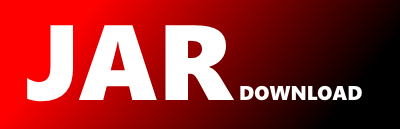
de.adorsys.psd2.api.AccountApi Maven / Gradle / Ivy
/**
* NOTE: This class is auto generated by the swagger code generator program (unset).
* https://github.com/swagger-api/swagger-codegen
* Do not edit the class manually.
*/
package de.adorsys.psd2.api;
import com.fasterxml.jackson.databind.ObjectMapper;
import de.adorsys.psd2.model.*;
import io.swagger.annotations.*;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.*;
import javax.servlet.http.HttpServletRequest;
import javax.validation.Valid;
import javax.validation.constraints.NotNull;
import java.time.LocalDate;
import java.util.Optional;
import java.util.UUID;
@javax.annotation.Generated(value = "io.swagger.codegen.v3.generators.java.SpringCodegen", date = "2018-08-13T14:16:46.416+02:00[Europe/Berlin]")
@Api(value = "v1", description = "account API")
public interface AccountApi {
Logger log = LoggerFactory.getLogger(AccountApi.class);
default Optional getObjectMapper() {
return Optional.empty();
}
default Optional getRequest() {
return Optional.empty();
}
default Optional getAcceptHeader() {
return getRequest().map(r -> r.getHeader("Accept"));
}
@ApiOperation(value = "Read Account List", nickname = "getAccountList", notes = "Read the identifiers of the available payment account together with booking balance information, depending on the consent granted. It is assumed that a consent of the PSU to this access is already given and stored on the ASPSP system. The addressed list of accounts depends then on the PSU ID and the stored consent addressed by consentId, respectively the OAuth2 access token. Returns all identifiers of the accounts, to which an account access has been granted to through the /consents endpoint by the PSU. In addition, relevant information about the accounts and hyperlinks to corresponding account information resources are provided if a related consent has been already granted. Remark: Note that the /consents endpoint optionally offers to grant an access on all available payment accounts of a PSU. In this case, this endpoint will deliver the information about all available payment accounts of the PSU at this ASPSP. ", response = AccountList.class, authorizations = {
@Authorization(value = "BearerAuthOAuth")
}, tags = {"Account Information Service (AIS)",})
@ApiResponses(value = {
@ApiResponse(code = 200, message = "OK", response = AccountList.class),
@ApiResponse(code = 400, message = "Bad Request", response = TppMessages400.class),
@ApiResponse(code = 401, message = "Unauthorized", response = TppMessages401.class),
@ApiResponse(code = 403, message = "Forbidden", response = TppMessages403.class),
@ApiResponse(code = 404, message = "Not found", response = TppMessages404.class),
@ApiResponse(code = 405, message = "Method Not Allowed", response = TppMessages405.class),
@ApiResponse(code = 406, message = "Not Acceptable", response = TppMessages406.class),
@ApiResponse(code = 408, message = "Request Timeout"),
@ApiResponse(code = 415, message = "Unsupported Media Type"),
@ApiResponse(code = 429, message = "Too Many Requests", response = TppMessages429.class),
@ApiResponse(code = 500, message = "Internal Server Error"),
@ApiResponse(code = 503, message = "Service Unavailable")})
@RequestMapping(value = "/v1/accounts",
produces = {"application/json"},
method = RequestMethod.GET)
default ResponseEntity _getAccountList(
@ApiParam(value = "ID of the request, unique to the call, as determined by the initiating party.", required = true) @RequestHeader(value = "X-Request-ID", required = true) UUID xRequestID,
@ApiParam(value = "This then contains the consentId of the related AIS consent, which was performed prior to this payment initiation. ", required = true) @RequestHeader(value = "Consent-ID", required = true) String consentID,
@ApiParam(value = "If contained, this function reads the list of accessible payment accounts including the booking balance, if granted by the PSU in the related consent and available by the ASPSP. This parameter might be ignored by the ASPSP. ") @Valid @RequestParam(value = "withBalance", required = false) Boolean withBalance,
@ApiParam(value = "Is contained if and only if the \"Signature\" element is contained in the header of the request.") @RequestHeader(value = "Digest", required = false) String digest,
@ApiParam(value = "A signature of the request by the TPP on application level. This might be mandated by ASPSP. ") @RequestHeader(value = "Signature", required = false) String signature,
@ApiParam(value = "The certificate used for signing the request, in base64 encoding. Must be contained if a signature is contained. ") @RequestHeader(value = "TPP-Signature-Certificate", required = false) byte[] tpPSignatureCertificate,
@ApiParam(value = "The forwarded IP Address header field consists of the corresponding http request IP Address field between PSU and TPP. ") @RequestHeader(value = "PSU-IP-Address", required = false) String psUIPAddress,
@ApiParam(value = "The forwarded IP Port header field consists of the corresponding HTTP request IP Port field between PSU and TPP, if available. ") @RequestHeader(value = "PSU-IP-Port", required = false) Object psUIPPort,
@ApiParam(value = "The forwarded IP Accept header fields consist of the corresponding HTTP request Accept header fields between PSU and TPP, if available. ") @RequestHeader(value = "PSU-Accept", required = false) String psUAccept,
@ApiParam(value = "The forwarded IP Accept header fields consist of the corresponding HTTP request Accept header fields between PSU and TPP, if available. ") @RequestHeader(value = "PSU-Accept-Charset", required = false) String psUAcceptCharset,
@ApiParam(value = "The forwarded IP Accept header fields consist of the corresponding HTTP request Accept header fields between PSU and TPP, if available. ") @RequestHeader(value = "PSU-Accept-Encoding", required = false) String psUAcceptEncoding,
@ApiParam(value = "The forwarded IP Accept header fields consist of the corresponding HTTP request Accept header fields between PSU and TPP, if available. ") @RequestHeader(value = "PSU-Accept-Language", required = false) String psUAcceptLanguage,
@ApiParam(value = "The forwarded Agent header field of the HTTP request between PSU and TPP, if available. ") @RequestHeader(value = "PSU-User-Agent", required = false) String psUUserAgent,
@ApiParam(value = "HTTP method used at the PSU ? TPP interface, if available. Valid values are: * GET * POST * PUT * PATCH * DELETE ", allowableValues = "GET, POST, PUT, PATCH, DELETE") @RequestHeader(value = "PSU-Http-Method", required = false) String psUHttpMethod,
@ApiParam(value = "UUID (Universally Unique Identifier) for a device, which is used by the PSU, if available. UUID identifies either a device or a device dependant application installation. In case of an installation identification this ID need to be unaltered until removal from device. ") @RequestHeader(value = "PSU-Device-ID", required = false) UUID psUDeviceID,
@ApiParam(value = "The forwarded Geo Location of the corresponding http request between PSU and TPP if available. ") @RequestHeader(value = "PSU-Geo-Location", required = false) String psUGeoLocation) {
return getAccountList(xRequestID, consentID, withBalance, digest, signature, tpPSignatureCertificate, psUIPAddress, psUIPPort, psUAccept, psUAcceptCharset, psUAcceptEncoding, psUAcceptLanguage, psUUserAgent, psUHttpMethod, psUDeviceID, psUGeoLocation);
}
// Override this method
default ResponseEntity getAccountList(UUID xRequestID, String consentID, Boolean withBalance, String digest, String signature, byte[] tpPSignatureCertificate, String psUIPAddress, Object psUIPPort, String psUAccept, String psUAcceptCharset, String psUAcceptEncoding, String psUAcceptLanguage, String psUUserAgent, String psUHttpMethod, UUID psUDeviceID, String psUGeoLocation) {
if (getObjectMapper().isPresent() && getAcceptHeader().isPresent()) {
} else {
log.warn("ObjectMapper or HttpServletRequest not configured in default V1Api interface so no example is generated");
}
return new ResponseEntity<>(HttpStatus.NOT_IMPLEMENTED);
}
@ApiOperation(value = "Read Balance", nickname = "getBalances", notes = "Reads account data from a given account addressed by \"account-id\". **Remark:** This account-id can be a tokenised identification due to data protection reason since the path information might be logged on intermediary servers within the ASPSP sphere. This account-id then can be retrieved by the \"GET Account List\" call. The account-id is constant at least throughout the lifecycle of a given consent. ", response = ReadBalanceResponse200.class, authorizations = {
@Authorization(value = "BearerAuthOAuth")
}, tags = {"Account Information Service (AIS)",})
@ApiResponses(value = {
@ApiResponse(code = 200, message = "OK", response = ReadBalanceResponse200.class),
@ApiResponse(code = 400, message = "Bad Request", response = TppMessages400.class),
@ApiResponse(code = 401, message = "Unauthorized", response = TppMessages401.class),
@ApiResponse(code = 403, message = "Forbidden", response = TppMessages403.class),
@ApiResponse(code = 404, message = "Not found", response = TppMessages404.class),
@ApiResponse(code = 405, message = "Method Not Allowed", response = TppMessages405.class),
@ApiResponse(code = 406, message = "Not Acceptable", response = TppMessages406.class),
@ApiResponse(code = 408, message = "Request Timeout"),
@ApiResponse(code = 415, message = "Unsupported Media Type"),
@ApiResponse(code = 429, message = "Too Many Requests", response = TppMessages429.class),
@ApiResponse(code = 500, message = "Internal Server Error"),
@ApiResponse(code = 503, message = "Service Unavailable")})
@RequestMapping(value = "/v1/accounts/{account-id}/balances",
produces = {"application/json"},
method = RequestMethod.GET)
default ResponseEntity _getBalances(
@ApiParam(value = "This identification is denoting the addressed account. The account-id is retrieved by using a \"Read Account List\" call. The account-id is the \"id\" attribute of the account structure. Its value is constant at least throughout the lifecycle of a given consent. ", required = true) @PathVariable("account-id") String accountId,
@ApiParam(value = "ID of the request, unique to the call, as determined by the initiating party.", required = true) @RequestHeader(value = "X-Request-ID", required = true) UUID xRequestID,
@ApiParam(value = "This then contains the consentId of the related AIS consent, which was performed prior to this payment initiation. ", required = true) @RequestHeader(value = "Consent-ID", required = true) String consentID,
@ApiParam(value = "Is contained if and only if the \"Signature\" element is contained in the header of the request.") @RequestHeader(value = "Digest", required = false) String digest,
@ApiParam(value = "A signature of the request by the TPP on application level. This might be mandated by ASPSP. ") @RequestHeader(value = "Signature", required = false) String signature,
@ApiParam(value = "The certificate used for signing the request, in base64 encoding. Must be contained if a signature is contained. ") @RequestHeader(value = "TPP-Signature-Certificate", required = false) byte[] tpPSignatureCertificate,
@ApiParam(value = "The forwarded IP Address header field consists of the corresponding http request IP Address field between PSU and TPP. ") @RequestHeader(value = "PSU-IP-Address", required = false) String psUIPAddress,
@ApiParam(value = "The forwarded IP Port header field consists of the corresponding HTTP request IP Port field between PSU and TPP, if available. ") @RequestHeader(value = "PSU-IP-Port", required = false) Object psUIPPort,
@ApiParam(value = "The forwarded IP Accept header fields consist of the corresponding HTTP request Accept header fields between PSU and TPP, if available. ") @RequestHeader(value = "PSU-Accept", required = false) String psUAccept,
@ApiParam(value = "The forwarded IP Accept header fields consist of the corresponding HTTP request Accept header fields between PSU and TPP, if available. ") @RequestHeader(value = "PSU-Accept-Charset", required = false) String psUAcceptCharset,
@ApiParam(value = "The forwarded IP Accept header fields consist of the corresponding HTTP request Accept header fields between PSU and TPP, if available. ") @RequestHeader(value = "PSU-Accept-Encoding", required = false) String psUAcceptEncoding,
@ApiParam(value = "The forwarded IP Accept header fields consist of the corresponding HTTP request Accept header fields between PSU and TPP, if available. ") @RequestHeader(value = "PSU-Accept-Language", required = false) String psUAcceptLanguage,
@ApiParam(value = "The forwarded Agent header field of the HTTP request between PSU and TPP, if available. ") @RequestHeader(value = "PSU-User-Agent", required = false) String psUUserAgent,
@ApiParam(value = "HTTP method used at the PSU ? TPP interface, if available. Valid values are: * GET * POST * PUT * PATCH * DELETE ", allowableValues = "GET, POST, PUT, PATCH, DELETE") @RequestHeader(value = "PSU-Http-Method", required = false) String psUHttpMethod,
@ApiParam(value = "UUID (Universally Unique Identifier) for a device, which is used by the PSU, if available. UUID identifies either a device or a device dependant application installation. In case of an installation identification this ID need to be unaltered until removal from device. ") @RequestHeader(value = "PSU-Device-ID", required = false) UUID psUDeviceID,
@ApiParam(value = "The forwarded Geo Location of the corresponding http request between PSU and TPP if available. ") @RequestHeader(value = "PSU-Geo-Location", required = false) String psUGeoLocation) {
return getBalances(accountId, xRequestID, consentID, digest, signature, tpPSignatureCertificate, psUIPAddress, psUIPPort, psUAccept, psUAcceptCharset, psUAcceptEncoding, psUAcceptLanguage, psUUserAgent, psUHttpMethod, psUDeviceID, psUGeoLocation);
}
// Override this method
default ResponseEntity getBalances(String accountId, UUID xRequestID, String consentID, String digest, String signature, byte[] tpPSignatureCertificate, String psUIPAddress, Object psUIPPort, String psUAccept, String psUAcceptCharset, String psUAcceptEncoding, String psUAcceptLanguage, String psUUserAgent, String psUHttpMethod, UUID psUDeviceID, String psUGeoLocation) {
if (getObjectMapper().isPresent() && getAcceptHeader().isPresent()) {
} else {
log.warn("ObjectMapper or HttpServletRequest not configured in default V1Api interface so no example is generated");
}
return new ResponseEntity<>(HttpStatus.NOT_IMPLEMENTED);
}
@ApiOperation(value = "Read Transaction Details", nickname = "getTransactionDetails", notes = "Reads transaction details from a given transaction addressed by \"resourceId\" on a given account addressed by \"account-id\". This call is only available on transactions as reported in a JSON format. **Remark:** Please note that the PATH might be already given in detail by the corresponding entry of the response of the \"Read Transaction List\" call within the _links subfield. ", response = TransactionDetails.class, authorizations = {
@Authorization(value = "BearerAuthOAuth")
}, tags = {"Account Information Service (AIS)",})
@ApiResponses(value = {
@ApiResponse(code = 200, message = "OK", response = TransactionDetails.class),
@ApiResponse(code = 400, message = "Bad Request", response = TppMessages400.class),
@ApiResponse(code = 401, message = "Unauthorized", response = TppMessages401.class),
@ApiResponse(code = 403, message = "Forbidden", response = TppMessages403.class),
@ApiResponse(code = 404, message = "Not found", response = TppMessages404.class),
@ApiResponse(code = 405, message = "Method Not Allowed", response = TppMessages405.class),
@ApiResponse(code = 406, message = "Not Acceptable", response = TppMessages406.class),
@ApiResponse(code = 408, message = "Request Timeout"),
@ApiResponse(code = 415, message = "Unsupported Media Type"),
@ApiResponse(code = 429, message = "Too Many Requests", response = TppMessages429.class),
@ApiResponse(code = 500, message = "Internal Server Error"),
@ApiResponse(code = 503, message = "Service Unavailable")})
@RequestMapping(value = "/v1/accounts/{account-id}/transactions/{resourceId}",
produces = {"application/json"},
method = RequestMethod.GET)
default ResponseEntity _getTransactionDetails(
@ApiParam(value = "This identification is denoting the addressed account. The account-id is retrieved by using a \"Read Account List\" call. The account-id is the \"id\" attribute of the account structure. Its value is constant at least throughout the lifecycle of a given consent. ", required = true) @PathVariable("account-id") String accountId,
@ApiParam(value = "This identification is given by the attribute resourceId of the corresponding entry of a transaction list. ", required = true) @PathVariable("resourceId") String resourceId,
@ApiParam(value = "ID of the request, unique to the call, as determined by the initiating party.", required = true) @RequestHeader(value = "X-Request-ID", required = true) UUID xRequestID,
@ApiParam(value = "This then contains the consentId of the related AIS consent, which was performed prior to this payment initiation. ", required = true) @RequestHeader(value = "Consent-ID", required = true) String consentID,
@ApiParam(value = "Is contained if and only if the \"Signature\" element is contained in the header of the request.") @RequestHeader(value = "Digest", required = false) String digest,
@ApiParam(value = "A signature of the request by the TPP on application level. This might be mandated by ASPSP. ") @RequestHeader(value = "Signature", required = false) String signature,
@ApiParam(value = "The certificate used for signing the request, in base64 encoding. Must be contained if a signature is contained. ") @RequestHeader(value = "TPP-Signature-Certificate", required = false) byte[] tpPSignatureCertificate,
@ApiParam(value = "The forwarded IP Address header field consists of the corresponding http request IP Address field between PSU and TPP. ") @RequestHeader(value = "PSU-IP-Address", required = false) String psUIPAddress,
@ApiParam(value = "The forwarded IP Port header field consists of the corresponding HTTP request IP Port field between PSU and TPP, if available. ") @RequestHeader(value = "PSU-IP-Port", required = false) Object psUIPPort,
@ApiParam(value = "The forwarded IP Accept header fields consist of the corresponding HTTP request Accept header fields between PSU and TPP, if available. ") @RequestHeader(value = "PSU-Accept", required = false) String psUAccept,
@ApiParam(value = "The forwarded IP Accept header fields consist of the corresponding HTTP request Accept header fields between PSU and TPP, if available. ") @RequestHeader(value = "PSU-Accept-Charset", required = false) String psUAcceptCharset,
@ApiParam(value = "The forwarded IP Accept header fields consist of the corresponding HTTP request Accept header fields between PSU and TPP, if available. ") @RequestHeader(value = "PSU-Accept-Encoding", required = false) String psUAcceptEncoding,
@ApiParam(value = "The forwarded IP Accept header fields consist of the corresponding HTTP request Accept header fields between PSU and TPP, if available. ") @RequestHeader(value = "PSU-Accept-Language", required = false) String psUAcceptLanguage,
@ApiParam(value = "The forwarded Agent header field of the HTTP request between PSU and TPP, if available. ") @RequestHeader(value = "PSU-User-Agent", required = false) String psUUserAgent,
@ApiParam(value = "HTTP method used at the PSU ? TPP interface, if available. Valid values are: * GET * POST * PUT * PATCH * DELETE ", allowableValues = "GET, POST, PUT, PATCH, DELETE") @RequestHeader(value = "PSU-Http-Method", required = false) String psUHttpMethod,
@ApiParam(value = "UUID (Universally Unique Identifier) for a device, which is used by the PSU, if available. UUID identifies either a device or a device dependant application installation. In case of an installation identification this ID need to be unaltered until removal from device. ") @RequestHeader(value = "PSU-Device-ID", required = false) UUID psUDeviceID,
@ApiParam(value = "The forwarded Geo Location of the corresponding http request between PSU and TPP if available. ") @RequestHeader(value = "PSU-Geo-Location", required = false) String psUGeoLocation) {
return getTransactionDetails(accountId, resourceId, xRequestID, consentID, digest, signature, tpPSignatureCertificate, psUIPAddress, psUIPPort, psUAccept, psUAcceptCharset, psUAcceptEncoding, psUAcceptLanguage, psUUserAgent, psUHttpMethod, psUDeviceID, psUGeoLocation);
}
// Override this method
default ResponseEntity getTransactionDetails(String accountId, String resourceId, UUID xRequestID, String consentID, String digest, String signature, byte[] tpPSignatureCertificate, String psUIPAddress, Object psUIPPort, String psUAccept, String psUAcceptCharset, String psUAcceptEncoding, String psUAcceptLanguage, String psUUserAgent, String psUHttpMethod, UUID psUDeviceID, String psUGeoLocation) {
if (getObjectMapper().isPresent() && getAcceptHeader().isPresent()) {
} else {
log.warn("ObjectMapper or HttpServletRequest not configured in default V1Api interface so no example is generated");
}
return new ResponseEntity<>(HttpStatus.NOT_IMPLEMENTED);
}
@ApiOperation(value = "Read Transaction List", nickname = "getTransactionList", notes = "Read transaction reports or transaction lists of a given account ddressed by \"account-id\", depending on the steering parameter \"bookingStatus\" together with balances. For a given account, additional parameters are e.g. the attributes \"dateFrom\" and \"dateTo\". The ASPSP might add balance information, if transaction lists without balances are not supported. ", response = TransactionsResponse200Json.class, authorizations = {
@Authorization(value = "BearerAuthOAuth")
}, tags = {"Account Information Service (AIS)",})
@ApiResponses(value = {
@ApiResponse(code = 200, message = "OK", response = TransactionsResponse200Json.class),
@ApiResponse(code = 400, message = "Bad Request", response = TppMessages400.class),
@ApiResponse(code = 401, message = "Unauthorized", response = TppMessages401.class),
@ApiResponse(code = 403, message = "Forbidden", response = TppMessages403.class),
@ApiResponse(code = 404, message = "Not found", response = TppMessages404.class),
@ApiResponse(code = 405, message = "Method Not Allowed", response = TppMessages405.class),
@ApiResponse(code = 406, message = "Not Acceptable", response = TppMessages406.class),
@ApiResponse(code = 408, message = "Request Timeout"),
@ApiResponse(code = 415, message = "Unsupported Media Type"),
@ApiResponse(code = 429, message = "Too Many Requests", response = TppMessages429.class),
@ApiResponse(code = 500, message = "Internal Server Error"),
@ApiResponse(code = 503, message = "Service Unavailable")})
@RequestMapping(value = "/v1/accounts/{account-id}/transactions/",
produces = {"application/json", "application/xml", "application/text"},
method = RequestMethod.GET)
default ResponseEntity _getTransactionList(
@ApiParam(value = "This identification is denoting the addressed account. The account-id is retrieved by using a \"Read Account List\" call. The account-id is the \"id\" attribute of the account structure. Its value is constant at least throughout the lifecycle of a given consent. ", required = true) @PathVariable("account-id") String accountId, @NotNull
@ApiParam(value = "Permitted codes are * \"booked\", * \"pending\" and * \"both\" \"booked\" shall be supported by the ASPSP. To support the \"pending\" and \"both\" feature is optional for the ASPSP, Error code if not supported in the online banking frontend ", required = true, allowableValues = "booked, pending, both") @Valid @RequestParam(value = "bookingStatus", required = true) String bookingStatus,
@ApiParam(value = "ID of the request, unique to the call, as determined by the initiating party.", required = true) @RequestHeader(value = "X-Request-ID", required = true) UUID xRequestID,
@ApiParam(value = "This then contains the consentId of the related AIS consent, which was performed prior to this payment initiation. ", required = true) @RequestHeader(value = "Consent-ID", required = true) String consentID,
@ApiParam(value = "Conditional: Starting date (inclusive the date dateFrom) of the transaction list, mandated if no delta access is required. ") @Valid @RequestParam(value = "dateFrom", required = false) LocalDate dateFrom,
@ApiParam(value = "End date (inclusive the data dateTo) of the transaction list, default is now if not given. ") @Valid @RequestParam(value = "dateTo", required = false) LocalDate dateTo,
@ApiParam(value = "This data attribute is indicating that the AISP is in favour to get all transactions after the transaction with identification entryReferenceFrom alternatively to the above defined period. This is a implementation of a delta access. If this data element is contained, the entries \"dateFrom\" and \"dateTo\" might be ignored by the ASPSP if a delta report is supported. Optional if supported by API provider. ") @Valid @RequestParam(value = "entryReferenceFrom", required = false) String entryReferenceFrom,
@ApiParam(value = "This data attribute is indicating that the AISP is in favour to get all transactions after the last report access for this PSU on the addressed account. This is another implementation of a delta access-report. This delta indicator might be rejected by the ASPSP if this function is not supported. Optional if supported by API provider") @Valid @RequestParam(value = "deltaList", required = false) Boolean deltaList,
@ApiParam(value = "If contained, this function reads the list of accessible payment accounts including the booking balance, if granted by the PSU in the related consent and available by the ASPSP. This parameter might be ignored by the ASPSP. ") @Valid @RequestParam(value = "withBalance", required = false) Boolean withBalance,
@ApiParam(value = "Is contained if and only if the \"Signature\" element is contained in the header of the request.") @RequestHeader(value = "Digest", required = false) String digest,
@ApiParam(value = "A signature of the request by the TPP on application level. This might be mandated by ASPSP. ") @RequestHeader(value = "Signature", required = false) String signature,
@ApiParam(value = "The certificate used for signing the request, in base64 encoding. Must be contained if a signature is contained. ") @RequestHeader(value = "TPP-Signature-Certificate", required = false) byte[] tpPSignatureCertificate,
@ApiParam(value = "The forwarded IP Address header field consists of the corresponding http request IP Address field between PSU and TPP. ") @RequestHeader(value = "PSU-IP-Address", required = false) String psUIPAddress,
@ApiParam(value = "The forwarded IP Port header field consists of the corresponding HTTP request IP Port field between PSU and TPP, if available. ") @RequestHeader(value = "PSU-IP-Port", required = false) Object psUIPPort,
@ApiParam(value = "The forwarded IP Accept header fields consist of the corresponding HTTP request Accept header fields between PSU and TPP, if available. ") @RequestHeader(value = "PSU-Accept", required = false) String psUAccept,
@ApiParam(value = "The forwarded IP Accept header fields consist of the corresponding HTTP request Accept header fields between PSU and TPP, if available. ") @RequestHeader(value = "PSU-Accept-Charset", required = false) String psUAcceptCharset,
@ApiParam(value = "The forwarded IP Accept header fields consist of the corresponding HTTP request Accept header fields between PSU and TPP, if available. ") @RequestHeader(value = "PSU-Accept-Encoding", required = false) String psUAcceptEncoding,
@ApiParam(value = "The forwarded IP Accept header fields consist of the corresponding HTTP request Accept header fields between PSU and TPP, if available. ") @RequestHeader(value = "PSU-Accept-Language", required = false) String psUAcceptLanguage,
@ApiParam(value = "The forwarded Agent header field of the HTTP request between PSU and TPP, if available. ") @RequestHeader(value = "PSU-User-Agent", required = false) String psUUserAgent,
@ApiParam(value = "HTTP method used at the PSU ? TPP interface, if available. Valid values are: * GET * POST * PUT * PATCH * DELETE ", allowableValues = "GET, POST, PUT, PATCH, DELETE") @RequestHeader(value = "PSU-Http-Method", required = false) String psUHttpMethod,
@ApiParam(value = "UUID (Universally Unique Identifier) for a device, which is used by the PSU, if available. UUID identifies either a device or a device dependant application installation. In case of an installation identification this ID need to be unaltered until removal from device. ") @RequestHeader(value = "PSU-Device-ID", required = false) UUID psUDeviceID,
@ApiParam(value = "The forwarded Geo Location of the corresponding http request between PSU and TPP if available. ") @RequestHeader(value = "PSU-Geo-Location", required = false) String psUGeoLocation) {
return getTransactionList(accountId, bookingStatus, xRequestID, consentID, dateFrom, dateTo, entryReferenceFrom, deltaList, withBalance, digest, signature, tpPSignatureCertificate, psUIPAddress, psUIPPort, psUAccept, psUAcceptCharset, psUAcceptEncoding, psUAcceptLanguage, psUUserAgent, psUHttpMethod, psUDeviceID, psUGeoLocation);
}
// Override this method
default ResponseEntity getTransactionList(String accountId, String bookingStatus, UUID xRequestID, String consentID, LocalDate dateFrom, LocalDate dateTo, String entryReferenceFrom, Boolean deltaList, Boolean withBalance, String digest, String signature, byte[] tpPSignatureCertificate, String psUIPAddress, Object psUIPPort, String psUAccept, String psUAcceptCharset, String psUAcceptEncoding, String psUAcceptLanguage, String psUUserAgent, String psUHttpMethod, UUID psUDeviceID, String psUGeoLocation) {
if (getObjectMapper().isPresent() && getAcceptHeader().isPresent()) {
} else {
log.warn("ObjectMapper or HttpServletRequest not configured in default V1Api interface so no example is generated");
}
return new ResponseEntity<>(HttpStatus.NOT_IMPLEMENTED);
}
@ApiOperation(value = "Read Account Details", nickname = "readAccountDetails", notes = "Reads details about an account, with balances where required. It is assumed that a consent of the PSU to this access is already given and stored on the ASPSP system. The addressed details of this account depends then on the stored consent addressed by consentId, respectively the OAuth2 access token. **NOTE:** The account-id can represent a multicurrency account. In this case the currency code is set to \"XXX\". Give detailed information about the addressed account. Give detailed information about the addressed account together with balance information ", response = AccountDetails.class, authorizations = {
@Authorization(value = "BearerAuthOAuth")
}, tags = {"Account Information Service (AIS)",})
@ApiResponses(value = {
@ApiResponse(code = 200, message = "OK", response = AccountDetails.class),
@ApiResponse(code = 400, message = "Bad Request", response = TppMessages400.class),
@ApiResponse(code = 401, message = "Unauthorized", response = TppMessages401.class),
@ApiResponse(code = 403, message = "Forbidden", response = TppMessages403.class),
@ApiResponse(code = 404, message = "Not found", response = TppMessages404.class),
@ApiResponse(code = 405, message = "Method Not Allowed", response = TppMessages405.class),
@ApiResponse(code = 406, message = "Not Acceptable", response = TppMessages406.class),
@ApiResponse(code = 408, message = "Request Timeout"),
@ApiResponse(code = 415, message = "Unsupported Media Type"),
@ApiResponse(code = 429, message = "Too Many Requests", response = TppMessages429.class),
@ApiResponse(code = 500, message = "Internal Server Error"),
@ApiResponse(code = 503, message = "Service Unavailable")})
@RequestMapping(value = "/v1/accounts/{account-id}",
produces = {"application/json"},
method = RequestMethod.GET)
default ResponseEntity _readAccountDetails(
@ApiParam(value = "This identification is denoting the addressed account. The account-id is retrieved by using a \"Read Account List\" call. The account-id is the \"id\" attribute of the account structure. Its value is constant at least throughout the lifecycle of a given consent. ", required = true) @PathVariable("account-id") String accountId,
@ApiParam(value = "ID of the request, unique to the call, as determined by the initiating party.", required = true) @RequestHeader(value = "X-Request-ID", required = true) UUID xRequestID,
@ApiParam(value = "This then contains the consentId of the related AIS consent, which was performed prior to this payment initiation. ", required = true) @RequestHeader(value = "Consent-ID", required = true) String consentID,
@ApiParam(value = "If contained, this function reads the list of accessible payment accounts including the booking balance, if granted by the PSU in the related consent and available by the ASPSP. This parameter might be ignored by the ASPSP. ") @Valid @RequestParam(value = "withBalance", required = false) Boolean withBalance,
@ApiParam(value = "Is contained if and only if the \"Signature\" element is contained in the header of the request.") @RequestHeader(value = "Digest", required = false) String digest,
@ApiParam(value = "A signature of the request by the TPP on application level. This might be mandated by ASPSP. ") @RequestHeader(value = "Signature", required = false) String signature,
@ApiParam(value = "The certificate used for signing the request, in base64 encoding. Must be contained if a signature is contained. ") @RequestHeader(value = "TPP-Signature-Certificate", required = false) byte[] tpPSignatureCertificate,
@ApiParam(value = "The forwarded IP Address header field consists of the corresponding http request IP Address field between PSU and TPP. ") @RequestHeader(value = "PSU-IP-Address", required = false) String psUIPAddress,
@ApiParam(value = "The forwarded IP Port header field consists of the corresponding HTTP request IP Port field between PSU and TPP, if available. ") @RequestHeader(value = "PSU-IP-Port", required = false) Object psUIPPort,
@ApiParam(value = "The forwarded IP Accept header fields consist of the corresponding HTTP request Accept header fields between PSU and TPP, if available. ") @RequestHeader(value = "PSU-Accept", required = false) String psUAccept,
@ApiParam(value = "The forwarded IP Accept header fields consist of the corresponding HTTP request Accept header fields between PSU and TPP, if available. ") @RequestHeader(value = "PSU-Accept-Charset", required = false) String psUAcceptCharset,
@ApiParam(value = "The forwarded IP Accept header fields consist of the corresponding HTTP request Accept header fields between PSU and TPP, if available. ") @RequestHeader(value = "PSU-Accept-Encoding", required = false) String psUAcceptEncoding,
@ApiParam(value = "The forwarded IP Accept header fields consist of the corresponding HTTP request Accept header fields between PSU and TPP, if available. ") @RequestHeader(value = "PSU-Accept-Language", required = false) String psUAcceptLanguage,
@ApiParam(value = "The forwarded Agent header field of the HTTP request between PSU and TPP, if available. ") @RequestHeader(value = "PSU-User-Agent", required = false) String psUUserAgent,
@ApiParam(value = "HTTP method used at the PSU ? TPP interface, if available. Valid values are: * GET * POST * PUT * PATCH * DELETE ", allowableValues = "GET, POST, PUT, PATCH, DELETE") @RequestHeader(value = "PSU-Http-Method", required = false) String psUHttpMethod,
@ApiParam(value = "UUID (Universally Unique Identifier) for a device, which is used by the PSU, if available. UUID identifies either a device or a device dependant application installation. In case of an installation identification this ID need to be unaltered until removal from device. ") @RequestHeader(value = "PSU-Device-ID", required = false) UUID psUDeviceID,
@ApiParam(value = "The forwarded Geo Location of the corresponding http request between PSU and TPP if available. ") @RequestHeader(value = "PSU-Geo-Location", required = false) String psUGeoLocation) {
return readAccountDetails(accountId, xRequestID, consentID, withBalance, digest, signature, tpPSignatureCertificate, psUIPAddress, psUIPPort, psUAccept, psUAcceptCharset, psUAcceptEncoding, psUAcceptLanguage, psUUserAgent, psUHttpMethod, psUDeviceID, psUGeoLocation);
}
// Override this method
default ResponseEntity readAccountDetails(String accountId, UUID xRequestID, String consentID, Boolean withBalance, String digest, String signature, byte[] tpPSignatureCertificate, String psUIPAddress, Object psUIPPort, String psUAccept, String psUAcceptCharset, String psUAcceptEncoding, String psUAcceptLanguage, String psUUserAgent, String psUHttpMethod, UUID psUDeviceID, String psUGeoLocation) {
if (getObjectMapper().isPresent() && getAcceptHeader().isPresent()) {
} else {
log.warn("ObjectMapper or HttpServletRequest not configured in default V1Api interface so no example is generated");
}
return new ResponseEntity<>(HttpStatus.NOT_IMPLEMENTED);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy