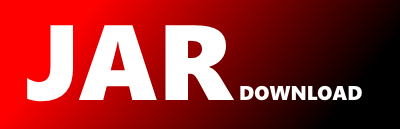
de.adrodoc55.minecraft.mpl.antlr.MplParser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mpl-compiler Show documentation
Show all versions of mpl-compiler Show documentation
MPL is a language that makes it easier to write applications for Minecraft 1.9 or higher. The final result of compiling an MPL application are command blocks that can be imported into your world in various ways. MPL comes with it's own editor that supports syntax- and error-highlighting and has a built in compiler.
// Generated from C:\Users\Adrian\Programme\workspace\MPL\compiler\src\antlr\def\de\adrodoc55\minecraft\mpl\antlr\Mpl.g4 by ANTLR 4.5.3
package de.adrodoc55.minecraft.mpl.antlr;
import org.antlr.v4.runtime.atn.*;
import org.antlr.v4.runtime.dfa.DFA;
import org.antlr.v4.runtime.*;
import org.antlr.v4.runtime.misc.*;
import org.antlr.v4.runtime.tree.*;
import java.util.List;
import java.util.Iterator;
import java.util.ArrayList;
@SuppressWarnings({"all", "warnings", "unchecked", "unused", "cast"})
public class MplParser extends Parser {
static { RuntimeMetaData.checkVersion("4.5.3", RuntimeMetaData.VERSION); }
protected static final DFA[] _decisionToDFA;
protected static final PredictionContextCache _sharedContextCache =
new PredictionContextCache();
public static final int
T__0=1, T__1=2, T__2=3, T__3=4, COMMENT=5, COMMAND=6, IMPORT=7, PROJECT=8,
INCLUDE=9, ORIENTATION=10, INSTALL=11, UNINSTALL=12, PROCESS=13, IMPULSE=14,
CHAIN=15, REPEAT=16, UNCONDITIONAL=17, CONDITIONAL=18, INVERT=19, ALWAYS_ACTIVE=20,
NEEDS_REDSTONE=21, START=22, STOP=23, WAITFOR=24, NOTIFY=25, INTERCEPT=26,
BREAKPOINT=27, SKIP_TOKEN=28, IF=29, NOT=30, THEN=31, ELSE=32, DO=33,
WHILE=34, BREAK=35, CONTINUE=36, UNSIGNED_INT=37, WS=38, STRING=39, IDENTIFIER=40,
UNRECOGNIZED=41;
public static final int
RULE_file = 0, RULE_scriptFile = 1, RULE_projectFile = 2, RULE_importDeclaration = 3,
RULE_project = 4, RULE_orientation = 5, RULE_include = 6, RULE_install = 7,
RULE_uninstall = 8, RULE_process = 9, RULE_chain = 10, RULE_ifDeclaration = 11,
RULE_then = 12, RULE_elseDeclaration = 13, RULE_whileDeclaration = 14,
RULE_mplCommand = 15, RULE_modifierList = 16, RULE_modus = 17, RULE_conditional = 18,
RULE_auto = 19, RULE_command = 20, RULE_start = 21, RULE_stop = 22, RULE_waitfor = 23,
RULE_notifyDeclaration = 24, RULE_intercept = 25, RULE_breakpoint = 26,
RULE_breakDeclaration = 27, RULE_continueDeclaration = 28, RULE_skipDeclaration = 29;
public static final String[] ruleNames = {
"file", "scriptFile", "projectFile", "importDeclaration", "project", "orientation",
"include", "install", "uninstall", "process", "chain", "ifDeclaration",
"then", "elseDeclaration", "whileDeclaration", "mplCommand", "modifierList",
"modus", "conditional", "auto", "command", "start", "stop", "waitfor",
"notifyDeclaration", "intercept", "breakpoint", "breakDeclaration", "continueDeclaration",
"skipDeclaration"
};
private static final String[] _LITERAL_NAMES = {
null, "'{'", "'}'", "':'", "','", null, null, "'import'", "'project'",
"'include'", "'orientation'", "'install'", "'uninstall'", "'process'",
"'impulse'", "'chain'", "'repeat'", "'unconditional'", "'conditional'",
"'invert'", "'always active'", "'needs redstone'", "'start'", "'stop'",
"'waitfor'", "'notify'", "'intercept'", "'breakpoint'", "'skip'", "'if'",
"'not'", "'then'", "'else'", "'do'", "'while'", "'break'", "'continue'"
};
private static final String[] _SYMBOLIC_NAMES = {
null, null, null, null, null, "COMMENT", "COMMAND", "IMPORT", "PROJECT",
"INCLUDE", "ORIENTATION", "INSTALL", "UNINSTALL", "PROCESS", "IMPULSE",
"CHAIN", "REPEAT", "UNCONDITIONAL", "CONDITIONAL", "INVERT", "ALWAYS_ACTIVE",
"NEEDS_REDSTONE", "START", "STOP", "WAITFOR", "NOTIFY", "INTERCEPT", "BREAKPOINT",
"SKIP_TOKEN", "IF", "NOT", "THEN", "ELSE", "DO", "WHILE", "BREAK", "CONTINUE",
"UNSIGNED_INT", "WS", "STRING", "IDENTIFIER", "UNRECOGNIZED"
};
public static final Vocabulary VOCABULARY = new VocabularyImpl(_LITERAL_NAMES, _SYMBOLIC_NAMES);
/**
* @deprecated Use {@link #VOCABULARY} instead.
*/
@Deprecated
public static final String[] tokenNames;
static {
tokenNames = new String[_SYMBOLIC_NAMES.length];
for (int i = 0; i < tokenNames.length; i++) {
tokenNames[i] = VOCABULARY.getLiteralName(i);
if (tokenNames[i] == null) {
tokenNames[i] = VOCABULARY.getSymbolicName(i);
}
if (tokenNames[i] == null) {
tokenNames[i] = "";
}
}
}
@Override
@Deprecated
public String[] getTokenNames() {
return tokenNames;
}
@Override
public Vocabulary getVocabulary() {
return VOCABULARY;
}
@Override
public String getGrammarFileName() { return "Mpl.g4"; }
@Override
public String[] getRuleNames() { return ruleNames; }
@Override
public String getSerializedATN() { return _serializedATN; }
@Override
public ATN getATN() { return _ATN; }
public MplParser(TokenStream input) {
super(input);
_interp = new ParserATNSimulator(this,_ATN,_decisionToDFA,_sharedContextCache);
}
public static class FileContext extends ParserRuleContext {
public TerminalNode EOF() { return getToken(MplParser.EOF, 0); }
public ScriptFileContext scriptFile() {
return getRuleContext(ScriptFileContext.class,0);
}
public ProjectFileContext projectFile() {
return getRuleContext(ProjectFileContext.class,0);
}
public FileContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_file; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).enterFile(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).exitFile(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof MplVisitor ) return ((MplVisitor extends T>)visitor).visitFile(this);
else return visitor.visitChildren(this);
}
}
public final FileContext file() throws RecognitionException {
FileContext _localctx = new FileContext(_ctx, getState());
enterRule(_localctx, 0, RULE_file);
try {
enterOuterAlt(_localctx, 1);
{
setState(62);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,0,_ctx) ) {
case 1:
{
setState(60);
scriptFile();
}
break;
case 2:
{
setState(61);
projectFile();
}
break;
}
setState(64);
match(EOF);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ScriptFileContext extends ParserRuleContext {
public List orientation() {
return getRuleContexts(OrientationContext.class);
}
public OrientationContext orientation(int i) {
return getRuleContext(OrientationContext.class,i);
}
public List install() {
return getRuleContexts(InstallContext.class);
}
public InstallContext install(int i) {
return getRuleContext(InstallContext.class,i);
}
public List uninstall() {
return getRuleContexts(UninstallContext.class);
}
public UninstallContext uninstall(int i) {
return getRuleContext(UninstallContext.class,i);
}
public List chain() {
return getRuleContexts(ChainContext.class);
}
public ChainContext chain(int i) {
return getRuleContext(ChainContext.class,i);
}
public ScriptFileContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_scriptFile; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).enterScriptFile(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).exitScriptFile(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof MplVisitor ) return ((MplVisitor extends T>)visitor).visitScriptFile(this);
else return visitor.visitChildren(this);
}
}
public final ScriptFileContext scriptFile() throws RecognitionException {
ScriptFileContext _localctx = new ScriptFileContext(_ctx, getState());
enterRule(_localctx, 2, RULE_scriptFile);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(72);
_errHandler.sync(this);
_la = _input.LA(1);
while ((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << COMMAND) | (1L << ORIENTATION) | (1L << INSTALL) | (1L << UNINSTALL) | (1L << IMPULSE) | (1L << CHAIN) | (1L << REPEAT) | (1L << UNCONDITIONAL) | (1L << CONDITIONAL) | (1L << INVERT) | (1L << ALWAYS_ACTIVE) | (1L << NEEDS_REDSTONE) | (1L << START) | (1L << STOP) | (1L << WAITFOR) | (1L << NOTIFY) | (1L << INTERCEPT) | (1L << BREAKPOINT) | (1L << SKIP_TOKEN) | (1L << IF) | (1L << WHILE) | (1L << BREAK) | (1L << CONTINUE) | (1L << IDENTIFIER))) != 0)) {
{
setState(70);
switch (_input.LA(1)) {
case ORIENTATION:
{
setState(66);
orientation();
}
break;
case INSTALL:
{
setState(67);
install();
}
break;
case UNINSTALL:
{
setState(68);
uninstall();
}
break;
case COMMAND:
case IMPULSE:
case CHAIN:
case REPEAT:
case UNCONDITIONAL:
case CONDITIONAL:
case INVERT:
case ALWAYS_ACTIVE:
case NEEDS_REDSTONE:
case START:
case STOP:
case WAITFOR:
case NOTIFY:
case INTERCEPT:
case BREAKPOINT:
case SKIP_TOKEN:
case IF:
case WHILE:
case BREAK:
case CONTINUE:
case IDENTIFIER:
{
setState(69);
chain();
}
break;
default:
throw new NoViableAltException(this);
}
}
setState(74);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ProjectFileContext extends ParserRuleContext {
public List importDeclaration() {
return getRuleContexts(ImportDeclarationContext.class);
}
public ImportDeclarationContext importDeclaration(int i) {
return getRuleContext(ImportDeclarationContext.class,i);
}
public List project() {
return getRuleContexts(ProjectContext.class);
}
public ProjectContext project(int i) {
return getRuleContext(ProjectContext.class,i);
}
public List install() {
return getRuleContexts(InstallContext.class);
}
public InstallContext install(int i) {
return getRuleContext(InstallContext.class,i);
}
public List uninstall() {
return getRuleContexts(UninstallContext.class);
}
public UninstallContext uninstall(int i) {
return getRuleContext(UninstallContext.class,i);
}
public List process() {
return getRuleContexts(ProcessContext.class);
}
public ProcessContext process(int i) {
return getRuleContext(ProcessContext.class,i);
}
public ProjectFileContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_projectFile; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).enterProjectFile(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).exitProjectFile(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof MplVisitor ) return ((MplVisitor extends T>)visitor).visitProjectFile(this);
else return visitor.visitChildren(this);
}
}
public final ProjectFileContext projectFile() throws RecognitionException {
ProjectFileContext _localctx = new ProjectFileContext(_ctx, getState());
enterRule(_localctx, 4, RULE_projectFile);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(78);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==IMPORT) {
{
{
setState(75);
importDeclaration();
}
}
setState(80);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(87);
_errHandler.sync(this);
_la = _input.LA(1);
while ((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << PROJECT) | (1L << INSTALL) | (1L << UNINSTALL) | (1L << PROCESS) | (1L << IMPULSE) | (1L << REPEAT))) != 0)) {
{
setState(85);
switch (_input.LA(1)) {
case PROJECT:
{
setState(81);
project();
}
break;
case INSTALL:
{
setState(82);
install();
}
break;
case UNINSTALL:
{
setState(83);
uninstall();
}
break;
case PROCESS:
case IMPULSE:
case REPEAT:
{
setState(84);
process();
}
break;
default:
throw new NoViableAltException(this);
}
}
setState(89);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ImportDeclarationContext extends ParserRuleContext {
public TerminalNode IMPORT() { return getToken(MplParser.IMPORT, 0); }
public TerminalNode STRING() { return getToken(MplParser.STRING, 0); }
public ImportDeclarationContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_importDeclaration; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).enterImportDeclaration(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).exitImportDeclaration(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof MplVisitor ) return ((MplVisitor extends T>)visitor).visitImportDeclaration(this);
else return visitor.visitChildren(this);
}
}
public final ImportDeclarationContext importDeclaration() throws RecognitionException {
ImportDeclarationContext _localctx = new ImportDeclarationContext(_ctx, getState());
enterRule(_localctx, 6, RULE_importDeclaration);
try {
enterOuterAlt(_localctx, 1);
{
setState(90);
match(IMPORT);
setState(91);
match(STRING);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ProjectContext extends ParserRuleContext {
public TerminalNode PROJECT() { return getToken(MplParser.PROJECT, 0); }
public TerminalNode IDENTIFIER() { return getToken(MplParser.IDENTIFIER, 0); }
public List orientation() {
return getRuleContexts(OrientationContext.class);
}
public OrientationContext orientation(int i) {
return getRuleContext(OrientationContext.class,i);
}
public List include() {
return getRuleContexts(IncludeContext.class);
}
public IncludeContext include(int i) {
return getRuleContext(IncludeContext.class,i);
}
public ProjectContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_project; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).enterProject(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).exitProject(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof MplVisitor ) return ((MplVisitor extends T>)visitor).visitProject(this);
else return visitor.visitChildren(this);
}
}
public final ProjectContext project() throws RecognitionException {
ProjectContext _localctx = new ProjectContext(_ctx, getState());
enterRule(_localctx, 8, RULE_project);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(93);
match(PROJECT);
setState(94);
match(IDENTIFIER);
setState(95);
match(T__0);
setState(100);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==INCLUDE || _la==ORIENTATION) {
{
setState(98);
switch (_input.LA(1)) {
case ORIENTATION:
{
setState(96);
orientation();
}
break;
case INCLUDE:
{
setState(97);
include();
}
break;
default:
throw new NoViableAltException(this);
}
}
setState(102);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(103);
match(T__1);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class OrientationContext extends ParserRuleContext {
public TerminalNode ORIENTATION() { return getToken(MplParser.ORIENTATION, 0); }
public TerminalNode STRING() { return getToken(MplParser.STRING, 0); }
public OrientationContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_orientation; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).enterOrientation(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).exitOrientation(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof MplVisitor ) return ((MplVisitor extends T>)visitor).visitOrientation(this);
else return visitor.visitChildren(this);
}
}
public final OrientationContext orientation() throws RecognitionException {
OrientationContext _localctx = new OrientationContext(_ctx, getState());
enterRule(_localctx, 10, RULE_orientation);
try {
enterOuterAlt(_localctx, 1);
{
setState(105);
match(ORIENTATION);
setState(106);
match(STRING);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class IncludeContext extends ParserRuleContext {
public TerminalNode INCLUDE() { return getToken(MplParser.INCLUDE, 0); }
public TerminalNode STRING() { return getToken(MplParser.STRING, 0); }
public IncludeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_include; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).enterInclude(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).exitInclude(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof MplVisitor ) return ((MplVisitor extends T>)visitor).visitInclude(this);
else return visitor.visitChildren(this);
}
}
public final IncludeContext include() throws RecognitionException {
IncludeContext _localctx = new IncludeContext(_ctx, getState());
enterRule(_localctx, 12, RULE_include);
try {
enterOuterAlt(_localctx, 1);
{
setState(108);
match(INCLUDE);
setState(109);
match(STRING);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class InstallContext extends ParserRuleContext {
public TerminalNode INSTALL() { return getToken(MplParser.INSTALL, 0); }
public ChainContext chain() {
return getRuleContext(ChainContext.class,0);
}
public InstallContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_install; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).enterInstall(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).exitInstall(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof MplVisitor ) return ((MplVisitor extends T>)visitor).visitInstall(this);
else return visitor.visitChildren(this);
}
}
public final InstallContext install() throws RecognitionException {
InstallContext _localctx = new InstallContext(_ctx, getState());
enterRule(_localctx, 14, RULE_install);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(111);
match(INSTALL);
setState(112);
match(T__0);
setState(114);
_la = _input.LA(1);
if ((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << COMMAND) | (1L << IMPULSE) | (1L << CHAIN) | (1L << REPEAT) | (1L << UNCONDITIONAL) | (1L << CONDITIONAL) | (1L << INVERT) | (1L << ALWAYS_ACTIVE) | (1L << NEEDS_REDSTONE) | (1L << START) | (1L << STOP) | (1L << WAITFOR) | (1L << NOTIFY) | (1L << INTERCEPT) | (1L << BREAKPOINT) | (1L << SKIP_TOKEN) | (1L << IF) | (1L << WHILE) | (1L << BREAK) | (1L << CONTINUE) | (1L << IDENTIFIER))) != 0)) {
{
setState(113);
chain();
}
}
setState(116);
match(T__1);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class UninstallContext extends ParserRuleContext {
public TerminalNode UNINSTALL() { return getToken(MplParser.UNINSTALL, 0); }
public ChainContext chain() {
return getRuleContext(ChainContext.class,0);
}
public UninstallContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_uninstall; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).enterUninstall(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).exitUninstall(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof MplVisitor ) return ((MplVisitor extends T>)visitor).visitUninstall(this);
else return visitor.visitChildren(this);
}
}
public final UninstallContext uninstall() throws RecognitionException {
UninstallContext _localctx = new UninstallContext(_ctx, getState());
enterRule(_localctx, 16, RULE_uninstall);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(118);
match(UNINSTALL);
setState(119);
match(T__0);
setState(121);
_la = _input.LA(1);
if ((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << COMMAND) | (1L << IMPULSE) | (1L << CHAIN) | (1L << REPEAT) | (1L << UNCONDITIONAL) | (1L << CONDITIONAL) | (1L << INVERT) | (1L << ALWAYS_ACTIVE) | (1L << NEEDS_REDSTONE) | (1L << START) | (1L << STOP) | (1L << WAITFOR) | (1L << NOTIFY) | (1L << INTERCEPT) | (1L << BREAKPOINT) | (1L << SKIP_TOKEN) | (1L << IF) | (1L << WHILE) | (1L << BREAK) | (1L << CONTINUE) | (1L << IDENTIFIER))) != 0)) {
{
setState(120);
chain();
}
}
setState(123);
match(T__1);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ProcessContext extends ParserRuleContext {
public TerminalNode PROCESS() { return getToken(MplParser.PROCESS, 0); }
public TerminalNode IDENTIFIER() { return getToken(MplParser.IDENTIFIER, 0); }
public ChainContext chain() {
return getRuleContext(ChainContext.class,0);
}
public TerminalNode IMPULSE() { return getToken(MplParser.IMPULSE, 0); }
public TerminalNode REPEAT() { return getToken(MplParser.REPEAT, 0); }
public ProcessContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_process; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).enterProcess(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).exitProcess(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof MplVisitor ) return ((MplVisitor extends T>)visitor).visitProcess(this);
else return visitor.visitChildren(this);
}
}
public final ProcessContext process() throws RecognitionException {
ProcessContext _localctx = new ProcessContext(_ctx, getState());
enterRule(_localctx, 18, RULE_process);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(126);
_la = _input.LA(1);
if (_la==IMPULSE || _la==REPEAT) {
{
setState(125);
_la = _input.LA(1);
if ( !(_la==IMPULSE || _la==REPEAT) ) {
_errHandler.recoverInline(this);
} else {
consume();
}
}
}
setState(128);
match(PROCESS);
setState(129);
match(IDENTIFIER);
setState(130);
match(T__0);
setState(132);
_la = _input.LA(1);
if ((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << COMMAND) | (1L << IMPULSE) | (1L << CHAIN) | (1L << REPEAT) | (1L << UNCONDITIONAL) | (1L << CONDITIONAL) | (1L << INVERT) | (1L << ALWAYS_ACTIVE) | (1L << NEEDS_REDSTONE) | (1L << START) | (1L << STOP) | (1L << WAITFOR) | (1L << NOTIFY) | (1L << INTERCEPT) | (1L << BREAKPOINT) | (1L << SKIP_TOKEN) | (1L << IF) | (1L << WHILE) | (1L << BREAK) | (1L << CONTINUE) | (1L << IDENTIFIER))) != 0)) {
{
setState(131);
chain();
}
}
setState(134);
match(T__1);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ChainContext extends ParserRuleContext {
public List ifDeclaration() {
return getRuleContexts(IfDeclarationContext.class);
}
public IfDeclarationContext ifDeclaration(int i) {
return getRuleContext(IfDeclarationContext.class,i);
}
public List whileDeclaration() {
return getRuleContexts(WhileDeclarationContext.class);
}
public WhileDeclarationContext whileDeclaration(int i) {
return getRuleContext(WhileDeclarationContext.class,i);
}
public List mplCommand() {
return getRuleContexts(MplCommandContext.class);
}
public MplCommandContext mplCommand(int i) {
return getRuleContext(MplCommandContext.class,i);
}
public List skipDeclaration() {
return getRuleContexts(SkipDeclarationContext.class);
}
public SkipDeclarationContext skipDeclaration(int i) {
return getRuleContext(SkipDeclarationContext.class,i);
}
public ChainContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_chain; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).enterChain(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).exitChain(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof MplVisitor ) return ((MplVisitor extends T>)visitor).visitChain(this);
else return visitor.visitChildren(this);
}
}
public final ChainContext chain() throws RecognitionException {
ChainContext _localctx = new ChainContext(_ctx, getState());
enterRule(_localctx, 20, RULE_chain);
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(140);
_errHandler.sync(this);
_alt = 1;
do {
switch (_alt) {
case 1:
{
setState(140);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,12,_ctx) ) {
case 1:
{
setState(136);
ifDeclaration();
}
break;
case 2:
{
setState(137);
whileDeclaration();
}
break;
case 3:
{
setState(138);
mplCommand();
}
break;
case 4:
{
setState(139);
skipDeclaration();
}
break;
}
}
break;
default:
throw new NoViableAltException(this);
}
setState(142);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,13,_ctx);
} while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER );
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class IfDeclarationContext extends ParserRuleContext {
public TerminalNode IF() { return getToken(MplParser.IF, 0); }
public TerminalNode COMMAND() { return getToken(MplParser.COMMAND, 0); }
public TerminalNode NOT() { return getToken(MplParser.NOT, 0); }
public ThenContext then() {
return getRuleContext(ThenContext.class,0);
}
public ElseDeclarationContext elseDeclaration() {
return getRuleContext(ElseDeclarationContext.class,0);
}
public IfDeclarationContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_ifDeclaration; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).enterIfDeclaration(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).exitIfDeclaration(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof MplVisitor ) return ((MplVisitor extends T>)visitor).visitIfDeclaration(this);
else return visitor.visitChildren(this);
}
}
public final IfDeclarationContext ifDeclaration() throws RecognitionException {
IfDeclarationContext _localctx = new IfDeclarationContext(_ctx, getState());
enterRule(_localctx, 22, RULE_ifDeclaration);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(144);
match(IF);
setState(146);
_la = _input.LA(1);
if (_la==NOT) {
{
setState(145);
match(NOT);
}
}
setState(148);
match(T__2);
setState(149);
match(COMMAND);
setState(151);
_la = _input.LA(1);
if (_la==THEN) {
{
setState(150);
then();
}
}
setState(154);
_la = _input.LA(1);
if (_la==ELSE) {
{
setState(153);
elseDeclaration();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ThenContext extends ParserRuleContext {
public TerminalNode THEN() { return getToken(MplParser.THEN, 0); }
public ChainContext chain() {
return getRuleContext(ChainContext.class,0);
}
public ThenContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_then; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).enterThen(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).exitThen(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof MplVisitor ) return ((MplVisitor extends T>)visitor).visitThen(this);
else return visitor.visitChildren(this);
}
}
public final ThenContext then() throws RecognitionException {
ThenContext _localctx = new ThenContext(_ctx, getState());
enterRule(_localctx, 24, RULE_then);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(156);
match(THEN);
setState(157);
match(T__0);
setState(159);
_la = _input.LA(1);
if ((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << COMMAND) | (1L << IMPULSE) | (1L << CHAIN) | (1L << REPEAT) | (1L << UNCONDITIONAL) | (1L << CONDITIONAL) | (1L << INVERT) | (1L << ALWAYS_ACTIVE) | (1L << NEEDS_REDSTONE) | (1L << START) | (1L << STOP) | (1L << WAITFOR) | (1L << NOTIFY) | (1L << INTERCEPT) | (1L << BREAKPOINT) | (1L << SKIP_TOKEN) | (1L << IF) | (1L << WHILE) | (1L << BREAK) | (1L << CONTINUE) | (1L << IDENTIFIER))) != 0)) {
{
setState(158);
chain();
}
}
setState(161);
match(T__1);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ElseDeclarationContext extends ParserRuleContext {
public TerminalNode ELSE() { return getToken(MplParser.ELSE, 0); }
public ChainContext chain() {
return getRuleContext(ChainContext.class,0);
}
public ElseDeclarationContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_elseDeclaration; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).enterElseDeclaration(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).exitElseDeclaration(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof MplVisitor ) return ((MplVisitor extends T>)visitor).visitElseDeclaration(this);
else return visitor.visitChildren(this);
}
}
public final ElseDeclarationContext elseDeclaration() throws RecognitionException {
ElseDeclarationContext _localctx = new ElseDeclarationContext(_ctx, getState());
enterRule(_localctx, 26, RULE_elseDeclaration);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(163);
match(ELSE);
setState(164);
match(T__0);
setState(166);
_la = _input.LA(1);
if ((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << COMMAND) | (1L << IMPULSE) | (1L << CHAIN) | (1L << REPEAT) | (1L << UNCONDITIONAL) | (1L << CONDITIONAL) | (1L << INVERT) | (1L << ALWAYS_ACTIVE) | (1L << NEEDS_REDSTONE) | (1L << START) | (1L << STOP) | (1L << WAITFOR) | (1L << NOTIFY) | (1L << INTERCEPT) | (1L << BREAKPOINT) | (1L << SKIP_TOKEN) | (1L << IF) | (1L << WHILE) | (1L << BREAK) | (1L << CONTINUE) | (1L << IDENTIFIER))) != 0)) {
{
setState(165);
chain();
}
}
setState(168);
match(T__1);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class WhileDeclarationContext extends ParserRuleContext {
public TerminalNode WHILE() { return getToken(MplParser.WHILE, 0); }
public TerminalNode COMMAND() { return getToken(MplParser.COMMAND, 0); }
public TerminalNode REPEAT() { return getToken(MplParser.REPEAT, 0); }
public TerminalNode IDENTIFIER() { return getToken(MplParser.IDENTIFIER, 0); }
public TerminalNode NOT() { return getToken(MplParser.NOT, 0); }
public ChainContext chain() {
return getRuleContext(ChainContext.class,0);
}
public TerminalNode DO() { return getToken(MplParser.DO, 0); }
public WhileDeclarationContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_whileDeclaration; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).enterWhileDeclaration(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).exitWhileDeclaration(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof MplVisitor ) return ((MplVisitor extends T>)visitor).visitWhileDeclaration(this);
else return visitor.visitChildren(this);
}
}
public final WhileDeclarationContext whileDeclaration() throws RecognitionException {
WhileDeclarationContext _localctx = new WhileDeclarationContext(_ctx, getState());
enterRule(_localctx, 28, RULE_whileDeclaration);
int _la;
try {
setState(205);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,26,_ctx) ) {
case 1:
enterOuterAlt(_localctx, 1);
{
setState(172);
_la = _input.LA(1);
if (_la==IDENTIFIER) {
{
setState(170);
match(IDENTIFIER);
setState(171);
match(T__2);
}
}
setState(174);
match(WHILE);
setState(176);
_la = _input.LA(1);
if (_la==NOT) {
{
setState(175);
match(NOT);
}
}
setState(178);
match(T__2);
setState(179);
match(COMMAND);
setState(180);
match(REPEAT);
setState(181);
match(T__0);
setState(183);
_la = _input.LA(1);
if ((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << COMMAND) | (1L << IMPULSE) | (1L << CHAIN) | (1L << REPEAT) | (1L << UNCONDITIONAL) | (1L << CONDITIONAL) | (1L << INVERT) | (1L << ALWAYS_ACTIVE) | (1L << NEEDS_REDSTONE) | (1L << START) | (1L << STOP) | (1L << WAITFOR) | (1L << NOTIFY) | (1L << INTERCEPT) | (1L << BREAKPOINT) | (1L << SKIP_TOKEN) | (1L << IF) | (1L << WHILE) | (1L << BREAK) | (1L << CONTINUE) | (1L << IDENTIFIER))) != 0)) {
{
setState(182);
chain();
}
}
setState(185);
match(T__1);
}
break;
case 2:
enterOuterAlt(_localctx, 2);
{
setState(188);
_la = _input.LA(1);
if (_la==IDENTIFIER) {
{
setState(186);
match(IDENTIFIER);
setState(187);
match(T__2);
}
}
setState(190);
match(REPEAT);
setState(191);
match(T__0);
setState(193);
_la = _input.LA(1);
if ((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << COMMAND) | (1L << IMPULSE) | (1L << CHAIN) | (1L << REPEAT) | (1L << UNCONDITIONAL) | (1L << CONDITIONAL) | (1L << INVERT) | (1L << ALWAYS_ACTIVE) | (1L << NEEDS_REDSTONE) | (1L << START) | (1L << STOP) | (1L << WAITFOR) | (1L << NOTIFY) | (1L << INTERCEPT) | (1L << BREAKPOINT) | (1L << SKIP_TOKEN) | (1L << IF) | (1L << WHILE) | (1L << BREAK) | (1L << CONTINUE) | (1L << IDENTIFIER))) != 0)) {
{
setState(192);
chain();
}
}
setState(195);
match(T__1);
setState(203);
_la = _input.LA(1);
if (_la==DO) {
{
setState(196);
match(DO);
setState(197);
match(WHILE);
setState(199);
_la = _input.LA(1);
if (_la==NOT) {
{
setState(198);
match(NOT);
}
}
setState(201);
match(T__2);
setState(202);
match(COMMAND);
}
}
}
break;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class MplCommandContext extends ParserRuleContext {
public CommandContext command() {
return getRuleContext(CommandContext.class,0);
}
public StartContext start() {
return getRuleContext(StartContext.class,0);
}
public StopContext stop() {
return getRuleContext(StopContext.class,0);
}
public WaitforContext waitfor() {
return getRuleContext(WaitforContext.class,0);
}
public NotifyDeclarationContext notifyDeclaration() {
return getRuleContext(NotifyDeclarationContext.class,0);
}
public InterceptContext intercept() {
return getRuleContext(InterceptContext.class,0);
}
public BreakpointContext breakpoint() {
return getRuleContext(BreakpointContext.class,0);
}
public BreakDeclarationContext breakDeclaration() {
return getRuleContext(BreakDeclarationContext.class,0);
}
public ContinueDeclarationContext continueDeclaration() {
return getRuleContext(ContinueDeclarationContext.class,0);
}
public ModifierListContext modifierList() {
return getRuleContext(ModifierListContext.class,0);
}
public MplCommandContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_mplCommand; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).enterMplCommand(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).exitMplCommand(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof MplVisitor ) return ((MplVisitor extends T>)visitor).visitMplCommand(this);
else return visitor.visitChildren(this);
}
}
public final MplCommandContext mplCommand() throws RecognitionException {
MplCommandContext _localctx = new MplCommandContext(_ctx, getState());
enterRule(_localctx, 30, RULE_mplCommand);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(208);
_la = _input.LA(1);
if ((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << IMPULSE) | (1L << CHAIN) | (1L << REPEAT) | (1L << UNCONDITIONAL) | (1L << CONDITIONAL) | (1L << INVERT) | (1L << ALWAYS_ACTIVE) | (1L << NEEDS_REDSTONE))) != 0)) {
{
setState(207);
modifierList();
}
}
setState(219);
switch (_input.LA(1)) {
case COMMAND:
{
setState(210);
command();
}
break;
case START:
{
setState(211);
start();
}
break;
case STOP:
{
setState(212);
stop();
}
break;
case WAITFOR:
{
setState(213);
waitfor();
}
break;
case NOTIFY:
{
setState(214);
notifyDeclaration();
}
break;
case INTERCEPT:
{
setState(215);
intercept();
}
break;
case BREAKPOINT:
{
setState(216);
breakpoint();
}
break;
case BREAK:
{
setState(217);
breakDeclaration();
}
break;
case CONTINUE:
{
setState(218);
continueDeclaration();
}
break;
default:
throw new NoViableAltException(this);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ModifierListContext extends ParserRuleContext {
public ModusContext modus() {
return getRuleContext(ModusContext.class,0);
}
public ConditionalContext conditional() {
return getRuleContext(ConditionalContext.class,0);
}
public AutoContext auto() {
return getRuleContext(AutoContext.class,0);
}
public ModifierListContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_modifierList; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).enterModifierList(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).exitModifierList(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof MplVisitor ) return ((MplVisitor extends T>)visitor).visitModifierList(this);
else return visitor.visitChildren(this);
}
}
public final ModifierListContext modifierList() throws RecognitionException {
ModifierListContext _localctx = new ModifierListContext(_ctx, getState());
enterRule(_localctx, 32, RULE_modifierList);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(236);
switch (_input.LA(1)) {
case IMPULSE:
case CHAIN:
case REPEAT:
{
setState(221);
modus();
setState(224);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,29,_ctx) ) {
case 1:
{
setState(222);
match(T__3);
setState(223);
conditional();
}
break;
}
setState(228);
_la = _input.LA(1);
if (_la==T__3) {
{
setState(226);
match(T__3);
setState(227);
auto();
}
}
}
break;
case UNCONDITIONAL:
case CONDITIONAL:
case INVERT:
{
setState(230);
conditional();
setState(233);
_la = _input.LA(1);
if (_la==T__3) {
{
setState(231);
match(T__3);
setState(232);
auto();
}
}
}
break;
case ALWAYS_ACTIVE:
case NEEDS_REDSTONE:
{
setState(235);
auto();
}
break;
default:
throw new NoViableAltException(this);
}
setState(238);
match(T__2);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ModusContext extends ParserRuleContext {
public TerminalNode IMPULSE() { return getToken(MplParser.IMPULSE, 0); }
public TerminalNode CHAIN() { return getToken(MplParser.CHAIN, 0); }
public TerminalNode REPEAT() { return getToken(MplParser.REPEAT, 0); }
public ModusContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_modus; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).enterModus(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).exitModus(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof MplVisitor ) return ((MplVisitor extends T>)visitor).visitModus(this);
else return visitor.visitChildren(this);
}
}
public final ModusContext modus() throws RecognitionException {
ModusContext _localctx = new ModusContext(_ctx, getState());
enterRule(_localctx, 34, RULE_modus);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(240);
_la = _input.LA(1);
if ( !((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << IMPULSE) | (1L << CHAIN) | (1L << REPEAT))) != 0)) ) {
_errHandler.recoverInline(this);
} else {
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ConditionalContext extends ParserRuleContext {
public TerminalNode UNCONDITIONAL() { return getToken(MplParser.UNCONDITIONAL, 0); }
public TerminalNode CONDITIONAL() { return getToken(MplParser.CONDITIONAL, 0); }
public TerminalNode INVERT() { return getToken(MplParser.INVERT, 0); }
public ConditionalContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_conditional; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).enterConditional(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).exitConditional(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof MplVisitor ) return ((MplVisitor extends T>)visitor).visitConditional(this);
else return visitor.visitChildren(this);
}
}
public final ConditionalContext conditional() throws RecognitionException {
ConditionalContext _localctx = new ConditionalContext(_ctx, getState());
enterRule(_localctx, 36, RULE_conditional);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(242);
_la = _input.LA(1);
if ( !((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << UNCONDITIONAL) | (1L << CONDITIONAL) | (1L << INVERT))) != 0)) ) {
_errHandler.recoverInline(this);
} else {
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class AutoContext extends ParserRuleContext {
public TerminalNode NEEDS_REDSTONE() { return getToken(MplParser.NEEDS_REDSTONE, 0); }
public TerminalNode ALWAYS_ACTIVE() { return getToken(MplParser.ALWAYS_ACTIVE, 0); }
public AutoContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_auto; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).enterAuto(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).exitAuto(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof MplVisitor ) return ((MplVisitor extends T>)visitor).visitAuto(this);
else return visitor.visitChildren(this);
}
}
public final AutoContext auto() throws RecognitionException {
AutoContext _localctx = new AutoContext(_ctx, getState());
enterRule(_localctx, 38, RULE_auto);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(244);
_la = _input.LA(1);
if ( !(_la==ALWAYS_ACTIVE || _la==NEEDS_REDSTONE) ) {
_errHandler.recoverInline(this);
} else {
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class CommandContext extends ParserRuleContext {
public TerminalNode COMMAND() { return getToken(MplParser.COMMAND, 0); }
public CommandContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_command; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).enterCommand(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).exitCommand(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof MplVisitor ) return ((MplVisitor extends T>)visitor).visitCommand(this);
else return visitor.visitChildren(this);
}
}
public final CommandContext command() throws RecognitionException {
CommandContext _localctx = new CommandContext(_ctx, getState());
enterRule(_localctx, 40, RULE_command);
try {
enterOuterAlt(_localctx, 1);
{
setState(246);
match(COMMAND);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class StartContext extends ParserRuleContext {
public TerminalNode START() { return getToken(MplParser.START, 0); }
public TerminalNode IDENTIFIER() { return getToken(MplParser.IDENTIFIER, 0); }
public StartContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_start; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).enterStart(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).exitStart(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof MplVisitor ) return ((MplVisitor extends T>)visitor).visitStart(this);
else return visitor.visitChildren(this);
}
}
public final StartContext start() throws RecognitionException {
StartContext _localctx = new StartContext(_ctx, getState());
enterRule(_localctx, 42, RULE_start);
try {
enterOuterAlt(_localctx, 1);
{
setState(248);
match(START);
setState(249);
match(IDENTIFIER);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class StopContext extends ParserRuleContext {
public TerminalNode STOP() { return getToken(MplParser.STOP, 0); }
public TerminalNode IDENTIFIER() { return getToken(MplParser.IDENTIFIER, 0); }
public StopContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_stop; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).enterStop(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).exitStop(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof MplVisitor ) return ((MplVisitor extends T>)visitor).visitStop(this);
else return visitor.visitChildren(this);
}
}
public final StopContext stop() throws RecognitionException {
StopContext _localctx = new StopContext(_ctx, getState());
enterRule(_localctx, 44, RULE_stop);
try {
enterOuterAlt(_localctx, 1);
{
setState(251);
match(STOP);
setState(253);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,33,_ctx) ) {
case 1:
{
setState(252);
match(IDENTIFIER);
}
break;
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class WaitforContext extends ParserRuleContext {
public TerminalNode WAITFOR() { return getToken(MplParser.WAITFOR, 0); }
public TerminalNode IDENTIFIER() { return getToken(MplParser.IDENTIFIER, 0); }
public TerminalNode NOTIFY() { return getToken(MplParser.NOTIFY, 0); }
public WaitforContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_waitfor; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).enterWaitfor(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).exitWaitfor(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof MplVisitor ) return ((MplVisitor extends T>)visitor).visitWaitfor(this);
else return visitor.visitChildren(this);
}
}
public final WaitforContext waitfor() throws RecognitionException {
WaitforContext _localctx = new WaitforContext(_ctx, getState());
enterRule(_localctx, 46, RULE_waitfor);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(255);
match(WAITFOR);
setState(260);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,35,_ctx) ) {
case 1:
{
setState(257);
_la = _input.LA(1);
if (_la==NOTIFY) {
{
setState(256);
match(NOTIFY);
}
}
setState(259);
match(IDENTIFIER);
}
break;
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class NotifyDeclarationContext extends ParserRuleContext {
public TerminalNode NOTIFY() { return getToken(MplParser.NOTIFY, 0); }
public NotifyDeclarationContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_notifyDeclaration; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).enterNotifyDeclaration(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).exitNotifyDeclaration(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof MplVisitor ) return ((MplVisitor extends T>)visitor).visitNotifyDeclaration(this);
else return visitor.visitChildren(this);
}
}
public final NotifyDeclarationContext notifyDeclaration() throws RecognitionException {
NotifyDeclarationContext _localctx = new NotifyDeclarationContext(_ctx, getState());
enterRule(_localctx, 48, RULE_notifyDeclaration);
try {
enterOuterAlt(_localctx, 1);
{
setState(262);
match(NOTIFY);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class InterceptContext extends ParserRuleContext {
public TerminalNode INTERCEPT() { return getToken(MplParser.INTERCEPT, 0); }
public TerminalNode IDENTIFIER() { return getToken(MplParser.IDENTIFIER, 0); }
public InterceptContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_intercept; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).enterIntercept(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).exitIntercept(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof MplVisitor ) return ((MplVisitor extends T>)visitor).visitIntercept(this);
else return visitor.visitChildren(this);
}
}
public final InterceptContext intercept() throws RecognitionException {
InterceptContext _localctx = new InterceptContext(_ctx, getState());
enterRule(_localctx, 50, RULE_intercept);
try {
enterOuterAlt(_localctx, 1);
{
setState(264);
match(INTERCEPT);
setState(265);
match(IDENTIFIER);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class BreakpointContext extends ParserRuleContext {
public TerminalNode BREAKPOINT() { return getToken(MplParser.BREAKPOINT, 0); }
public BreakpointContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_breakpoint; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).enterBreakpoint(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).exitBreakpoint(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof MplVisitor ) return ((MplVisitor extends T>)visitor).visitBreakpoint(this);
else return visitor.visitChildren(this);
}
}
public final BreakpointContext breakpoint() throws RecognitionException {
BreakpointContext _localctx = new BreakpointContext(_ctx, getState());
enterRule(_localctx, 52, RULE_breakpoint);
try {
enterOuterAlt(_localctx, 1);
{
setState(267);
match(BREAKPOINT);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class BreakDeclarationContext extends ParserRuleContext {
public TerminalNode BREAK() { return getToken(MplParser.BREAK, 0); }
public TerminalNode IDENTIFIER() { return getToken(MplParser.IDENTIFIER, 0); }
public BreakDeclarationContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_breakDeclaration; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).enterBreakDeclaration(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).exitBreakDeclaration(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof MplVisitor ) return ((MplVisitor extends T>)visitor).visitBreakDeclaration(this);
else return visitor.visitChildren(this);
}
}
public final BreakDeclarationContext breakDeclaration() throws RecognitionException {
BreakDeclarationContext _localctx = new BreakDeclarationContext(_ctx, getState());
enterRule(_localctx, 54, RULE_breakDeclaration);
try {
enterOuterAlt(_localctx, 1);
{
setState(269);
match(BREAK);
setState(271);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,36,_ctx) ) {
case 1:
{
setState(270);
match(IDENTIFIER);
}
break;
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ContinueDeclarationContext extends ParserRuleContext {
public TerminalNode CONTINUE() { return getToken(MplParser.CONTINUE, 0); }
public TerminalNode IDENTIFIER() { return getToken(MplParser.IDENTIFIER, 0); }
public ContinueDeclarationContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_continueDeclaration; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).enterContinueDeclaration(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).exitContinueDeclaration(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof MplVisitor ) return ((MplVisitor extends T>)visitor).visitContinueDeclaration(this);
else return visitor.visitChildren(this);
}
}
public final ContinueDeclarationContext continueDeclaration() throws RecognitionException {
ContinueDeclarationContext _localctx = new ContinueDeclarationContext(_ctx, getState());
enterRule(_localctx, 56, RULE_continueDeclaration);
try {
enterOuterAlt(_localctx, 1);
{
setState(273);
match(CONTINUE);
setState(275);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,37,_ctx) ) {
case 1:
{
setState(274);
match(IDENTIFIER);
}
break;
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class SkipDeclarationContext extends ParserRuleContext {
public TerminalNode SKIP_TOKEN() { return getToken(MplParser.SKIP_TOKEN, 0); }
public SkipDeclarationContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_skipDeclaration; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).enterSkipDeclaration(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof MplListener ) ((MplListener)listener).exitSkipDeclaration(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof MplVisitor ) return ((MplVisitor extends T>)visitor).visitSkipDeclaration(this);
else return visitor.visitChildren(this);
}
}
public final SkipDeclarationContext skipDeclaration() throws RecognitionException {
SkipDeclarationContext _localctx = new SkipDeclarationContext(_ctx, getState());
enterRule(_localctx, 58, RULE_skipDeclaration);
try {
enterOuterAlt(_localctx, 1);
{
setState(277);
match(SKIP_TOKEN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static final String _serializedATN =
"\3\u0430\ud6d1\u8206\uad2d\u4417\uaef1\u8d80\uaadd\3+\u011a\4\2\t\2\4"+
"\3\t\3\4\4\t\4\4\5\t\5\4\6\t\6\4\7\t\7\4\b\t\b\4\t\t\t\4\n\t\n\4\13\t"+
"\13\4\f\t\f\4\r\t\r\4\16\t\16\4\17\t\17\4\20\t\20\4\21\t\21\4\22\t\22"+
"\4\23\t\23\4\24\t\24\4\25\t\25\4\26\t\26\4\27\t\27\4\30\t\30\4\31\t\31"+
"\4\32\t\32\4\33\t\33\4\34\t\34\4\35\t\35\4\36\t\36\4\37\t\37\3\2\3\2\5"+
"\2A\n\2\3\2\3\2\3\3\3\3\3\3\3\3\7\3I\n\3\f\3\16\3L\13\3\3\4\7\4O\n\4\f"+
"\4\16\4R\13\4\3\4\3\4\3\4\3\4\7\4X\n\4\f\4\16\4[\13\4\3\5\3\5\3\5\3\6"+
"\3\6\3\6\3\6\3\6\7\6e\n\6\f\6\16\6h\13\6\3\6\3\6\3\7\3\7\3\7\3\b\3\b\3"+
"\b\3\t\3\t\3\t\5\tu\n\t\3\t\3\t\3\n\3\n\3\n\5\n|\n\n\3\n\3\n\3\13\5\13"+
"\u0081\n\13\3\13\3\13\3\13\3\13\5\13\u0087\n\13\3\13\3\13\3\f\3\f\3\f"+
"\3\f\6\f\u008f\n\f\r\f\16\f\u0090\3\r\3\r\5\r\u0095\n\r\3\r\3\r\3\r\5"+
"\r\u009a\n\r\3\r\5\r\u009d\n\r\3\16\3\16\3\16\5\16\u00a2\n\16\3\16\3\16"+
"\3\17\3\17\3\17\5\17\u00a9\n\17\3\17\3\17\3\20\3\20\5\20\u00af\n\20\3"+
"\20\3\20\5\20\u00b3\n\20\3\20\3\20\3\20\3\20\3\20\5\20\u00ba\n\20\3\20"+
"\3\20\3\20\5\20\u00bf\n\20\3\20\3\20\3\20\5\20\u00c4\n\20\3\20\3\20\3"+
"\20\3\20\5\20\u00ca\n\20\3\20\3\20\5\20\u00ce\n\20\5\20\u00d0\n\20\3\21"+
"\5\21\u00d3\n\21\3\21\3\21\3\21\3\21\3\21\3\21\3\21\3\21\3\21\5\21\u00de"+
"\n\21\3\22\3\22\3\22\5\22\u00e3\n\22\3\22\3\22\5\22\u00e7\n\22\3\22\3"+
"\22\3\22\5\22\u00ec\n\22\3\22\5\22\u00ef\n\22\3\22\3\22\3\23\3\23\3\24"+
"\3\24\3\25\3\25\3\26\3\26\3\27\3\27\3\27\3\30\3\30\5\30\u0100\n\30\3\31"+
"\3\31\5\31\u0104\n\31\3\31\5\31\u0107\n\31\3\32\3\32\3\33\3\33\3\33\3"+
"\34\3\34\3\35\3\35\5\35\u0112\n\35\3\36\3\36\5\36\u0116\n\36\3\37\3\37"+
"\3\37\2\2 \2\4\6\b\n\f\16\20\22\24\26\30\32\34\36 \"$&(*,.\60\62\64\66"+
"8:<\2\6\4\2\20\20\22\22\3\2\20\22\3\2\23\25\3\2\26\27\u012f\2@\3\2\2\2"+
"\4J\3\2\2\2\6P\3\2\2\2\b\\\3\2\2\2\n_\3\2\2\2\fk\3\2\2\2\16n\3\2\2\2\20"+
"q\3\2\2\2\22x\3\2\2\2\24\u0080\3\2\2\2\26\u008e\3\2\2\2\30\u0092\3\2\2"+
"\2\32\u009e\3\2\2\2\34\u00a5\3\2\2\2\36\u00cf\3\2\2\2 \u00d2\3\2\2\2\""+
"\u00ee\3\2\2\2$\u00f2\3\2\2\2&\u00f4\3\2\2\2(\u00f6\3\2\2\2*\u00f8\3\2"+
"\2\2,\u00fa\3\2\2\2.\u00fd\3\2\2\2\60\u0101\3\2\2\2\62\u0108\3\2\2\2\64"+
"\u010a\3\2\2\2\66\u010d\3\2\2\28\u010f\3\2\2\2:\u0113\3\2\2\2<\u0117\3"+
"\2\2\2>A\5\4\3\2?A\5\6\4\2@>\3\2\2\2@?\3\2\2\2AB\3\2\2\2BC\7\2\2\3C\3"+
"\3\2\2\2DI\5\f\7\2EI\5\20\t\2FI\5\22\n\2GI\5\26\f\2HD\3\2\2\2HE\3\2\2"+
"\2HF\3\2\2\2HG\3\2\2\2IL\3\2\2\2JH\3\2\2\2JK\3\2\2\2K\5\3\2\2\2LJ\3\2"+
"\2\2MO\5\b\5\2NM\3\2\2\2OR\3\2\2\2PN\3\2\2\2PQ\3\2\2\2QY\3\2\2\2RP\3\2"+
"\2\2SX\5\n\6\2TX\5\20\t\2UX\5\22\n\2VX\5\24\13\2WS\3\2\2\2WT\3\2\2\2W"+
"U\3\2\2\2WV\3\2\2\2X[\3\2\2\2YW\3\2\2\2YZ\3\2\2\2Z\7\3\2\2\2[Y\3\2\2\2"+
"\\]\7\t\2\2]^\7)\2\2^\t\3\2\2\2_`\7\n\2\2`a\7*\2\2af\7\3\2\2be\5\f\7\2"+
"ce\5\16\b\2db\3\2\2\2dc\3\2\2\2eh\3\2\2\2fd\3\2\2\2fg\3\2\2\2gi\3\2\2"+
"\2hf\3\2\2\2ij\7\4\2\2j\13\3\2\2\2kl\7\f\2\2lm\7)\2\2m\r\3\2\2\2no\7\13"+
"\2\2op\7)\2\2p\17\3\2\2\2qr\7\r\2\2rt\7\3\2\2su\5\26\f\2ts\3\2\2\2tu\3"+
"\2\2\2uv\3\2\2\2vw\7\4\2\2w\21\3\2\2\2xy\7\16\2\2y{\7\3\2\2z|\5\26\f\2"+
"{z\3\2\2\2{|\3\2\2\2|}\3\2\2\2}~\7\4\2\2~\23\3\2\2\2\177\u0081\t\2\2\2"+
"\u0080\177\3\2\2\2\u0080\u0081\3\2\2\2\u0081\u0082\3\2\2\2\u0082\u0083"+
"\7\17\2\2\u0083\u0084\7*\2\2\u0084\u0086\7\3\2\2\u0085\u0087\5\26\f\2"+
"\u0086\u0085\3\2\2\2\u0086\u0087\3\2\2\2\u0087\u0088\3\2\2\2\u0088\u0089"+
"\7\4\2\2\u0089\25\3\2\2\2\u008a\u008f\5\30\r\2\u008b\u008f\5\36\20\2\u008c"+
"\u008f\5 \21\2\u008d\u008f\5<\37\2\u008e\u008a\3\2\2\2\u008e\u008b\3\2"+
"\2\2\u008e\u008c\3\2\2\2\u008e\u008d\3\2\2\2\u008f\u0090\3\2\2\2\u0090"+
"\u008e\3\2\2\2\u0090\u0091\3\2\2\2\u0091\27\3\2\2\2\u0092\u0094\7\37\2"+
"\2\u0093\u0095\7 \2\2\u0094\u0093\3\2\2\2\u0094\u0095\3\2\2\2\u0095\u0096"+
"\3\2\2\2\u0096\u0097\7\5\2\2\u0097\u0099\7\b\2\2\u0098\u009a\5\32\16\2"+
"\u0099\u0098\3\2\2\2\u0099\u009a\3\2\2\2\u009a\u009c\3\2\2\2\u009b\u009d"+
"\5\34\17\2\u009c\u009b\3\2\2\2\u009c\u009d\3\2\2\2\u009d\31\3\2\2\2\u009e"+
"\u009f\7!\2\2\u009f\u00a1\7\3\2\2\u00a0\u00a2\5\26\f\2\u00a1\u00a0\3\2"+
"\2\2\u00a1\u00a2\3\2\2\2\u00a2\u00a3\3\2\2\2\u00a3\u00a4\7\4\2\2\u00a4"+
"\33\3\2\2\2\u00a5\u00a6\7\"\2\2\u00a6\u00a8\7\3\2\2\u00a7\u00a9\5\26\f"+
"\2\u00a8\u00a7\3\2\2\2\u00a8\u00a9\3\2\2\2\u00a9\u00aa\3\2\2\2\u00aa\u00ab"+
"\7\4\2\2\u00ab\35\3\2\2\2\u00ac\u00ad\7*\2\2\u00ad\u00af\7\5\2\2\u00ae"+
"\u00ac\3\2\2\2\u00ae\u00af\3\2\2\2\u00af\u00b0\3\2\2\2\u00b0\u00b2\7$"+
"\2\2\u00b1\u00b3\7 \2\2\u00b2\u00b1\3\2\2\2\u00b2\u00b3\3\2\2\2\u00b3"+
"\u00b4\3\2\2\2\u00b4\u00b5\7\5\2\2\u00b5\u00b6\7\b\2\2\u00b6\u00b7\7\22"+
"\2\2\u00b7\u00b9\7\3\2\2\u00b8\u00ba\5\26\f\2\u00b9\u00b8\3\2\2\2\u00b9"+
"\u00ba\3\2\2\2\u00ba\u00bb\3\2\2\2\u00bb\u00d0\7\4\2\2\u00bc\u00bd\7*"+
"\2\2\u00bd\u00bf\7\5\2\2\u00be\u00bc\3\2\2\2\u00be\u00bf\3\2\2\2\u00bf"+
"\u00c0\3\2\2\2\u00c0\u00c1\7\22\2\2\u00c1\u00c3\7\3\2\2\u00c2\u00c4\5"+
"\26\f\2\u00c3\u00c2\3\2\2\2\u00c3\u00c4\3\2\2\2\u00c4\u00c5\3\2\2\2\u00c5"+
"\u00cd\7\4\2\2\u00c6\u00c7\7#\2\2\u00c7\u00c9\7$\2\2\u00c8\u00ca\7 \2"+
"\2\u00c9\u00c8\3\2\2\2\u00c9\u00ca\3\2\2\2\u00ca\u00cb\3\2\2\2\u00cb\u00cc"+
"\7\5\2\2\u00cc\u00ce\7\b\2\2\u00cd\u00c6\3\2\2\2\u00cd\u00ce\3\2\2\2\u00ce"+
"\u00d0\3\2\2\2\u00cf\u00ae\3\2\2\2\u00cf\u00be\3\2\2\2\u00d0\37\3\2\2"+
"\2\u00d1\u00d3\5\"\22\2\u00d2\u00d1\3\2\2\2\u00d2\u00d3\3\2\2\2\u00d3"+
"\u00dd\3\2\2\2\u00d4\u00de\5*\26\2\u00d5\u00de\5,\27\2\u00d6\u00de\5."+
"\30\2\u00d7\u00de\5\60\31\2\u00d8\u00de\5\62\32\2\u00d9\u00de\5\64\33"+
"\2\u00da\u00de\5\66\34\2\u00db\u00de\58\35\2\u00dc\u00de\5:\36\2\u00dd"+
"\u00d4\3\2\2\2\u00dd\u00d5\3\2\2\2\u00dd\u00d6\3\2\2\2\u00dd\u00d7\3\2"+
"\2\2\u00dd\u00d8\3\2\2\2\u00dd\u00d9\3\2\2\2\u00dd\u00da\3\2\2\2\u00dd"+
"\u00db\3\2\2\2\u00dd\u00dc\3\2\2\2\u00de!\3\2\2\2\u00df\u00e2\5$\23\2"+
"\u00e0\u00e1\7\6\2\2\u00e1\u00e3\5&\24\2\u00e2\u00e0\3\2\2\2\u00e2\u00e3"+
"\3\2\2\2\u00e3\u00e6\3\2\2\2\u00e4\u00e5\7\6\2\2\u00e5\u00e7\5(\25\2\u00e6"+
"\u00e4\3\2\2\2\u00e6\u00e7\3\2\2\2\u00e7\u00ef\3\2\2\2\u00e8\u00eb\5&"+
"\24\2\u00e9\u00ea\7\6\2\2\u00ea\u00ec\5(\25\2\u00eb\u00e9\3\2\2\2\u00eb"+
"\u00ec\3\2\2\2\u00ec\u00ef\3\2\2\2\u00ed\u00ef\5(\25\2\u00ee\u00df\3\2"+
"\2\2\u00ee\u00e8\3\2\2\2\u00ee\u00ed\3\2\2\2\u00ef\u00f0\3\2\2\2\u00f0"+
"\u00f1\7\5\2\2\u00f1#\3\2\2\2\u00f2\u00f3\t\3\2\2\u00f3%\3\2\2\2\u00f4"+
"\u00f5\t\4\2\2\u00f5\'\3\2\2\2\u00f6\u00f7\t\5\2\2\u00f7)\3\2\2\2\u00f8"+
"\u00f9\7\b\2\2\u00f9+\3\2\2\2\u00fa\u00fb\7\30\2\2\u00fb\u00fc\7*\2\2"+
"\u00fc-\3\2\2\2\u00fd\u00ff\7\31\2\2\u00fe\u0100\7*\2\2\u00ff\u00fe\3"+
"\2\2\2\u00ff\u0100\3\2\2\2\u0100/\3\2\2\2\u0101\u0106\7\32\2\2\u0102\u0104"+
"\7\33\2\2\u0103\u0102\3\2\2\2\u0103\u0104\3\2\2\2\u0104\u0105\3\2\2\2"+
"\u0105\u0107\7*\2\2\u0106\u0103\3\2\2\2\u0106\u0107\3\2\2\2\u0107\61\3"+
"\2\2\2\u0108\u0109\7\33\2\2\u0109\63\3\2\2\2\u010a\u010b\7\34\2\2\u010b"+
"\u010c\7*\2\2\u010c\65\3\2\2\2\u010d\u010e\7\35\2\2\u010e\67\3\2\2\2\u010f"+
"\u0111\7%\2\2\u0110\u0112\7*\2\2\u0111\u0110\3\2\2\2\u0111\u0112\3\2\2"+
"\2\u01129\3\2\2\2\u0113\u0115\7&\2\2\u0114\u0116\7*\2\2\u0115\u0114\3"+
"\2\2\2\u0115\u0116\3\2\2\2\u0116;\3\2\2\2\u0117\u0118\7\36\2\2\u0118="+
"\3\2\2\2(@HJPWYdft{\u0080\u0086\u008e\u0090\u0094\u0099\u009c\u00a1\u00a8"+
"\u00ae\u00b2\u00b9\u00be\u00c3\u00c9\u00cd\u00cf\u00d2\u00dd\u00e2\u00e6"+
"\u00eb\u00ee\u00ff\u0103\u0106\u0111\u0115";
public static final ATN _ATN =
new ATNDeserializer().deserialize(_serializedATN.toCharArray());
static {
_decisionToDFA = new DFA[_ATN.getNumberOfDecisions()];
for (int i = 0; i < _ATN.getNumberOfDecisions(); i++) {
_decisionToDFA[i] = new DFA(_ATN.getDecisionState(i), i);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy