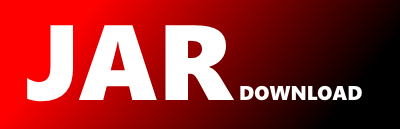
de.adorsys.ledgers.postings.impl.converter.LedgerAccountMapperImpl Maven / Gradle / Ivy
package de.adorsys.ledgers.postings.impl.converter;
import de.adorsys.ledgers.postings.api.domain.AccountCategoryBO;
import de.adorsys.ledgers.postings.api.domain.BalanceSideBO;
import de.adorsys.ledgers.postings.api.domain.ChartOfAccountBO;
import de.adorsys.ledgers.postings.api.domain.LedgerAccountBO;
import de.adorsys.ledgers.postings.db.domain.AccountCategory;
import de.adorsys.ledgers.postings.db.domain.BalanceSide;
import de.adorsys.ledgers.postings.db.domain.ChartOfAccount;
import de.adorsys.ledgers.postings.db.domain.LedgerAccount;
import java.util.ArrayList;
import java.util.List;
import javax.annotation.processing.Generated;
import org.mapstruct.factory.Mappers;
@Generated(
value = "org.mapstruct.ap.MappingProcessor",
date = "2021-01-28T10:29:40+0000",
comments = "version: 1.3.1.Final, compiler: javac, environment: Java 11.0.10 (Azul Systems, Inc.)"
)
public class LedgerAccountMapperImpl implements LedgerAccountMapper {
private final LedgerMapper ledgerMapper = Mappers.getMapper( LedgerMapper.class );
@Override
public LedgerAccountBO toLedgerAccountBO(LedgerAccount ledgerAccount) {
if ( ledgerAccount == null ) {
return null;
}
LedgerAccountBO ledgerAccountBO = new LedgerAccountBO();
ledgerAccountBO.setName( ledgerAccount.getName() );
ledgerAccountBO.setId( ledgerAccount.getId() );
ledgerAccountBO.setCreated( ledgerAccount.getCreated() );
ledgerAccountBO.setUserDetails( ledgerAccount.getUserDetails() );
ledgerAccountBO.setShortDesc( ledgerAccount.getShortDesc() );
ledgerAccountBO.setLongDesc( ledgerAccount.getLongDesc() );
ledgerAccountBO.setLedger( ledgerMapper.toLedgerBO( ledgerAccount.getLedger() ) );
ledgerAccountBO.setParent( toLedgerAccountBO( ledgerAccount.getParent() ) );
ledgerAccountBO.setCoa( chartOfAccountToChartOfAccountBO( ledgerAccount.getCoa() ) );
ledgerAccountBO.setBalanceSide( balanceSideToBalanceSideBO( ledgerAccount.getBalanceSide() ) );
ledgerAccountBO.setCategory( accountCategoryToAccountCategoryBO( ledgerAccount.getCategory() ) );
return ledgerAccountBO;
}
@Override
public LedgerAccount toLedgerAccount(LedgerAccountBO ledgerAccount) {
if ( ledgerAccount == null ) {
return null;
}
LedgerAccount ledgerAccount1 = new LedgerAccount();
ledgerAccount1.setLedger( ledgerMapper.toLedger( ledgerAccount.getLedger() ) );
ledgerAccount1.setParent( toLedgerAccount( ledgerAccount.getParent() ) );
ledgerAccount1.setCoa( chartOfAccountBOToChartOfAccount( ledgerAccount.getCoa() ) );
ledgerAccount1.setBalanceSide( balanceSideBOToBalanceSide( ledgerAccount.getBalanceSide() ) );
ledgerAccount1.setName( ledgerAccount.getName() );
ledgerAccount1.setCategory( accountCategoryBOToAccountCategory( ledgerAccount.getCategory() ) );
ledgerAccount1.setId( ledgerAccount.getId() );
ledgerAccount1.setCreated( ledgerAccount.getCreated() );
ledgerAccount1.setUserDetails( ledgerAccount.getUserDetails() );
ledgerAccount1.setShortDesc( ledgerAccount.getShortDesc() );
ledgerAccount1.setLongDesc( ledgerAccount.getLongDesc() );
return ledgerAccount1;
}
@Override
public List toLedgerAccountsBO(List accounts) {
if ( accounts == null ) {
return null;
}
List list = new ArrayList( accounts.size() );
for ( LedgerAccount ledgerAccount : accounts ) {
list.add( toLedgerAccountBO( ledgerAccount ) );
}
return list;
}
protected ChartOfAccountBO chartOfAccountToChartOfAccountBO(ChartOfAccount chartOfAccount) {
if ( chartOfAccount == null ) {
return null;
}
ChartOfAccountBO chartOfAccountBO = new ChartOfAccountBO();
chartOfAccountBO.setName( chartOfAccount.getName() );
chartOfAccountBO.setId( chartOfAccount.getId() );
chartOfAccountBO.setCreated( chartOfAccount.getCreated() );
chartOfAccountBO.setUserDetails( chartOfAccount.getUserDetails() );
chartOfAccountBO.setShortDesc( chartOfAccount.getShortDesc() );
chartOfAccountBO.setLongDesc( chartOfAccount.getLongDesc() );
return chartOfAccountBO;
}
protected BalanceSideBO balanceSideToBalanceSideBO(BalanceSide balanceSide) {
if ( balanceSide == null ) {
return null;
}
BalanceSideBO balanceSideBO;
switch ( balanceSide ) {
case Dr: balanceSideBO = BalanceSideBO.Dr;
break;
case Cr: balanceSideBO = BalanceSideBO.Cr;
break;
case DrCr: balanceSideBO = BalanceSideBO.DrCr;
break;
default: throw new IllegalArgumentException( "Unexpected enum constant: " + balanceSide );
}
return balanceSideBO;
}
protected AccountCategoryBO accountCategoryToAccountCategoryBO(AccountCategory accountCategory) {
if ( accountCategory == null ) {
return null;
}
AccountCategoryBO accountCategoryBO;
switch ( accountCategory ) {
case RE: accountCategoryBO = AccountCategoryBO.RE;
break;
case EX: accountCategoryBO = AccountCategoryBO.EX;
break;
case AS: accountCategoryBO = AccountCategoryBO.AS;
break;
case LI: accountCategoryBO = AccountCategoryBO.LI;
break;
case EQ: accountCategoryBO = AccountCategoryBO.EQ;
break;
case NOOP: accountCategoryBO = AccountCategoryBO.NOOP;
break;
case NORE: accountCategoryBO = AccountCategoryBO.NORE;
break;
case NOEX: accountCategoryBO = AccountCategoryBO.NOEX;
break;
default: throw new IllegalArgumentException( "Unexpected enum constant: " + accountCategory );
}
return accountCategoryBO;
}
protected ChartOfAccount chartOfAccountBOToChartOfAccount(ChartOfAccountBO chartOfAccountBO) {
if ( chartOfAccountBO == null ) {
return null;
}
ChartOfAccount chartOfAccount = new ChartOfAccount();
chartOfAccount.setId( chartOfAccountBO.getId() );
chartOfAccount.setCreated( chartOfAccountBO.getCreated() );
chartOfAccount.setUserDetails( chartOfAccountBO.getUserDetails() );
chartOfAccount.setShortDesc( chartOfAccountBO.getShortDesc() );
chartOfAccount.setLongDesc( chartOfAccountBO.getLongDesc() );
chartOfAccount.setName( chartOfAccountBO.getName() );
return chartOfAccount;
}
protected BalanceSide balanceSideBOToBalanceSide(BalanceSideBO balanceSideBO) {
if ( balanceSideBO == null ) {
return null;
}
BalanceSide balanceSide;
switch ( balanceSideBO ) {
case Dr: balanceSide = BalanceSide.Dr;
break;
case Cr: balanceSide = BalanceSide.Cr;
break;
case DrCr: balanceSide = BalanceSide.DrCr;
break;
default: throw new IllegalArgumentException( "Unexpected enum constant: " + balanceSideBO );
}
return balanceSide;
}
protected AccountCategory accountCategoryBOToAccountCategory(AccountCategoryBO accountCategoryBO) {
if ( accountCategoryBO == null ) {
return null;
}
AccountCategory accountCategory;
switch ( accountCategoryBO ) {
case RE: accountCategory = AccountCategory.RE;
break;
case EX: accountCategory = AccountCategory.EX;
break;
case AS: accountCategory = AccountCategory.AS;
break;
case LI: accountCategory = AccountCategory.LI;
break;
case EQ: accountCategory = AccountCategory.EQ;
break;
case NOOP: accountCategory = AccountCategory.NOOP;
break;
case NORE: accountCategory = AccountCategory.NORE;
break;
case NOEX: accountCategory = AccountCategory.NOEX;
break;
default: throw new IllegalArgumentException( "Unexpected enum constant: " + accountCategoryBO );
}
return accountCategory;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy