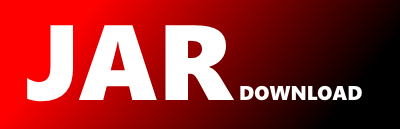
cz.wicketstuff.boss.flow.processor.ext.DefaultFlowProcessor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of boss-flow Show documentation
Show all versions of boss-flow Show documentation
Workflow control and configuration java framework
package cz.wicketstuff.boss.flow.processor.ext;
import java.io.InputStream;
import java.io.Serializable;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import cz.wicketstuff.boss.flow.FlowException;
import cz.wicketstuff.boss.flow.builder.IFlowBuilder;
import cz.wicketstuff.boss.flow.builder.xml.JaxbFlowBuilder;
import cz.wicketstuff.boss.flow.model.IFlowCarter;
import cz.wicketstuff.boss.flow.model.IFlowState;
import cz.wicketstuff.boss.flow.model.IFlowTree;
import cz.wicketstuff.boss.flow.model.basic.FlowCarter;
import cz.wicketstuff.boss.flow.processor.IFlowCarterFactory;
import cz.wicketstuff.boss.flow.processor.IFlowProcessor;
import cz.wicketstuff.boss.flow.processor.IFlowStatePersister;
import cz.wicketstuff.boss.flow.processor.IFlowStateProcessor;
import cz.wicketstuff.boss.flow.processor.IFlowStateResolver;
import cz.wicketstuff.boss.flow.processor.IFlowTransitionResolver;
import cz.wicketstuff.boss.flow.processor.NoSuchStateException;
import cz.wicketstuff.boss.flow.processor.basic.DefaultFlowStateProcessor;
import cz.wicketstuff.boss.flow.processor.basic.SimpleFlowProcessor;
import cz.wicketstuff.boss.flow.processor.basic.SimpleFlowStateProcessor;
import cz.wicketstuff.boss.flow.processor.basic.SimpleFlowStateResolver;
import cz.wicketstuff.boss.flow.processor.basic.SimpleFlowTransitionResolver;
/**
* @author Martin Strejc
*
* @param
*/
public class DefaultFlowProcessor extends
SimpleFlowProcessor {
private static final Logger log = LoggerFactory.getLogger(DefaultFlowProcessor.class);
private static final long serialVersionUID = 1L;
private String defaultInitialStateName;
private IFlowTree flowTree;
private InputStream flowInputStream;
/**
* Default constructor
*/
public DefaultFlowProcessor() {
super();
}
public IFlowProcessor initializeProcessor() throws FlowException {
onInitializeProcessor();
IFlowBuilder flowBuilder = defaultFlowBuilder();
flowTree = flowBuilder.buildFlowTree(
getFlowInputStream(), getFlowId(), getFlowName());
setCarterFactory(defaultCarterFactory());
setStateProcessor(defaultFlowStateProcessor(flowTree));
setStateResolver(defaultFlowStateResolver(flowTree));
setTransitionResolver(defaultFlowTransitionResolver(flowTree));
scanAnnotedBeans();
IFlowState initialState = defaultInitialState();
if(initialState != null) {
setDefaultInitialState(initialState);
}
if(getDefaultInitialState() == null) {
log.warn("Default flow initial state is NULL!");
}
IFlowStatePersister statePersister = defaultFlowStatePersister();
if(statePersister != null) {
setFlowStatePersister(statePersister);
}
if(getFlowStatePersister() == null) {
log.warn("Missing flow state persister");
}
onAfterInitializeProcessor();
return this;
}
public void onInitializeProcessor() {
}
public void onAfterInitializeProcessor() {
}
public IFlowCarter defaultFlowCarter(Long flowProcessId,
IFlowState initialState) {
return new FlowCarter(flowProcessId, initialState);
}
public IFlowCarterFactory defaultCarterFactory() {
return new IFlowCarterFactory() {
@Override
public IFlowCarter createFlowCarter(Long flowProcessId,
IFlowState initialState) throws FlowException {
return defaultFlowCarter(flowProcessId, initialState);
}
};
}
public void scanAnnotedBeans() throws FlowAnnotationException {
scanAnnotedBeans(defaultConditionBean(), defaultSwitchBean(),
defaultStateListenerBean(), defaultTransitionListenerBean());
}
public void scanAnnotedBeans(Object conditionBean, Object switchBean,
Object stateListenerBean, Object transitionListenerBean)
throws FlowAnnotationException {
SimpleFlowStateProcessor stateProcessor = getSimpleFlowStateProcessor();
AnnotationFlowFactory annotFactory = defaultAnnotationFlowFactory(getFlowTree());
if (conditionBean != null) {
stateProcessor.setConditionProcessor(annotFactory
.getFlowConditionProcessors(conditionBean));
}
if (switchBean != null) {
stateProcessor.setSwitchProcessor(annotFactory
.getFlowSwitchProcessors(switchBean));
}
if (stateListenerBean != null) {
setStateChangeListener(annotFactory
.getStateChangeListeners(stateListenerBean));
}
if (transitionListenerBean != null) {
setTransitionChangeListener(annotFactory
.getTransitionChangeListeners(transitionListenerBean));
}
}
public Object defaultConditionBean() {
return null;
}
public Object defaultSwitchBean() {
return null;
}
public Object defaultStateListenerBean() {
return null;
}
public Object defaultTransitionListenerBean() {
return null;
}
public IFlowStatePersister defaultFlowStatePersister() {
return null;
}
protected SimpleFlowStateProcessor getSimpleFlowStateProcessor() {
IFlowStateProcessor p = getStateProcessor();
if (p instanceof SimpleFlowStateProcessor) {
return (SimpleFlowStateProcessor) p;
}
throw new ClassCastException(
"An instance of SimpleFlowStateProcessor is required. Do not use annotation factory that need this class or check your class hierarchy.");
}
public IFlowBuilder defaultFlowBuilder() {
return JaxbFlowBuilder.newInstance();
}
public IFlowStateProcessor defaultFlowStateProcessor(IFlowTree flowTree) {
return new DefaultFlowStateProcessor();
}
public IFlowStateResolver defaultFlowStateResolver(IFlowTree flowTree) {
return new SimpleFlowStateResolver(flowTree);
}
public IFlowTransitionResolver defaultFlowTransitionResolver(
IFlowTree flowTree) {
return new SimpleFlowTransitionResolver(flowTree);
}
public AnnotationFlowFactory defaultAnnotationFlowFactory(
IFlowTree flowTree) {
return new AnnotationFlowFactory();
}
public IFlowState defaultInitialState() throws NoSuchStateException {
String stateName = getDefaultInitialStateName();
if(stateName == null) {
return flowTree.getDefaultInitialState();
}
return getStateResolver().resolveState(stateName);
}
public String getDefaultInitialStateName() {
return defaultInitialStateName;
}
public void setDefaultInitialStateName(String defaultInitialStateName) {
this.defaultInitialStateName = defaultInitialStateName;
}
public IFlowTree getFlowTree() {
return flowTree;
}
public void setFlowTree(IFlowTree flowTree) {
this.flowTree = flowTree;
}
public InputStream getFlowInputStream() {
return flowInputStream;
}
public void setFlowInputStream(InputStream flowInputStream) {
this.flowInputStream = flowInputStream;
}
@Override
protected void finalize() throws Throwable {
super.finalize();
defaultInitialStateName = null;
flowTree = null;
flowInputStream = null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy