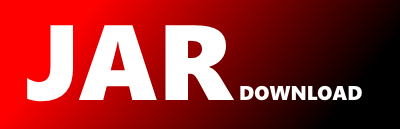
cz.mmsparams.api.websocket.model.sms.SmsReceiveModel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of MmsParamsAPI Show documentation
Show all versions of MmsParamsAPI Show documentation
Common library for MmsParams system
The newest version!
package cz.mmsparams.api.websocket.model.sms;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Arrays;
import cz.mmsparams.api.enums.SmsClass;
import cz.mmsparams.api.websocket.WebSocketModelBase;
public class SmsReceiveModel extends WebSocketModelBase implements Serializable
{
private String serviceCenterAddress;
private String originatingAddress;
private String displayOriginatingAddress;
private String messageBody;
private ArrayList displayMessageBody;
private String pseudoSubject;
private long timestampMillis;
private boolean email;
private String emailBody;
private String emailFrom;
private int protocolIdentifier;
private boolean replace;
private boolean cphsMwiMessage;
private boolean MWIClearMessage;
private boolean MWISetMessage;
private boolean mwiDontStore;
private byte[] userData;
private int statusOnIcc;
private int indexOnIcc;
private int status;
private boolean statusReportMessage;
private boolean replyPathPresent;
private SmsClass messageClass;
public void setServiceCenterAddress(String serviceCenterAddress)
{
this.serviceCenterAddress = serviceCenterAddress;
}
public String getServiceCenterAddress()
{
return serviceCenterAddress;
}
public void setOriginatingAddress(String originatingAddress)
{
this.originatingAddress = originatingAddress;
}
public String getOriginatingAddress()
{
return originatingAddress;
}
public void setDisplayOriginatingAddress(String displayOriginatingAddress)
{
this.displayOriginatingAddress = displayOriginatingAddress;
}
public String getDisplayOriginatingAddress()
{
return displayOriginatingAddress;
}
public void setMessageBody(String messageBody)
{
this.messageBody = messageBody;
}
public String getMessageBody()
{
return messageBody;
}
public ArrayList getDisplayMessageBody()
{
return displayMessageBody;
}
public void setDisplayMessageBody(ArrayList displayMessageBody)
{
this.displayMessageBody = displayMessageBody;
}
public void setPseudoSubject(String pseudoSubject)
{
this.pseudoSubject = pseudoSubject;
}
public String getPseudoSubject()
{
return pseudoSubject;
}
public void setTimestampMillis(long timestampMillis)
{
this.timestampMillis = timestampMillis;
}
public long getTimestampMillis()
{
return timestampMillis;
}
public void setEmail(boolean email)
{
this.email = email;
}
public boolean getEmail()
{
return email;
}
public void setEmailBody(String emailBody)
{
this.emailBody = emailBody;
}
public String getEmailBody()
{
return emailBody;
}
public void setEmailFrom(String emailFrom)
{
this.emailFrom = emailFrom;
}
public String getEmailFrom()
{
return emailFrom;
}
public void setProtocolIdentifier(int protocolIdentifier)
{
this.protocolIdentifier = protocolIdentifier;
}
public int getProtocolIdentifier()
{
return protocolIdentifier;
}
public void setReplace(boolean replace)
{
this.replace = replace;
}
public boolean getReplace()
{
return replace;
}
public void setCphsMwiMessage(boolean cphsMwiMessage)
{
this.cphsMwiMessage = cphsMwiMessage;
}
public boolean getCphsMwiMessage()
{
return cphsMwiMessage;
}
public void setMWIClearMessage(boolean mwiClearMessage)
{
this.MWIClearMessage = mwiClearMessage;
}
public boolean getMWIClearMessage()
{
return MWIClearMessage;
}
public void setMWISetMessage(boolean mwiSetMessage)
{
this.MWISetMessage = mwiSetMessage;
}
public boolean getMWISetMessage()
{
return MWISetMessage;
}
public void setMwiDontStore(boolean mwiDontStore)
{
this.mwiDontStore = mwiDontStore;
}
public boolean getMwiDontStore()
{
return mwiDontStore;
}
public void setUserData(byte[] userData)
{
this.userData = userData;
}
public byte[] getUserData()
{
return userData;
}
public void setStatusOnIcc(int statusOnIcc)
{
this.statusOnIcc = statusOnIcc;
}
public int getStatusOnIcc()
{
return statusOnIcc;
}
public void setIndexOnIcc(int indexOnIcc)
{
this.indexOnIcc = indexOnIcc;
}
public int getIndexOnIcc()
{
return indexOnIcc;
}
public void setStatus(int status)
{
this.status = status;
}
public int getStatus()
{
return status;
}
public void setStatusReportMessage(boolean statusReportMessage)
{
this.statusReportMessage = statusReportMessage;
}
public boolean getStatusReportMessage()
{
return statusReportMessage;
}
public void setReplyPathPresent(boolean replyPathPresent)
{
this.replyPathPresent = replyPathPresent;
}
public boolean getReplyPathPresent()
{
return replyPathPresent;
}
public void setMessageClass(SmsClass messageClass)
{
this.messageClass = messageClass;
}
public SmsClass getMessageClass()
{
return messageClass;
}
public String getMessage()
{
StringBuilder sb = new StringBuilder();
for (String s : getDisplayMessageBody())
{
sb.append(s);
}
return sb.toString();
}
@Override
public String toString()
{
return "SmsReceiveModel{" +
"serviceCenterAddress='" + serviceCenterAddress + '\'' +
", originatingAddress='" + originatingAddress + '\'' +
", displayOriginatingAddress='" + displayOriginatingAddress + '\'' +
", messageBody='" + messageBody + '\'' +
", displayMessageBody=" + displayMessageBody +
", pseudoSubject='" + pseudoSubject + '\'' +
", timestampMillis=" + timestampMillis +
", email=" + email +
", emailBody='" + emailBody + '\'' +
", emailFrom='" + emailFrom + '\'' +
", protocolIdentifier=" + protocolIdentifier +
", replace=" + replace +
", cphsMwiMessage=" + cphsMwiMessage +
", MWIClearMessage=" + MWIClearMessage +
", MWISetMessage=" + MWISetMessage +
", mwiDontStore=" + mwiDontStore +
", userData=" + Arrays.toString(userData) +
", statusOnIcc=" + statusOnIcc +
", indexOnIcc=" + indexOnIcc +
", status=" + status +
", statusReportMessage=" + statusReportMessage +
", replyPathPresent=" + replyPathPresent +
", messageClass=" + messageClass +
"} " + super.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy