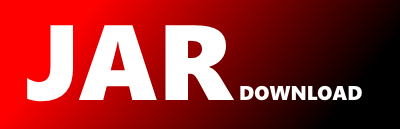
de.adorsys.multibanking.mongo.mapper.MongoEntityMapperImpl Maven / Gradle / Ivy
package de.adorsys.multibanking.mongo.mapper;
import de.adorsys.multibanking.domain.AccountAnalyticsEntity;
import de.adorsys.multibanking.domain.AnonymizedBookingEntity;
import de.adorsys.multibanking.domain.BankAccessEntity;
import de.adorsys.multibanking.domain.BankAccountEntity;
import de.adorsys.multibanking.domain.BankApi;
import de.adorsys.multibanking.domain.BankApiUser;
import de.adorsys.multibanking.domain.BankEntity;
import de.adorsys.multibanking.domain.BookingEntity;
import de.adorsys.multibanking.domain.BookingGroup;
import de.adorsys.multibanking.domain.BookingsIndexEntity;
import de.adorsys.multibanking.domain.BulkPaymentEntity;
import de.adorsys.multibanking.domain.ConsentEntity;
import de.adorsys.multibanking.domain.ContractEntity;
import de.adorsys.multibanking.domain.MlAnonymizedBookingEntity;
import de.adorsys.multibanking.domain.RawSepaTransactionEntity;
import de.adorsys.multibanking.domain.RuleEntity;
import de.adorsys.multibanking.domain.SinglePaymentEntity;
import de.adorsys.multibanking.domain.StandingOrderEntity;
import de.adorsys.multibanking.domain.TanTransportType;
import de.adorsys.multibanking.domain.UserEntity;
import de.adorsys.multibanking.domain.transaction.SinglePayment;
import de.adorsys.multibanking.mongo.entity.AccountAnalyticsMongoEntity;
import de.adorsys.multibanking.mongo.entity.AnonymizedBookingMongoEntity;
import de.adorsys.multibanking.mongo.entity.BankAccessMongoEntity;
import de.adorsys.multibanking.mongo.entity.BankAccountMongoEntity;
import de.adorsys.multibanking.mongo.entity.BankMongoEntity;
import de.adorsys.multibanking.mongo.entity.BookingMongoEntity;
import de.adorsys.multibanking.mongo.entity.BookingsIndexMongoEntity;
import de.adorsys.multibanking.mongo.entity.BulkPaymentMongoEntity;
import de.adorsys.multibanking.mongo.entity.ConsentMongoEntity;
import de.adorsys.multibanking.mongo.entity.ContractMongoEntity;
import de.adorsys.multibanking.mongo.entity.MlAnonymizedBookingMongoEntity;
import de.adorsys.multibanking.mongo.entity.PaymentMongoEntity;
import de.adorsys.multibanking.mongo.entity.RawSepaTransactionMongoEntity;
import de.adorsys.multibanking.mongo.entity.RuleMongoEntity;
import de.adorsys.multibanking.mongo.entity.StandingOrderMongoEntity;
import de.adorsys.multibanking.mongo.entity.UserMongoEntity;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.annotation.Generated;
import org.springframework.stereotype.Component;
@Generated(
value = "org.mapstruct.ap.MappingProcessor",
date = "2019-08-20T13:12:24+0000",
comments = "version: 1.3.0.Final, compiler: javac, environment: Java 1.8.0_151 (Oracle Corporation)"
)
@Component
public class MongoEntityMapperImpl implements MongoEntityMapper {
@Override
public AccountAnalyticsEntity mapToAccountAnalyticsEntity(AccountAnalyticsMongoEntity accountAnalyticsEntity) {
if ( accountAnalyticsEntity == null ) {
return null;
}
AccountAnalyticsEntity accountAnalyticsEntity1 = new AccountAnalyticsEntity();
accountAnalyticsEntity1.setId( accountAnalyticsEntity.getId() );
accountAnalyticsEntity1.setAccountId( accountAnalyticsEntity.getAccountId() );
accountAnalyticsEntity1.setUserId( accountAnalyticsEntity.getUserId() );
accountAnalyticsEntity1.setAnalyticsDate( accountAnalyticsEntity.getAnalyticsDate() );
List list = accountAnalyticsEntity.getBookingGroups();
if ( list != null ) {
accountAnalyticsEntity1.setBookingGroups( new ArrayList( list ) );
}
return accountAnalyticsEntity1;
}
@Override
public AccountAnalyticsMongoEntity mapToAccountAnalyticsMongoEntity(AccountAnalyticsEntity accountAnalyticsEntity) {
if ( accountAnalyticsEntity == null ) {
return null;
}
AccountAnalyticsMongoEntity accountAnalyticsMongoEntity = new AccountAnalyticsMongoEntity();
accountAnalyticsMongoEntity.setId( accountAnalyticsEntity.getId() );
accountAnalyticsMongoEntity.setAccountId( accountAnalyticsEntity.getAccountId() );
accountAnalyticsMongoEntity.setUserId( accountAnalyticsEntity.getUserId() );
accountAnalyticsMongoEntity.setAnalyticsDate( accountAnalyticsEntity.getAnalyticsDate() );
List list = accountAnalyticsEntity.getBookingGroups();
if ( list != null ) {
accountAnalyticsMongoEntity.setBookingGroups( new ArrayList( list ) );
}
return accountAnalyticsMongoEntity;
}
@Override
public List mapToAnonymizedBookingMongoEntities(List bookingEntities) {
if ( bookingEntities == null ) {
return null;
}
List list = new ArrayList( bookingEntities.size() );
for ( AnonymizedBookingEntity anonymizedBookingEntity : bookingEntities ) {
list.add( anonymizedBookingEntityToAnonymizedBookingMongoEntity( anonymizedBookingEntity ) );
}
return list;
}
@Override
public BankAccessEntity mapToBankAccessEntity(BankAccessMongoEntity entity) {
if ( entity == null ) {
return null;
}
BankAccessEntity bankAccessEntity = new BankAccessEntity();
bankAccessEntity.setBankName( entity.getBankName() );
bankAccessEntity.setBankCode( entity.getBankCode() );
bankAccessEntity.setIban( entity.getIban() );
Map> map = entity.getTanTransportTypes();
if ( map != null ) {
bankAccessEntity.setTanTransportTypes( new HashMap>( map ) );
}
bankAccessEntity.setHbciPassportState( entity.getHbciPassportState() );
Map map1 = entity.getExternalIdMap();
if ( map1 != null ) {
bankAccessEntity.setExternalIdMap( new HashMap( map1 ) );
}
bankAccessEntity.setId( entity.getId() );
bankAccessEntity.setUserId( entity.getUserId() );
bankAccessEntity.setConsentId( entity.getConsentId() );
bankAccessEntity.setTemporary( entity.isTemporary() );
bankAccessEntity.setStoreBookings( entity.isStoreBookings() );
bankAccessEntity.setCategorizeBookings( entity.isCategorizeBookings() );
bankAccessEntity.setStoreAnalytics( entity.isStoreAnalytics() );
bankAccessEntity.setStoreAnonymizedBookings( entity.isStoreAnonymizedBookings() );
bankAccessEntity.setProvideDataForMachineLearning( entity.isProvideDataForMachineLearning() );
return bankAccessEntity;
}
@Override
public List mapToBankAccessEntities(List byUserId) {
if ( byUserId == null ) {
return null;
}
List list = new ArrayList( byUserId.size() );
for ( BankAccessMongoEntity bankAccessMongoEntity : byUserId ) {
list.add( mapToBankAccessEntity( bankAccessMongoEntity ) );
}
return list;
}
@Override
public BankAccessMongoEntity mapToBankAccessMongoEntity(BankAccessEntity bankAccess) {
if ( bankAccess == null ) {
return null;
}
BankAccessMongoEntity bankAccessMongoEntity = new BankAccessMongoEntity();
bankAccessMongoEntity.setBankName( bankAccess.getBankName() );
bankAccessMongoEntity.setBankCode( bankAccess.getBankCode() );
bankAccessMongoEntity.setIban( bankAccess.getIban() );
Map> map = bankAccess.getTanTransportTypes();
if ( map != null ) {
bankAccessMongoEntity.setTanTransportTypes( new HashMap>( map ) );
}
bankAccessMongoEntity.setHbciPassportState( bankAccess.getHbciPassportState() );
Map map1 = bankAccess.getExternalIdMap();
if ( map1 != null ) {
bankAccessMongoEntity.setExternalIdMap( new HashMap( map1 ) );
}
bankAccessMongoEntity.setId( bankAccess.getId() );
bankAccessMongoEntity.setConsentId( bankAccess.getConsentId() );
bankAccessMongoEntity.setUserId( bankAccess.getUserId() );
bankAccessMongoEntity.setTemporary( bankAccess.isTemporary() );
bankAccessMongoEntity.setStoreBookings( bankAccess.isStoreBookings() );
bankAccessMongoEntity.setCategorizeBookings( bankAccess.isCategorizeBookings() );
bankAccessMongoEntity.setStoreAnalytics( bankAccess.isStoreAnalytics() );
bankAccessMongoEntity.setStoreAnonymizedBookings( bankAccess.isStoreAnonymizedBookings() );
bankAccessMongoEntity.setProvideDataForMachineLearning( bankAccess.isProvideDataForMachineLearning() );
return bankAccessMongoEntity;
}
@Override
public List mapToBankAccountEntities(List byUserId) {
if ( byUserId == null ) {
return null;
}
List list = new ArrayList( byUserId.size() );
for ( BankAccountMongoEntity bankAccountMongoEntity : byUserId ) {
list.add( mapToBankAccountEntity( bankAccountMongoEntity ) );
}
return list;
}
@Override
public BankAccountEntity mapToBankAccountEntity(BankAccountMongoEntity bankAccountMongoEntity) {
if ( bankAccountMongoEntity == null ) {
return null;
}
BankAccountEntity bankAccountEntity = new BankAccountEntity();
bankAccountEntity.setBalances( bankAccountMongoEntity.getBalances() );
bankAccountEntity.setCountry( bankAccountMongoEntity.getCountry() );
bankAccountEntity.setBlz( bankAccountMongoEntity.getBlz() );
bankAccountEntity.setAccountNumber( bankAccountMongoEntity.getAccountNumber() );
bankAccountEntity.setType( bankAccountMongoEntity.getType() );
bankAccountEntity.setCurrency( bankAccountMongoEntity.getCurrency() );
bankAccountEntity.setName( bankAccountMongoEntity.getName() );
bankAccountEntity.setBankName( bankAccountMongoEntity.getBankName() );
bankAccountEntity.setBic( bankAccountMongoEntity.getBic() );
bankAccountEntity.setIban( bankAccountMongoEntity.getIban() );
bankAccountEntity.setOwner( bankAccountMongoEntity.getOwner() );
Map map = bankAccountMongoEntity.getExternalIdMap();
if ( map != null ) {
bankAccountEntity.setExternalIdMap( new HashMap( map ) );
}
bankAccountEntity.setSyncStatus( bankAccountMongoEntity.getSyncStatus() );
bankAccountEntity.setLastSync( bankAccountMongoEntity.getLastSync() );
bankAccountEntity.setId( bankAccountMongoEntity.getId() );
bankAccountEntity.setBankAccessId( bankAccountMongoEntity.getBankAccessId() );
bankAccountEntity.setUserId( bankAccountMongoEntity.getUserId() );
return bankAccountEntity;
}
@Override
public List mapToBankAccountMongoEntities(List bankAccounts) {
if ( bankAccounts == null ) {
return null;
}
List list = new ArrayList( bankAccounts.size() );
for ( BankAccountEntity bankAccountEntity : bankAccounts ) {
list.add( mapToBankAccountMongoEntity( bankAccountEntity ) );
}
return list;
}
@Override
public BankAccountMongoEntity mapToBankAccountMongoEntity(BankAccountEntity bankAccount) {
if ( bankAccount == null ) {
return null;
}
BankAccountMongoEntity bankAccountMongoEntity = new BankAccountMongoEntity();
bankAccountMongoEntity.setBalances( bankAccount.getBalances() );
bankAccountMongoEntity.setCountry( bankAccount.getCountry() );
bankAccountMongoEntity.setBlz( bankAccount.getBlz() );
bankAccountMongoEntity.setAccountNumber( bankAccount.getAccountNumber() );
bankAccountMongoEntity.setType( bankAccount.getType() );
bankAccountMongoEntity.setCurrency( bankAccount.getCurrency() );
bankAccountMongoEntity.setName( bankAccount.getName() );
bankAccountMongoEntity.setBankName( bankAccount.getBankName() );
bankAccountMongoEntity.setBic( bankAccount.getBic() );
bankAccountMongoEntity.setIban( bankAccount.getIban() );
bankAccountMongoEntity.setOwner( bankAccount.getOwner() );
Map map = bankAccount.getExternalIdMap();
if ( map != null ) {
bankAccountMongoEntity.setExternalIdMap( new HashMap( map ) );
}
bankAccountMongoEntity.setSyncStatus( bankAccount.getSyncStatus() );
bankAccountMongoEntity.setLastSync( bankAccount.getLastSync() );
bankAccountMongoEntity.setId( bankAccount.getId() );
bankAccountMongoEntity.setBankAccessId( bankAccount.getBankAccessId() );
bankAccountMongoEntity.setUserId( bankAccount.getUserId() );
return bankAccountMongoEntity;
}
@Override
public BankEntity mapToBankEntity(BankMongoEntity bankMongoEntity) {
if ( bankMongoEntity == null ) {
return null;
}
BankEntity bankEntity = new BankEntity();
bankEntity.setBankingUrl( bankMongoEntity.getBankingUrl() );
bankEntity.setBankCode( bankMongoEntity.getBankCode() );
bankEntity.setBic( bankMongoEntity.getBic() );
bankEntity.setName( bankMongoEntity.getName() );
bankEntity.setLoginSettings( bankMongoEntity.getLoginSettings() );
bankEntity.setBankApi( bankMongoEntity.getBankApi() );
bankEntity.setRedirectPreferred( bankMongoEntity.isRedirectPreferred() );
bankEntity.setId( bankMongoEntity.getId() );
bankEntity.setBankApiBankCode( bankMongoEntity.getBankApiBankCode() );
List list = bankMongoEntity.getSearchIndex();
if ( list != null ) {
bankEntity.setSearchIndex( new ArrayList( list ) );
}
return bankEntity;
}
@Override
public List mapToBankMongoEntities(Iterable bankEntities) {
if ( bankEntities == null ) {
return null;
}
List list = new ArrayList();
for ( BankEntity bankEntity : bankEntities ) {
list.add( mapToBankMongoEntity( bankEntity ) );
}
return list;
}
@Override
public BankMongoEntity mapToBankMongoEntity(BankEntity bank) {
if ( bank == null ) {
return null;
}
BankMongoEntity bankMongoEntity = new BankMongoEntity();
bankMongoEntity.setBankingUrl( bank.getBankingUrl() );
bankMongoEntity.setBankCode( bank.getBankCode() );
bankMongoEntity.setBic( bank.getBic() );
bankMongoEntity.setName( bank.getName() );
bankMongoEntity.setLoginSettings( bank.getLoginSettings() );
bankMongoEntity.setBankApi( bank.getBankApi() );
bankMongoEntity.setRedirectPreferred( bank.isRedirectPreferred() );
bankMongoEntity.setId( bank.getId() );
bankMongoEntity.setBankApiBankCode( bank.getBankApiBankCode() );
List list = bank.getSearchIndex();
if ( list != null ) {
bankMongoEntity.setSearchIndex( new ArrayList( list ) );
}
return bankMongoEntity;
}
@Override
public BookingEntity mapToBookingEntity(BookingMongoEntity bookingMongoEntity) {
if ( bookingMongoEntity == null ) {
return null;
}
BookingEntity bookingEntity = new BookingEntity();
bookingEntity.setExternalId( bookingMongoEntity.getExternalId() );
bookingEntity.setOtherAccount( bookingMongoEntity.getOtherAccount() );
bookingEntity.setValutaDate( bookingMongoEntity.getValutaDate() );
bookingEntity.setBookingDate( bookingMongoEntity.getBookingDate() );
bookingEntity.setAmount( bookingMongoEntity.getAmount() );
bookingEntity.setCurrency( bookingMongoEntity.getCurrency() );
bookingEntity.setReversal( bookingMongoEntity.isReversal() );
bookingEntity.setBalance( bookingMongoEntity.getBalance() );
bookingEntity.setCustomerRef( bookingMongoEntity.getCustomerRef() );
bookingEntity.setInstRef( bookingMongoEntity.getInstRef() );
bookingEntity.setOrigValue( bookingMongoEntity.getOrigValue() );
bookingEntity.setChargeValue( bookingMongoEntity.getChargeValue() );
bookingEntity.setText( bookingMongoEntity.getText() );
bookingEntity.setAdditional( bookingMongoEntity.getAdditional() );
bookingEntity.setPrimanota( bookingMongoEntity.getPrimanota() );
bookingEntity.setUsage( bookingMongoEntity.getUsage() );
bookingEntity.setAddkey( bookingMongoEntity.getAddkey() );
bookingEntity.setSepa( bookingMongoEntity.isSepa() );
bookingEntity.setStandingOrder( bookingMongoEntity.isStandingOrder() );
bookingEntity.setCreditorId( bookingMongoEntity.getCreditorId() );
bookingEntity.setMandateReference( bookingMongoEntity.getMandateReference() );
bookingEntity.setBankApi( bookingMongoEntity.getBankApi() );
bookingEntity.setBookingCategory( bookingMongoEntity.getBookingCategory() );
bookingEntity.setTransactionCode( bookingMongoEntity.getTransactionCode() );
bookingEntity.setId( bookingMongoEntity.getId() );
bookingEntity.setAccountId( bookingMongoEntity.getAccountId() );
bookingEntity.setUserId( bookingMongoEntity.getUserId() );
return bookingEntity;
}
@Override
public List mapToBookingEntities(List valutaDate) {
if ( valutaDate == null ) {
return null;
}
List list = new ArrayList( valutaDate.size() );
for ( BookingMongoEntity bookingMongoEntity : valutaDate ) {
list.add( mapToBookingEntity( bookingMongoEntity ) );
}
return list;
}
@Override
public List mapToBookingMongoEntities(List newEntities) {
if ( newEntities == null ) {
return null;
}
List list = new ArrayList( newEntities.size() );
for ( BookingEntity bookingEntity : newEntities ) {
list.add( bookingEntityToBookingMongoEntity( bookingEntity ) );
}
return list;
}
@Override
public List mapToRuleEntities(List byUserId) {
if ( byUserId == null ) {
return null;
}
List list = new ArrayList( byUserId.size() );
for ( RuleMongoEntity ruleMongoEntity : byUserId ) {
list.add( mapToRuleEntity( ruleMongoEntity ) );
}
return list;
}
@Override
public RuleEntity mapToRuleEntity(RuleMongoEntity ruleMongoEntity) {
if ( ruleMongoEntity == null ) {
return null;
}
RuleEntity ruleEntity = new RuleEntity();
ruleEntity.setRuleId( ruleMongoEntity.getRuleId() );
ruleEntity.setMainCategory( ruleMongoEntity.getMainCategory() );
ruleEntity.setSubCategory( ruleMongoEntity.getSubCategory() );
ruleEntity.setSpecification( ruleMongoEntity.getSpecification() );
ruleEntity.setSimilarityMatchType( ruleMongoEntity.getSimilarityMatchType() );
ruleEntity.setCreditorId( ruleMongoEntity.getCreditorId() );
ruleEntity.setExpression( ruleMongoEntity.getExpression() );
ruleEntity.setReceiver( ruleMongoEntity.getReceiver() );
ruleEntity.setRuleType( ruleMongoEntity.getRuleType() );
ruleEntity.setLogo( ruleMongoEntity.getLogo() );
ruleEntity.setHotline( ruleMongoEntity.getHotline() );
ruleEntity.setHomepage( ruleMongoEntity.getHomepage() );
ruleEntity.setEmail( ruleMongoEntity.getEmail() );
ruleEntity.setIncoming( ruleMongoEntity.isIncoming() );
ruleEntity.setId( ruleMongoEntity.getId() );
ruleEntity.setUserId( ruleMongoEntity.getUserId() );
List list = ruleMongoEntity.getSearchIndex();
if ( list != null ) {
ruleEntity.setSearchIndex( new ArrayList( list ) );
}
return ruleEntity;
}
@Override
public RuleMongoEntity mapToRuleMongoEntity(RuleEntity ruleEntity) {
if ( ruleEntity == null ) {
return null;
}
RuleMongoEntity ruleMongoEntity = new RuleMongoEntity();
ruleMongoEntity.setRuleId( ruleEntity.getRuleId() );
ruleMongoEntity.setMainCategory( ruleEntity.getMainCategory() );
ruleMongoEntity.setSubCategory( ruleEntity.getSubCategory() );
ruleMongoEntity.setSpecification( ruleEntity.getSpecification() );
ruleMongoEntity.setSimilarityMatchType( ruleEntity.getSimilarityMatchType() );
ruleMongoEntity.setCreditorId( ruleEntity.getCreditorId() );
ruleMongoEntity.setExpression( ruleEntity.getExpression() );
ruleMongoEntity.setReceiver( ruleEntity.getReceiver() );
ruleMongoEntity.setRuleType( ruleEntity.getRuleType() );
ruleMongoEntity.setLogo( ruleEntity.getLogo() );
ruleMongoEntity.setHotline( ruleEntity.getHotline() );
ruleMongoEntity.setHomepage( ruleEntity.getHomepage() );
ruleMongoEntity.setEmail( ruleEntity.getEmail() );
ruleMongoEntity.setIncoming( ruleEntity.isIncoming() );
ruleMongoEntity.setId( ruleEntity.getId() );
ruleMongoEntity.setUserId( ruleEntity.getUserId() );
List list = ruleEntity.getSearchIndex();
if ( list != null ) {
ruleMongoEntity.setSearchIndex( new ArrayList( list ) );
}
return ruleMongoEntity;
}
@Override
public BookingsIndexMongoEntity mapToBookingsIndexMongoEntity(BookingsIndexEntity entity) {
if ( entity == null ) {
return null;
}
BookingsIndexMongoEntity bookingsIndexMongoEntity = new BookingsIndexMongoEntity();
bookingsIndexMongoEntity.setId( entity.getId() );
bookingsIndexMongoEntity.setAccountId( entity.getAccountId() );
bookingsIndexMongoEntity.setUserId( entity.getUserId() );
Map> map = entity.getBookingIdSearchList();
if ( map != null ) {
bookingsIndexMongoEntity.setBookingIdSearchList( new HashMap>( map ) );
}
return bookingsIndexMongoEntity;
}
@Override
public BookingsIndexEntity mapToBookingsIndexEntity(BookingsIndexMongoEntity bookingsIndexMongoEntity) {
if ( bookingsIndexMongoEntity == null ) {
return null;
}
BookingsIndexEntity bookingsIndexEntity = new BookingsIndexEntity();
bookingsIndexEntity.setId( bookingsIndexMongoEntity.getId() );
bookingsIndexEntity.setAccountId( bookingsIndexMongoEntity.getAccountId() );
bookingsIndexEntity.setUserId( bookingsIndexMongoEntity.getUserId() );
Map> map = bookingsIndexMongoEntity.getBookingIdSearchList();
if ( map != null ) {
bookingsIndexEntity.setBookingIdSearchList( new HashMap>( map ) );
}
return bookingsIndexEntity;
}
@Override
public BulkPaymentMongoEntity mapToBulkPaymentMongoEntity(BulkPaymentEntity target) {
if ( target == null ) {
return null;
}
BulkPaymentMongoEntity bulkPaymentMongoEntity = new BulkPaymentMongoEntity();
bulkPaymentMongoEntity.setPsuAccount( target.getPsuAccount() );
bulkPaymentMongoEntity.setOrderId( target.getOrderId() );
bulkPaymentMongoEntity.setPaymentId( target.getPaymentId() );
bulkPaymentMongoEntity.setProduct( target.getProduct() );
List list = target.getPayments();
if ( list != null ) {
bulkPaymentMongoEntity.setPayments( new ArrayList( list ) );
}
bulkPaymentMongoEntity.setBatchbooking( target.getBatchbooking() );
bulkPaymentMongoEntity.setId( target.getId() );
bulkPaymentMongoEntity.setUserId( target.getUserId() );
bulkPaymentMongoEntity.setCreatedDateTime( target.getCreatedDateTime() );
bulkPaymentMongoEntity.setTanSubmitExternal( target.getTanSubmitExternal() );
return bulkPaymentMongoEntity;
}
@Override
public List mapToContractEntities(List byUserIdAndAccountId) {
if ( byUserIdAndAccountId == null ) {
return null;
}
List list = new ArrayList( byUserIdAndAccountId.size() );
for ( ContractMongoEntity contractMongoEntity : byUserIdAndAccountId ) {
list.add( contractMongoEntityToContractEntity( contractMongoEntity ) );
}
return list;
}
@Override
public List mapToContractMongoEntities(List contractEntities) {
if ( contractEntities == null ) {
return null;
}
List list = new ArrayList( contractEntities.size() );
for ( ContractEntity contractEntity : contractEntities ) {
list.add( contractEntityToContractMongoEntity( contractEntity ) );
}
return list;
}
@Override
public MlAnonymizedBookingEntity mapToMlAnonymizedBookingEntity(MlAnonymizedBookingMongoEntity mlAnonymizedBookingMongoEntity) {
if ( mlAnonymizedBookingMongoEntity == null ) {
return null;
}
MlAnonymizedBookingEntity mlAnonymizedBookingEntity = new MlAnonymizedBookingEntity();
mlAnonymizedBookingEntity.setId( mlAnonymizedBookingMongoEntity.getId() );
mlAnonymizedBookingEntity.setUserId( mlAnonymizedBookingMongoEntity.getUserId() );
mlAnonymizedBookingEntity.setTransactionPurpose( mlAnonymizedBookingMongoEntity.getTransactionPurpose() );
mlAnonymizedBookingEntity.setAmountBin( mlAnonymizedBookingMongoEntity.getAmountBin() );
mlAnonymizedBookingEntity.setAmountRemainder( mlAnonymizedBookingMongoEntity.getAmountRemainder() );
mlAnonymizedBookingEntity.setCreditorId( mlAnonymizedBookingMongoEntity.getCreditorId() );
mlAnonymizedBookingEntity.setReferenceName( mlAnonymizedBookingMongoEntity.getReferenceName() );
mlAnonymizedBookingEntity.setExecutionDate( mlAnonymizedBookingMongoEntity.getExecutionDate() );
mlAnonymizedBookingEntity.setMainCategory( mlAnonymizedBookingMongoEntity.getMainCategory() );
mlAnonymizedBookingEntity.setSubCategory( mlAnonymizedBookingMongoEntity.getSubCategory() );
mlAnonymizedBookingEntity.setSpecification( mlAnonymizedBookingMongoEntity.getSpecification() );
return mlAnonymizedBookingEntity;
}
@Override
public List mapToMlAnonymizedBookingEntities(List byUserId) {
if ( byUserId == null ) {
return null;
}
List list = new ArrayList( byUserId.size() );
for ( MlAnonymizedBookingMongoEntity mlAnonymizedBookingMongoEntity : byUserId ) {
list.add( mapToMlAnonymizedBookingEntity( mlAnonymizedBookingMongoEntity ) );
}
return list;
}
@Override
public MlAnonymizedBookingMongoEntity mapToMlAnonymizedBookingMongoEntity(MlAnonymizedBookingEntity booking) {
if ( booking == null ) {
return null;
}
MlAnonymizedBookingMongoEntity mlAnonymizedBookingMongoEntity = new MlAnonymizedBookingMongoEntity();
mlAnonymizedBookingMongoEntity.setId( booking.getId() );
mlAnonymizedBookingMongoEntity.setUserId( booking.getUserId() );
mlAnonymizedBookingMongoEntity.setTransactionPurpose( booking.getTransactionPurpose() );
mlAnonymizedBookingMongoEntity.setAmountBin( booking.getAmountBin() );
mlAnonymizedBookingMongoEntity.setAmountRemainder( booking.getAmountRemainder() );
mlAnonymizedBookingMongoEntity.setCreditorId( booking.getCreditorId() );
mlAnonymizedBookingMongoEntity.setReferenceName( booking.getReferenceName() );
mlAnonymizedBookingMongoEntity.setExecutionDate( booking.getExecutionDate() );
mlAnonymizedBookingMongoEntity.setMainCategory( booking.getMainCategory() );
mlAnonymizedBookingMongoEntity.setSubCategory( booking.getSubCategory() );
mlAnonymizedBookingMongoEntity.setSpecification( booking.getSpecification() );
return mlAnonymizedBookingMongoEntity;
}
@Override
public RawSepaTransactionEntity mapToRawSepaTransactionEntity(RawSepaTransactionMongoEntity rawSepaTransactionMongoEntity) {
if ( rawSepaTransactionMongoEntity == null ) {
return null;
}
RawSepaTransactionEntity rawSepaTransactionEntity = new RawSepaTransactionEntity();
rawSepaTransactionEntity.setPsuAccount( rawSepaTransactionMongoEntity.getPsuAccount() );
rawSepaTransactionEntity.setOrderId( rawSepaTransactionMongoEntity.getOrderId() );
rawSepaTransactionEntity.setPaymentId( rawSepaTransactionMongoEntity.getPaymentId() );
rawSepaTransactionEntity.setProduct( rawSepaTransactionMongoEntity.getProduct() );
rawSepaTransactionEntity.setPainXml( rawSepaTransactionMongoEntity.getPainXml() );
rawSepaTransactionEntity.setService( rawSepaTransactionMongoEntity.getService() );
rawSepaTransactionEntity.setSepaTransactionType( rawSepaTransactionMongoEntity.getSepaTransactionType() );
rawSepaTransactionEntity.setId( rawSepaTransactionMongoEntity.getId() );
rawSepaTransactionEntity.setUserId( rawSepaTransactionMongoEntity.getUserId() );
rawSepaTransactionEntity.setCreatedDateTime( rawSepaTransactionMongoEntity.getCreatedDateTime() );
rawSepaTransactionEntity.setTanSubmitExternal( rawSepaTransactionMongoEntity.getTanSubmitExternal() );
return rawSepaTransactionEntity;
}
@Override
public RawSepaTransactionMongoEntity mapToRawSepaTransactionMongoEntity(RawSepaTransactionEntity paymentEntity) {
if ( paymentEntity == null ) {
return null;
}
RawSepaTransactionMongoEntity rawSepaTransactionMongoEntity = new RawSepaTransactionMongoEntity();
rawSepaTransactionMongoEntity.setPsuAccount( paymentEntity.getPsuAccount() );
rawSepaTransactionMongoEntity.setOrderId( paymentEntity.getOrderId() );
rawSepaTransactionMongoEntity.setPaymentId( paymentEntity.getPaymentId() );
rawSepaTransactionMongoEntity.setProduct( paymentEntity.getProduct() );
rawSepaTransactionMongoEntity.setPainXml( paymentEntity.getPainXml() );
rawSepaTransactionMongoEntity.setService( paymentEntity.getService() );
rawSepaTransactionMongoEntity.setSepaTransactionType( paymentEntity.getSepaTransactionType() );
rawSepaTransactionMongoEntity.setId( paymentEntity.getId() );
rawSepaTransactionMongoEntity.setUserId( paymentEntity.getUserId() );
rawSepaTransactionMongoEntity.setCreatedDateTime( paymentEntity.getCreatedDateTime() );
rawSepaTransactionMongoEntity.setTanSubmitExternal( paymentEntity.getTanSubmitExternal() );
return rawSepaTransactionMongoEntity;
}
@Override
public SinglePaymentEntity mapToPaymentEntity(PaymentMongoEntity paymentMongoEntity) {
if ( paymentMongoEntity == null ) {
return null;
}
SinglePaymentEntity singlePaymentEntity = new SinglePaymentEntity();
singlePaymentEntity.setPsuAccount( paymentMongoEntity.getPsuAccount() );
singlePaymentEntity.setOrderId( paymentMongoEntity.getOrderId() );
singlePaymentEntity.setPaymentId( paymentMongoEntity.getPaymentId() );
singlePaymentEntity.setProduct( paymentMongoEntity.getProduct() );
singlePaymentEntity.setReceiver( paymentMongoEntity.getReceiver() );
singlePaymentEntity.setReceiverBic( paymentMongoEntity.getReceiverBic() );
singlePaymentEntity.setReceiverIban( paymentMongoEntity.getReceiverIban() );
singlePaymentEntity.setReceiverBankCode( paymentMongoEntity.getReceiverBankCode() );
singlePaymentEntity.setReceiverAccountNumber( paymentMongoEntity.getReceiverAccountNumber() );
singlePaymentEntity.setReceiverAccountCurrency( paymentMongoEntity.getReceiverAccountCurrency() );
singlePaymentEntity.setPurpose( paymentMongoEntity.getPurpose() );
singlePaymentEntity.setPurposecode( paymentMongoEntity.getPurposecode() );
singlePaymentEntity.setAmount( paymentMongoEntity.getAmount() );
singlePaymentEntity.setCurrency( paymentMongoEntity.getCurrency() );
singlePaymentEntity.setId( paymentMongoEntity.getId() );
singlePaymentEntity.setUserId( paymentMongoEntity.getUserId() );
singlePaymentEntity.setCreatedDateTime( paymentMongoEntity.getCreatedDateTime() );
singlePaymentEntity.setTanSubmitExternal( paymentMongoEntity.getTanSubmitExternal() );
return singlePaymentEntity;
}
@Override
public PaymentMongoEntity mapToPaymentMongoEntity(SinglePaymentEntity paymentMongoEntity) {
if ( paymentMongoEntity == null ) {
return null;
}
PaymentMongoEntity paymentMongoEntity1 = new PaymentMongoEntity();
paymentMongoEntity1.setPsuAccount( paymentMongoEntity.getPsuAccount() );
paymentMongoEntity1.setOrderId( paymentMongoEntity.getOrderId() );
paymentMongoEntity1.setPaymentId( paymentMongoEntity.getPaymentId() );
paymentMongoEntity1.setProduct( paymentMongoEntity.getProduct() );
paymentMongoEntity1.setReceiver( paymentMongoEntity.getReceiver() );
paymentMongoEntity1.setReceiverBic( paymentMongoEntity.getReceiverBic() );
paymentMongoEntity1.setReceiverIban( paymentMongoEntity.getReceiverIban() );
paymentMongoEntity1.setReceiverBankCode( paymentMongoEntity.getReceiverBankCode() );
paymentMongoEntity1.setReceiverAccountNumber( paymentMongoEntity.getReceiverAccountNumber() );
paymentMongoEntity1.setReceiverAccountCurrency( paymentMongoEntity.getReceiverAccountCurrency() );
paymentMongoEntity1.setPurpose( paymentMongoEntity.getPurpose() );
paymentMongoEntity1.setPurposecode( paymentMongoEntity.getPurposecode() );
paymentMongoEntity1.setAmount( paymentMongoEntity.getAmount() );
paymentMongoEntity1.setCurrency( paymentMongoEntity.getCurrency() );
paymentMongoEntity1.setId( paymentMongoEntity.getId() );
paymentMongoEntity1.setUserId( paymentMongoEntity.getUserId() );
paymentMongoEntity1.setCreatedDateTime( paymentMongoEntity.getCreatedDateTime() );
paymentMongoEntity1.setTanSubmitExternal( paymentMongoEntity.getTanSubmitExternal() );
return paymentMongoEntity1;
}
@Override
public StandingOrderMongoEntity mapToStandingOrderMongoEntity(StandingOrderEntity paymentMongoEntity) {
if ( paymentMongoEntity == null ) {
return null;
}
StandingOrderMongoEntity standingOrderMongoEntity = new StandingOrderMongoEntity();
standingOrderMongoEntity.setPsuAccount( paymentMongoEntity.getPsuAccount() );
standingOrderMongoEntity.setOrderId( paymentMongoEntity.getOrderId() );
standingOrderMongoEntity.setPaymentId( paymentMongoEntity.getPaymentId() );
standingOrderMongoEntity.setProduct( paymentMongoEntity.getProduct() );
standingOrderMongoEntity.setCycle( paymentMongoEntity.getCycle() );
standingOrderMongoEntity.setExecutionDay( paymentMongoEntity.getExecutionDay() );
standingOrderMongoEntity.setFirstExecutionDate( paymentMongoEntity.getFirstExecutionDate() );
standingOrderMongoEntity.setLastExecutionDate( paymentMongoEntity.getLastExecutionDate() );
standingOrderMongoEntity.setAmount( paymentMongoEntity.getAmount() );
standingOrderMongoEntity.setCurrency( paymentMongoEntity.getCurrency() );
standingOrderMongoEntity.setOtherAccount( paymentMongoEntity.getOtherAccount() );
standingOrderMongoEntity.setUsage( paymentMongoEntity.getUsage() );
standingOrderMongoEntity.setDelete( paymentMongoEntity.isDelete() );
standingOrderMongoEntity.setPurposecode( paymentMongoEntity.getPurposecode() );
standingOrderMongoEntity.setId( paymentMongoEntity.getId() );
standingOrderMongoEntity.setAccountId( paymentMongoEntity.getAccountId() );
standingOrderMongoEntity.setUserId( paymentMongoEntity.getUserId() );
standingOrderMongoEntity.setTanSubmitExternal( paymentMongoEntity.getTanSubmitExternal() );
standingOrderMongoEntity.setCreatedDateTime( paymentMongoEntity.getCreatedDateTime() );
return standingOrderMongoEntity;
}
@Override
public List mapToStandingOrderEntities(List byUserIdAndAccountId) {
if ( byUserIdAndAccountId == null ) {
return null;
}
List list = new ArrayList( byUserIdAndAccountId.size() );
for ( StandingOrderMongoEntity standingOrderMongoEntity : byUserIdAndAccountId ) {
list.add( standingOrderMongoEntityToStandingOrderEntity( standingOrderMongoEntity ) );
}
return list;
}
@Override
public List mapToStandingOrderMongoEntities(List standingOrders) {
if ( standingOrders == null ) {
return null;
}
List list = new ArrayList( standingOrders.size() );
for ( StandingOrderEntity standingOrderEntity : standingOrders ) {
list.add( mapToStandingOrderMongoEntity( standingOrderEntity ) );
}
return list;
}
@Override
public UserEntity mapToUserEntity(UserMongoEntity userMongoEntity) {
if ( userMongoEntity == null ) {
return null;
}
UserEntity userEntity = new UserEntity();
userEntity.setId( userMongoEntity.getId() );
userEntity.setExpireUser( userMongoEntity.getExpireUser() );
userEntity.setRulesLastChangeDate( userMongoEntity.getRulesLastChangeDate() );
List list = userMongoEntity.getApiUser();
if ( list != null ) {
userEntity.setApiUser( new ArrayList( list ) );
}
return userEntity;
}
@Override
public UserMongoEntity mapToUserMongoEntity(UserEntity userEntity) {
if ( userEntity == null ) {
return null;
}
UserMongoEntity userMongoEntity = new UserMongoEntity();
userMongoEntity.setId( userEntity.getId() );
userMongoEntity.setExpireUser( userEntity.getExpireUser() );
userMongoEntity.setRulesLastChangeDate( userEntity.getRulesLastChangeDate() );
List list = userEntity.getApiUser();
if ( list != null ) {
userMongoEntity.setApiUser( new ArrayList( list ) );
}
return userMongoEntity;
}
@Override
public List mapToBankEntities(List bankMongoEntities) {
if ( bankMongoEntities == null ) {
return null;
}
List list = new ArrayList( bankMongoEntities.size() );
for ( BankMongoEntity bankMongoEntity : bankMongoEntities ) {
list.add( mapToBankEntity( bankMongoEntity ) );
}
return list;
}
@Override
public List mapToBookingsIndexEntities(List bookingsIndexMongoEntities) {
if ( bookingsIndexMongoEntities == null ) {
return null;
}
List list = new ArrayList( bookingsIndexMongoEntities.size() );
for ( BookingsIndexMongoEntity bookingsIndexMongoEntity : bookingsIndexMongoEntities ) {
list.add( mapToBookingsIndexEntity( bookingsIndexMongoEntity ) );
}
return list;
}
@Override
public ConsentEntity toConsentEntity(ConsentMongoEntity consentMongoEntity) {
if ( consentMongoEntity == null ) {
return null;
}
ConsentEntity consentEntity = new ConsentEntity();
consentEntity.setId( consentMongoEntity.getId() );
consentEntity.setAuthorisationId( consentMongoEntity.getAuthorisationId() );
consentEntity.setBankApi( consentMongoEntity.getBankApi() );
consentEntity.setPsuAccountIban( consentMongoEntity.getPsuAccountIban() );
consentEntity.setBankApiConsentData( consentMongoEntity.getBankApiConsentData() );
return consentEntity;
}
@Override
public ConsentMongoEntity toConsentMongoEntity(ConsentEntity consentEntity) {
if ( consentEntity == null ) {
return null;
}
ConsentMongoEntity consentMongoEntity = new ConsentMongoEntity();
consentMongoEntity.setId( consentEntity.getId() );
consentMongoEntity.setAuthorisationId( consentEntity.getAuthorisationId() );
consentMongoEntity.setBankApi( consentEntity.getBankApi() );
consentMongoEntity.setPsuAccountIban( consentEntity.getPsuAccountIban() );
consentMongoEntity.setBankApiConsentData( consentEntity.getBankApiConsentData() );
return consentMongoEntity;
}
protected AnonymizedBookingMongoEntity anonymizedBookingEntityToAnonymizedBookingMongoEntity(AnonymizedBookingEntity anonymizedBookingEntity) {
if ( anonymizedBookingEntity == null ) {
return null;
}
AnonymizedBookingMongoEntity anonymizedBookingMongoEntity = new AnonymizedBookingMongoEntity();
anonymizedBookingMongoEntity.setCreditorId( anonymizedBookingEntity.getCreditorId() );
anonymizedBookingMongoEntity.setAmount( anonymizedBookingEntity.getAmount() );
anonymizedBookingMongoEntity.setPurpose( anonymizedBookingEntity.getPurpose() );
return anonymizedBookingMongoEntity;
}
protected BookingMongoEntity bookingEntityToBookingMongoEntity(BookingEntity bookingEntity) {
if ( bookingEntity == null ) {
return null;
}
BookingMongoEntity bookingMongoEntity = new BookingMongoEntity();
bookingMongoEntity.setExternalId( bookingEntity.getExternalId() );
bookingMongoEntity.setOtherAccount( bookingEntity.getOtherAccount() );
bookingMongoEntity.setValutaDate( bookingEntity.getValutaDate() );
bookingMongoEntity.setBookingDate( bookingEntity.getBookingDate() );
bookingMongoEntity.setAmount( bookingEntity.getAmount() );
bookingMongoEntity.setCurrency( bookingEntity.getCurrency() );
bookingMongoEntity.setReversal( bookingEntity.isReversal() );
bookingMongoEntity.setBalance( bookingEntity.getBalance() );
bookingMongoEntity.setCustomerRef( bookingEntity.getCustomerRef() );
bookingMongoEntity.setInstRef( bookingEntity.getInstRef() );
bookingMongoEntity.setOrigValue( bookingEntity.getOrigValue() );
bookingMongoEntity.setChargeValue( bookingEntity.getChargeValue() );
bookingMongoEntity.setText( bookingEntity.getText() );
bookingMongoEntity.setAdditional( bookingEntity.getAdditional() );
bookingMongoEntity.setPrimanota( bookingEntity.getPrimanota() );
bookingMongoEntity.setUsage( bookingEntity.getUsage() );
bookingMongoEntity.setAddkey( bookingEntity.getAddkey() );
bookingMongoEntity.setSepa( bookingEntity.isSepa() );
bookingMongoEntity.setStandingOrder( bookingEntity.isStandingOrder() );
bookingMongoEntity.setCreditorId( bookingEntity.getCreditorId() );
bookingMongoEntity.setMandateReference( bookingEntity.getMandateReference() );
bookingMongoEntity.setBankApi( bookingEntity.getBankApi() );
bookingMongoEntity.setBookingCategory( bookingEntity.getBookingCategory() );
bookingMongoEntity.setTransactionCode( bookingEntity.getTransactionCode() );
bookingMongoEntity.setId( bookingEntity.getId() );
bookingMongoEntity.setAccountId( bookingEntity.getAccountId() );
bookingMongoEntity.setUserId( bookingEntity.getUserId() );
return bookingMongoEntity;
}
protected ContractEntity contractMongoEntityToContractEntity(ContractMongoEntity contractMongoEntity) {
if ( contractMongoEntity == null ) {
return null;
}
ContractEntity contractEntity = new ContractEntity();
contractEntity.setLogo( contractMongoEntity.getLogo() );
contractEntity.setHomepage( contractMongoEntity.getHomepage() );
contractEntity.setHotline( contractMongoEntity.getHotline() );
contractEntity.setEmail( contractMongoEntity.getEmail() );
contractEntity.setMandateReference( contractMongoEntity.getMandateReference() );
contractEntity.setInterval( contractMongoEntity.getInterval() );
contractEntity.setCancelled( contractMongoEntity.isCancelled() );
contractEntity.setAmount( contractMongoEntity.getAmount() );
contractEntity.setMainCategory( contractMongoEntity.getMainCategory() );
contractEntity.setSubCategory( contractMongoEntity.getSubCategory() );
contractEntity.setSpecification( contractMongoEntity.getSpecification() );
contractEntity.setProvider( contractMongoEntity.getProvider() );
contractEntity.setId( contractMongoEntity.getId() );
contractEntity.setUserId( contractMongoEntity.getUserId() );
contractEntity.setAccountId( contractMongoEntity.getAccountId() );
return contractEntity;
}
protected ContractMongoEntity contractEntityToContractMongoEntity(ContractEntity contractEntity) {
if ( contractEntity == null ) {
return null;
}
ContractMongoEntity contractMongoEntity = new ContractMongoEntity();
contractMongoEntity.setLogo( contractEntity.getLogo() );
contractMongoEntity.setHomepage( contractEntity.getHomepage() );
contractMongoEntity.setHotline( contractEntity.getHotline() );
contractMongoEntity.setEmail( contractEntity.getEmail() );
contractMongoEntity.setMandateReference( contractEntity.getMandateReference() );
contractMongoEntity.setInterval( contractEntity.getInterval() );
contractMongoEntity.setCancelled( contractEntity.isCancelled() );
contractMongoEntity.setAmount( contractEntity.getAmount() );
contractMongoEntity.setMainCategory( contractEntity.getMainCategory() );
contractMongoEntity.setSubCategory( contractEntity.getSubCategory() );
contractMongoEntity.setSpecification( contractEntity.getSpecification() );
contractMongoEntity.setProvider( contractEntity.getProvider() );
contractMongoEntity.setId( contractEntity.getId() );
contractMongoEntity.setUserId( contractEntity.getUserId() );
contractMongoEntity.setAccountId( contractEntity.getAccountId() );
return contractMongoEntity;
}
protected StandingOrderEntity standingOrderMongoEntityToStandingOrderEntity(StandingOrderMongoEntity standingOrderMongoEntity) {
if ( standingOrderMongoEntity == null ) {
return null;
}
StandingOrderEntity standingOrderEntity = new StandingOrderEntity();
standingOrderEntity.setPsuAccount( standingOrderMongoEntity.getPsuAccount() );
standingOrderEntity.setOrderId( standingOrderMongoEntity.getOrderId() );
standingOrderEntity.setPaymentId( standingOrderMongoEntity.getPaymentId() );
standingOrderEntity.setProduct( standingOrderMongoEntity.getProduct() );
standingOrderEntity.setCycle( standingOrderMongoEntity.getCycle() );
standingOrderEntity.setExecutionDay( standingOrderMongoEntity.getExecutionDay() );
standingOrderEntity.setFirstExecutionDate( standingOrderMongoEntity.getFirstExecutionDate() );
standingOrderEntity.setLastExecutionDate( standingOrderMongoEntity.getLastExecutionDate() );
standingOrderEntity.setAmount( standingOrderMongoEntity.getAmount() );
standingOrderEntity.setCurrency( standingOrderMongoEntity.getCurrency() );
standingOrderEntity.setOtherAccount( standingOrderMongoEntity.getOtherAccount() );
standingOrderEntity.setUsage( standingOrderMongoEntity.getUsage() );
standingOrderEntity.setDelete( standingOrderMongoEntity.isDelete() );
standingOrderEntity.setPurposecode( standingOrderMongoEntity.getPurposecode() );
standingOrderEntity.setId( standingOrderMongoEntity.getId() );
standingOrderEntity.setAccountId( standingOrderMongoEntity.getAccountId() );
standingOrderEntity.setUserId( standingOrderMongoEntity.getUserId() );
standingOrderEntity.setCreatedDateTime( standingOrderMongoEntity.getCreatedDateTime() );
standingOrderEntity.setTanSubmitExternal( standingOrderMongoEntity.getTanSubmitExternal() );
return standingOrderEntity;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy