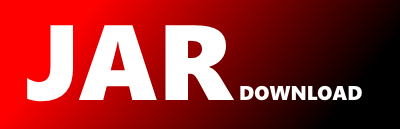
de.adorsys.multibanking.jpa.mapper.JpaEntityMapperImpl Maven / Gradle / Ivy
package de.adorsys.multibanking.jpa.mapper;
import de.adorsys.multibanking.domain.AccountAnalyticsEntity;
import de.adorsys.multibanking.domain.AnonymizedBookingEntity;
import de.adorsys.multibanking.domain.Balance;
import de.adorsys.multibanking.domain.Balance.BalanceBuilder;
import de.adorsys.multibanking.domain.BalancesReport;
import de.adorsys.multibanking.domain.BankAccessEntity;
import de.adorsys.multibanking.domain.BankAccount;
import de.adorsys.multibanking.domain.BankAccountEntity;
import de.adorsys.multibanking.domain.BankApi;
import de.adorsys.multibanking.domain.BankApiUser;
import de.adorsys.multibanking.domain.BankEntity;
import de.adorsys.multibanking.domain.BankLoginCredentialInfo;
import de.adorsys.multibanking.domain.BankLoginSettings;
import de.adorsys.multibanking.domain.BookingCategory;
import de.adorsys.multibanking.domain.BookingEntity;
import de.adorsys.multibanking.domain.BookingGroup;
import de.adorsys.multibanking.domain.BookingGroup.BookingGroupBuilder;
import de.adorsys.multibanking.domain.BookingPeriod;
import de.adorsys.multibanking.domain.BookingPeriod.BookingPeriodBuilder;
import de.adorsys.multibanking.domain.BookingsIndexEntity;
import de.adorsys.multibanking.domain.BulkPaymentEntity;
import de.adorsys.multibanking.domain.ConsentEntity;
import de.adorsys.multibanking.domain.Contract;
import de.adorsys.multibanking.domain.ContractEntity;
import de.adorsys.multibanking.domain.ExecutedBooking;
import de.adorsys.multibanking.domain.MlAnonymizedBookingEntity;
import de.adorsys.multibanking.domain.RawSepaTransactionEntity;
import de.adorsys.multibanking.domain.Rule.SIMILARITY_MATCH_TYPE;
import de.adorsys.multibanking.domain.RuleEntity;
import de.adorsys.multibanking.domain.SinglePaymentEntity;
import de.adorsys.multibanking.domain.StandingOrderEntity;
import de.adorsys.multibanking.domain.TanTransportType;
import de.adorsys.multibanking.domain.TanTransportType.TanTransportTypeBuilder;
import de.adorsys.multibanking.domain.UserEntity;
import de.adorsys.multibanking.domain.transaction.SinglePayment;
import de.adorsys.multibanking.jpa.entity.AccountAnalyticsJpaEntity;
import de.adorsys.multibanking.jpa.entity.AnonymizedBookingJpaEntity;
import de.adorsys.multibanking.jpa.entity.BalanceEntity;
import de.adorsys.multibanking.jpa.entity.BalancesReportEntity;
import de.adorsys.multibanking.jpa.entity.BankAccessJpaEntity;
import de.adorsys.multibanking.jpa.entity.BankAccountCommonJpaEntity;
import de.adorsys.multibanking.jpa.entity.BankAccountJpaEntity;
import de.adorsys.multibanking.jpa.entity.BankJpaEntity;
import de.adorsys.multibanking.jpa.entity.BankLoginCredentialInfoJpaEntity;
import de.adorsys.multibanking.jpa.entity.BankLoginSettingsJpaEntity;
import de.adorsys.multibanking.jpa.entity.BookingCategoryJpaEntity;
import de.adorsys.multibanking.jpa.entity.BookingGroupJpaEntity;
import de.adorsys.multibanking.jpa.entity.BookingGroupJpaEntity.Type;
import de.adorsys.multibanking.jpa.entity.BookingJpaEntity;
import de.adorsys.multibanking.jpa.entity.BookingPeriodJpaEntity;
import de.adorsys.multibanking.jpa.entity.BookingsIndexJpaEntity;
import de.adorsys.multibanking.jpa.entity.BulkPaymentJpaEntity;
import de.adorsys.multibanking.jpa.entity.ConsentJpaEntity;
import de.adorsys.multibanking.jpa.entity.ContractJpaEntity;
import de.adorsys.multibanking.jpa.entity.ExecutedBookingJpaEntity;
import de.adorsys.multibanking.jpa.entity.MlAnonymizedBookingJpaEntity;
import de.adorsys.multibanking.jpa.entity.RawSepaTransactionJpaEntity;
import de.adorsys.multibanking.jpa.entity.RuleJpaEntity;
import de.adorsys.multibanking.jpa.entity.SinglePaymentJpaEntity;
import de.adorsys.multibanking.jpa.entity.StandingOrderJpaEntity;
import de.adorsys.multibanking.jpa.entity.TanTransportTypeJpaEntity;
import de.adorsys.multibanking.jpa.entity.UserJpaEntity;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import javax.annotation.Generated;
import org.springframework.stereotype.Component;
@Generated(
value = "org.mapstruct.ap.MappingProcessor",
date = "2019-09-27T12:32:58+0200",
comments = "version: 1.3.0.Final, compiler: javac, environment: Java 1.8.0_211 (Oracle Corporation)"
)
@Component
public class JpaEntityMapperImpl implements JpaEntityMapper {
@Override
public AccountAnalyticsEntity mapToAccountAnalyticsEntity(AccountAnalyticsJpaEntity accountAnalyticsEntity) {
if ( accountAnalyticsEntity == null ) {
return null;
}
AccountAnalyticsEntity accountAnalyticsEntity1 = new AccountAnalyticsEntity();
if ( accountAnalyticsEntity.getId() != null ) {
accountAnalyticsEntity1.setId( String.valueOf( accountAnalyticsEntity.getId() ) );
}
accountAnalyticsEntity1.setAccountId( accountAnalyticsEntity.getAccountId() );
accountAnalyticsEntity1.setUserId( accountAnalyticsEntity.getUserId() );
accountAnalyticsEntity1.setAnalyticsDate( accountAnalyticsEntity.getAnalyticsDate() );
accountAnalyticsEntity1.setBookingGroups( bookingGroupJpaEntityListToBookingGroupList( accountAnalyticsEntity.getBookingGroups() ) );
return accountAnalyticsEntity1;
}
@Override
public BookingPeriod mapToBookingPeriodEntity(BookingPeriodJpaEntity bookingPeriod) {
if ( bookingPeriod == null ) {
return null;
}
BookingPeriodBuilder bookingPeriod1 = BookingPeriod.builder();
bookingPeriod1.start( bookingPeriod.getPeriodStart() );
bookingPeriod1.end( bookingPeriod.getPeriodEnd() );
bookingPeriod1.amount( bookingPeriod.getAmount() );
bookingPeriod1.bookings( executedBookingJpaEntityListToExecutedBookingList( bookingPeriod.getBookings() ) );
return bookingPeriod1.build();
}
@Override
public AccountAnalyticsJpaEntity mapToAccountAnalyticsJpaEntity(AccountAnalyticsEntity accountAnalyticsEntity) {
if ( accountAnalyticsEntity == null ) {
return null;
}
AccountAnalyticsJpaEntity accountAnalyticsJpaEntity = new AccountAnalyticsJpaEntity();
if ( accountAnalyticsEntity.getId() != null ) {
accountAnalyticsJpaEntity.setId( Long.parseLong( accountAnalyticsEntity.getId() ) );
}
accountAnalyticsJpaEntity.setAccountId( accountAnalyticsEntity.getAccountId() );
accountAnalyticsJpaEntity.setUserId( accountAnalyticsEntity.getUserId() );
accountAnalyticsJpaEntity.setAnalyticsDate( accountAnalyticsEntity.getAnalyticsDate() );
accountAnalyticsJpaEntity.setBookingGroups( bookingGroupListToBookingGroupJpaEntityList( accountAnalyticsEntity.getBookingGroups() ) );
return accountAnalyticsJpaEntity;
}
@Override
public ContractJpaEntity mapToContractJpaEntity(ContractEntity contract) {
if ( contract == null ) {
return null;
}
ContractJpaEntity contractJpaEntity = new ContractJpaEntity();
if ( contract.getId() != null ) {
contractJpaEntity.setId( Long.parseLong( contract.getId() ) );
}
contractJpaEntity.setUserId( contract.getUserId() );
contractJpaEntity.setAccountId( contract.getAccountId() );
contractJpaEntity.setLogo( contract.getLogo() );
contractJpaEntity.setHomepage( contract.getHomepage() );
contractJpaEntity.setHotline( contract.getHotline() );
contractJpaEntity.setEmail( contract.getEmail() );
contractJpaEntity.setMandateReference( contract.getMandateReference() );
contractJpaEntity.setInterval( contract.getInterval() );
contractJpaEntity.setCancelled( contract.isCancelled() );
contractJpaEntity.setAmount( contract.getAmount() );
contractJpaEntity.setMainCategory( contract.getMainCategory() );
contractJpaEntity.setSubCategory( contract.getSubCategory() );
contractJpaEntity.setSpecification( contract.getSpecification() );
contractJpaEntity.setProvider( contract.getProvider() );
return contractJpaEntity;
}
@Override
public ContractJpaEntity mapToContractJpaEntity(Contract contract) {
if ( contract == null ) {
return null;
}
ContractJpaEntity contractJpaEntity = new ContractJpaEntity();
contractJpaEntity.setLogo( contract.getLogo() );
contractJpaEntity.setHomepage( contract.getHomepage() );
contractJpaEntity.setHotline( contract.getHotline() );
contractJpaEntity.setEmail( contract.getEmail() );
contractJpaEntity.setMandateReference( contract.getMandateReference() );
contractJpaEntity.setInterval( contract.getInterval() );
contractJpaEntity.setCancelled( contract.isCancelled() );
contractJpaEntity.setAmount( contract.getAmount() );
contractJpaEntity.setMainCategory( contract.getMainCategory() );
contractJpaEntity.setSubCategory( contract.getSubCategory() );
contractJpaEntity.setSpecification( contract.getSpecification() );
contractJpaEntity.setProvider( contract.getProvider() );
return contractJpaEntity;
}
@Override
public BookingGroupJpaEntity mapToBookingGroupJpaEntity(BookingGroup bookingGroup) {
if ( bookingGroup == null ) {
return null;
}
BookingGroupJpaEntity bookingGroupJpaEntity = new BookingGroupJpaEntity();
bookingGroupJpaEntity.setType( typeToType1( bookingGroup.getType() ) );
bookingGroupJpaEntity.setName( bookingGroup.getName() );
bookingGroupJpaEntity.setSalaryWage( bookingGroup.isSalaryWage() );
bookingGroupJpaEntity.setMainCategory( bookingGroup.getMainCategory() );
bookingGroupJpaEntity.setSubCategory( bookingGroup.getSubCategory() );
bookingGroupJpaEntity.setSpecification( bookingGroup.getSpecification() );
bookingGroupJpaEntity.setOtherAccount( bookingGroup.getOtherAccount() );
bookingGroupJpaEntity.setAmount( bookingGroup.getAmount() );
bookingGroupJpaEntity.setBookingPeriods( bookingPeriodListToBookingPeriodJpaEntityList( bookingGroup.getBookingPeriods() ) );
bookingGroupJpaEntity.setContract( mapToContractJpaEntity( bookingGroup.getContract() ) );
return bookingGroupJpaEntity;
}
@Override
public BookingPeriodJpaEntity mapToBookingPeriodJpaEntity(BookingPeriod bookingPeriod) {
if ( bookingPeriod == null ) {
return null;
}
BookingPeriodJpaEntity bookingPeriodJpaEntity = new BookingPeriodJpaEntity();
bookingPeriodJpaEntity.setPeriodStart( bookingPeriod.getStart() );
bookingPeriodJpaEntity.setPeriodEnd( bookingPeriod.getEnd() );
bookingPeriodJpaEntity.setAmount( bookingPeriod.getAmount() );
bookingPeriodJpaEntity.setBookings( executedBookingListToExecutedBookingJpaEntityList( bookingPeriod.getBookings() ) );
return bookingPeriodJpaEntity;
}
@Override
public ExecutedBookingJpaEntity mapToExecutedBookingJpaEntity(ExecutedBooking executedBooking) {
if ( executedBooking == null ) {
return null;
}
ExecutedBookingJpaEntity executedBookingJpaEntity = new ExecutedBookingJpaEntity();
executedBookingJpaEntity.setBookingId( executedBooking.getBookingId() );
executedBookingJpaEntity.setExecutionDate( executedBooking.getExecutionDate() );
executedBookingJpaEntity.setExecuted( executedBooking.isExecuted() );
return executedBookingJpaEntity;
}
@Override
public List mapToAnonymizedBookingJpaEntities(List bookingEntities) {
if ( bookingEntities == null ) {
return null;
}
List list = new ArrayList( bookingEntities.size() );
for ( AnonymizedBookingEntity anonymizedBookingEntity : bookingEntities ) {
list.add( anonymizedBookingEntityToAnonymizedBookingJpaEntity( anonymizedBookingEntity ) );
}
return list;
}
@Override
public BankAccessEntity mapToBankAccessEntity(BankAccessJpaEntity entity) {
if ( entity == null ) {
return null;
}
BankAccessEntity bankAccessEntity = new BankAccessEntity();
bankAccessEntity.setConsentId( entity.getConsentId() );
bankAccessEntity.setBankName( entity.getBankName() );
bankAccessEntity.setBankCode( entity.getBankCode() );
bankAccessEntity.setIban( entity.getIban() );
bankAccessEntity.setTanTransportTypes( stringListHashMapToBankApiListMap( entity.getTanTransportTypes() ) );
bankAccessEntity.setHbciPassportState( entity.getHbciPassportState() );
Map map1 = entity.getExternalIdMap();
if ( map1 != null ) {
bankAccessEntity.setExternalIdMap( new HashMap( map1 ) );
}
if ( entity.getId() != null ) {
bankAccessEntity.setId( String.valueOf( entity.getId() ) );
}
bankAccessEntity.setUserId( entity.getUserId() );
bankAccessEntity.setTemporary( entity.isTemporary() );
bankAccessEntity.setStoreBookings( entity.isStoreBookings() );
bankAccessEntity.setCategorizeBookings( entity.isCategorizeBookings() );
bankAccessEntity.setStoreAnalytics( entity.isStoreAnalytics() );
bankAccessEntity.setStoreAnonymizedBookings( entity.isStoreAnonymizedBookings() );
bankAccessEntity.setProvideDataForMachineLearning( entity.isProvideDataForMachineLearning() );
return bankAccessEntity;
}
@Override
public List mapToTanTransportTypeList(List value) {
if ( value == null ) {
return null;
}
List list = new ArrayList( value.size() );
for ( TanTransportTypeJpaEntity tanTransportTypeJpaEntity : value ) {
list.add( mapToTanTransportType( tanTransportTypeJpaEntity ) );
}
return list;
}
@Override
public TanTransportType mapToTanTransportType(TanTransportTypeJpaEntity value) {
if ( value == null ) {
return null;
}
TanTransportTypeBuilder tanTransportType = TanTransportType.builder();
tanTransportType.id( value.getId() );
tanTransportType.name( value.getName() );
tanTransportType.medium( value.getMedium() );
tanTransportType.inputInfo( value.getInputInfo() );
tanTransportType.type( value.getType() );
return tanTransportType.build();
}
@Override
public List mapToBankAccessEntities(List byUserId) {
if ( byUserId == null ) {
return null;
}
List list = new ArrayList( byUserId.size() );
for ( BankAccessJpaEntity bankAccessJpaEntity : byUserId ) {
list.add( mapToBankAccessEntity( bankAccessJpaEntity ) );
}
return list;
}
@Override
public BankAccessJpaEntity mapToBankAccessJpaEntity(BankAccessEntity bankAccess) {
if ( bankAccess == null ) {
return null;
}
BankAccessJpaEntity bankAccessJpaEntity = new BankAccessJpaEntity();
if ( bankAccess.getId() != null ) {
bankAccessJpaEntity.setId( Long.parseLong( bankAccess.getId() ) );
}
bankAccessJpaEntity.setUserId( bankAccess.getUserId() );
bankAccessJpaEntity.setConsentId( bankAccess.getConsentId() );
bankAccessJpaEntity.setTemporary( bankAccess.isTemporary() );
bankAccessJpaEntity.setStoreBookings( bankAccess.isStoreBookings() );
bankAccessJpaEntity.setCategorizeBookings( bankAccess.isCategorizeBookings() );
bankAccessJpaEntity.setStoreAnalytics( bankAccess.isStoreAnalytics() );
bankAccessJpaEntity.setStoreAnonymizedBookings( bankAccess.isStoreAnonymizedBookings() );
bankAccessJpaEntity.setProvideDataForMachineLearning( bankAccess.isProvideDataForMachineLearning() );
bankAccessJpaEntity.setBankName( bankAccess.getBankName() );
bankAccessJpaEntity.setBankCode( bankAccess.getBankCode() );
bankAccessJpaEntity.setIban( bankAccess.getIban() );
bankAccessJpaEntity.setHbciPassportState( bankAccess.getHbciPassportState() );
bankAccessJpaEntity.setTanTransportTypes( bankApiListMapToStringListHashMap( bankAccess.getTanTransportTypes() ) );
Map map = bankAccess.getExternalIdMap();
if ( map != null ) {
bankAccessJpaEntity.setExternalIdMap( new HashMap( map ) );
}
return bankAccessJpaEntity;
}
@Override
public List mapToTanTransportTypeJpaEntityList(List value) {
if ( value == null ) {
return null;
}
List list = new ArrayList( value.size() );
for ( TanTransportType tanTransportType : value ) {
list.add( tanTransportTypeToTanTransportTypeJpaEntity( tanTransportType ) );
}
return list;
}
@Override
public List mapToBankAccountEntities(List byUserId) {
if ( byUserId == null ) {
return null;
}
List list = new ArrayList( byUserId.size() );
for ( BankAccountJpaEntity bankAccountJpaEntity : byUserId ) {
list.add( mapToBankAccountEntity( bankAccountJpaEntity ) );
}
return list;
}
@Override
public BankAccountEntity mapToBankAccountEntity(BankAccountJpaEntity bankAccountJpaEntity) {
if ( bankAccountJpaEntity == null ) {
return null;
}
BankAccountEntity bankAccountEntity = new BankAccountEntity();
bankAccountEntity.setBalances( balancesReportEntityToBalancesReport( bankAccountJpaEntity.getBalances() ) );
bankAccountEntity.setCountry( bankAccountJpaEntity.getCountry() );
bankAccountEntity.setBlz( bankAccountJpaEntity.getBlz() );
bankAccountEntity.setAccountNumber( bankAccountJpaEntity.getAccountNumber() );
bankAccountEntity.setType( bankAccountJpaEntity.getType() );
bankAccountEntity.setCurrency( bankAccountJpaEntity.getCurrency() );
bankAccountEntity.setName( bankAccountJpaEntity.getName() );
bankAccountEntity.setBankName( bankAccountJpaEntity.getBankName() );
bankAccountEntity.setBic( bankAccountJpaEntity.getBic() );
bankAccountEntity.setIban( bankAccountJpaEntity.getIban() );
bankAccountEntity.setOwner( bankAccountJpaEntity.getOwner() );
Map map = bankAccountJpaEntity.getExternalIdMap();
if ( map != null ) {
bankAccountEntity.setExternalIdMap( new HashMap( map ) );
}
bankAccountEntity.setSyncStatus( bankAccountJpaEntity.getSyncStatus() );
bankAccountEntity.setLastSync( bankAccountJpaEntity.getLastSync() );
if ( bankAccountJpaEntity.getId() != null ) {
bankAccountEntity.setId( String.valueOf( bankAccountJpaEntity.getId() ) );
}
bankAccountEntity.setBankAccessId( bankAccountJpaEntity.getBankAccessId() );
bankAccountEntity.setUserId( bankAccountJpaEntity.getUserId() );
return bankAccountEntity;
}
@Override
public List mapToBankAccountJpaEntities(List bankAccounts) {
if ( bankAccounts == null ) {
return null;
}
List list = new ArrayList( bankAccounts.size() );
for ( BankAccountEntity bankAccountEntity : bankAccounts ) {
list.add( mapToBankAccountJpaEntity( bankAccountEntity ) );
}
return list;
}
@Override
public BankAccountJpaEntity mapToBankAccountJpaEntity(BankAccountEntity bankAccount) {
if ( bankAccount == null ) {
return null;
}
BankAccountJpaEntity bankAccountJpaEntity = new BankAccountJpaEntity();
bankAccountJpaEntity.setOwner( bankAccount.getOwner() );
bankAccountJpaEntity.setCountry( bankAccount.getCountry() );
bankAccountJpaEntity.setBlz( bankAccount.getBlz() );
bankAccountJpaEntity.setBankName( bankAccount.getBankName() );
bankAccountJpaEntity.setAccountNumber( bankAccount.getAccountNumber() );
bankAccountJpaEntity.setType( bankAccount.getType() );
bankAccountJpaEntity.setCurrency( bankAccount.getCurrency() );
bankAccountJpaEntity.setName( bankAccount.getName() );
bankAccountJpaEntity.setBic( bankAccount.getBic() );
bankAccountJpaEntity.setIban( bankAccount.getIban() );
bankAccountJpaEntity.setSyncStatus( bankAccount.getSyncStatus() );
bankAccountJpaEntity.setLastSync( bankAccount.getLastSync() );
if ( bankAccount.getId() != null ) {
bankAccountJpaEntity.setId( Long.parseLong( bankAccount.getId() ) );
}
bankAccountJpaEntity.setBankAccessId( bankAccount.getBankAccessId() );
bankAccountJpaEntity.setUserId( bankAccount.getUserId() );
Map map = bankAccount.getExternalIdMap();
if ( map != null ) {
bankAccountJpaEntity.setExternalIdMap( new HashMap( map ) );
}
bankAccountJpaEntity.setBalances( balancesReportToBalancesReportEntity( bankAccount.getBalances() ) );
return bankAccountJpaEntity;
}
@Override
public BankEntity mapToBankEntity(BankJpaEntity bankJpaEntity) {
if ( bankJpaEntity == null ) {
return null;
}
BankEntity bankEntity = new BankEntity();
bankEntity.setLoginSettings( bankLoginSettingsJpaEntityToBankLoginSettings( bankJpaEntity.getLoginSettings() ) );
bankEntity.setBankingUrl( bankJpaEntity.getBankingUrl() );
bankEntity.setBankCode( bankJpaEntity.getBankCode() );
bankEntity.setBankApiBankCode( bankJpaEntity.getBankApiBankCode() );
bankEntity.setBic( bankJpaEntity.getBic() );
bankEntity.setName( bankJpaEntity.getName() );
bankEntity.setBankApi( bankJpaEntity.getBankApi() );
bankEntity.setRedirectPreferred( bankJpaEntity.isRedirectPreferred() );
if ( bankJpaEntity.getId() != null ) {
bankEntity.setId( String.valueOf( bankJpaEntity.getId() ) );
}
return bankEntity;
}
@Override
public List mapToBankJpaEntities(Iterable bankEntities) {
if ( bankEntities == null ) {
return null;
}
List list = new ArrayList();
for ( BankEntity bankEntity : bankEntities ) {
list.add( mapToBankJpaEntity( bankEntity ) );
}
return list;
}
@Override
public BankJpaEntity mapToBankJpaEntity(BankEntity bank) {
if ( bank == null ) {
return null;
}
BankJpaEntity bankJpaEntity = new BankJpaEntity();
bankJpaEntity.setLoginSettings( bankLoginSettingsToBankLoginSettingsJpaEntity( bank.getLoginSettings() ) );
if ( bank.getId() != null ) {
bankJpaEntity.setId( Long.parseLong( bank.getId() ) );
}
bankJpaEntity.setBankApiBankCode( bank.getBankApiBankCode() );
bankJpaEntity.setBankingUrl( bank.getBankingUrl() );
bankJpaEntity.setBankCode( bank.getBankCode() );
bankJpaEntity.setBic( bank.getBic() );
bankJpaEntity.setName( bank.getName() );
bankJpaEntity.setBankApi( bank.getBankApi() );
bankJpaEntity.setRedirectPreferred( bank.isRedirectPreferred() );
return bankJpaEntity;
}
@Override
public BookingEntity mapToBookingEntity(BookingJpaEntity bookingJpaEntity) {
if ( bookingJpaEntity == null ) {
return null;
}
BookingEntity bookingEntity = new BookingEntity();
bookingEntity.setExternalId( bookingJpaEntity.getExternalId() );
bookingEntity.setOtherAccount( bankAccountCommonJpaEntityToBankAccount( bookingJpaEntity.getOtherAccount() ) );
bookingEntity.setValutaDate( bookingJpaEntity.getValutaDate() );
bookingEntity.setBookingDate( bookingJpaEntity.getBookingDate() );
bookingEntity.setAmount( bookingJpaEntity.getAmount() );
bookingEntity.setCurrency( bookingJpaEntity.getCurrency() );
bookingEntity.setReversal( bookingJpaEntity.isReversal() );
bookingEntity.setBalance( bookingJpaEntity.getBalance() );
bookingEntity.setCustomerRef( bookingJpaEntity.getCustomerRef() );
bookingEntity.setInstRef( bookingJpaEntity.getInstRef() );
bookingEntity.setOrigValue( bookingJpaEntity.getOrigValue() );
bookingEntity.setChargeValue( bookingJpaEntity.getChargeValue() );
bookingEntity.setText( bookingJpaEntity.getText() );
bookingEntity.setAdditional( bookingJpaEntity.getAdditional() );
bookingEntity.setPrimanota( bookingJpaEntity.getPrimanota() );
bookingEntity.setUsage( bookingJpaEntity.getUsage() );
bookingEntity.setAddkey( bookingJpaEntity.getAddkey() );
bookingEntity.setSepa( bookingJpaEntity.isSepa() );
bookingEntity.setCamt( bookingJpaEntity.isCamt() );
bookingEntity.setStandingOrder( bookingJpaEntity.isStandingOrder() );
bookingEntity.setCreditorId( bookingJpaEntity.getCreditorId() );
bookingEntity.setMandateReference( bookingJpaEntity.getMandateReference() );
bookingEntity.setBankApi( bookingJpaEntity.getBankApi() );
bookingEntity.setBookingCategory( bookingCategoryJpaEntityToBookingCategory( bookingJpaEntity.getBookingCategory() ) );
bookingEntity.setTransactionCode( bookingJpaEntity.getTransactionCode() );
bookingEntity.setId( bookingJpaEntity.getId() );
bookingEntity.setAccountId( bookingJpaEntity.getAccountId() );
bookingEntity.setUserId( bookingJpaEntity.getUserId() );
return bookingEntity;
}
@Override
public List mapToBookingEntities(List valutaDate) {
if ( valutaDate == null ) {
return null;
}
List list = new ArrayList( valutaDate.size() );
for ( BookingJpaEntity bookingJpaEntity : valutaDate ) {
list.add( mapToBookingEntity( bookingJpaEntity ) );
}
return list;
}
@Override
public List mapToBookingJpaEntities(List newEntities) {
if ( newEntities == null ) {
return null;
}
List list = new ArrayList( newEntities.size() );
for ( BookingEntity bookingEntity : newEntities ) {
list.add( bookingEntityToBookingJpaEntity( bookingEntity ) );
}
return list;
}
@Override
public BookingCategoryJpaEntity mapToBookingCategoryJpaEntity(BookingCategory bookingCategory) {
if ( bookingCategory == null ) {
return null;
}
BookingCategoryJpaEntity bookingCategoryJpaEntity = new BookingCategoryJpaEntity();
bookingCategoryJpaEntity.setLogo( bookingCategory.getLogo() );
bookingCategoryJpaEntity.setHomepage( bookingCategory.getHomepage() );
bookingCategoryJpaEntity.setHotline( bookingCategory.getHotline() );
bookingCategoryJpaEntity.setEmail( bookingCategory.getEmail() );
bookingCategoryJpaEntity.setMandateReference( bookingCategory.getMandateReference() );
bookingCategoryJpaEntity.setInterval( bookingCategory.getInterval() );
bookingCategoryJpaEntity.setCancelled( bookingCategory.isCancelled() );
bookingCategoryJpaEntity.setAmount( bookingCategory.getAmount() );
bookingCategoryJpaEntity.setMainCategory( bookingCategory.getMainCategory() );
bookingCategoryJpaEntity.setSubCategory( bookingCategory.getSubCategory() );
bookingCategoryJpaEntity.setSpecification( bookingCategory.getSpecification() );
bookingCategoryJpaEntity.setProvider( bookingCategory.getProvider() );
Set set = bookingCategory.getRules();
if ( set != null ) {
bookingCategoryJpaEntity.setRules( new ArrayList( set ) );
}
bookingCategoryJpaEntity.setReceiver( bookingCategory.getReceiver() );
Map map = bookingCategory.getCustom();
if ( map != null ) {
bookingCategoryJpaEntity.setCustom( new HashMap( map ) );
}
return bookingCategoryJpaEntity;
}
@Override
public List mapToRuleEntities(List byUserId) {
if ( byUserId == null ) {
return null;
}
List list = new ArrayList( byUserId.size() );
for ( RuleJpaEntity ruleJpaEntity : byUserId ) {
list.add( mapToRuleEntity( ruleJpaEntity ) );
}
return list;
}
@Override
public RuleEntity mapToRuleEntity(RuleJpaEntity ruleJpaEntity) {
if ( ruleJpaEntity == null ) {
return null;
}
RuleEntity ruleEntity = new RuleEntity();
ruleEntity.setRuleId( ruleJpaEntity.getRuleId() );
ruleEntity.setMainCategory( ruleJpaEntity.getMainCategory() );
ruleEntity.setSubCategory( ruleJpaEntity.getSubCategory() );
ruleEntity.setSpecification( ruleJpaEntity.getSpecification() );
ruleEntity.setSimilarityMatchType( sIMILARITY_MATCH_TYPEToSIMILARITY_MATCH_TYPE( ruleJpaEntity.getSimilarityMatchType() ) );
ruleEntity.setCreditorId( ruleJpaEntity.getCreditorId() );
ruleEntity.setExpression( ruleJpaEntity.getExpression() );
ruleEntity.setReceiver( ruleJpaEntity.getReceiver() );
ruleEntity.setRuleType( ruleJpaEntity.getRuleType() );
ruleEntity.setLogo( ruleJpaEntity.getLogo() );
ruleEntity.setHotline( ruleJpaEntity.getHotline() );
ruleEntity.setHomepage( ruleJpaEntity.getHomepage() );
ruleEntity.setEmail( ruleJpaEntity.getEmail() );
ruleEntity.setIncoming( ruleJpaEntity.isIncoming() );
ruleEntity.setId( ruleJpaEntity.getId() );
ruleEntity.setUserId( ruleJpaEntity.getUserId() );
List list = ruleJpaEntity.getSearchIndex();
if ( list != null ) {
ruleEntity.setSearchIndex( new ArrayList( list ) );
}
return ruleEntity;
}
@Override
public RuleJpaEntity mapToRuleJpaEntity(RuleEntity ruleEntity) {
if ( ruleEntity == null ) {
return null;
}
RuleJpaEntity ruleJpaEntity = new RuleJpaEntity();
ruleJpaEntity.setId( ruleEntity.getId() );
ruleJpaEntity.setUserId( ruleEntity.getUserId() );
List list = ruleEntity.getSearchIndex();
if ( list != null ) {
ruleJpaEntity.setSearchIndex( new ArrayList( list ) );
}
ruleJpaEntity.setRuleId( ruleEntity.getRuleId() );
ruleJpaEntity.setMainCategory( ruleEntity.getMainCategory() );
ruleJpaEntity.setSubCategory( ruleEntity.getSubCategory() );
ruleJpaEntity.setSpecification( ruleEntity.getSpecification() );
ruleJpaEntity.setSimilarityMatchType( sIMILARITY_MATCH_TYPEToSIMILARITY_MATCH_TYPE1( ruleEntity.getSimilarityMatchType() ) );
ruleJpaEntity.setCreditorId( ruleEntity.getCreditorId() );
ruleJpaEntity.setExpression( ruleEntity.getExpression() );
ruleJpaEntity.setReceiver( ruleEntity.getReceiver() );
ruleJpaEntity.setRuleType( ruleEntity.getRuleType() );
ruleJpaEntity.setLogo( ruleEntity.getLogo() );
ruleJpaEntity.setHotline( ruleEntity.getHotline() );
ruleJpaEntity.setHomepage( ruleEntity.getHomepage() );
ruleJpaEntity.setEmail( ruleEntity.getEmail() );
ruleJpaEntity.setIncoming( ruleEntity.isIncoming() );
return ruleJpaEntity;
}
@Override
public BookingsIndexJpaEntity mapToBookingsIndexJpaEntity(BookingsIndexEntity entity) {
if ( entity == null ) {
return null;
}
BookingsIndexJpaEntity bookingsIndexJpaEntity = new BookingsIndexJpaEntity();
if ( entity.getId() != null ) {
bookingsIndexJpaEntity.setId( Long.parseLong( entity.getId() ) );
}
bookingsIndexJpaEntity.setAccountId( entity.getAccountId() );
bookingsIndexJpaEntity.setUserId( entity.getUserId() );
Map> map = entity.getBookingIdSearchList();
if ( map != null ) {
bookingsIndexJpaEntity.setBookingIdSearchList( new HashMap>( map ) );
}
return bookingsIndexJpaEntity;
}
@Override
public BookingsIndexEntity mapToBookingsIndexEntity(BookingsIndexJpaEntity bookingsIndexJpaEntity) {
if ( bookingsIndexJpaEntity == null ) {
return null;
}
BookingsIndexEntity bookingsIndexEntity = new BookingsIndexEntity();
if ( bookingsIndexJpaEntity.getId() != null ) {
bookingsIndexEntity.setId( String.valueOf( bookingsIndexJpaEntity.getId() ) );
}
bookingsIndexEntity.setAccountId( bookingsIndexJpaEntity.getAccountId() );
bookingsIndexEntity.setUserId( bookingsIndexJpaEntity.getUserId() );
Map> map = bookingsIndexJpaEntity.getBookingIdSearchList();
if ( map != null ) {
bookingsIndexEntity.setBookingIdSearchList( new HashMap>( map ) );
}
return bookingsIndexEntity;
}
@Override
public BulkPaymentJpaEntity mapToBulkPaymentJpaEntity(BulkPaymentEntity target) {
if ( target == null ) {
return null;
}
BulkPaymentJpaEntity bulkPaymentJpaEntity = new BulkPaymentJpaEntity();
if ( target.getId() != null ) {
bulkPaymentJpaEntity.setId( Long.parseLong( target.getId() ) );
}
bulkPaymentJpaEntity.setUserId( target.getUserId() );
bulkPaymentJpaEntity.setCreatedDateTime( target.getCreatedDateTime() );
bulkPaymentJpaEntity.setTanSubmitExternal( target.getTanSubmitExternal() );
List list = target.getPayments();
if ( list != null ) {
bulkPaymentJpaEntity.setPayments( new ArrayList( list ) );
}
return bulkPaymentJpaEntity;
}
@Override
public List mapToContractEntities(List byUserIdAndAccountId) {
if ( byUserIdAndAccountId == null ) {
return null;
}
List list = new ArrayList( byUserIdAndAccountId.size() );
for ( ContractJpaEntity contractJpaEntity : byUserIdAndAccountId ) {
list.add( contractJpaEntityToContractEntity( contractJpaEntity ) );
}
return list;
}
@Override
public List mapToContractJpaEntities(List contractEntities) {
if ( contractEntities == null ) {
return null;
}
List list = new ArrayList( contractEntities.size() );
for ( ContractEntity contractEntity : contractEntities ) {
list.add( mapToContractJpaEntity( contractEntity ) );
}
return list;
}
@Override
public MlAnonymizedBookingEntity mapToMlAnonymizedBookingEntity(MlAnonymizedBookingJpaEntity mlAnonymizedBookingJpaEntity) {
if ( mlAnonymizedBookingJpaEntity == null ) {
return null;
}
MlAnonymizedBookingEntity mlAnonymizedBookingEntity = new MlAnonymizedBookingEntity();
if ( mlAnonymizedBookingJpaEntity.getId() != null ) {
mlAnonymizedBookingEntity.setId( String.valueOf( mlAnonymizedBookingJpaEntity.getId() ) );
}
mlAnonymizedBookingEntity.setUserId( mlAnonymizedBookingJpaEntity.getUserId() );
mlAnonymizedBookingEntity.setTransactionPurpose( mlAnonymizedBookingJpaEntity.getTransactionPurpose() );
mlAnonymizedBookingEntity.setAmountBin( mlAnonymizedBookingJpaEntity.getAmountBin() );
mlAnonymizedBookingEntity.setAmountRemainder( mlAnonymizedBookingJpaEntity.getAmountRemainder() );
mlAnonymizedBookingEntity.setCreditorId( mlAnonymizedBookingJpaEntity.getCreditorId() );
mlAnonymizedBookingEntity.setReferenceName( mlAnonymizedBookingJpaEntity.getReferenceName() );
mlAnonymizedBookingEntity.setExecutionDate( mlAnonymizedBookingJpaEntity.getExecutionDate() );
mlAnonymizedBookingEntity.setMainCategory( mlAnonymizedBookingJpaEntity.getMainCategory() );
mlAnonymizedBookingEntity.setSubCategory( mlAnonymizedBookingJpaEntity.getSubCategory() );
mlAnonymizedBookingEntity.setSpecification( mlAnonymizedBookingJpaEntity.getSpecification() );
return mlAnonymizedBookingEntity;
}
@Override
public List mapToMlAnonymizedBookingEntities(List byUserId) {
if ( byUserId == null ) {
return null;
}
List list = new ArrayList( byUserId.size() );
for ( MlAnonymizedBookingJpaEntity mlAnonymizedBookingJpaEntity : byUserId ) {
list.add( mapToMlAnonymizedBookingEntity( mlAnonymizedBookingJpaEntity ) );
}
return list;
}
@Override
public MlAnonymizedBookingJpaEntity mapToMlAnonymizedBookingJpaEntity(MlAnonymizedBookingEntity booking) {
if ( booking == null ) {
return null;
}
MlAnonymizedBookingJpaEntity mlAnonymizedBookingJpaEntity = new MlAnonymizedBookingJpaEntity();
if ( booking.getId() != null ) {
mlAnonymizedBookingJpaEntity.setId( Long.parseLong( booking.getId() ) );
}
mlAnonymizedBookingJpaEntity.setUserId( booking.getUserId() );
mlAnonymizedBookingJpaEntity.setTransactionPurpose( booking.getTransactionPurpose() );
mlAnonymizedBookingJpaEntity.setAmountBin( booking.getAmountBin() );
mlAnonymizedBookingJpaEntity.setAmountRemainder( booking.getAmountRemainder() );
mlAnonymizedBookingJpaEntity.setCreditorId( booking.getCreditorId() );
mlAnonymizedBookingJpaEntity.setReferenceName( booking.getReferenceName() );
mlAnonymizedBookingJpaEntity.setExecutionDate( booking.getExecutionDate() );
mlAnonymizedBookingJpaEntity.setMainCategory( booking.getMainCategory() );
mlAnonymizedBookingJpaEntity.setSubCategory( booking.getSubCategory() );
mlAnonymizedBookingJpaEntity.setSpecification( booking.getSpecification() );
return mlAnonymizedBookingJpaEntity;
}
@Override
public RawSepaTransactionEntity mapToRawSepaTransactionEntity(RawSepaTransactionJpaEntity rawSepaTransactionJpaEntity) {
if ( rawSepaTransactionJpaEntity == null ) {
return null;
}
RawSepaTransactionEntity rawSepaTransactionEntity = new RawSepaTransactionEntity();
rawSepaTransactionEntity.setOrderId( rawSepaTransactionJpaEntity.getOrderId() );
rawSepaTransactionEntity.setPaymentId( rawSepaTransactionJpaEntity.getPaymentId() );
rawSepaTransactionEntity.setProduct( rawSepaTransactionJpaEntity.getProduct() );
rawSepaTransactionEntity.setPainXml( rawSepaTransactionJpaEntity.getPainXml() );
rawSepaTransactionEntity.setService( rawSepaTransactionJpaEntity.getService() );
rawSepaTransactionEntity.setSepaTransactionType( rawSepaTransactionJpaEntity.getSepaTransactionType() );
if ( rawSepaTransactionJpaEntity.getId() != null ) {
rawSepaTransactionEntity.setId( String.valueOf( rawSepaTransactionJpaEntity.getId() ) );
}
rawSepaTransactionEntity.setUserId( rawSepaTransactionJpaEntity.getUserId() );
rawSepaTransactionEntity.setCreatedDateTime( rawSepaTransactionJpaEntity.getCreatedDateTime() );
rawSepaTransactionEntity.setTanSubmitExternal( rawSepaTransactionJpaEntity.getTanSubmitExternal() );
rawSepaTransactionEntity.setPsuAccount( rawSepaTransactionJpaEntityToBankAccount( rawSepaTransactionJpaEntity ) );
return rawSepaTransactionEntity;
}
@Override
public RawSepaTransactionJpaEntity mapToRawSepaTransactionJpaEntity(RawSepaTransactionEntity paymentEntity) {
if ( paymentEntity == null ) {
return null;
}
RawSepaTransactionJpaEntity rawSepaTransactionJpaEntity = new RawSepaTransactionJpaEntity();
rawSepaTransactionJpaEntity.setUserId( paymentEntity.getUserId() );
rawSepaTransactionJpaEntity.setCreatedDateTime( paymentEntity.getCreatedDateTime() );
rawSepaTransactionJpaEntity.setOrderId( paymentEntity.getOrderId() );
rawSepaTransactionJpaEntity.setPaymentId( paymentEntity.getPaymentId() );
rawSepaTransactionJpaEntity.setProduct( paymentEntity.getProduct() );
rawSepaTransactionJpaEntity.setTanSubmitExternal( paymentEntity.getTanSubmitExternal() );
if ( paymentEntity.getId() != null ) {
rawSepaTransactionJpaEntity.setId( Long.parseLong( paymentEntity.getId() ) );
}
rawSepaTransactionJpaEntity.setPainXml( paymentEntity.getPainXml() );
rawSepaTransactionJpaEntity.setService( paymentEntity.getService() );
rawSepaTransactionJpaEntity.setSepaTransactionType( paymentEntity.getSepaTransactionType() );
rawSepaTransactionJpaEntity.setDebtorBankAccount( rawSepaTransactionEntityToBankAccountJpaEntity( paymentEntity ) );
return rawSepaTransactionJpaEntity;
}
@Override
public SinglePaymentEntity mapToPaymentEntity(SinglePaymentJpaEntity paymentJpaEntity) {
if ( paymentJpaEntity == null ) {
return null;
}
SinglePaymentEntity singlePaymentEntity = new SinglePaymentEntity();
singlePaymentEntity.setOrderId( paymentJpaEntity.getOrderId() );
singlePaymentEntity.setPaymentId( paymentJpaEntity.getPaymentId() );
singlePaymentEntity.setProduct( paymentJpaEntity.getProduct() );
singlePaymentEntity.setReceiver( paymentJpaEntity.getReceiver() );
singlePaymentEntity.setReceiverBic( paymentJpaEntity.getReceiverBic() );
singlePaymentEntity.setReceiverIban( paymentJpaEntity.getReceiverIban() );
singlePaymentEntity.setReceiverBankCode( paymentJpaEntity.getReceiverBankCode() );
singlePaymentEntity.setReceiverAccountNumber( paymentJpaEntity.getReceiverAccountNumber() );
singlePaymentEntity.setReceiverAccountCurrency( paymentJpaEntity.getReceiverAccountCurrency() );
singlePaymentEntity.setPurpose( paymentJpaEntity.getPurpose() );
singlePaymentEntity.setPurposecode( paymentJpaEntity.getPurposecode() );
singlePaymentEntity.setAmount( paymentJpaEntity.getAmount() );
singlePaymentEntity.setCurrency( paymentJpaEntity.getCurrency() );
if ( paymentJpaEntity.getId() != null ) {
singlePaymentEntity.setId( String.valueOf( paymentJpaEntity.getId() ) );
}
singlePaymentEntity.setUserId( paymentJpaEntity.getUserId() );
singlePaymentEntity.setCreatedDateTime( paymentJpaEntity.getCreatedDateTime() );
singlePaymentEntity.setTanSubmitExternal( paymentJpaEntity.getTanSubmitExternal() );
singlePaymentEntity.setPsuAccount( singlePaymentJpaEntityToBankAccount( paymentJpaEntity ) );
return singlePaymentEntity;
}
@Override
public SinglePaymentJpaEntity mapToPaymentJpaEntity(SinglePaymentEntity paymentJpaEntity) {
if ( paymentJpaEntity == null ) {
return null;
}
SinglePaymentJpaEntity singlePaymentJpaEntity = new SinglePaymentJpaEntity();
singlePaymentJpaEntity.setUserId( paymentJpaEntity.getUserId() );
singlePaymentJpaEntity.setCreatedDateTime( paymentJpaEntity.getCreatedDateTime() );
singlePaymentJpaEntity.setOrderId( paymentJpaEntity.getOrderId() );
singlePaymentJpaEntity.setPaymentId( paymentJpaEntity.getPaymentId() );
singlePaymentJpaEntity.setProduct( paymentJpaEntity.getProduct() );
singlePaymentJpaEntity.setTanSubmitExternal( paymentJpaEntity.getTanSubmitExternal() );
if ( paymentJpaEntity.getId() != null ) {
singlePaymentJpaEntity.setId( Long.parseLong( paymentJpaEntity.getId() ) );
}
singlePaymentJpaEntity.setReceiver( paymentJpaEntity.getReceiver() );
singlePaymentJpaEntity.setReceiverBic( paymentJpaEntity.getReceiverBic() );
singlePaymentJpaEntity.setReceiverIban( paymentJpaEntity.getReceiverIban() );
singlePaymentJpaEntity.setReceiverBankCode( paymentJpaEntity.getReceiverBankCode() );
singlePaymentJpaEntity.setReceiverAccountNumber( paymentJpaEntity.getReceiverAccountNumber() );
singlePaymentJpaEntity.setReceiverAccountCurrency( paymentJpaEntity.getReceiverAccountCurrency() );
singlePaymentJpaEntity.setPurpose( paymentJpaEntity.getPurpose() );
singlePaymentJpaEntity.setPurposecode( paymentJpaEntity.getPurposecode() );
singlePaymentJpaEntity.setAmount( paymentJpaEntity.getAmount() );
singlePaymentJpaEntity.setCurrency( paymentJpaEntity.getCurrency() );
singlePaymentJpaEntity.setDebtorBankAccount( singlePaymentEntityToBankAccountJpaEntity( paymentJpaEntity ) );
return singlePaymentJpaEntity;
}
@Override
public StandingOrderJpaEntity mapToStandingOrderJpaEntity(StandingOrderEntity paymentJpaEntity) {
if ( paymentJpaEntity == null ) {
return null;
}
StandingOrderJpaEntity standingOrderJpaEntity = new StandingOrderJpaEntity();
standingOrderJpaEntity.setUserId( paymentJpaEntity.getUserId() );
standingOrderJpaEntity.setCreatedDateTime( paymentJpaEntity.getCreatedDateTime() );
standingOrderJpaEntity.setOrderId( paymentJpaEntity.getOrderId() );
standingOrderJpaEntity.setPaymentId( paymentJpaEntity.getPaymentId() );
standingOrderJpaEntity.setProduct( paymentJpaEntity.getProduct() );
standingOrderJpaEntity.setTanSubmitExternal( paymentJpaEntity.getTanSubmitExternal() );
if ( paymentJpaEntity.getId() != null ) {
standingOrderJpaEntity.setId( Long.parseLong( paymentJpaEntity.getId() ) );
}
standingOrderJpaEntity.setAccountId( paymentJpaEntity.getAccountId() );
standingOrderJpaEntity.setCycle( paymentJpaEntity.getCycle() );
standingOrderJpaEntity.setExecutionDay( paymentJpaEntity.getExecutionDay() );
standingOrderJpaEntity.setFirstExecutionDate( paymentJpaEntity.getFirstExecutionDate() );
standingOrderJpaEntity.setLastExecutionDate( paymentJpaEntity.getLastExecutionDate() );
standingOrderJpaEntity.setAmount( paymentJpaEntity.getAmount() );
standingOrderJpaEntity.setCurrency( paymentJpaEntity.getCurrency() );
standingOrderJpaEntity.setOtherAccount( bankAccountToBankAccountCommonJpaEntity( paymentJpaEntity.getOtherAccount() ) );
standingOrderJpaEntity.setUsage( paymentJpaEntity.getUsage() );
standingOrderJpaEntity.setPurposecode( paymentJpaEntity.getPurposecode() );
standingOrderJpaEntity.setDelete( paymentJpaEntity.isDelete() );
standingOrderJpaEntity.setDebtorBankAccount( standingOrderEntityToBankAccountJpaEntity( paymentJpaEntity ) );
return standingOrderJpaEntity;
}
@Override
public StandingOrderEntity mapToStandingOrderEntity(StandingOrderJpaEntity paymentJpaEntity) {
if ( paymentJpaEntity == null ) {
return null;
}
StandingOrderEntity standingOrderEntity = new StandingOrderEntity();
standingOrderEntity.setOrderId( paymentJpaEntity.getOrderId() );
standingOrderEntity.setPaymentId( paymentJpaEntity.getPaymentId() );
standingOrderEntity.setProduct( paymentJpaEntity.getProduct() );
standingOrderEntity.setCycle( paymentJpaEntity.getCycle() );
standingOrderEntity.setExecutionDay( paymentJpaEntity.getExecutionDay() );
standingOrderEntity.setFirstExecutionDate( paymentJpaEntity.getFirstExecutionDate() );
standingOrderEntity.setLastExecutionDate( paymentJpaEntity.getLastExecutionDate() );
standingOrderEntity.setAmount( paymentJpaEntity.getAmount() );
standingOrderEntity.setCurrency( paymentJpaEntity.getCurrency() );
standingOrderEntity.setOtherAccount( bankAccountCommonJpaEntityToBankAccount( paymentJpaEntity.getOtherAccount() ) );
standingOrderEntity.setUsage( paymentJpaEntity.getUsage() );
standingOrderEntity.setDelete( paymentJpaEntity.isDelete() );
standingOrderEntity.setPurposecode( paymentJpaEntity.getPurposecode() );
if ( paymentJpaEntity.getId() != null ) {
standingOrderEntity.setId( String.valueOf( paymentJpaEntity.getId() ) );
}
standingOrderEntity.setAccountId( paymentJpaEntity.getAccountId() );
standingOrderEntity.setUserId( paymentJpaEntity.getUserId() );
standingOrderEntity.setCreatedDateTime( paymentJpaEntity.getCreatedDateTime() );
standingOrderEntity.setTanSubmitExternal( paymentJpaEntity.getTanSubmitExternal() );
standingOrderEntity.setPsuAccount( standingOrderJpaEntityToBankAccount( paymentJpaEntity ) );
return standingOrderEntity;
}
@Override
public List mapToStandingOrderEntities(List byUserIdAndAccountId) {
if ( byUserIdAndAccountId == null ) {
return null;
}
List list = new ArrayList( byUserIdAndAccountId.size() );
for ( StandingOrderJpaEntity standingOrderJpaEntity : byUserIdAndAccountId ) {
list.add( mapToStandingOrderEntity( standingOrderJpaEntity ) );
}
return list;
}
@Override
public List mapToStandingOrderJpaEntities(List standingOrders) {
if ( standingOrders == null ) {
return null;
}
List list = new ArrayList( standingOrders.size() );
for ( StandingOrderEntity standingOrderEntity : standingOrders ) {
list.add( mapToStandingOrderJpaEntity( standingOrderEntity ) );
}
return list;
}
@Override
public UserEntity mapToUserEntity(UserJpaEntity userJpaEntity) {
if ( userJpaEntity == null ) {
return null;
}
UserEntity userEntity = new UserEntity();
userEntity.setId( userJpaEntity.getId() );
userEntity.setExpireUser( userJpaEntity.getExpireUser() );
userEntity.setRulesLastChangeDate( userJpaEntity.getRulesLastChangeDate() );
List list = userJpaEntity.getApiUser();
if ( list != null ) {
userEntity.setApiUser( new ArrayList( list ) );
}
return userEntity;
}
@Override
public UserJpaEntity mapToUserJpaEntity(UserEntity userEntity) {
if ( userEntity == null ) {
return null;
}
UserJpaEntity userJpaEntity = new UserJpaEntity();
userJpaEntity.setId( userEntity.getId() );
userJpaEntity.setExpireUser( userEntity.getExpireUser() );
userJpaEntity.setRulesLastChangeDate( userEntity.getRulesLastChangeDate() );
List list = userEntity.getApiUser();
if ( list != null ) {
userJpaEntity.setApiUser( new ArrayList( list ) );
}
return userJpaEntity;
}
@Override
public List mapToBankEntities(List bankJpaEntities) {
if ( bankJpaEntities == null ) {
return null;
}
List list = new ArrayList( bankJpaEntities.size() );
for ( BankJpaEntity bankJpaEntity : bankJpaEntities ) {
list.add( mapToBankEntity( bankJpaEntity ) );
}
return list;
}
@Override
public List mapToBookingsIndexEntities(List bookingsIndexJpaEntities) {
if ( bookingsIndexJpaEntities == null ) {
return null;
}
List list = new ArrayList( bookingsIndexJpaEntities.size() );
for ( BookingsIndexJpaEntity bookingsIndexJpaEntity : bookingsIndexJpaEntities ) {
list.add( mapToBookingsIndexEntity( bookingsIndexJpaEntity ) );
}
return list;
}
@Override
public ConsentEntity toConsentEntity(ConsentJpaEntity consentJpaEntity) {
if ( consentJpaEntity == null ) {
return null;
}
ConsentEntity consentEntity = new ConsentEntity();
consentEntity.setId( consentJpaEntity.getId() );
consentEntity.setAuthorisationId( consentJpaEntity.getAuthorisationId() );
consentEntity.setRedirectId( consentJpaEntity.getRedirectId() );
consentEntity.setBankApi( consentJpaEntity.getBankApi() );
consentEntity.setPsuAccountIban( consentJpaEntity.getPsuAccountIban() );
consentEntity.setBankApiConsentData( consentJpaEntity.getBankApiConsentData() );
return consentEntity;
}
@Override
public ConsentJpaEntity toConsentJpaEntity(ConsentEntity consentEntity) {
if ( consentEntity == null ) {
return null;
}
ConsentJpaEntity consentJpaEntity = new ConsentJpaEntity();
consentJpaEntity.setId( consentEntity.getId() );
consentJpaEntity.setAuthorisationId( consentEntity.getAuthorisationId() );
consentJpaEntity.setRedirectId( consentEntity.getRedirectId() );
consentJpaEntity.setBankApi( consentEntity.getBankApi() );
consentJpaEntity.setPsuAccountIban( consentEntity.getPsuAccountIban() );
consentJpaEntity.setBankApiConsentData( consentEntity.getBankApiConsentData() );
return consentJpaEntity;
}
protected de.adorsys.multibanking.domain.BookingGroup.Type typeToType(Type type) {
if ( type == null ) {
return null;
}
de.adorsys.multibanking.domain.BookingGroup.Type type1;
switch ( type ) {
case STANDING_ORDER: type1 = de.adorsys.multibanking.domain.BookingGroup.Type.STANDING_ORDER;
break;
case RECURRENT_INCOME: type1 = de.adorsys.multibanking.domain.BookingGroup.Type.RECURRENT_INCOME;
break;
case RECURRENT_SEPA: type1 = de.adorsys.multibanking.domain.BookingGroup.Type.RECURRENT_SEPA;
break;
case RECURRENT_NONSEPA: type1 = de.adorsys.multibanking.domain.BookingGroup.Type.RECURRENT_NONSEPA;
break;
case CUSTOM: type1 = de.adorsys.multibanking.domain.BookingGroup.Type.CUSTOM;
break;
case OTHER_INCOME: type1 = de.adorsys.multibanking.domain.BookingGroup.Type.OTHER_INCOME;
break;
case OTHER_EXPENSES: type1 = de.adorsys.multibanking.domain.BookingGroup.Type.OTHER_EXPENSES;
break;
default: throw new IllegalArgumentException( "Unexpected enum constant: " + type );
}
return type1;
}
protected List bookingPeriodJpaEntityListToBookingPeriodList(List list) {
if ( list == null ) {
return null;
}
List list1 = new ArrayList( list.size() );
for ( BookingPeriodJpaEntity bookingPeriodJpaEntity : list ) {
list1.add( mapToBookingPeriodEntity( bookingPeriodJpaEntity ) );
}
return list1;
}
protected Contract contractJpaEntityToContract(ContractJpaEntity contractJpaEntity) {
if ( contractJpaEntity == null ) {
return null;
}
Contract contract = new Contract();
contract.setLogo( contractJpaEntity.getLogo() );
contract.setHomepage( contractJpaEntity.getHomepage() );
contract.setHotline( contractJpaEntity.getHotline() );
contract.setEmail( contractJpaEntity.getEmail() );
contract.setMandateReference( contractJpaEntity.getMandateReference() );
contract.setInterval( contractJpaEntity.getInterval() );
contract.setCancelled( contractJpaEntity.isCancelled() );
contract.setAmount( contractJpaEntity.getAmount() );
contract.setMainCategory( contractJpaEntity.getMainCategory() );
contract.setSubCategory( contractJpaEntity.getSubCategory() );
contract.setSpecification( contractJpaEntity.getSpecification() );
contract.setProvider( contractJpaEntity.getProvider() );
return contract;
}
protected BookingGroup bookingGroupJpaEntityToBookingGroup(BookingGroupJpaEntity bookingGroupJpaEntity) {
if ( bookingGroupJpaEntity == null ) {
return null;
}
BookingGroupBuilder bookingGroup = BookingGroup.builder();
bookingGroup.type( typeToType( bookingGroupJpaEntity.getType() ) );
bookingGroup.name( bookingGroupJpaEntity.getName() );
bookingGroup.salaryWage( bookingGroupJpaEntity.isSalaryWage() );
bookingGroup.mainCategory( bookingGroupJpaEntity.getMainCategory() );
bookingGroup.subCategory( bookingGroupJpaEntity.getSubCategory() );
bookingGroup.specification( bookingGroupJpaEntity.getSpecification() );
bookingGroup.otherAccount( bookingGroupJpaEntity.getOtherAccount() );
bookingGroup.amount( bookingGroupJpaEntity.getAmount() );
bookingGroup.bookingPeriods( bookingPeriodJpaEntityListToBookingPeriodList( bookingGroupJpaEntity.getBookingPeriods() ) );
bookingGroup.contract( contractJpaEntityToContract( bookingGroupJpaEntity.getContract() ) );
return bookingGroup.build();
}
protected List bookingGroupJpaEntityListToBookingGroupList(List list) {
if ( list == null ) {
return null;
}
List list1 = new ArrayList( list.size() );
for ( BookingGroupJpaEntity bookingGroupJpaEntity : list ) {
list1.add( bookingGroupJpaEntityToBookingGroup( bookingGroupJpaEntity ) );
}
return list1;
}
protected ExecutedBooking executedBookingJpaEntityToExecutedBooking(ExecutedBookingJpaEntity executedBookingJpaEntity) {
if ( executedBookingJpaEntity == null ) {
return null;
}
ExecutedBooking executedBooking = new ExecutedBooking();
executedBooking.setBookingId( executedBookingJpaEntity.getBookingId() );
executedBooking.setExecutionDate( executedBookingJpaEntity.getExecutionDate() );
executedBooking.setExecuted( executedBookingJpaEntity.isExecuted() );
return executedBooking;
}
protected List executedBookingJpaEntityListToExecutedBookingList(List list) {
if ( list == null ) {
return null;
}
List list1 = new ArrayList( list.size() );
for ( ExecutedBookingJpaEntity executedBookingJpaEntity : list ) {
list1.add( executedBookingJpaEntityToExecutedBooking( executedBookingJpaEntity ) );
}
return list1;
}
protected List bookingGroupListToBookingGroupJpaEntityList(List list) {
if ( list == null ) {
return null;
}
List list1 = new ArrayList( list.size() );
for ( BookingGroup bookingGroup : list ) {
list1.add( mapToBookingGroupJpaEntity( bookingGroup ) );
}
return list1;
}
protected Type typeToType1(de.adorsys.multibanking.domain.BookingGroup.Type type) {
if ( type == null ) {
return null;
}
Type type1;
switch ( type ) {
case STANDING_ORDER: type1 = Type.STANDING_ORDER;
break;
case RECURRENT_INCOME: type1 = Type.RECURRENT_INCOME;
break;
case RECURRENT_SEPA: type1 = Type.RECURRENT_SEPA;
break;
case RECURRENT_NONSEPA: type1 = Type.RECURRENT_NONSEPA;
break;
case CUSTOM: type1 = Type.CUSTOM;
break;
case OTHER_INCOME: type1 = Type.OTHER_INCOME;
break;
case OTHER_EXPENSES: type1 = Type.OTHER_EXPENSES;
break;
default: throw new IllegalArgumentException( "Unexpected enum constant: " + type );
}
return type1;
}
protected List bookingPeriodListToBookingPeriodJpaEntityList(List list) {
if ( list == null ) {
return null;
}
List list1 = new ArrayList( list.size() );
for ( BookingPeriod bookingPeriod : list ) {
list1.add( mapToBookingPeriodJpaEntity( bookingPeriod ) );
}
return list1;
}
protected List executedBookingListToExecutedBookingJpaEntityList(List list) {
if ( list == null ) {
return null;
}
List list1 = new ArrayList( list.size() );
for ( ExecutedBooking executedBooking : list ) {
list1.add( mapToExecutedBookingJpaEntity( executedBooking ) );
}
return list1;
}
protected AnonymizedBookingJpaEntity anonymizedBookingEntityToAnonymizedBookingJpaEntity(AnonymizedBookingEntity anonymizedBookingEntity) {
if ( anonymizedBookingEntity == null ) {
return null;
}
AnonymizedBookingJpaEntity anonymizedBookingJpaEntity = new AnonymizedBookingJpaEntity();
if ( anonymizedBookingEntity.getId() != null ) {
anonymizedBookingJpaEntity.setId( Long.parseLong( anonymizedBookingEntity.getId() ) );
}
anonymizedBookingJpaEntity.setCreditorId( anonymizedBookingEntity.getCreditorId() );
anonymizedBookingJpaEntity.setAmount( anonymizedBookingEntity.getAmount() );
anonymizedBookingJpaEntity.setPurpose( anonymizedBookingEntity.getPurpose() );
return anonymizedBookingJpaEntity;
}
protected Map> stringListHashMapToBankApiListMap(HashMap> hashMap) {
if ( hashMap == null ) {
return null;
}
Map> map = new HashMap>( Math.max( (int) ( hashMap.size() / .75f ) + 1, 16 ) );
for ( java.util.Map.Entry> entry : hashMap.entrySet() ) {
BankApi key = Enum.valueOf( BankApi.class, entry.getKey() );
List value = mapToTanTransportTypeList( entry.getValue() );
map.put( key, value );
}
return map;
}
protected HashMap> bankApiListMapToStringListHashMap(Map> map) {
if ( map == null ) {
return null;
}
HashMap> hashMap = new HashMap>();
for ( java.util.Map.Entry> entry : map.entrySet() ) {
String key = entry.getKey().name();
List value = mapToTanTransportTypeJpaEntityList( entry.getValue() );
hashMap.put( key, value );
}
return hashMap;
}
protected TanTransportTypeJpaEntity tanTransportTypeToTanTransportTypeJpaEntity(TanTransportType tanTransportType) {
if ( tanTransportType == null ) {
return null;
}
TanTransportTypeJpaEntity tanTransportTypeJpaEntity = new TanTransportTypeJpaEntity();
tanTransportTypeJpaEntity.setId( tanTransportType.getId() );
tanTransportTypeJpaEntity.setName( tanTransportType.getName() );
tanTransportTypeJpaEntity.setMedium( tanTransportType.getMedium() );
tanTransportTypeJpaEntity.setInputInfo( tanTransportType.getInputInfo() );
tanTransportTypeJpaEntity.setType( tanTransportType.getType() );
return tanTransportTypeJpaEntity;
}
protected Balance balanceEntityToBalance(BalanceEntity balanceEntity) {
if ( balanceEntity == null ) {
return null;
}
BalanceBuilder balance = Balance.builder();
balance.date( balanceEntity.getDate() );
balance.amount( balanceEntity.getAmount() );
balance.currency( balanceEntity.getCurrency() );
return balance.build();
}
protected BalancesReport balancesReportEntityToBalancesReport(BalancesReportEntity balancesReportEntity) {
if ( balancesReportEntity == null ) {
return null;
}
BalancesReport balancesReport = new BalancesReport();
balancesReport.setReadyBalance( balanceEntityToBalance( balancesReportEntity.getReadyBalance() ) );
balancesReport.setUnreadyBalance( balanceEntityToBalance( balancesReportEntity.getUnreadyBalance() ) );
balancesReport.setCreditBalance( balanceEntityToBalance( balancesReportEntity.getCreditBalance() ) );
balancesReport.setAvailableBalance( balanceEntityToBalance( balancesReportEntity.getAvailableBalance() ) );
balancesReport.setUsedBalance( balanceEntityToBalance( balancesReportEntity.getUsedBalance() ) );
return balancesReport;
}
protected BalanceEntity balanceToBalanceEntity(Balance balance) {
if ( balance == null ) {
return null;
}
BalanceEntity balanceEntity = new BalanceEntity();
balanceEntity.setDate( balance.getDate() );
balanceEntity.setAmount( balance.getAmount() );
balanceEntity.setCurrency( balance.getCurrency() );
return balanceEntity;
}
protected BalancesReportEntity balancesReportToBalancesReportEntity(BalancesReport balancesReport) {
if ( balancesReport == null ) {
return null;
}
BalancesReportEntity balancesReportEntity = new BalancesReportEntity();
balancesReportEntity.setReadyBalance( balanceToBalanceEntity( balancesReport.getReadyBalance() ) );
balancesReportEntity.setUnreadyBalance( balanceToBalanceEntity( balancesReport.getUnreadyBalance() ) );
balancesReportEntity.setCreditBalance( balanceToBalanceEntity( balancesReport.getCreditBalance() ) );
balancesReportEntity.setAvailableBalance( balanceToBalanceEntity( balancesReport.getAvailableBalance() ) );
balancesReportEntity.setUsedBalance( balanceToBalanceEntity( balancesReport.getUsedBalance() ) );
return balancesReportEntity;
}
protected BankLoginCredentialInfo bankLoginCredentialInfoJpaEntityToBankLoginCredentialInfo(BankLoginCredentialInfoJpaEntity bankLoginCredentialInfoJpaEntity) {
if ( bankLoginCredentialInfoJpaEntity == null ) {
return null;
}
BankLoginCredentialInfo bankLoginCredentialInfo = new BankLoginCredentialInfo();
bankLoginCredentialInfo.setLabel( bankLoginCredentialInfoJpaEntity.getLabel() );
bankLoginCredentialInfo.setFieldName( bankLoginCredentialInfoJpaEntity.getFieldName() );
bankLoginCredentialInfo.setMasked( bankLoginCredentialInfoJpaEntity.isMasked() );
bankLoginCredentialInfo.setOptional( bankLoginCredentialInfoJpaEntity.isOptional() );
return bankLoginCredentialInfo;
}
protected List bankLoginCredentialInfoJpaEntityListToBankLoginCredentialInfoList(List list) {
if ( list == null ) {
return null;
}
List list1 = new ArrayList( list.size() );
for ( BankLoginCredentialInfoJpaEntity bankLoginCredentialInfoJpaEntity : list ) {
list1.add( bankLoginCredentialInfoJpaEntityToBankLoginCredentialInfo( bankLoginCredentialInfoJpaEntity ) );
}
return list1;
}
protected BankLoginSettings bankLoginSettingsJpaEntityToBankLoginSettings(BankLoginSettingsJpaEntity bankLoginSettingsJpaEntity) {
if ( bankLoginSettingsJpaEntity == null ) {
return null;
}
BankLoginSettings bankLoginSettings = new BankLoginSettings();
bankLoginSettings.setAuth_type( bankLoginSettingsJpaEntity.getAuthType() );
bankLoginSettings.setIcon( bankLoginSettingsJpaEntity.getIcon() );
bankLoginSettings.setCredentials( bankLoginCredentialInfoJpaEntityListToBankLoginCredentialInfoList( bankLoginSettingsJpaEntity.getCredentials() ) );
bankLoginSettings.setAdvice( bankLoginSettingsJpaEntity.getAdvice() );
return bankLoginSettings;
}
protected BankLoginCredentialInfoJpaEntity bankLoginCredentialInfoToBankLoginCredentialInfoJpaEntity(BankLoginCredentialInfo bankLoginCredentialInfo) {
if ( bankLoginCredentialInfo == null ) {
return null;
}
BankLoginCredentialInfoJpaEntity bankLoginCredentialInfoJpaEntity = new BankLoginCredentialInfoJpaEntity();
bankLoginCredentialInfoJpaEntity.setLabel( bankLoginCredentialInfo.getLabel() );
bankLoginCredentialInfoJpaEntity.setFieldName( bankLoginCredentialInfo.getFieldName() );
bankLoginCredentialInfoJpaEntity.setMasked( bankLoginCredentialInfo.isMasked() );
bankLoginCredentialInfoJpaEntity.setOptional( bankLoginCredentialInfo.isOptional() );
return bankLoginCredentialInfoJpaEntity;
}
protected List bankLoginCredentialInfoListToBankLoginCredentialInfoJpaEntityList(List list) {
if ( list == null ) {
return null;
}
List list1 = new ArrayList( list.size() );
for ( BankLoginCredentialInfo bankLoginCredentialInfo : list ) {
list1.add( bankLoginCredentialInfoToBankLoginCredentialInfoJpaEntity( bankLoginCredentialInfo ) );
}
return list1;
}
protected BankLoginSettingsJpaEntity bankLoginSettingsToBankLoginSettingsJpaEntity(BankLoginSettings bankLoginSettings) {
if ( bankLoginSettings == null ) {
return null;
}
BankLoginSettingsJpaEntity bankLoginSettingsJpaEntity = new BankLoginSettingsJpaEntity();
bankLoginSettingsJpaEntity.setAuthType( bankLoginSettings.getAuth_type() );
bankLoginSettingsJpaEntity.setIcon( bankLoginSettings.getIcon() );
bankLoginSettingsJpaEntity.setCredentials( bankLoginCredentialInfoListToBankLoginCredentialInfoJpaEntityList( bankLoginSettings.getCredentials() ) );
bankLoginSettingsJpaEntity.setAdvice( bankLoginSettings.getAdvice() );
return bankLoginSettingsJpaEntity;
}
protected BankAccount bankAccountCommonJpaEntityToBankAccount(BankAccountCommonJpaEntity bankAccountCommonJpaEntity) {
if ( bankAccountCommonJpaEntity == null ) {
return null;
}
BankAccount bankAccount = new BankAccount();
bankAccount.setCountry( bankAccountCommonJpaEntity.getCountry() );
bankAccount.setBlz( bankAccountCommonJpaEntity.getBlz() );
bankAccount.setAccountNumber( bankAccountCommonJpaEntity.getAccountNumber() );
bankAccount.setType( bankAccountCommonJpaEntity.getType() );
bankAccount.setCurrency( bankAccountCommonJpaEntity.getCurrency() );
bankAccount.setName( bankAccountCommonJpaEntity.getName() );
bankAccount.setBankName( bankAccountCommonJpaEntity.getBankName() );
bankAccount.setBic( bankAccountCommonJpaEntity.getBic() );
bankAccount.setIban( bankAccountCommonJpaEntity.getIban() );
bankAccount.setOwner( bankAccountCommonJpaEntity.getOwner() );
bankAccount.setSyncStatus( bankAccountCommonJpaEntity.getSyncStatus() );
bankAccount.setLastSync( bankAccountCommonJpaEntity.getLastSync() );
return bankAccount;
}
protected BookingCategory bookingCategoryJpaEntityToBookingCategory(BookingCategoryJpaEntity bookingCategoryJpaEntity) {
if ( bookingCategoryJpaEntity == null ) {
return null;
}
BookingCategory bookingCategory = new BookingCategory();
bookingCategory.setLogo( bookingCategoryJpaEntity.getLogo() );
bookingCategory.setHomepage( bookingCategoryJpaEntity.getHomepage() );
bookingCategory.setHotline( bookingCategoryJpaEntity.getHotline() );
bookingCategory.setEmail( bookingCategoryJpaEntity.getEmail() );
bookingCategory.setMandateReference( bookingCategoryJpaEntity.getMandateReference() );
bookingCategory.setInterval( bookingCategoryJpaEntity.getInterval() );
bookingCategory.setCancelled( bookingCategoryJpaEntity.isCancelled() );
bookingCategory.setAmount( bookingCategoryJpaEntity.getAmount() );
bookingCategory.setMainCategory( bookingCategoryJpaEntity.getMainCategory() );
bookingCategory.setSubCategory( bookingCategoryJpaEntity.getSubCategory() );
bookingCategory.setSpecification( bookingCategoryJpaEntity.getSpecification() );
bookingCategory.setProvider( bookingCategoryJpaEntity.getProvider() );
List list = bookingCategoryJpaEntity.getRules();
if ( list != null ) {
bookingCategory.setRules( new HashSet( list ) );
}
bookingCategory.setReceiver( bookingCategoryJpaEntity.getReceiver() );
Map map = bookingCategoryJpaEntity.getCustom();
if ( map != null ) {
bookingCategory.setCustom( new HashMap( map ) );
}
return bookingCategory;
}
protected BankAccountCommonJpaEntity bankAccountToBankAccountCommonJpaEntity(BankAccount bankAccount) {
if ( bankAccount == null ) {
return null;
}
BankAccountCommonJpaEntity bankAccountCommonJpaEntity = new BankAccountCommonJpaEntity();
bankAccountCommonJpaEntity.setOwner( bankAccount.getOwner() );
bankAccountCommonJpaEntity.setCountry( bankAccount.getCountry() );
bankAccountCommonJpaEntity.setBlz( bankAccount.getBlz() );
bankAccountCommonJpaEntity.setBankName( bankAccount.getBankName() );
bankAccountCommonJpaEntity.setAccountNumber( bankAccount.getAccountNumber() );
bankAccountCommonJpaEntity.setType( bankAccount.getType() );
bankAccountCommonJpaEntity.setCurrency( bankAccount.getCurrency() );
bankAccountCommonJpaEntity.setName( bankAccount.getName() );
bankAccountCommonJpaEntity.setBic( bankAccount.getBic() );
bankAccountCommonJpaEntity.setIban( bankAccount.getIban() );
bankAccountCommonJpaEntity.setSyncStatus( bankAccount.getSyncStatus() );
bankAccountCommonJpaEntity.setLastSync( bankAccount.getLastSync() );
return bankAccountCommonJpaEntity;
}
protected BookingJpaEntity bookingEntityToBookingJpaEntity(BookingEntity bookingEntity) {
if ( bookingEntity == null ) {
return null;
}
BookingJpaEntity bookingJpaEntity = new BookingJpaEntity();
bookingJpaEntity.setId( bookingEntity.getId() );
bookingJpaEntity.setAccountId( bookingEntity.getAccountId() );
bookingJpaEntity.setUserId( bookingEntity.getUserId() );
bookingJpaEntity.setExternalId( bookingEntity.getExternalId() );
bookingJpaEntity.setOtherAccount( bankAccountToBankAccountCommonJpaEntity( bookingEntity.getOtherAccount() ) );
bookingJpaEntity.setValutaDate( bookingEntity.getValutaDate() );
bookingJpaEntity.setBookingDate( bookingEntity.getBookingDate() );
bookingJpaEntity.setAmount( bookingEntity.getAmount() );
bookingJpaEntity.setCurrency( bookingEntity.getCurrency() );
bookingJpaEntity.setReversal( bookingEntity.isReversal() );
bookingJpaEntity.setBalance( bookingEntity.getBalance() );
bookingJpaEntity.setCustomerRef( bookingEntity.getCustomerRef() );
bookingJpaEntity.setInstRef( bookingEntity.getInstRef() );
bookingJpaEntity.setOrigValue( bookingEntity.getOrigValue() );
bookingJpaEntity.setChargeValue( bookingEntity.getChargeValue() );
bookingJpaEntity.setText( bookingEntity.getText() );
bookingJpaEntity.setAdditional( bookingEntity.getAdditional() );
bookingJpaEntity.setPrimanota( bookingEntity.getPrimanota() );
bookingJpaEntity.setUsage( bookingEntity.getUsage() );
bookingJpaEntity.setAddkey( bookingEntity.getAddkey() );
bookingJpaEntity.setSepa( bookingEntity.isSepa() );
bookingJpaEntity.setStandingOrder( bookingEntity.isStandingOrder() );
bookingJpaEntity.setCreditorId( bookingEntity.getCreditorId() );
bookingJpaEntity.setMandateReference( bookingEntity.getMandateReference() );
bookingJpaEntity.setBankApi( bookingEntity.getBankApi() );
bookingJpaEntity.setBookingCategory( mapToBookingCategoryJpaEntity( bookingEntity.getBookingCategory() ) );
bookingJpaEntity.setTransactionCode( bookingEntity.getTransactionCode() );
bookingJpaEntity.setCamt( bookingEntity.isCamt() );
return bookingJpaEntity;
}
protected SIMILARITY_MATCH_TYPE sIMILARITY_MATCH_TYPEToSIMILARITY_MATCH_TYPE(de.adorsys.multibanking.jpa.entity.RuleJpaEntity.SIMILARITY_MATCH_TYPE sIMILARITY_MATCH_TYPE) {
if ( sIMILARITY_MATCH_TYPE == null ) {
return null;
}
SIMILARITY_MATCH_TYPE sIMILARITY_MATCH_TYPE1;
switch ( sIMILARITY_MATCH_TYPE ) {
case IBAN: sIMILARITY_MATCH_TYPE1 = SIMILARITY_MATCH_TYPE.IBAN;
break;
case REFERENCE_NAME: sIMILARITY_MATCH_TYPE1 = SIMILARITY_MATCH_TYPE.REFERENCE_NAME;
break;
case PURPOSE: sIMILARITY_MATCH_TYPE1 = SIMILARITY_MATCH_TYPE.PURPOSE;
break;
default: throw new IllegalArgumentException( "Unexpected enum constant: " + sIMILARITY_MATCH_TYPE );
}
return sIMILARITY_MATCH_TYPE1;
}
protected de.adorsys.multibanking.jpa.entity.RuleJpaEntity.SIMILARITY_MATCH_TYPE sIMILARITY_MATCH_TYPEToSIMILARITY_MATCH_TYPE1(SIMILARITY_MATCH_TYPE sIMILARITY_MATCH_TYPE) {
if ( sIMILARITY_MATCH_TYPE == null ) {
return null;
}
de.adorsys.multibanking.jpa.entity.RuleJpaEntity.SIMILARITY_MATCH_TYPE sIMILARITY_MATCH_TYPE1;
switch ( sIMILARITY_MATCH_TYPE ) {
case IBAN: sIMILARITY_MATCH_TYPE1 = de.adorsys.multibanking.jpa.entity.RuleJpaEntity.SIMILARITY_MATCH_TYPE.IBAN;
break;
case REFERENCE_NAME: sIMILARITY_MATCH_TYPE1 = de.adorsys.multibanking.jpa.entity.RuleJpaEntity.SIMILARITY_MATCH_TYPE.REFERENCE_NAME;
break;
case PURPOSE: sIMILARITY_MATCH_TYPE1 = de.adorsys.multibanking.jpa.entity.RuleJpaEntity.SIMILARITY_MATCH_TYPE.PURPOSE;
break;
default: throw new IllegalArgumentException( "Unexpected enum constant: " + sIMILARITY_MATCH_TYPE );
}
return sIMILARITY_MATCH_TYPE1;
}
protected ContractEntity contractJpaEntityToContractEntity(ContractJpaEntity contractJpaEntity) {
if ( contractJpaEntity == null ) {
return null;
}
ContractEntity contractEntity = new ContractEntity();
contractEntity.setLogo( contractJpaEntity.getLogo() );
contractEntity.setHomepage( contractJpaEntity.getHomepage() );
contractEntity.setHotline( contractJpaEntity.getHotline() );
contractEntity.setEmail( contractJpaEntity.getEmail() );
contractEntity.setMandateReference( contractJpaEntity.getMandateReference() );
contractEntity.setInterval( contractJpaEntity.getInterval() );
contractEntity.setCancelled( contractJpaEntity.isCancelled() );
contractEntity.setAmount( contractJpaEntity.getAmount() );
contractEntity.setMainCategory( contractJpaEntity.getMainCategory() );
contractEntity.setSubCategory( contractJpaEntity.getSubCategory() );
contractEntity.setSpecification( contractJpaEntity.getSpecification() );
contractEntity.setProvider( contractJpaEntity.getProvider() );
if ( contractJpaEntity.getId() != null ) {
contractEntity.setId( String.valueOf( contractJpaEntity.getId() ) );
}
contractEntity.setUserId( contractJpaEntity.getUserId() );
contractEntity.setAccountId( contractJpaEntity.getAccountId() );
return contractEntity;
}
protected BankAccount rawSepaTransactionJpaEntityToBankAccount(RawSepaTransactionJpaEntity rawSepaTransactionJpaEntity) {
if ( rawSepaTransactionJpaEntity == null ) {
return null;
}
BankAccount bankAccount = new BankAccount();
return bankAccount;
}
protected BankAccountJpaEntity rawSepaTransactionEntityToBankAccountJpaEntity(RawSepaTransactionEntity rawSepaTransactionEntity) {
if ( rawSepaTransactionEntity == null ) {
return null;
}
BankAccountJpaEntity bankAccountJpaEntity = new BankAccountJpaEntity();
return bankAccountJpaEntity;
}
protected BankAccount singlePaymentJpaEntityToBankAccount(SinglePaymentJpaEntity singlePaymentJpaEntity) {
if ( singlePaymentJpaEntity == null ) {
return null;
}
BankAccount bankAccount = new BankAccount();
return bankAccount;
}
protected BankAccountJpaEntity singlePaymentEntityToBankAccountJpaEntity(SinglePaymentEntity singlePaymentEntity) {
if ( singlePaymentEntity == null ) {
return null;
}
BankAccountJpaEntity bankAccountJpaEntity = new BankAccountJpaEntity();
return bankAccountJpaEntity;
}
protected BankAccountJpaEntity standingOrderEntityToBankAccountJpaEntity(StandingOrderEntity standingOrderEntity) {
if ( standingOrderEntity == null ) {
return null;
}
BankAccountJpaEntity bankAccountJpaEntity = new BankAccountJpaEntity();
return bankAccountJpaEntity;
}
protected BankAccount standingOrderJpaEntityToBankAccount(StandingOrderJpaEntity standingOrderJpaEntity) {
if ( standingOrderJpaEntity == null ) {
return null;
}
BankAccount bankAccount = new BankAccount();
return bankAccount;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy