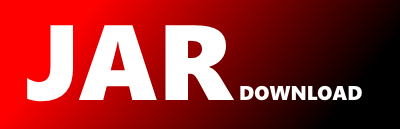
cz.mmsparams.api.websocket.model.smsc.SmscModelBase Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of MmsParamsAPI Show documentation
Show all versions of MmsParamsAPI Show documentation
Common library for MmsParams system
The newest version!
package cz.mmsparams.api.websocket.model.smsc;
import java.io.Serializable;
import java.util.ArrayList;
import cz.mmsparams.api.websocket.WebSocketModelBase;
public class SmscModelBase extends WebSocketModelBase implements Serializable
{
private String name;
private int commandId;
private int commandLength;
private int commandStatus;
private boolean isRequest;
private boolean isResponse;
private int sequenceNumber;
private ArrayList optionalParameters;
public void setCommandId(int commandId)
{
this.commandId = commandId;
}
public int getCommandId()
{
return commandId;
}
public void setCommandLength(int commandLength)
{
this.commandLength = commandLength;
}
public int getCommandLength()
{
return commandLength;
}
public void setCommandStatus(int commandStatus)
{
this.commandStatus = commandStatus;
}
public int getCommandStatus()
{
return commandStatus;
}
public void setName(String name)
{
this.name = name;
}
public String getName()
{
return name;
}
public void setSequenceNumber(int sequenceNumber)
{
this.sequenceNumber = sequenceNumber;
}
public int getSequenceNumber()
{
return sequenceNumber;
}
public boolean getRequest()
{
return isRequest;
}
public void setRequest(boolean request)
{
isRequest = request;
}
public boolean getResponse()
{
return isResponse;
}
public void setResponse(boolean response)
{
isResponse = response;
}
public ArrayList getOptionalParameters()
{
return optionalParameters;
}
public void setOptionalParameters(ArrayList optionalParameters)
{
this.optionalParameters = optionalParameters;
}
@Override
public String toString()
{
return "SmscModelBase{" +
"name='" + name + '\'' +
", commandId=" + commandId +
", commandLength=" + commandLength +
", commandStatus=" + commandStatus +
", isRequest=" + isRequest +
", isResponse=" + isResponse +
", sequenceNumber=" + sequenceNumber +
", optionalParameters=" + optionalParameters +
"} " + super.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy