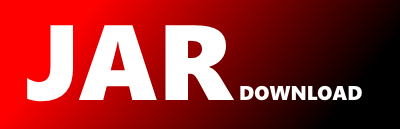
de.adorsys.psd2.api.ConsentApi Maven / Gradle / Ivy
/**
* NOTE: This class is auto generated by the swagger code generator program (unset).
* https://github.com/swagger-api/swagger-codegen
* Do not edit the class manually.
*/
package de.adorsys.psd2.api;
import com.fasterxml.jackson.databind.ObjectMapper;
import de.adorsys.psd2.model.*;
import io.swagger.annotations.*;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.*;
import javax.servlet.http.HttpServletRequest;
import javax.validation.Valid;
import java.util.Optional;
import java.util.UUID;
@javax.annotation.Generated(value = "io.swagger.codegen.v3.generators.java.SpringCodegen", date = "2018-08-13T14:16:46.416+02:00[Europe/Berlin]")
@Api(value = "v1", description = "consent API")
public interface ConsentApi {
Logger log = LoggerFactory.getLogger(ConsentApi.class);
default Optional getObjectMapper() {
return Optional.empty();
}
default Optional getRequest() {
return Optional.empty();
}
default Optional getAcceptHeader() {
return getRequest().map(r -> r.getHeader("Accept"));
}
@ApiOperation(value = "Create consent", nickname = "createConsent", notes = "This method create a consent resource, defining access rights to dedicated accounts of a given PSU-ID. These accounts are addressed explicitly in the method as parameters as a core function. **Side Effects** When this Consent Request is a request where the \"recurringIndicator\" equals \"true\", and if it exists already a former consent for recurring access on account information for the addressed PSU, then the former consent automatically expires as soon as the new consent request is authorised by the PSU. Optional Extension: As an option, an ASPSP might optionally accept a specific access right on the access on all psd2 related services for all available accounts. As another option an ASPSP might optionally also accept a command, where only access rights are inserted without mentioning the addressed account. The relation to accounts is then handled afterwards between PSU and ASPSP. This option is supported only within the Decoupled, OAuth2 or Re-direct SCA Approach. As a last option, an ASPSP might in addition accept a command with access rights * to see the list of available payment accounts or * to see the list of available payment accounts with balances. ", response = ConsentsResponse201.class, authorizations = {
@Authorization(value = "BearerAuthOAuth")
}, tags = {"Account Information Service (AIS)",})
@ApiResponses(value = {
@ApiResponse(code = 201, message = "Created", response = ConsentsResponse201.class),
@ApiResponse(code = 400, message = "Bad Request", response = TppMessages400.class),
@ApiResponse(code = 401, message = "Unauthorized", response = TppMessages401.class),
@ApiResponse(code = 403, message = "Forbidden", response = TppMessages403.class),
@ApiResponse(code = 404, message = "Not found", response = TppMessages404.class),
@ApiResponse(code = 405, message = "Method Not Allowed", response = TppMessages405.class),
@ApiResponse(code = 406, message = "Not Acceptable", response = TppMessages406.class),
@ApiResponse(code = 408, message = "Request Timeout"),
@ApiResponse(code = 415, message = "Unsupported Media Type"),
@ApiResponse(code = 429, message = "Too Many Requests", response = TppMessages429.class),
@ApiResponse(code = 500, message = "Internal Server Error"),
@ApiResponse(code = 503, message = "Service Unavailable")})
@RequestMapping(value = "/v1/consents",
produces = {"application/json"},
method = RequestMethod.POST)
default ResponseEntity _createConsent(
@ApiParam(value = "ID of the request, unique to the call, as determined by the initiating party.", required = true) @RequestHeader(value = "X-Request-ID", required = true) UUID xRequestID,
@ApiParam(value = "Requestbody for a consents request ") @Valid @RequestBody Consents body,
@ApiParam(value = "Is contained if and only if the \"Signature\" element is contained in the header of the request.") @RequestHeader(value = "Digest", required = false) String digest,
@ApiParam(value = "A signature of the request by the TPP on application level. This might be mandated by ASPSP. ") @RequestHeader(value = "Signature", required = false) String signature,
@ApiParam(value = "The certificate used for signing the request, in base64 encoding. Must be contained if a signature is contained. ") @RequestHeader(value = "TPP-Signature-Certificate", required = false) byte[] tpPSignatureCertificate,
@ApiParam(value = "Client ID of the PSU in the ASPSP client interface. Might be mandated in the ASPSP's documentation. Is not contained if an OAuth2 based authentication was performed in a pre-step or an OAuth2 based SCA was performed in an preceeding AIS service in the same session. ") @RequestHeader(value = "PSU-ID", required = false) String PSU_ID,
@ApiParam(value = "Type of the PSU-ID, needed in scenarios where PSUs have several PSU-IDs as access possibility. ") @RequestHeader(value = "PSU-ID-Type", required = false) String psUIDType,
@ApiParam(value = "Might be mandated in the ASPSP's documentation. Only used in a corporate context. ") @RequestHeader(value = "PSU-Corporate-ID", required = false) String psUCorporateID,
@ApiParam(value = "Might be mandated in the ASPSP's documentation. Only used in a corporate context. ") @RequestHeader(value = "PSU-Corporate-ID-Type", required = false) String psUCorporateIDType,
@ApiParam(value = "If it equals \"true\", the TPP prefers a redirect over an embedded SCA approach. If it equals \"false\", the TPP prefers not to be redirected for SCA. The ASPSP will then choose between the Embedded or the Decoupled SCA approach, depending on the choice of the SCA procedure by the TPP/PSU. If the parameter is not used, the ASPSP will choose the SCA approach to be applied depending on the SCA method chosen by the TPP/PSU. ") @RequestHeader(value = "TPP-Redirect-Preferred", required = false) Boolean tpPRedirectPreferred,
@ApiParam(value = "URI of the TPP, where the transaction flow shall be redirected to after a Redirect. Mandated for the Redirect SCA Approach (including OAuth2 SCA approach), specifically when TPP-Redirect-Preferred equals \"true\". It is recommended to always use this header field. **Remark for Future:** This field might be changed to mandatory in the next version of the specification. ") @RequestHeader(value = "TPP-Redirect-URI", required = false) String tpPRedirectURI,
@ApiParam(value = "If this URI is contained, the TPP is asking to redirect the transaction flow to this address instead of the TPP-Redirect-URI in case of a negative result of the redirect SCA method. This might be ignored by the ASPSP. ") @RequestHeader(value = "TPP-Nok-Redirect-URI", required = false) String tpPNokRedirectURI,
@ApiParam(value = "If it equals \"true\", the TPP prefers to start the authorisation process separately, e.g. because of the usage of a signing basket. This preference might be ignored by the ASPSP, if a signing basket is not supported as functionality. If it equals \"false\" or if the parameter is not used, there is no preference of the TPP. This especially indicates that the TPP assumes a direct authorisation of the transaction in the next step, without using a signing basket. ") @RequestHeader(value = "TPP-Explicit-Authorisation-Preferred", required = false) Boolean tpPExplicitAuthorisationPreferred,
@ApiParam(value = "The forwarded IP Address header field consists of the corresponding http request IP Address field between PSU and TPP. ") @RequestHeader(value = "PSU-IP-Address", required = false) String psUIPAddress,
@ApiParam(value = "The forwarded IP Port header field consists of the corresponding HTTP request IP Port field between PSU and TPP, if available. ") @RequestHeader(value = "PSU-IP-Port", required = false) Object psUIPPort,
@ApiParam(value = "The forwarded IP Accept header fields consist of the corresponding HTTP request Accept header fields between PSU and TPP, if available. ") @RequestHeader(value = "PSU-Accept", required = false) String psUAccept,
@ApiParam(value = "The forwarded IP Accept header fields consist of the corresponding HTTP request Accept header fields between PSU and TPP, if available. ") @RequestHeader(value = "PSU-Accept-Charset", required = false) String psUAcceptCharset,
@ApiParam(value = "The forwarded IP Accept header fields consist of the corresponding HTTP request Accept header fields between PSU and TPP, if available. ") @RequestHeader(value = "PSU-Accept-Encoding", required = false) String psUAcceptEncoding,
@ApiParam(value = "The forwarded IP Accept header fields consist of the corresponding HTTP request Accept header fields between PSU and TPP, if available. ") @RequestHeader(value = "PSU-Accept-Language", required = false) String psUAcceptLanguage,
@ApiParam(value = "The forwarded Agent header field of the HTTP request between PSU and TPP, if available. ") @RequestHeader(value = "PSU-User-Agent", required = false) String psUUserAgent,
@ApiParam(value = "HTTP method used at the PSU ? TPP interface, if available. Valid values are: * GET * POST * PUT * PATCH * DELETE ", allowableValues = "GET, POST, PUT, PATCH, DELETE") @RequestHeader(value = "PSU-Http-Method", required = false) String psUHttpMethod,
@ApiParam(value = "UUID (Universally Unique Identifier) for a device, which is used by the PSU, if available. UUID identifies either a device or a device dependant application installation. In case of an installation identification this ID need to be unaltered until removal from device. ") @RequestHeader(value = "PSU-Device-ID", required = false) UUID psUDeviceID,
@ApiParam(value = "The forwarded Geo Location of the corresponding http request between PSU and TPP if available. ") @RequestHeader(value = "PSU-Geo-Location", required = false) String psUGeoLocation) {
return createConsent(xRequestID, body, digest, signature, tpPSignatureCertificate, PSU_ID, psUIDType, psUCorporateID, psUCorporateIDType, tpPRedirectPreferred, tpPRedirectURI, tpPNokRedirectURI, tpPExplicitAuthorisationPreferred, psUIPAddress, psUIPPort, psUAccept, psUAcceptCharset, psUAcceptEncoding, psUAcceptLanguage, psUUserAgent, psUHttpMethod, psUDeviceID, psUGeoLocation);
}
// Override this method
default ResponseEntity createConsent(UUID xRequestID, Consents body, String digest, String signature, byte[] tpPSignatureCertificate, String PSU_ID, String psUIDType, String psUCorporateID, String psUCorporateIDType, Boolean tpPRedirectPreferred, String tpPRedirectURI, String tpPNokRedirectURI, Boolean tpPExplicitAuthorisationPreferred, String psUIPAddress, Object psUIPPort, String psUAccept, String psUAcceptCharset, String psUAcceptEncoding, String psUAcceptLanguage, String psUUserAgent, String psUHttpMethod, UUID psUDeviceID, String psUGeoLocation) {
if (getObjectMapper().isPresent() && getAcceptHeader().isPresent()) {
} else {
log.warn("ObjectMapper or HttpServletRequest not configured in default V1Api interface so no example is generated");
}
return new ResponseEntity<>(HttpStatus.NOT_IMPLEMENTED);
}
@ApiOperation(value = "Delete Consent", nickname = "deleteConsent", notes = "The TPP can delete an account information consent object if needed.", authorizations = {
@Authorization(value = "BearerAuthOAuth")
}, tags = {"Account Information Service (AIS)",})
@ApiResponses(value = {
@ApiResponse(code = 204, message = "No Content"),
@ApiResponse(code = 400, message = "Bad Request", response = TppMessages400.class),
@ApiResponse(code = 401, message = "Unauthorized", response = TppMessages401.class),
@ApiResponse(code = 403, message = "Forbidden", response = TppMessages403.class),
@ApiResponse(code = 404, message = "Not found", response = TppMessages404.class),
@ApiResponse(code = 405, message = "Method Not Allowed", response = TppMessages405.class),
@ApiResponse(code = 406, message = "Not Acceptable", response = TppMessages406.class),
@ApiResponse(code = 408, message = "Request Timeout"),
@ApiResponse(code = 415, message = "Unsupported Media Type"),
@ApiResponse(code = 429, message = "Too Many Requests", response = TppMessages429.class),
@ApiResponse(code = 500, message = "Internal Server Error"),
@ApiResponse(code = 503, message = "Service Unavailable")})
@RequestMapping(value = "/v1/consents/{consentId}",
produces = {"application/json"},
method = RequestMethod.DELETE)
default ResponseEntity _deleteConsent(
@ApiParam(value = "ID of the corresponding consent object as returned by an Account Information Consent Request. ", required = true) @PathVariable("consentId") String consentId,
@ApiParam(value = "ID of the request, unique to the call, as determined by the initiating party.", required = true) @RequestHeader(value = "X-Request-ID", required = true) UUID xRequestID,
@ApiParam(value = "Is contained if and only if the \"Signature\" element is contained in the header of the request.") @RequestHeader(value = "Digest", required = false) String digest,
@ApiParam(value = "A signature of the request by the TPP on application level. This might be mandated by ASPSP. ") @RequestHeader(value = "Signature", required = false) String signature,
@ApiParam(value = "The certificate used for signing the request, in base64 encoding. Must be contained if a signature is contained. ") @RequestHeader(value = "TPP-Signature-Certificate", required = false) byte[] tpPSignatureCertificate,
@ApiParam(value = "The forwarded IP Address header field consists of the corresponding http request IP Address field between PSU and TPP. ") @RequestHeader(value = "PSU-IP-Address", required = false) String psUIPAddress,
@ApiParam(value = "The forwarded IP Port header field consists of the corresponding HTTP request IP Port field between PSU and TPP, if available. ") @RequestHeader(value = "PSU-IP-Port", required = false) Object psUIPPort,
@ApiParam(value = "The forwarded IP Accept header fields consist of the corresponding HTTP request Accept header fields between PSU and TPP, if available. ") @RequestHeader(value = "PSU-Accept", required = false) String psUAccept,
@ApiParam(value = "The forwarded IP Accept header fields consist of the corresponding HTTP request Accept header fields between PSU and TPP, if available. ") @RequestHeader(value = "PSU-Accept-Charset", required = false) String psUAcceptCharset,
@ApiParam(value = "The forwarded IP Accept header fields consist of the corresponding HTTP request Accept header fields between PSU and TPP, if available. ") @RequestHeader(value = "PSU-Accept-Encoding", required = false) String psUAcceptEncoding,
@ApiParam(value = "The forwarded IP Accept header fields consist of the corresponding HTTP request Accept header fields between PSU and TPP, if available. ") @RequestHeader(value = "PSU-Accept-Language", required = false) String psUAcceptLanguage,
@ApiParam(value = "The forwarded Agent header field of the HTTP request between PSU and TPP, if available. ") @RequestHeader(value = "PSU-User-Agent", required = false) String psUUserAgent,
@ApiParam(value = "HTTP method used at the PSU ? TPP interface, if available. Valid values are: * GET * POST * PUT * PATCH * DELETE ", allowableValues = "GET, POST, PUT, PATCH, DELETE") @RequestHeader(value = "PSU-Http-Method", required = false) String psUHttpMethod,
@ApiParam(value = "UUID (Universally Unique Identifier) for a device, which is used by the PSU, if available. UUID identifies either a device or a device dependant application installation. In case of an installation identification this ID need to be unaltered until removal from device. ") @RequestHeader(value = "PSU-Device-ID", required = false) UUID psUDeviceID,
@ApiParam(value = "The forwarded Geo Location of the corresponding http request between PSU and TPP if available. ") @RequestHeader(value = "PSU-Geo-Location", required = false) String psUGeoLocation) {
return deleteConsent(consentId, xRequestID, digest, signature, tpPSignatureCertificate, psUIPAddress, psUIPPort, psUAccept, psUAcceptCharset, psUAcceptEncoding, psUAcceptLanguage, psUUserAgent, psUHttpMethod, psUDeviceID, psUGeoLocation);
}
// Override this method
default ResponseEntity deleteConsent(String consentId, UUID xRequestID, String digest, String signature, byte[] tpPSignatureCertificate, String psUIPAddress, Object psUIPPort, String psUAccept, String psUAcceptCharset, String psUAcceptEncoding, String psUAcceptLanguage, String psUUserAgent, String psUHttpMethod, UUID psUDeviceID, String psUGeoLocation) {
if (getObjectMapper().isPresent() && getAcceptHeader().isPresent()) {
} else {
log.warn("ObjectMapper or HttpServletRequest not configured in default V1Api interface so no example is generated");
}
return new ResponseEntity<>(HttpStatus.NOT_IMPLEMENTED);
}
@ApiOperation(value = "Get Consent Authorisation Sub-Resources Request", nickname = "getConsentAuthorisations", notes = "Return a list of all authorisation subresources IDs which have been created. This function returns an array of hyperlinks to all generated authorisation sub-resources. ", response = Authorisations.class, authorizations = {
@Authorization(value = "BearerAuthOAuth")
}, tags = {"Account Information Service (AIS)", "Common AIS and PIS Services",})
@ApiResponses(value = {
@ApiResponse(code = 200, message = "OK", response = Authorisations.class),
@ApiResponse(code = 400, message = "Bad Request", response = TppMessages400.class),
@ApiResponse(code = 401, message = "Unauthorized", response = TppMessages401.class),
@ApiResponse(code = 403, message = "Forbidden", response = TppMessages403.class),
@ApiResponse(code = 404, message = "Not found", response = TppMessages404.class),
@ApiResponse(code = 405, message = "Method Not Allowed", response = TppMessages405.class),
@ApiResponse(code = 406, message = "Not Acceptable", response = TppMessages406.class),
@ApiResponse(code = 408, message = "Request Timeout"),
@ApiResponse(code = 415, message = "Unsupported Media Type"),
@ApiResponse(code = 429, message = "Too Many Requests", response = TppMessages429.class),
@ApiResponse(code = 500, message = "Internal Server Error"),
@ApiResponse(code = 503, message = "Service Unavailable")})
@RequestMapping(value = "/v1/consents/{consentId}/authorisations",
produces = {"application/json"},
method = RequestMethod.GET)
default ResponseEntity _getConsentAuthorisations(
@ApiParam(value = "ID of the corresponding consent object as returned by an Account Information Consent Request. ", required = true) @PathVariable("consentId") String consentId,
@ApiParam(value = "ID of the request, unique to the call, as determined by the initiating party.", required = true) @RequestHeader(value = "X-Request-ID", required = true) UUID xRequestID,
@ApiParam(value = "Is contained if and only if the \"Signature\" element is contained in the header of the request.") @RequestHeader(value = "Digest", required = false) String digest,
@ApiParam(value = "A signature of the request by the TPP on application level. This might be mandated by ASPSP. ") @RequestHeader(value = "Signature", required = false) String signature,
@ApiParam(value = "The certificate used for signing the request, in base64 encoding. Must be contained if a signature is contained. ") @RequestHeader(value = "TPP-Signature-Certificate", required = false) byte[] tpPSignatureCertificate,
@ApiParam(value = "The forwarded IP Address header field consists of the corresponding http request IP Address field between PSU and TPP. ") @RequestHeader(value = "PSU-IP-Address", required = false) String psUIPAddress,
@ApiParam(value = "The forwarded IP Port header field consists of the corresponding HTTP request IP Port field between PSU and TPP, if available. ") @RequestHeader(value = "PSU-IP-Port", required = false) Object psUIPPort,
@ApiParam(value = "The forwarded IP Accept header fields consist of the corresponding HTTP request Accept header fields between PSU and TPP, if available. ") @RequestHeader(value = "PSU-Accept", required = false) String psUAccept,
@ApiParam(value = "The forwarded IP Accept header fields consist of the corresponding HTTP request Accept header fields between PSU and TPP, if available. ") @RequestHeader(value = "PSU-Accept-Charset", required = false) String psUAcceptCharset,
@ApiParam(value = "The forwarded IP Accept header fields consist of the corresponding HTTP request Accept header fields between PSU and TPP, if available. ") @RequestHeader(value = "PSU-Accept-Encoding", required = false) String psUAcceptEncoding,
@ApiParam(value = "The forwarded IP Accept header fields consist of the corresponding HTTP request Accept header fields between PSU and TPP, if available. ") @RequestHeader(value = "PSU-Accept-Language", required = false) String psUAcceptLanguage,
@ApiParam(value = "The forwarded Agent header field of the HTTP request between PSU and TPP, if available. ") @RequestHeader(value = "PSU-User-Agent", required = false) String psUUserAgent,
@ApiParam(value = "HTTP method used at the PSU ? TPP interface, if available. Valid values are: * GET * POST * PUT * PATCH * DELETE ", allowableValues = "GET, POST, PUT, PATCH, DELETE") @RequestHeader(value = "PSU-Http-Method", required = false) String psUHttpMethod,
@ApiParam(value = "UUID (Universally Unique Identifier) for a device, which is used by the PSU, if available. UUID identifies either a device or a device dependant application installation. In case of an installation identification this ID need to be unaltered until removal from device. ") @RequestHeader(value = "PSU-Device-ID", required = false) UUID psUDeviceID,
@ApiParam(value = "The forwarded Geo Location of the corresponding http request between PSU and TPP if available. ") @RequestHeader(value = "PSU-Geo-Location", required = false) String psUGeoLocation) {
return getConsentAuthorisations(consentId, xRequestID, digest, signature, tpPSignatureCertificate, psUIPAddress, psUIPPort, psUAccept, psUAcceptCharset, psUAcceptEncoding, psUAcceptLanguage, psUUserAgent, psUHttpMethod, psUDeviceID, psUGeoLocation);
}
// Override this method
default ResponseEntity getConsentAuthorisations(String consentId, UUID xRequestID, String digest, String signature, byte[] tpPSignatureCertificate, String psUIPAddress, Object psUIPPort, String psUAccept, String psUAcceptCharset, String psUAcceptEncoding, String psUAcceptLanguage, String psUUserAgent, String psUHttpMethod, UUID psUDeviceID, String psUGeoLocation) {
if (getObjectMapper().isPresent() && getAcceptHeader().isPresent()) {
} else {
log.warn("ObjectMapper or HttpServletRequest not configured in default V1Api interface so no example is generated");
}
return new ResponseEntity<>(HttpStatus.NOT_IMPLEMENTED);
}
@ApiOperation(value = "Get Consent Request", nickname = "getConsentInformation", notes = "Returns the content of an account information consent object. This is returning the data for the TPP especially in cases, where the consent was directly managed between ASPSP and PSU e.g. in a re-direct SCA Approach. ", response = ConsentInformationResponse200Json.class, authorizations = {
@Authorization(value = "BearerAuthOAuth")
}, tags = {"Account Information Service (AIS)",})
@ApiResponses(value = {
@ApiResponse(code = 200, message = "OK", response = ConsentInformationResponse200Json.class),
@ApiResponse(code = 400, message = "Bad Request", response = TppMessages400.class),
@ApiResponse(code = 401, message = "Unauthorized", response = TppMessages401.class),
@ApiResponse(code = 403, message = "Forbidden", response = TppMessages403.class),
@ApiResponse(code = 404, message = "Not found", response = TppMessages404.class),
@ApiResponse(code = 405, message = "Method Not Allowed", response = TppMessages405.class),
@ApiResponse(code = 406, message = "Not Acceptable", response = TppMessages406.class),
@ApiResponse(code = 408, message = "Request Timeout"),
@ApiResponse(code = 415, message = "Unsupported Media Type"),
@ApiResponse(code = 429, message = "Too Many Requests", response = TppMessages429.class),
@ApiResponse(code = 500, message = "Internal Server Error"),
@ApiResponse(code = 503, message = "Service Unavailable")})
@RequestMapping(value = "/v1/consents/{consentId}",
produces = {"application/json"},
method = RequestMethod.GET)
default ResponseEntity _getConsentInformation(
@ApiParam(value = "ID of the corresponding consent object as returned by an Account Information Consent Request. ", required = true) @PathVariable("consentId") String consentId,
@ApiParam(value = "ID of the request, unique to the call, as determined by the initiating party.", required = true) @RequestHeader(value = "X-Request-ID", required = true) UUID xRequestID,
@ApiParam(value = "Is contained if and only if the \"Signature\" element is contained in the header of the request.") @RequestHeader(value = "Digest", required = false) String digest,
@ApiParam(value = "A signature of the request by the TPP on application level. This might be mandated by ASPSP. ") @RequestHeader(value = "Signature", required = false) String signature,
@ApiParam(value = "The certificate used for signing the request, in base64 encoding. Must be contained if a signature is contained. ") @RequestHeader(value = "TPP-Signature-Certificate", required = false) byte[] tpPSignatureCertificate,
@ApiParam(value = "The forwarded IP Address header field consists of the corresponding http request IP Address field between PSU and TPP. ") @RequestHeader(value = "PSU-IP-Address", required = false) String psUIPAddress,
@ApiParam(value = "The forwarded IP Port header field consists of the corresponding HTTP request IP Port field between PSU and TPP, if available. ") @RequestHeader(value = "PSU-IP-Port", required = false) Object psUIPPort,
@ApiParam(value = "The forwarded IP Accept header fields consist of the corresponding HTTP request Accept header fields between PSU and TPP, if available. ") @RequestHeader(value = "PSU-Accept", required = false) String psUAccept,
@ApiParam(value = "The forwarded IP Accept header fields consist of the corresponding HTTP request Accept header fields between PSU and TPP, if available. ") @RequestHeader(value = "PSU-Accept-Charset", required = false) String psUAcceptCharset,
@ApiParam(value = "The forwarded IP Accept header fields consist of the corresponding HTTP request Accept header fields between PSU and TPP, if available. ") @RequestHeader(value = "PSU-Accept-Encoding", required = false) String psUAcceptEncoding,
@ApiParam(value = "The forwarded IP Accept header fields consist of the corresponding HTTP request Accept header fields between PSU and TPP, if available. ") @RequestHeader(value = "PSU-Accept-Language", required = false) String psUAcceptLanguage,
@ApiParam(value = "The forwarded Agent header field of the HTTP request between PSU and TPP, if available. ") @RequestHeader(value = "PSU-User-Agent", required = false) String psUUserAgent,
@ApiParam(value = "HTTP method used at the PSU ? TPP interface, if available. Valid values are: * GET * POST * PUT * PATCH * DELETE ", allowableValues = "GET, POST, PUT, PATCH, DELETE") @RequestHeader(value = "PSU-Http-Method", required = false) String psUHttpMethod,
@ApiParam(value = "UUID (Universally Unique Identifier) for a device, which is used by the PSU, if available. UUID identifies either a device or a device dependant application installation. In case of an installation identification this ID need to be unaltered until removal from device. ") @RequestHeader(value = "PSU-Device-ID", required = false) UUID psUDeviceID,
@ApiParam(value = "The forwarded Geo Location of the corresponding http request between PSU and TPP if available. ") @RequestHeader(value = "PSU-Geo-Location", required = false) String psUGeoLocation) {
return getConsentInformation(consentId, xRequestID, digest, signature, tpPSignatureCertificate, psUIPAddress, psUIPPort, psUAccept, psUAcceptCharset, psUAcceptEncoding, psUAcceptLanguage, psUUserAgent, psUHttpMethod, psUDeviceID, psUGeoLocation);
}
// Override this method
default ResponseEntity getConsentInformation(String consentId, UUID xRequestID, String digest, String signature, byte[] tpPSignatureCertificate, String psUIPAddress, Object psUIPPort, String psUAccept, String psUAcceptCharset, String psUAcceptEncoding, String psUAcceptLanguage, String psUUserAgent, String psUHttpMethod, UUID psUDeviceID, String psUGeoLocation) {
if (getObjectMapper().isPresent() && getAcceptHeader().isPresent()) {
} else {
log.warn("ObjectMapper or HttpServletRequest not configured in default V1Api interface so no example is generated");
}
return new ResponseEntity<>(HttpStatus.NOT_IMPLEMENTED);
}
@ApiOperation(value = "Read the SCA status of the consent authorisation.", nickname = "getConsentScaStatus", notes = "This method returns the SCA status of a consent initiation's authorisation sub-resource. ", response = ScaStatusResponse.class, authorizations = {
@Authorization(value = "BearerAuthOAuth")
}, tags = {"Account Information Service (AIS)", "Common AIS and PIS Services",})
@ApiResponses(value = {
@ApiResponse(code = 200, message = "OK", response = ScaStatusResponse.class),
@ApiResponse(code = 400, message = "Bad Request", response = TppMessages400.class),
@ApiResponse(code = 401, message = "Unauthorized", response = TppMessages401.class),
@ApiResponse(code = 403, message = "Forbidden", response = TppMessages403.class),
@ApiResponse(code = 404, message = "Not found", response = TppMessages404.class),
@ApiResponse(code = 405, message = "Method Not Allowed", response = TppMessages405.class),
@ApiResponse(code = 406, message = "Not Acceptable", response = TppMessages406.class),
@ApiResponse(code = 408, message = "Request Timeout"),
@ApiResponse(code = 415, message = "Unsupported Media Type"),
@ApiResponse(code = 429, message = "Too Many Requests", response = TppMessages429.class),
@ApiResponse(code = 500, message = "Internal Server Error"),
@ApiResponse(code = 503, message = "Service Unavailable")})
@RequestMapping(value = "/v1/consents/{consentId}/authorisations/{authorisationId}",
produces = {"application/json"},
method = RequestMethod.GET)
default ResponseEntity _getConsentScaStatus(
@ApiParam(value = "ID of the corresponding consent object as returned by an Account Information Consent Request. ", required = true) @PathVariable("consentId") String consentId,
@ApiParam(value = "Resource identification of the related SCA.", required = true) @PathVariable("authorisationId") String authorisationId,
@ApiParam(value = "ID of the request, unique to the call, as determined by the initiating party.", required = true) @RequestHeader(value = "X-Request-ID", required = true) UUID xRequestID,
@ApiParam(value = "Is contained if and only if the \"Signature\" element is contained in the header of the request.") @RequestHeader(value = "Digest", required = false) String digest,
@ApiParam(value = "A signature of the request by the TPP on application level. This might be mandated by ASPSP. ") @RequestHeader(value = "Signature", required = false) String signature,
@ApiParam(value = "The certificate used for signing the request, in base64 encoding. Must be contained if a signature is contained. ") @RequestHeader(value = "TPP-Signature-Certificate", required = false) byte[] tpPSignatureCertificate,
@ApiParam(value = "The forwarded IP Address header field consists of the corresponding http request IP Address field between PSU and TPP. ") @RequestHeader(value = "PSU-IP-Address", required = false) String psUIPAddress,
@ApiParam(value = "The forwarded IP Port header field consists of the corresponding HTTP request IP Port field between PSU and TPP, if available. ") @RequestHeader(value = "PSU-IP-Port", required = false) Object psUIPPort,
@ApiParam(value = "The forwarded IP Accept header fields consist of the corresponding HTTP request Accept header fields between PSU and TPP, if available. ") @RequestHeader(value = "PSU-Accept", required = false) String psUAccept,
@ApiParam(value = "The forwarded IP Accept header fields consist of the corresponding HTTP request Accept header fields between PSU and TPP, if available. ") @RequestHeader(value = "PSU-Accept-Charset", required = false) String psUAcceptCharset,
@ApiParam(value = "The forwarded IP Accept header fields consist of the corresponding HTTP request Accept header fields between PSU and TPP, if available. ") @RequestHeader(value = "PSU-Accept-Encoding", required = false) String psUAcceptEncoding,
@ApiParam(value = "The forwarded IP Accept header fields consist of the corresponding HTTP request Accept header fields between PSU and TPP, if available. ") @RequestHeader(value = "PSU-Accept-Language", required = false) String psUAcceptLanguage,
@ApiParam(value = "The forwarded Agent header field of the HTTP request between PSU and TPP, if available. ") @RequestHeader(value = "PSU-User-Agent", required = false) String psUUserAgent,
@ApiParam(value = "HTTP method used at the PSU ? TPP interface, if available. Valid values are: * GET * POST * PUT * PATCH * DELETE ", allowableValues = "GET, POST, PUT, PATCH, DELETE") @RequestHeader(value = "PSU-Http-Method", required = false) String psUHttpMethod,
@ApiParam(value = "UUID (Universally Unique Identifier) for a device, which is used by the PSU, if available. UUID identifies either a device or a device dependant application installation. In case of an installation identification this ID need to be unaltered until removal from device. ") @RequestHeader(value = "PSU-Device-ID", required = false) UUID psUDeviceID,
@ApiParam(value = "The forwarded Geo Location of the corresponding http request between PSU and TPP if available. ") @RequestHeader(value = "PSU-Geo-Location", required = false) String psUGeoLocation) {
return getConsentScaStatus(consentId, authorisationId, xRequestID, digest, signature, tpPSignatureCertificate, psUIPAddress, psUIPPort, psUAccept, psUAcceptCharset, psUAcceptEncoding, psUAcceptLanguage, psUUserAgent, psUHttpMethod, psUDeviceID, psUGeoLocation);
}
// Override this method
default ResponseEntity getConsentScaStatus(String consentId, String authorisationId, UUID xRequestID, String digest, String signature, byte[] tpPSignatureCertificate, String psUIPAddress, Object psUIPPort, String psUAccept, String psUAcceptCharset, String psUAcceptEncoding, String psUAcceptLanguage, String psUUserAgent, String psUHttpMethod, UUID psUDeviceID, String psUGeoLocation) {
if (getObjectMapper().isPresent() && getAcceptHeader().isPresent()) {
} else {
log.warn("ObjectMapper or HttpServletRequest not configured in default V1Api interface so no example is generated");
}
return new ResponseEntity<>(HttpStatus.NOT_IMPLEMENTED);
}
@ApiOperation(value = "Consent status request", nickname = "getConsentStatus", notes = "Read the status of an account information consent resource.", response = ConsentStatusResponse200.class, authorizations = {
@Authorization(value = "BearerAuthOAuth")
}, tags = {"Account Information Service (AIS)",})
@ApiResponses(value = {
@ApiResponse(code = 200, message = "OK", response = ConsentStatusResponse200.class),
@ApiResponse(code = 400, message = "Bad Request", response = TppMessages400.class),
@ApiResponse(code = 401, message = "Unauthorized", response = TppMessages401.class),
@ApiResponse(code = 403, message = "Forbidden", response = TppMessages403.class),
@ApiResponse(code = 404, message = "Not found", response = TppMessages404.class),
@ApiResponse(code = 405, message = "Method Not Allowed", response = TppMessages405.class),
@ApiResponse(code = 406, message = "Not Acceptable", response = TppMessages406.class),
@ApiResponse(code = 408, message = "Request Timeout"),
@ApiResponse(code = 415, message = "Unsupported Media Type"),
@ApiResponse(code = 429, message = "Too Many Requests", response = TppMessages429.class),
@ApiResponse(code = 500, message = "Internal Server Error"),
@ApiResponse(code = 503, message = "Service Unavailable")})
@RequestMapping(value = "/v1/consents/{consentId}/status",
produces = {"application/json"},
method = RequestMethod.GET)
default ResponseEntity _getConsentStatus(
@ApiParam(value = "ID of the corresponding consent object as returned by an Account Information Consent Request. ", required = true) @PathVariable("consentId") String consentId,
@ApiParam(value = "ID of the request, unique to the call, as determined by the initiating party.", required = true) @RequestHeader(value = "X-Request-ID", required = true) UUID xRequestID,
@ApiParam(value = "Is contained if and only if the \"Signature\" element is contained in the header of the request.") @RequestHeader(value = "Digest", required = false) String digest,
@ApiParam(value = "A signature of the request by the TPP on application level. This might be mandated by ASPSP. ") @RequestHeader(value = "Signature", required = false) String signature,
@ApiParam(value = "The certificate used for signing the request, in base64 encoding. Must be contained if a signature is contained. ") @RequestHeader(value = "TPP-Signature-Certificate", required = false) byte[] tpPSignatureCertificate,
@ApiParam(value = "The forwarded IP Address header field consists of the corresponding http request IP Address field between PSU and TPP. ") @RequestHeader(value = "PSU-IP-Address", required = false) String psUIPAddress,
@ApiParam(value = "The forwarded IP Port header field consists of the corresponding HTTP request IP Port field between PSU and TPP, if available. ") @RequestHeader(value = "PSU-IP-Port", required = false) Object psUIPPort,
@ApiParam(value = "The forwarded IP Accept header fields consist of the corresponding HTTP request Accept header fields between PSU and TPP, if available. ") @RequestHeader(value = "PSU-Accept", required = false) String psUAccept,
@ApiParam(value = "The forwarded IP Accept header fields consist of the corresponding HTTP request Accept header fields between PSU and TPP, if available. ") @RequestHeader(value = "PSU-Accept-Charset", required = false) String psUAcceptCharset,
@ApiParam(value = "The forwarded IP Accept header fields consist of the corresponding HTTP request Accept header fields between PSU and TPP, if available. ") @RequestHeader(value = "PSU-Accept-Encoding", required = false) String psUAcceptEncoding,
@ApiParam(value = "The forwarded IP Accept header fields consist of the corresponding HTTP request Accept header fields between PSU and TPP, if available. ") @RequestHeader(value = "PSU-Accept-Language", required = false) String psUAcceptLanguage,
@ApiParam(value = "The forwarded Agent header field of the HTTP request between PSU and TPP, if available. ") @RequestHeader(value = "PSU-User-Agent", required = false) String psUUserAgent,
@ApiParam(value = "HTTP method used at the PSU ? TPP interface, if available. Valid values are: * GET * POST * PUT * PATCH * DELETE ", allowableValues = "GET, POST, PUT, PATCH, DELETE") @RequestHeader(value = "PSU-Http-Method", required = false) String psUHttpMethod,
@ApiParam(value = "UUID (Universally Unique Identifier) for a device, which is used by the PSU, if available. UUID identifies either a device or a device dependant application installation. In case of an installation identification this ID need to be unaltered until removal from device. ") @RequestHeader(value = "PSU-Device-ID", required = false) UUID psUDeviceID,
@ApiParam(value = "The forwarded Geo Location of the corresponding http request between PSU and TPP if available. ") @RequestHeader(value = "PSU-Geo-Location", required = false) String psUGeoLocation) {
return getConsentStatus(consentId, xRequestID, digest, signature, tpPSignatureCertificate, psUIPAddress, psUIPPort, psUAccept, psUAcceptCharset, psUAcceptEncoding, psUAcceptLanguage, psUUserAgent, psUHttpMethod, psUDeviceID, psUGeoLocation);
}
// Override this method
default ResponseEntity getConsentStatus(String consentId, UUID xRequestID, String digest, String signature, byte[] tpPSignatureCertificate, String psUIPAddress, Object psUIPPort, String psUAccept, String psUAcceptCharset, String psUAcceptEncoding, String psUAcceptLanguage, String psUUserAgent, String psUHttpMethod, UUID psUDeviceID, String psUGeoLocation) {
if (getObjectMapper().isPresent() && getAcceptHeader().isPresent()) {
} else {
log.warn("ObjectMapper or HttpServletRequest not configured in default V1Api interface so no example is generated");
}
return new ResponseEntity<>(HttpStatus.NOT_IMPLEMENTED);
}
@ApiOperation(value = "Start the authorisation process for a consent", nickname = "startConsentAuthorisation", notes = "Create an authorisation sub-resource and start the authorisation process of a consent. The message might in addition transmit authentication and authorisation related data. his method is iterated n times for a n times SCA authorisation in a corporate context, each creating an own authorisation sub-endpoint for the corresponding PSU authorising the consent. The ASPSP might make the usage of this access method unnecessary, since the related authorisation resource will be automatically created by the ASPSP after the submission of the consent data with the first POST consents call. The start authorisation process is a process which is needed for creating a new authorisation or cancellation sub-resource. This applies in the following scenarios: * The ASPSP has indicated with an 'startAuthorisation' hyperlink in the preceeding Payment Initiation Response that an explicit start of the authorisation process is needed by the TPP. The 'startAuthorisation' hyperlink can transport more information about data which needs to be uploaded by using the extended forms. * 'startAuthorisationWithPsuIdentfication', * 'startAuthorisationWithPsuAuthentication' * 'startAuthorisationWithAuthentciationMethodSelection' * The related payment initiation cannot yet be executed since a multilevel SCA is mandated. * The ASPSP has indicated with an 'startAuthorisation' hyperlink in the preceeding Payment Cancellation Response that an explicit start of the authorisation process is needed by the TPP. The 'startAuthorisation' hyperlink can transport more information about data which needs to be uploaded by using the extended forms as indicated above. * The related payment cancellation request cannot be applied yet since a multilevel SCA is mandate for executing the cancellation. * The signing basket needs to be authorised yet. ", response = StartScaprocessResponse.class, authorizations = {
@Authorization(value = "BearerAuthOAuth")
}, tags = {"Account Information Service (AIS)", "Common AIS and PIS Services",})
@ApiResponses(value = {
@ApiResponse(code = 201, message = "Created", response = StartScaprocessResponse.class),
@ApiResponse(code = 400, message = "Bad Request", response = TppMessages400.class),
@ApiResponse(code = 401, message = "Unauthorized", response = TppMessages401.class),
@ApiResponse(code = 403, message = "Forbidden", response = TppMessages403.class),
@ApiResponse(code = 404, message = "Not found", response = TppMessages404.class),
@ApiResponse(code = 405, message = "Method Not Allowed", response = TppMessages405.class),
@ApiResponse(code = 406, message = "Not Acceptable", response = TppMessages406.class),
@ApiResponse(code = 408, message = "Request Timeout"),
@ApiResponse(code = 415, message = "Unsupported Media Type"),
@ApiResponse(code = 429, message = "Too Many Requests", response = TppMessages429.class),
@ApiResponse(code = 500, message = "Internal Server Error"),
@ApiResponse(code = 503, message = "Service Unavailable")})
@RequestMapping(value = "/v1/consents/{consentId}/authorisations",
produces = {"application/json"},
method = RequestMethod.POST)
default ResponseEntity _startConsentAuthorisation(
@ApiParam(value = "ID of the corresponding consent object as returned by an Account Information Consent Request. ", required = true) @PathVariable("consentId") String consentId,
@ApiParam(value = "ID of the request, unique to the call, as determined by the initiating party.", required = true) @RequestHeader(value = "X-Request-ID", required = true) UUID xRequestID,
@ApiParam(value = "Is contained if and only if the \"Signature\" element is contained in the header of the request.") @RequestHeader(value = "Digest", required = false) String digest,
@ApiParam(value = "A signature of the request by the TPP on application level. This might be mandated by ASPSP. ") @RequestHeader(value = "Signature", required = false) String signature,
@ApiParam(value = "The certificate used for signing the request, in base64 encoding. Must be contained if a signature is contained. ") @RequestHeader(value = "TPP-Signature-Certificate", required = false) byte[] tpPSignatureCertificate,
@ApiParam(value = "Client ID of the PSU in the ASPSP client interface. Might be mandated in the ASPSP's documentation. Is not contained if an OAuth2 based authentication was performed in a pre-step or an OAuth2 based SCA was performed in an preceeding AIS service in the same session. ") @RequestHeader(value = "PSU-ID", required = false) String PSU_ID,
@ApiParam(value = "Type of the PSU-ID, needed in scenarios where PSUs have several PSU-IDs as access possibility. ") @RequestHeader(value = "PSU-ID-Type", required = false) String psUIDType,
@ApiParam(value = "Might be mandated in the ASPSP's documentation. Only used in a corporate context. ") @RequestHeader(value = "PSU-Corporate-ID", required = false) String psUCorporateID,
@ApiParam(value = "Might be mandated in the ASPSP's documentation. Only used in a corporate context. ") @RequestHeader(value = "PSU-Corporate-ID-Type", required = false) String psUCorporateIDType,
@ApiParam(value = "The forwarded IP Address header field consists of the corresponding http request IP Address field between PSU and TPP. ") @RequestHeader(value = "PSU-IP-Address", required = false) String psUIPAddress,
@ApiParam(value = "The forwarded IP Port header field consists of the corresponding HTTP request IP Port field between PSU and TPP, if available. ") @RequestHeader(value = "PSU-IP-Port", required = false) Object psUIPPort,
@ApiParam(value = "The forwarded IP Accept header fields consist of the corresponding HTTP request Accept header fields between PSU and TPP, if available. ") @RequestHeader(value = "PSU-Accept", required = false) String psUAccept,
@ApiParam(value = "The forwarded IP Accept header fields consist of the corresponding HTTP request Accept header fields between PSU and TPP, if available. ") @RequestHeader(value = "PSU-Accept-Charset", required = false) String psUAcceptCharset,
@ApiParam(value = "The forwarded IP Accept header fields consist of the corresponding HTTP request Accept header fields between PSU and TPP, if available. ") @RequestHeader(value = "PSU-Accept-Encoding", required = false) String psUAcceptEncoding,
@ApiParam(value = "The forwarded IP Accept header fields consist of the corresponding HTTP request Accept header fields between PSU and TPP, if available. ") @RequestHeader(value = "PSU-Accept-Language", required = false) String psUAcceptLanguage,
@ApiParam(value = "The forwarded Agent header field of the HTTP request between PSU and TPP, if available. ") @RequestHeader(value = "PSU-User-Agent", required = false) String psUUserAgent,
@ApiParam(value = "HTTP method used at the PSU ? TPP interface, if available. Valid values are: * GET * POST * PUT * PATCH * DELETE ", allowableValues = "GET, POST, PUT, PATCH, DELETE") @RequestHeader(value = "PSU-Http-Method", required = false) String psUHttpMethod,
@ApiParam(value = "UUID (Universally Unique Identifier) for a device, which is used by the PSU, if available. UUID identifies either a device or a device dependant application installation. In case of an installation identification this ID need to be unaltered until removal from device. ") @RequestHeader(value = "PSU-Device-ID", required = false) UUID psUDeviceID,
@ApiParam(value = "The forwarded Geo Location of the corresponding http request between PSU and TPP if available. ") @RequestHeader(value = "PSU-Geo-Location", required = false) String psUGeoLocation) {
return startConsentAuthorisation(consentId, xRequestID, digest, signature, tpPSignatureCertificate, PSU_ID, psUIDType, psUCorporateID, psUCorporateIDType, psUIPAddress, psUIPPort, psUAccept, psUAcceptCharset, psUAcceptEncoding, psUAcceptLanguage, psUUserAgent, psUHttpMethod, psUDeviceID, psUGeoLocation);
}
// Override this method
default ResponseEntity startConsentAuthorisation(String consentId, UUID xRequestID, String digest, String signature, byte[] tpPSignatureCertificate, String PSU_ID, String psUIDType, String psUCorporateID, String psUCorporateIDType, String psUIPAddress, Object psUIPPort, String psUAccept, String psUAcceptCharset, String psUAcceptEncoding, String psUAcceptLanguage, String psUUserAgent, String psUHttpMethod, UUID psUDeviceID, String psUGeoLocation) {
if (getObjectMapper().isPresent() && getAcceptHeader().isPresent()) {
} else {
log.warn("ObjectMapper or HttpServletRequest not configured in default V1Api interface so no example is generated");
}
return new ResponseEntity<>(HttpStatus.NOT_IMPLEMENTED);
}
@ApiOperation(value = "Update PSU Data for consents", nickname = "updateConsentsPsuData", notes = "This method update PSU data on the consents resource if needed. It may authorise a consent within the Embedded SCA Approach where needed. Independently from the SCA Approach it supports e.g. the selection of the authentication method and a non-SCA PSU authentication. This methods updates PSU data on the cancellation authorisation resource if needed. There are several possible Update PSU Data requests in the context of a consent request if needed, which depends on the SCA approach: * Redirect SCA Approach: A specific Update PSU Data Request is applicable for * the selection of authentication methods, before choosing the actual SCA approach. * Decoupled SCA Approach: A specific Update PSU Data Request is only applicable for * adding the PSU Identification, if not provided yet in the Payment Initiation Request or the Account Information Consent Request, or if no OAuth2 access token is used, or * the selection of authentication methods. * Embedded SCA Approach: The Update PSU Data Request might be used * to add credentials as a first factor authentication data of the PSU and * to select the authentication method and * transaction authorisation. The SCA Approach might depend on the chosen SCA method. For that reason, the following possible Update PSU Data request can apply to all SCA approaches: * Select an SCA method in case of several SCA methods are available for the customer. There are the following request types on this access path: * Update PSU Identification * Update PSU Authentication * Select PSU Autorization Method WARNING: This method need a reduced header, therefore many optional elements are not present. Maybe in a later version the access path will change. * Transaction Authorisation WARNING: This method need a reduced header, therefore many optional elements are not present. Maybe in a later version the access path will change. ", response = Object.class, authorizations = {
@Authorization(value = "BearerAuthOAuth")
}, tags = {"Account Information Service (AIS)", "Common AIS and PIS Services",})
@ApiResponses(value = {
@ApiResponse(code = 200, message = "OK", response = Object.class),
@ApiResponse(code = 400, message = "Bad Request", response = TppMessages400.class),
@ApiResponse(code = 401, message = "Unauthorized", response = TppMessages401.class),
@ApiResponse(code = 403, message = "Forbidden", response = TppMessages403.class),
@ApiResponse(code = 404, message = "Not found", response = TppMessages404.class),
@ApiResponse(code = 405, message = "Method Not Allowed", response = TppMessages405.class),
@ApiResponse(code = 406, message = "Not Acceptable", response = TppMessages406.class),
@ApiResponse(code = 408, message = "Request Timeout"),
@ApiResponse(code = 415, message = "Unsupported Media Type"),
@ApiResponse(code = 429, message = "Too Many Requests", response = TppMessages429.class),
@ApiResponse(code = 500, message = "Internal Server Error"),
@ApiResponse(code = 503, message = "Service Unavailable")})
@RequestMapping(value = "/v1/consents/{consentId}/authorisations/{authorisationId}",
produces = {"application/json"},
consumes = {"application/json"},
method = RequestMethod.PUT)
default ResponseEntity
© 2015 - 2025 Weber Informatics LLC | Privacy Policy