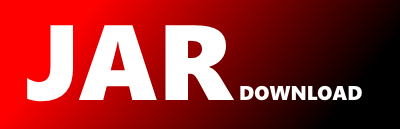
de.albionco.apex.Apex Maven / Gradle / Ivy
The newest version!
/*
* Apex - Advanced permission management plugin for BungeeCord networks
* Copyright (C) 2015 Connor Spencer Harries
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
package de.albionco.apex;
import com.google.common.base.Preconditions;
import de.albionco.apex.api.backend.PermissionManager;
import de.albionco.apex.api.command.CommandManager;
import net.md_5.bungee.api.plugin.Plugin;
import net.md_5.bungee.config.Configuration;
import java.util.logging.Level;
/**
* Created by Connor Harries on 18/01/2015.
*
* @author Connor Spencer Harries
*/
@SuppressWarnings("ALL")
public abstract class Apex extends Plugin {
/**
* Store the active instance of Apex, used in conjunction with {@link #setInstance(Apex)}
* when the actual plugin is enabled
*/
private static Apex instance;
/**
* Get the plugin instance, if this returns null then
* somebody else has tampered with the {@link #instance} field.
*
* @return the active instance of Apex
*/
public static Apex getInstance() {
return Apex.instance;
}
/**
* Set the instance of our plugin so that the API is usable by others.
*
* @param instance implementation of {@link Apex}
*/
protected static void setInstance(Apex instance) {
Preconditions.checkNotNull(instance, "instance");
Apex.instance = instance;
}
/**
* Force the plugin to reload the primary configuration,
* and the active permission manager
*
* @return true if successful
*/
public abstract boolean reload();
/**
* Check if the plugin is running in debug mode,
* if your only use for this is logging messages then
* consider using {@link #debug(Level, String, Object...)} instead as it
* is setup to do this and uses the Apex logger by default.
*
* @return true if debug mode is enabled
*/
public abstract boolean isDebug();
/**
* Check whether Apex is being used for permission
* checking or whether it has been disabled.
*
* @return true if enabled
*/
public abstract boolean isEnabled();
/**
* Set whether Apex should be used for permission checking
*
* @param enabled true if it should be used
*/
public abstract void setEnabled(boolean enabled);
/**
* Get the primary configuration {@link Configuration} that the plugin is using,
* not particularly useful to end users.
*
* @return active {@link Configuration} or null if there was an error loading it
*/
public abstract Configuration getConfig();
/**
* Get the {@link CommandManager} that the plugin is using to
* register and execute commands.
*
* @return a {@link CommandManager} instance or null if one has not been set
*/
public abstract CommandManager getCommandManager();
/**
* Get the {@link PermissionManager} that the plugin is currently using
*
* @return a {@link PermissionManager} instance or null if one has not been set
*/
public abstract PermissionManager getPermissionManager();
/**
* Get the translation of a message
* @param name message to lookup
* @return "translated" string
*/
public abstract String getTranslation(String name, Object... args);
/**
* Log an optionally formatted message at a specific log level,
* only prints to console if the plugin is running in debug mode
*
* @param level level to log at
* @param message message to log, automatically formatted
* @param args optional arguments to use in formatting
*/
public abstract void debug(Level level, String message, Object... args);
/**
* Log a message and exception at a specific log level,
* only prints to console if the plugin is running in debug mode
*
* @param level level to log at
* @param message message to log
* @param ex exception
*/
public abstract void debug(Level level, String message, Exception ex);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy