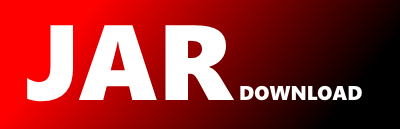
de.adorsys.datasafe.encrypiton.impl.cmsencryption.CMSEncryptionServiceImplRuntimeDelegatable Maven / Gradle / Ivy
The newest version!
package de.adorsys.datasafe.encrypiton.impl.cmsencryption;
import de.adorsys.datasafe.encrypiton.api.types.keystore.KeyID;
import de.adorsys.datasafe.encrypiton.api.types.keystore.PublicKeyIDWithPublicKey;
import de.adorsys.datasafe.types.api.context.overrides.OverridesRegistry;
import jakarta.annotation.Generated;
import java.io.InputStream;
import java.io.OutputStream;
import java.lang.Override;
import java.lang.String;
import java.security.Key;
import java.util.Map;
import java.util.Set;
import java.util.function.Function;
import javax.annotation.Nullable;
import javax.crypto.SecretKey;
import javax.inject.Inject;
@Generated(
value = "de.adorsys.datasafe.runtimedelegate.RuntimeDelegateGenerator",
comments = "This class performs functionality delegation based on contextClass content. If contextClass contains overriding class - it will be used."
)
public class CMSEncryptionServiceImplRuntimeDelegatable extends CMSEncryptionServiceImpl {
private final CMSEncryptionServiceImpl delegate;
/**
* @param context Context class to search for overrides.
*/
@Inject
public CMSEncryptionServiceImplRuntimeDelegatable(@Nullable OverridesRegistry context,
ASNCmsEncryptionConfig encryptionConfig) {
super(encryptionConfig);
ArgumentsCaptor argumentsCaptor = new ArgumentsCaptor(encryptionConfig);
delegate = context != null ? context.findOverride(CMSEncryptionServiceImpl.class, argumentsCaptor) : null;
}
@Override
public OutputStream buildEncryptionOutputStream(OutputStream dataContentStream,
Set publicKeys) {
if (null == delegate) {
return super.buildEncryptionOutputStream(dataContentStream, publicKeys);
} else {
return delegate.buildEncryptionOutputStream(dataContentStream, publicKeys);
}
}
@Override
public OutputStream buildEncryptionOutputStream(OutputStream dataContentStream,
SecretKey secretKey, KeyID keyID) {
if (null == delegate) {
return super.buildEncryptionOutputStream(dataContentStream, secretKey, keyID);
} else {
return delegate.buildEncryptionOutputStream(dataContentStream, secretKey, keyID);
}
}
@Override
public InputStream buildDecryptionInputStream(InputStream inputStream,
Function, Map> keysByIds) {
if (null == delegate) {
return super.buildDecryptionInputStream(inputStream, keysByIds);
} else {
return delegate.buildDecryptionInputStream(inputStream, keysByIds);
}
}
/**
* This is a typesafe function to register overriding class into context.
*/
public static void overrideWith(OverridesRegistry context,
Function ctorCaptor) {
context.override(CMSEncryptionServiceImpl.class, args -> ctorCaptor.apply((ArgumentsCaptor) args));
}
public static class ArgumentsCaptor {
private final ASNCmsEncryptionConfig encryptionConfig;
private ArgumentsCaptor(ASNCmsEncryptionConfig encryptionConfig) {
this.encryptionConfig = encryptionConfig;
}
public ASNCmsEncryptionConfig getEncryptionConfig() {
return encryptionConfig;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy