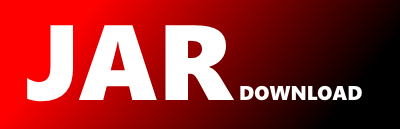
de.audioattack.io.Console Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of console Show documentation
Show all versions of console Show documentation
A replacement for java.io.Console with a System.in and System.out based fallback
/*
* SPDX-FileCopyrightText: 2018 Marc Nause
*
* SPDX-License-Identifier: Apache-2.0
*/
package de.audioattack.io;
import java.io.Flushable;
import java.io.PrintWriter;
import java.io.Reader;
/**
* Mirrors {@link java.io.Console java.io.Console} to allow implementations to work as drop-in replacement for this
* class.
*
* @since 1.0.0
*/
public interface Console extends Flushable {
/**
* Gets the {@link Reader} associated with the console. Invoking {@code close()} on this object will not close the
* underlying stream.
*
* @return the reader
* @see java.io.Console#reader()
*/
Reader reader();
/**
* Gets the {@link PrintWriter} associated with the console. Invoking {@code close()} on this object will not close
* the underlying stream.
*
* @return the writer
* @see java.io.Console#writer()
*/
PrintWriter writer();
/**
* Writes formatted String.
*
* @param fmt format String
* @param args arguments to replace format specifiers in format String
* @return this console
* @see java.io.Console#format(String, Object...)
*/
Console format(String fmt, Object... args);
/**
* Convenience method which writes formatted String.
*
* @param fmt format String
* @param args arguments to replace format specifiers in format String
* @return this console
* @see java.io.Console#printf(String, Object...)
*/
Console printf(String fmt, Object... args);
/**
* Writes formatted prompt and reads single line.
*
* @param fmt format String
* @param args arguments to replace format specifiers in format String
* @return the line
* @see java.io.Console#readLine(String, Object...)
*/
String readLine(String fmt, Object... args);
/**
* Reads single line.
*
* @return the line
* @see java.io.Console#readLine()
*/
String readLine();
/**
* Writes formatted prompt and reads password. Hidden input should be preferred
* if possible. Implementations which cannot hide input should display a warning
* that entered text will be visible.
*
* @param fmt format String
* @param args arguments to replace format specifiers in format String
* @return the password
* @see java.io.Console#readPassword(String, Object...)
*/
char[] readPassword(String fmt, Object... args);
/**
* Reads password. Hidden input should be preferred if possible. Implementations
* which cannot hide input should display a warning that entered text will be
* visible.
*
* @return the password
* @see java.io.Console#readPassword()
*/
char[] readPassword();
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy