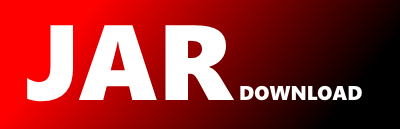
de.adorsys.xs2a.adapter.fiducia.model.FiduciaPaymentInitationRequestResponse201 Maven / Gradle / Ivy
package de.adorsys.xs2a.adapter.fiducia.model;
import com.fasterxml.jackson.annotation.JsonProperty;
import de.adorsys.xs2a.adapter.api.model.*;
import java.util.List;
import java.util.Map;
import java.util.Objects;
public class FiduciaPaymentInitationRequestResponse201 {
private TransactionStatus transactionStatus;
private String paymentId;
private Amount transactionFees;
private Boolean transactionFeeIndicator;
private List scaMethods;
private AuthenticationObject chosenScaMethod;
private FiduciaChallengeData challengeData;
@JsonProperty("_links")
private Map links;
private String psuMessage;
private List tppMessages;
public TransactionStatus getTransactionStatus() {
return transactionStatus;
}
public void setTransactionStatus(TransactionStatus transactionStatus) {
this.transactionStatus = transactionStatus;
}
public String getPaymentId() {
return paymentId;
}
public void setPaymentId(String paymentId) {
this.paymentId = paymentId;
}
public Amount getTransactionFees() {
return transactionFees;
}
public void setTransactionFees(Amount transactionFees) {
this.transactionFees = transactionFees;
}
public Boolean getTransactionFeeIndicator() {
return transactionFeeIndicator;
}
public void setTransactionFeeIndicator(Boolean transactionFeeIndicator) {
this.transactionFeeIndicator = transactionFeeIndicator;
}
public List getScaMethods() {
return scaMethods;
}
public void setScaMethods(List scaMethods) {
this.scaMethods = scaMethods;
}
public AuthenticationObject getChosenScaMethod() {
return chosenScaMethod;
}
public void setChosenScaMethod(AuthenticationObject chosenScaMethod) {
this.chosenScaMethod = chosenScaMethod;
}
public FiduciaChallengeData getChallengeData() {
return challengeData;
}
public void setChallengeData(FiduciaChallengeData challengeData) {
this.challengeData = challengeData;
}
public Map getLinks() {
return links;
}
public void setLinks(Map links) {
this.links = links;
}
public String getPsuMessage() {
return psuMessage;
}
public void setPsuMessage(String psuMessage) {
this.psuMessage = psuMessage;
}
public List getTppMessages() {
return tppMessages;
}
public void setTppMessages(List tppMessages) {
this.tppMessages = tppMessages;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
FiduciaPaymentInitationRequestResponse201 that = (FiduciaPaymentInitationRequestResponse201) o;
return Objects.equals(transactionStatus, that.transactionStatus) &&
Objects.equals(paymentId, that.paymentId) &&
Objects.equals(transactionFees, that.transactionFees) &&
Objects.equals(transactionFeeIndicator, that.transactionFeeIndicator) &&
Objects.equals(scaMethods, that.scaMethods) &&
Objects.equals(chosenScaMethod, that.chosenScaMethod) &&
Objects.equals(challengeData, that.challengeData) &&
Objects.equals(links, that.links) &&
Objects.equals(psuMessage, that.psuMessage) &&
Objects.equals(tppMessages, that.tppMessages);
}
@Override
public int hashCode() {
return Objects.hash(transactionStatus,
paymentId,
transactionFees,
transactionFeeIndicator,
scaMethods,
chosenScaMethod,
challengeData,
links,
psuMessage,
tppMessages);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy