String release;
private int codesBase;
private int versionSdkInt;
private String phoneKey;
private SubscriptionInfoModel[] subscriptionInfoModel;
private String phoneNumber;
private String phoneCustomName;
private String imsi;
/**
* @deprecated Are you sure?
*/
@Deprecated
public PhoneInfoModel()
{
}
/**
* @deprecated Are you sure?
*/
@Deprecated
public PhoneInfoModel(String serial, String model, String id, String manufacturer,
String brand, String user, String type, String versionIncremental,
String versionSdk, String board, String host,
String fingerprint, String release, int codesBase,
int versionSdkInt, String phoneKey,
SubscriptionInfoModel[] subscriptionInfoModel, String phoneNumber)
{
this.serial = serial;
this.model = model;
this.id = id;
this.manufacturer = manufacturer;
this.brand = brand;
this.user = user;
this.type = type;
this.versionIncremental = versionIncremental;
this.versionSdk = versionSdk;
this.board = board;
this.host = host;
this.fingerprint = fingerprint;
this.release = release;
this.codesBase = codesBase;
this.versionSdkInt = versionSdkInt;
this.phoneKey = phoneKey;
this.subscriptionInfoModel = subscriptionInfoModel;
this.phoneNumber = phoneNumber;
}
public String getSerial()
{
return serial;
}
public void setSerial(String serial)
{
this.serial = serial;
}
public String getModel()
{
return model;
}
public void setModel(String model)
{
this.model = model;
}
public String getId()
{
return id;
}
public void setId(String id)
{
this.id = id;
}
public String getManufacturer()
{
return manufacturer;
}
public void setManufacturer(String manufacturer)
{
this.manufacturer = manufacturer;
}
public String getBrand()
{
return brand;
}
public void setBrand(String brand)
{
this.brand = brand;
}
public String getUser()
{
return user;
}
public void setUser(String user)
{
this.user = user;
}
public String getType()
{
return type;
}
public void setType(String type)
{
this.type = type;
}
public String getVersionIncremental()
{
return versionIncremental;
}
public void setVersionIncremental(String versionIncremental)
{
this.versionIncremental = versionIncremental;
}
public String getVersionSdk()
{
return versionSdk;
}
public void setVersionSdk(String versionSdk)
{
this.versionSdk = versionSdk;
}
public String getBoard()
{
return board;
}
public void setBoard(String board)
{
this.board = board;
}
public String getHost()
{
return host;
}
public void setHost(String host)
{
this.host = host;
}
public String getFingerprint()
{
return fingerprint;
}
public void setFingerprint(String fingerprint)
{
this.fingerprint = fingerprint;
}
public String getRelease()
{
return release;
}
public void setRelease(String release)
{
this.release = release;
}
public int getCodesBase()
{
return codesBase;
}
public void setCodesBase(int codesBase)
{
this.codesBase = codesBase;
}
public int getVersionSdkInt()
{
return versionSdkInt;
}
public void setVersionSdkInt(int versionSdkInt)
{
this.versionSdkInt = versionSdkInt;
}
public String getPhoneKey()
{
return phoneKey;
}
public void setPhoneKey(String phoneKey)
{
this.phoneKey = phoneKey;
}
public SubscriptionInfoModel[] getSubscriptionInfoModel()
{
return subscriptionInfoModel;
}
public void setSubscriptionInfoModel(SubscriptionInfoModel[] subscriptionInfoModel)
{
this.subscriptionInfoModel = subscriptionInfoModel;
}
public String getPhoneNumber()
{
return phoneNumber;
}
public void setPhoneNumber(String phoneNumber)
{
this.phoneNumber = phoneNumber;
}
public void setImsi(String imsi)
{
this.imsi = imsi;
}
public String getImsi()
{
return imsi;
}
public String getPhoneCustomName()
{
return phoneCustomName;
}
public void setPhoneCustomName(String phoneCustomName)
{
this.phoneCustomName = phoneCustomName;
}
@Override
public boolean equals(Object o)
{
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
PhoneInfoModel that = (PhoneInfoModel) o;
return codesBase == that.codesBase &&
versionSdkInt == that.versionSdkInt &&
Objects.equals(serial, that.serial) &&
Objects.equals(model, that.model) &&
Objects.equals(id, that.id) &&
Objects.equals(manufacturer, that.manufacturer) &&
Objects.equals(brand, that.brand) &&
Objects.equals(user, that.user) &&
Objects.equals(type, that.type) &&
Objects.equals(versionIncremental, that.versionIncremental) &&
Objects.equals(versionSdk, that.versionSdk) &&
Objects.equals(board, that.board) &&
Objects.equals(host, that.host) &&
Objects.equals(fingerprint, that.fingerprint) &&
Objects.equals(release, that.release) &&
Objects.equals(phoneKey, that.phoneKey) &&
Arrays.equals(subscriptionInfoModel, that.subscriptionInfoModel) &&
Objects.equals(phoneNumber, that.phoneNumber) &&
Objects.equals(phoneCustomName, that.phoneCustomName) &&
Objects.equals(imsi, that.imsi);
}
@Override
public int hashCode()
{
int result = Objects.hash(serial, model, id, manufacturer, brand, user, type, versionIncremental, versionSdk, board, host, fingerprint, release, codesBase, versionSdkInt, phoneKey, phoneNumber, phoneCustomName, imsi);
result = 31 * result + Arrays.hashCode(subscriptionInfoModel);
return result;
}
@Override
public String toString()
{
return "DeviceInfo{" +
"serial='" + serial + '\'' +
", model='" + model + '\'' +
", id='" + id + '\'' +
", manufacturer='" + manufacturer + '\'' +
", brand='" + brand + '\'' +
", user='" + user + '\'' +
", type='" + type + '\'' +
", versionIncremental='" + versionIncremental + '\'' +
", versionSdk='" + versionSdk + '\'' +
", board='" + board + '\'' +
", host='" + host + '\'' +
", fingerprint='" + fingerprint + '\'' +
", release='" + release + '\'' +
", codesBase=" + codesBase +
", versionSdkInt=" + versionSdkInt +
", phoneKey='" + phoneKey + '\'' +
", subscriptionInfoModel=" + Arrays.toString(subscriptionInfoModel) +
", phoneNumber='" + phoneNumber + '\'' +
", phoneCustomName='" + phoneCustomName + '\'' +
", imsi='" + imsi + '\'' +
"} " + super.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy