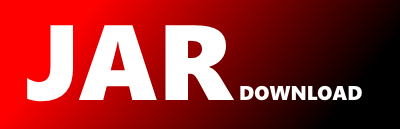
de.alpharogroup.wicket.base.application.OnlineSessionUsers Maven / Gradle / Ivy
/**
* Copyright (C) 2010 Asterios Raptis
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package de.alpharogroup.wicket.base.application;
import java.util.HashMap;
import java.util.Map;
/**
* The Class OnlineSessionUsers holds the mapping between the users that are online and can be
* applied to get the functionality how many users are online. It saves also the session object.
*
* @param
* the generic type for the users object.
* @param
* the generic type for the id the references to the user object.
* @param
* the generic type for the session object.
*/
public class OnlineSessionUsers extends OnlineUsers
{
/** The Constant serialVersionUID. */
private static final long serialVersionUID = 1L;
/** This map holds which session id references to which session. */
private final Map sessionIdToSession = new HashMap<>();
/**
* Adds the user online.
*
* @param user
* the user
* @param sessionId
* the session id
* @param session
* the session object
* @return the session id
*/
public synchronized ID addOnline(final USER user, final ID sessionId, final SESSION session)
{
sessionIdToSession.put(sessionId, session);
return super.addOnline(user, sessionId);
}
/**
* Gets the session from the given user.
*
* @param user
* the user
* @return the session
*/
public synchronized SESSION get(final USER user)
{
return sessionIdToSession.get(getSessionId(user));
}
/**
* Gets the session from the given session id.
*
* @param sessionId
* the session id
* @return the session
*/
public synchronized SESSION getSession(final ID sessionId)
{
return sessionIdToSession.get(sessionId);
}
/**
* Removes the user from the map with the session id.
*
* @param sessionId
* the session id
* @return the user
*/
@Override
public synchronized USER remove(final ID sessionId)
{
sessionIdToSession.remove(sessionId);
return super.remove(sessionId);
}
/**
* Removes the user from the map. This method shell be invoked when the session is unbounded
* from the Application. In wicket is the best way to do that in the
* {@code WebApplication#sessionUnbound(String)}.
*
* @param user
* the user
* @return the session id
*/
@Override
public synchronized ID removeOnline(final USER user)
{
final ID sessionId = super.removeOnline(user);
if (sessionId != null)
{
sessionIdToSession.remove(sessionId);
}
return sessionId;
}
/**
* Replace the given old session id with the new one.
*
* @param user
* the user
* @param oldSessionId
* the old session id
* @param newSessionId
* the new session id
* @param newSession
* the new session object
* @return the new session id that is associated with the given user.
*/
public synchronized ID replaceSessionId(final USER user, final ID oldSessionId,
final ID newSessionId, final SESSION newSession)
{
remove(oldSessionId);
return addOnline(user, newSessionId, newSession);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy