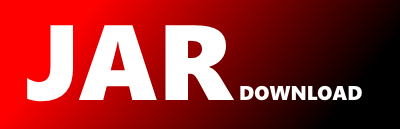
de.akquinet.jbosscc.guttenbase.export.AbstractExportDumpObject Maven / Gradle / Ivy
The newest version!
package de.akquinet.jbosscc.guttenbase.export;
import de.akquinet.jbosscc.guttenbase.connector.GuttenBaseException;
import org.apache.commons.io.IOUtils;
import java.io.*;
/**
* Since CLOBs/BLOBs may be quite big. we do not load them into memory
* completely, but read them in chunks and write the data to the output stream
* in a loop.
*
*
* © 2012-2034 akquinet tech@spree
*
*
* @author M. Dahm
*/
public abstract class AbstractExportDumpObject implements Externalizable {
private static final long serialVersionUID = 1L;
public static final int DEFAULT_BUFFER_SIZE = 1024 * 1024 * 10;
private transient final InputStream _inputStream;
private transient File _tempFile;
private transient FileInputStream _fileInputStream;
public AbstractExportDumpObject() {
this(null);
}
public AbstractExportDumpObject(final InputStream inputStream) {
_inputStream = inputStream;
}
/**
* Read data in chunks and write it to the outputstream to avoid out of memory
* errors.
*/
@Override
public void writeExternal(final ObjectOutput output) throws IOException {
final byte[] buffer = new byte[DEFAULT_BUFFER_SIZE];
for (int n = _inputStream.read(buffer); n > 0; n = _inputStream.read(buffer)) {
byte[] buf = buffer;
if (n < DEFAULT_BUFFER_SIZE) {
buf = new byte[n];
System.arraycopy(buffer, 0, buf, 0, n);
}
output.writeObject(buf);
output.flush();
}
output.writeObject(null);
}
/**
* Store read data in temporary file to avoid out of memory errors.
*/
@Override
public void readExternal(final ObjectInput input) throws IOException, ClassNotFoundException {
if (_tempFile == null) {
_tempFile = File.createTempFile("GB-DUMP-", null);
_tempFile.deleteOnExit();
}
final FileOutputStream fileOutputStream = new FileOutputStream(_tempFile);
for (byte[] buffer = (byte[]) input.readObject(); buffer != null; buffer = (byte[]) input.readObject()) {
fileOutputStream.write(buffer, 0, buffer.length);
}
fileOutputStream.close();
}
public long length() {
return _tempFile.length();
}
public byte[] getBytes(final long pos, final int length) {
try {
final InputStream inputStream = getBinaryStream(pos, length);
final byte[] bytes = new byte[length];
inputStream.read(bytes);
inputStream.close();
return bytes;
} catch (final IOException e) {
throw new GuttenBaseException("getBytes", e);
}
}
public InputStream getBinaryStream() {
return getBinaryStream(0, length());
}
public InputStream getBinaryStream(final long pos, @SuppressWarnings("unused") final long length) {
try {
_fileInputStream = new FileInputStream(_tempFile);
_fileInputStream.skip(pos);
return _fileInputStream;
} catch (final IOException e) {
throw new GuttenBaseException("getBinaryStream", e);
}
}
public final void free() {
if (_tempFile != null && _tempFile.exists()) {
_tempFile.delete();
_tempFile = null;
}
if (_fileInputStream != null) {
IOUtils.closeQuietly(_fileInputStream);
_fileInputStream = null;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy