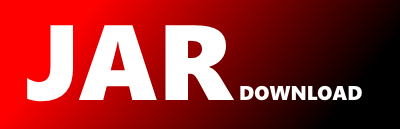
cz.wicketstuff.boss.flow.builder.xml.FlowObjectFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of boss-flow Show documentation
Show all versions of boss-flow Show documentation
Workflow control and configuration java framework
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package cz.wicketstuff.boss.flow.builder.xml;
import cz.wicketstuff.boss.flow.builder.xml.jaxb.ConditionStateType;
import cz.wicketstuff.boss.flow.builder.xml.jaxb.JoinStateType;
import cz.wicketstuff.boss.flow.builder.xml.jaxb.RealStateType;
import cz.wicketstuff.boss.flow.builder.xml.jaxb.StateType;
import cz.wicketstuff.boss.flow.builder.xml.jaxb.SwitchStateType;
import cz.wicketstuff.boss.flow.builder.xml.jaxb.TransitionType;
import cz.wicketstuff.boss.flow.builder.xml.jaxb.ViewStateType;
import cz.wicketstuff.boss.flow.builder.xml.jaxb.VirtualStateType;
import cz.wicketstuff.boss.flow.model.IFlowConditionState;
import cz.wicketstuff.boss.flow.model.IFlowJoinState;
import cz.wicketstuff.boss.flow.model.IFlowRealState;
import cz.wicketstuff.boss.flow.model.IFlowState;
import cz.wicketstuff.boss.flow.model.IFlowSwitchState;
import cz.wicketstuff.boss.flow.model.IFlowTransition;
import cz.wicketstuff.boss.flow.model.IFlowViewState;
import cz.wicketstuff.boss.flow.model.IFlowVirtualState;
import cz.wicketstuff.boss.flow.model.basic.FlowConditionState;
import cz.wicketstuff.boss.flow.model.basic.FlowJoinState;
import cz.wicketstuff.boss.flow.model.basic.FlowRealState;
import cz.wicketstuff.boss.flow.model.basic.FlowState;
import cz.wicketstuff.boss.flow.model.basic.FlowSwitchState;
import cz.wicketstuff.boss.flow.model.basic.FlowTransition;
import cz.wicketstuff.boss.flow.model.basic.FlowViewState;
import cz.wicketstuff.boss.flow.model.basic.FlowVirtualState;
/**
* Object factory that creates implementations of flow objects
* those are defined just by their interfaces.
*
* Objects are usually created from their XML descriptors.
*
* @author Martin Strejc
*
*/
/**
* @author Martin Strejc
*
*/
public class FlowObjectFactory {
/**
* Enable or disable autogeneration of missing flow or transition IDs
*/
private boolean autoGenerateId = true;
/**
* The last autogenerated transition ID value.
*/
private int lastTransitionId = Integer.MAX_VALUE;
/**
* The last autogenerated state ID value.
*/
private int lastStateId = Integer.MAX_VALUE;
/**
* Default empty constructor.
*/
public FlowObjectFactory() {
}
/**
* Create flow transition object from XML transition descriptor
* as an implementation of {@link IFlowTransition}
*
* Generate ID if ID is null and {@link #autoGenerateId} is enabled
*
* @param transition
* @return transtion object
*/
public FlowTransition createFlowTransition(TransitionType transition) {
if(transition.getId() == null && isAutoGenerateId()) {
transition.setId(generateTransitionId());
}
FlowTransition ft = new FlowTransition(transition.getId(), transition.getName());
ft.setHitCountable(transition.isHitCountable());
return ft;
}
/**
* Create flow state object from XML state descriptor
* as an implementation of {@link IFlowState}
*
* Generate ID if ID is null and {@link #autoGenerateId} is enabled
*
* It decides which implementation has to be used itself.
*
* @param state
* @return state object
*/
public FlowState createFlowState(StateType state) {
if(state.getId() == null && isAutoGenerateId()) {
state.setId(generateStateId());
}
FlowState fs;
if(state instanceof ViewStateType) {
fs = createFlowViewState((ViewStateType)state);
} else if(state instanceof RealStateType) {
fs = createFlowRealState((RealStateType)state);
} else if(state instanceof JoinStateType) {
fs = createFlowJoinState((JoinStateType)state);
} else if(state instanceof ConditionStateType) {
fs = createFlowConditionState((ConditionStateType)state);
} else if(state instanceof SwitchStateType) {
fs = createFlowSwitchState((SwitchStateType)state);
} else if(state instanceof VirtualStateType) {
fs = createFlowVirtualState((VirtualStateType)state);
} else {
fs = createDefaultFlowState(state);
}
fs.setStateId(state.getId());
fs.setStateName(state.getName());
fs.setInitialState(state.isInitialState() || state.isDefaultInitialState());
fs.setFinalState(state.isFinalState());
fs.setStateValidatable(state.isStateValidatable());
fs.setPersistableState(state.isPersistableState());
return fs;
}
/**
* Internal factory method to create {@link IFlowViewState}
*
* @param state
* @return view state
*/
protected FlowViewState createFlowViewState(ViewStateType state) {
if(state.getViewName() == null) {
state.setViewName(state.getName());
}
FlowViewState fs = new FlowViewState(state.getId(), state.getName());
fs.setViewName(state.getViewName());
return fs;
}
/**
* Internal factory method to create {@link IFlowRealState}
*
* @param state
* @return real state
*/
protected FlowRealState createFlowRealState(RealStateType state) {
FlowRealState fs = new FlowRealState(state.getId(), state.getName());
return fs;
}
/**
* Internal factory method to create {@link IFlowJoinState}
*
* @param state
* @return join state
*/
protected FlowJoinState createFlowJoinState(JoinStateType state) {
FlowJoinState fs = new FlowJoinState(state.getId(), state.getName());
return fs;
}
/**
* Internal factory method to create {@link IFlowConditionState}
*
* @param state
* @return condition state
*/
protected FlowConditionState createFlowConditionState(ConditionStateType state) {
FlowConditionState fs = new FlowConditionState(state.getId(), state.getName());
fs.setConditionExpression(state.getConditionExpression());
return fs;
}
/**
* Internal factory method to create {@link IFlowSwitchState}
*
* @param state
* @return switch state
*/
protected FlowSwitchState createFlowSwitchState(SwitchStateType state) {
FlowSwitchState fs = new FlowSwitchState(state.getId(), state.getName());
fs.setSwitchExpression(state.getSwitchExpression());
return fs;
}
/**
* Internal factory method to create {@link IFlowVirtualState}
*
* @param state
* @return virtual state
*/
protected FlowVirtualState createFlowVirtualState(VirtualStateType state) {
FlowVirtualState fs = new FlowVirtualState(state.getId(), state.getName());
return fs;
}
/**
* Internal factory method to create {@link IFlowState}
* when none of other subtypes is applicable.
*
* @param state
* @return flow state
*/
protected FlowState createDefaultFlowState(StateType state) {
FlowState fs = new FlowState(state.getId(), state.getName());
return fs;
}
/**
* Return {@code true} if IDs are generated. If false IDs are used from XML.
*
* @return {@code true} to generate IDs
*/
public boolean isAutoGenerateId() {
return autoGenerateId;
}
public void setAutoGenerateId(boolean autoGenerateId) {
this.autoGenerateId = autoGenerateId;
}
/**
* Generate new state id
*
* @return new state id
*/
public int generateStateId() {
return --lastStateId;
}
/**
* Generate new transition id
*
* @return new transition id
*/
public int generateTransitionId() {
return --lastTransitionId;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy