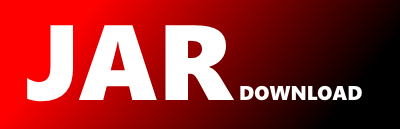
de.alpharogroup.file.zip.ZipModel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of file-worker Show documentation
Show all versions of file-worker Show documentation
Project that holds utility class for file operations.
/**
* The MIT License
*
* Copyright (C) 2007 Asterios Raptis
*
* Permission is hereby granted, free of charge, to any person obtaining
* a copy of this software and associated documentation files (the
* "Software"), to deal in the Software without restriction, including
* without limitation the rights to use, copy, modify, merge, publish,
* distribute, sublicense, and/or sell copies of the Software, and to
* permit persons to whom the Software is furnished to do so, subject to
* the following conditions:
*
* The above copyright notice and this permission notice shall be
* included in all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
*/
package de.alpharogroup.file.zip;
import java.io.File;
import java.io.FilenameFilter;
import java.util.zip.ZipFile;
/**
* The Interface ZipModel.
*
* @version 1.0
* @author Asterios Raptis
*/
public interface ZipModel
{
/**
* Returns the field compressionMethod
.
*
* @return The field compressionMethod
.
*/
public int getCompressionMethod();
/**
* Returns the field directoryToZip
.
*
* @return The field directoryToZip
.
*/
public File getDirectoryToZip();
/**
* Returns the field dirToStart
.
*
* @return The field dirToStart
.
*/
public String getDirToStart();
/**
* Returns the field fileCounter
.
*
* @return The field fileCounter
.
*/
public int getFileCounter();
/**
* Returns the field fileFilter
.
*
* @return The field fileFilter
.
*/
public FilenameFilter getFileFilter();
/**
* Returns the field fileLength
.
*
* @return The field fileLength
.
*/
public long getFileLength();
/**
* Returns the field zipFile
.
*
* @return The field zipFile
.
*/
public File getZipFile();
/**
* Returns the field zipFileComment
.
*
* @return The field zipFileComment
.
*/
public String getZipFileComment();
/**
* Returns the field zipFileName
.
*
* @return The field zipFileName
.
*/
public String getZipFileName();
/**
* Returns the field zipFileObj
.
*
* @return The field zipFileObj
.
*/
public ZipFile getZipFileObj();
/**
* Returns the field zipLevel
.
*
* @return The field zipLevel
.
*/
public int getZipLevel();
/**
* Sets the field compressionMethod
.
*
* @param compressionMethod
* The compressionMethod
to set
*/
public void setCompressionMethod(final int compressionMethod);
/**
* Sets the field directoryToZip
.
*
* @param directoryToZip
* The directoryToZip
to set
*/
public void setDirectoryToZip(final File directoryToZip);
/**
* Sets the field dirToStart
.
*
* @param dirToStart
* The dirToStart
to set
*/
public void setDirToStart(final String dirToStart);
/**
* Sets the field fileCounter
.
*
* @param fileCounter
* The fileCounter
to set
*/
public void setFileCounter(final int fileCounter);
/**
* Sets the field fileFilter
.
*
* @param fileFilter
* The fileFilter
to set
*/
public void setFileFilter(final FilenameFilter fileFilter);
/**
* Sets the field fileLength
.
*
* @param fileLength
* The fileLength
to set
*/
public void setFileLength(final long fileLength);
/**
* Sets the field zipFile
.
*
* @param zipFile
* The zipFile
to set
*/
public void setZipFile(final File zipFile);
/**
* Sets the field zipFileComment
.
*
* @param zipFileComment
* The zipFileComment
to set
*/
public void setZipFileComment(final String zipFileComment);
/**
* Sets the field zipFileName
.
*
* @param zipFileName
* The zipFileName
to set
*/
public void setZipFileName(final String zipFileName);
/**
* Sets the field zipFileObj
.
*
* @param zipFileObj
* The zipFileObj
to set
*/
public void setZipFileObj(final ZipFile zipFileObj);
/**
* Sets the field zipLevel
.
*
* @param zipLevel
* The zipLevel
to set
*/
public void setZipLevel(final int zipLevel);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy